#14 What is Data Binding | Angular Components & Directives | A Complete Angular Course
Summary
TLDRThis lecture delves into the concept of data binding in Angular, a vital feature for two-way communication between a component class and its view template. It explains the essence of data binding, highlighting its role in passing data in both directions. The video outlines the types of data binding available in Angular, including one-way binding achieved through string interpolation, property binding, and event binding, as well as two-way binding facilitated by the NG model. The script offers a high-level overview, promising detailed explanations in subsequent lectures, piquing interest in the intricacies of Angular's data binding capabilities.
Takeaways
- 🔗 Data Binding is a feature in Angular that allows communication between a component class and its view template.
- 📚 The two main parts of an Angular component are the component class, which contains the UI logic, and the view template, which contains the HTML rendered in the browser.
- 🔄 Data binding can be unidirectional, from the component class to the view template, or bidirectional, allowing data to flow in both directions.
- 👉 One-way data binding can be achieved using string interpolation or property binding for data flow from component to view template.
- 📌 Property binding is used to assign values from the component class to attributes in the view template, such as the 'hidden' attribute in an HTML element.
- 🔑 Square brackets in Angular are used for property binding, indicating that the value of a property should be assigned to an attribute.
- 📈 Event binding is a form of one-way data binding from the view template to the component class, allowing the component to respond to user actions like button clicks.
- 🔄 Two-way data binding is facilitated by the 'ngModel' directive in Angular, enabling real-time data synchronization between the component and the view.
- 📝 The script provides an overview of data binding in Angular, including its importance, types, and how to implement one-way and two-way data binding.
- 📚 The next lecture will delve into string interpolation as a method for achieving one-way data binding from the component to the view template.
- 👋 The lecture concludes with a thank you to the audience, indicating the end of the overview on data binding in Angular.
Q & A
What is data binding in the context of Angular?
-Data binding in Angular is a feature that allows communication between a component class and its view template, enabling the flow of data from the component class to the view template and vice versa.
Why is data binding important in Angular?
-Data binding is important in Angular because it facilitates the interaction between the UI logic in the component class and the HTML in the view template, making it easier to create dynamic and responsive user interfaces.
What are the two main parts of a component in Angular?
-The two main parts of a component in Angular are the component class, which contains the UI logic, and the view template, which contains the HTML that gets rendered in the browser.
How is data passed from the component class to the view template in Angular?
-Data is passed from the component class to the view template in Angular using data binding techniques such as string interpolation or property binding, where properties are wrapped within double curly braces in the HTML.
What is string interpolation and how is it used in data binding?
-String interpolation is a data binding technique in Angular where properties are embedded within double curly braces in the HTML template, allowing the values of these properties to be rendered directly into the HTML.
How can data be passed from the view template back to the component class?
-Data can be passed from the view template back to the component class using event binding, where event listeners are set up in the template to call methods in the component class when certain events occur.
What is the difference between one-way and two-way data binding?
-One-way data binding allows data to flow in one direction, either from the component to the view template or vice versa, while two-way data binding allows data to flow in both directions simultaneously.
How is the hidden attribute used in data binding with property binding?
-In property binding, the hidden attribute is used within square brackets to bind the property value from the component class to the attribute in the view template, controlling the visibility of the element based on the property's value.
What is the role of the 'ngModel' in two-way data binding?
-The 'ngModel' directive in Angular is used to achieve two-way data binding, allowing for a two-way synchronization between the form elements in the view template and the data properties in the component class.
Can you provide an example of how to use event binding to pass data from the view template to the component class?
-Event binding can be used by assigning a method from the component class to an event, such as a click event on a button. When the button is clicked, the method in the component class is executed, allowing data to be passed from the view template to the component class.
What are the different types of one-way data binding in Angular?
-The different types of one-way data binding in Angular include string interpolation for passing data from the component to the view template, and event binding for passing data from the view template back to the component class.
Outlines
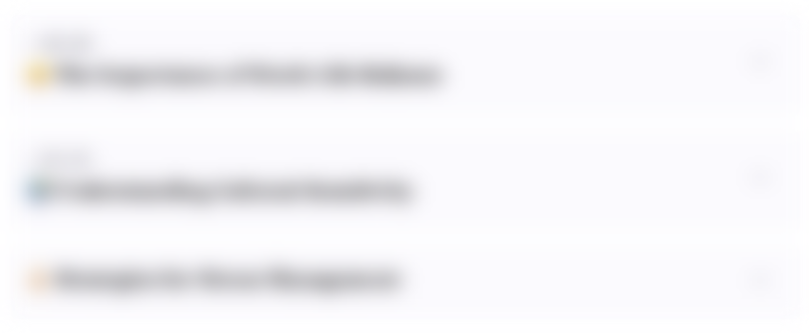
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
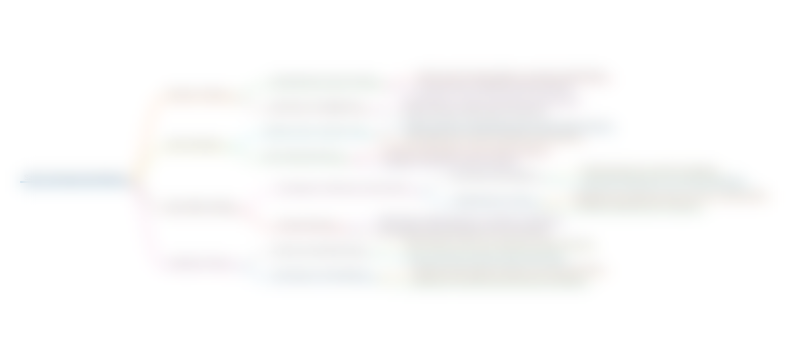
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
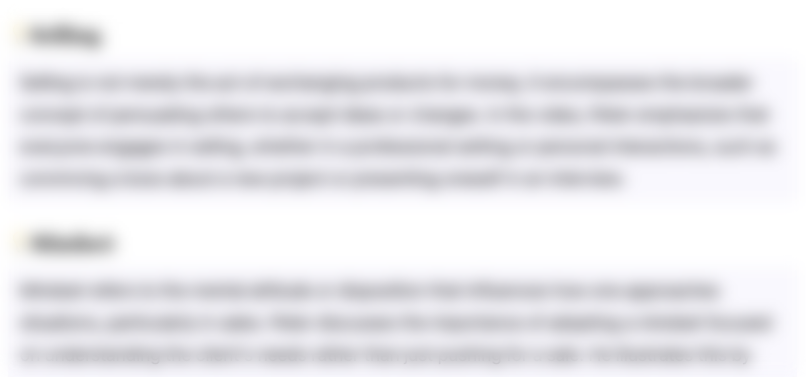
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
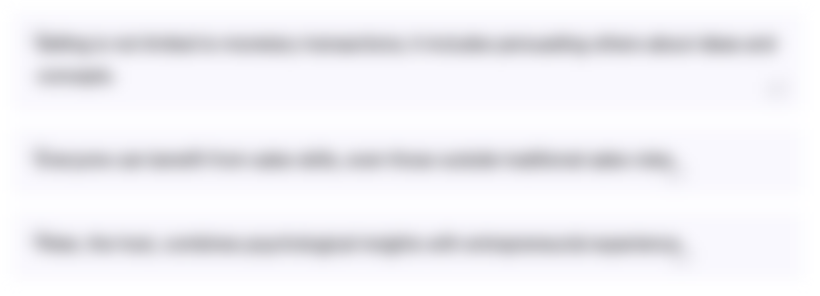
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
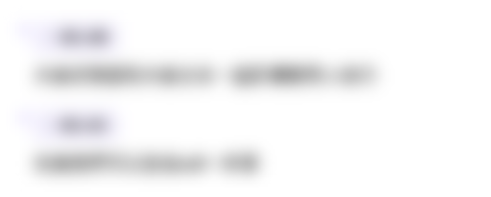
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة

#18 Two way Data Binding | Angular Components & Directives | A Complete Angular Course

#17 Event Binding | Angular Components & Directives | A Complete Angular Course
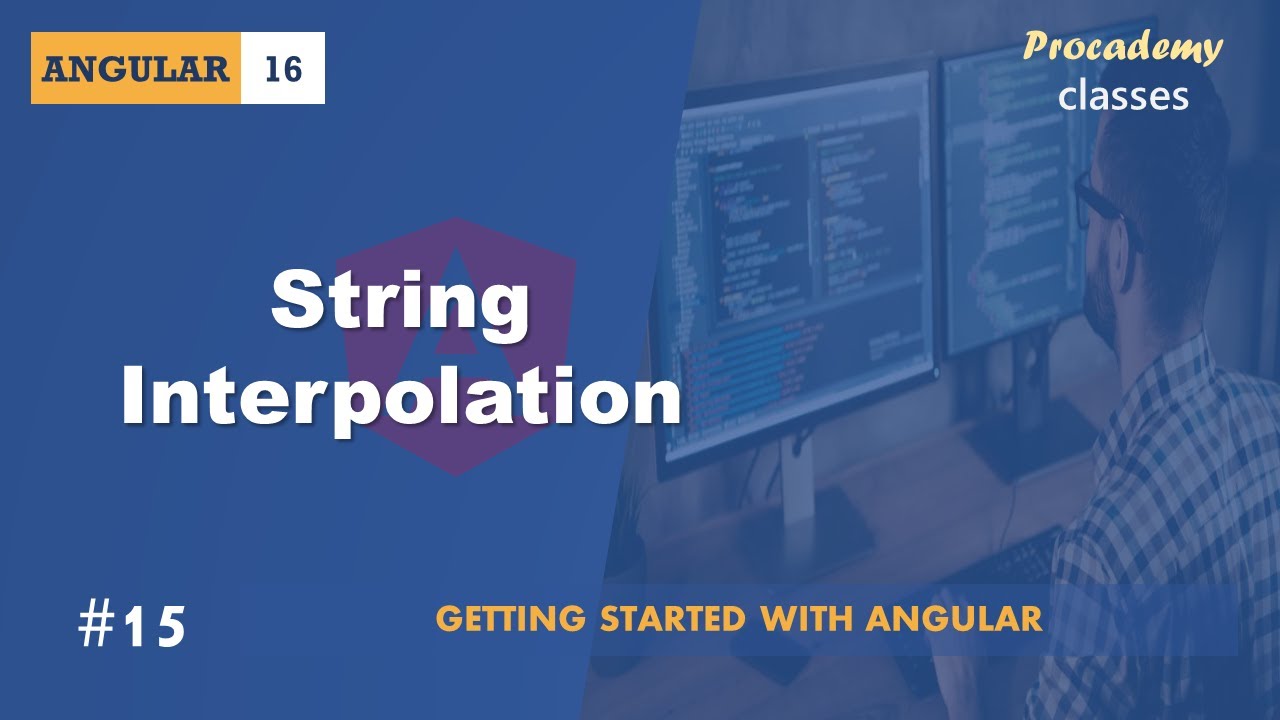
#15 String Interpolation | Angular Components & Directives | A Complete Angular Course
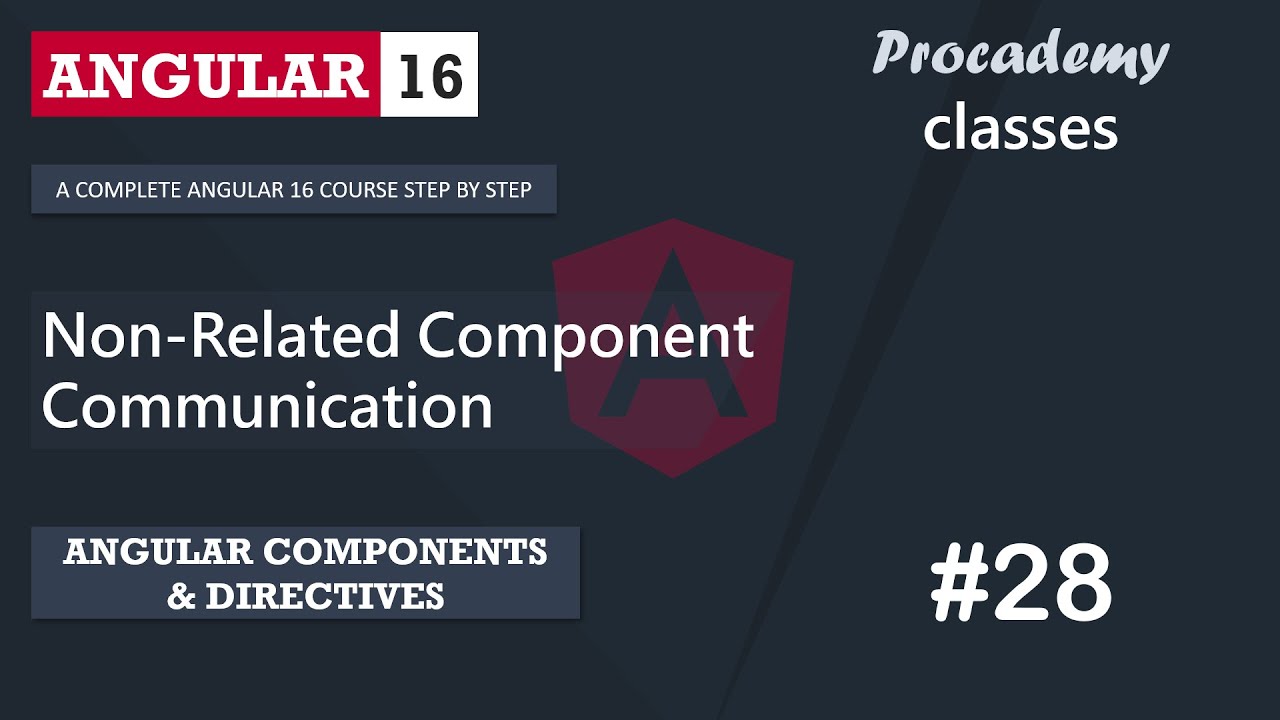
#28 Non-Related Component Communication | Angular Component & Directives | A Complete Angular Course
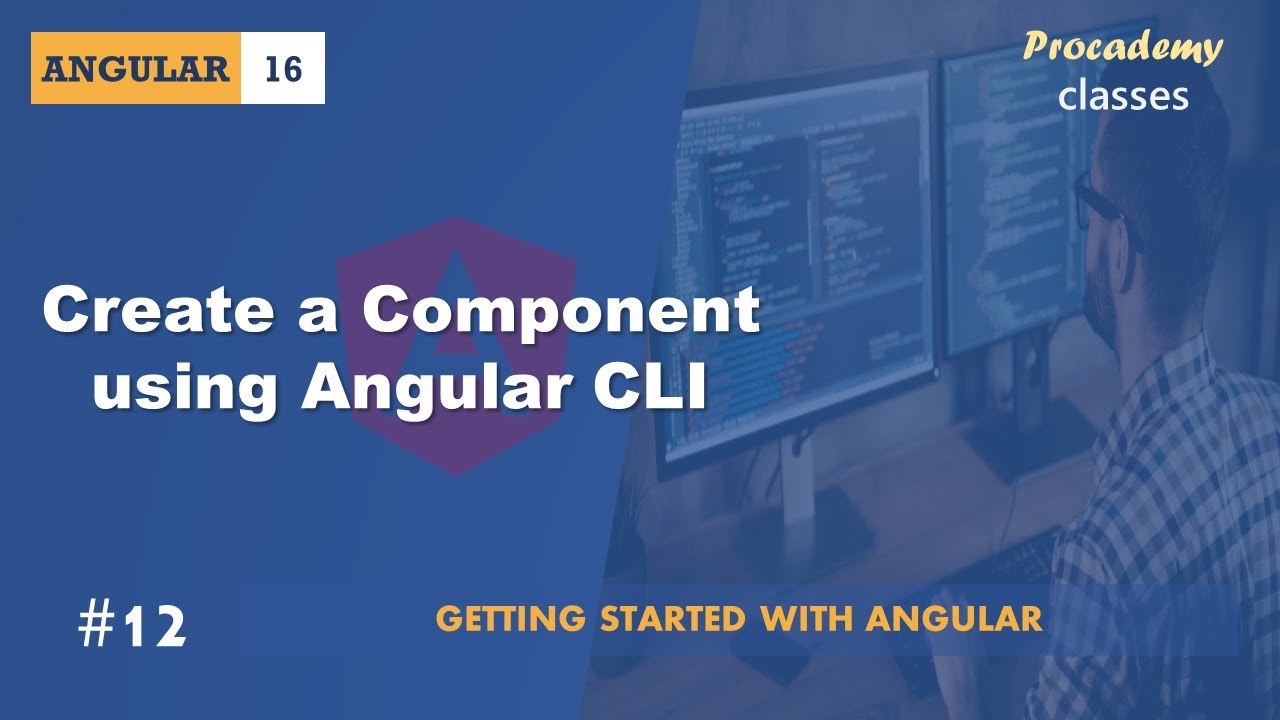
#12 Create Component using Angular CLI | Angular Components & Directives | A Complete Angular Course
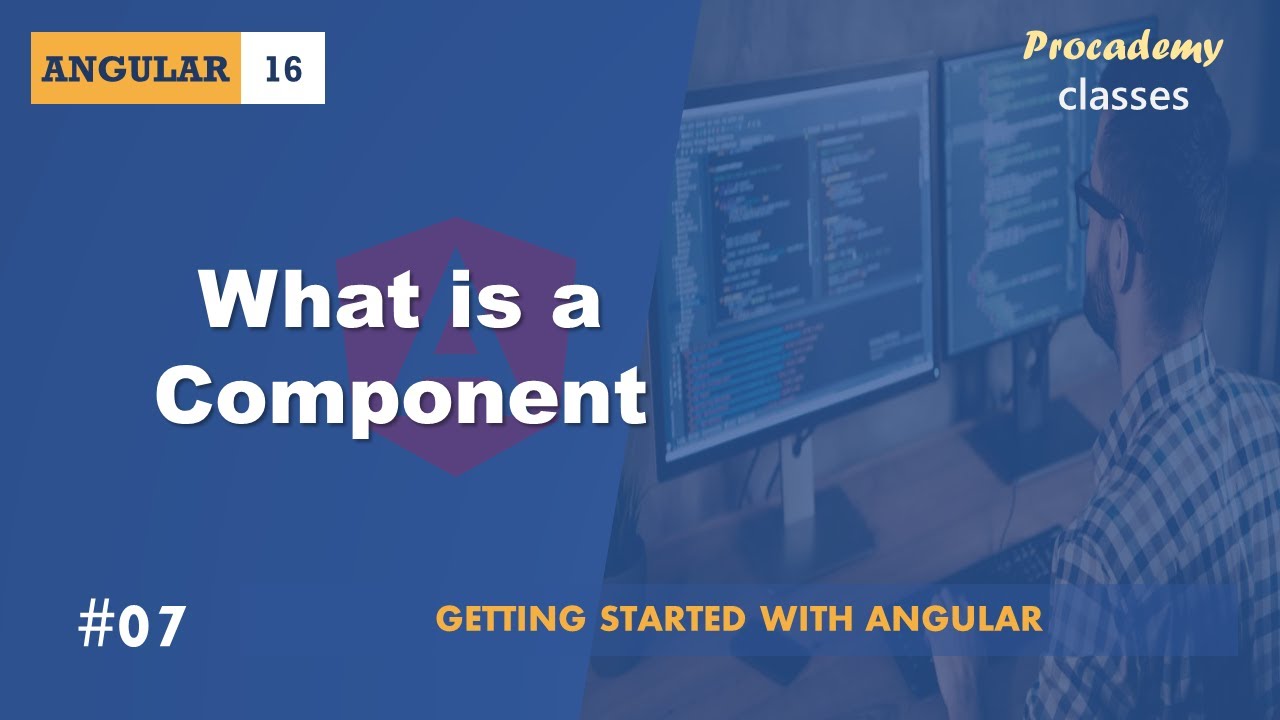
#07 What is a Component | Angular Components & Directives| A Complete Angular Course
5.0 / 5 (0 votes)