#38 Spring Security | Validating JWT Token
Summary
TLDRThis video tutorial demonstrates the process of implementing JWT authentication in a Spring Security application. It covers creating and verifying JWT tokens, modifying the security configuration to prioritize JWT over traditional username/password authentication, and adding a custom filter to handle token validation. The presenter guides viewers through coding examples, troubleshooting, and adapting to changes in library versions, ultimately enabling a secure, token-based authentication system.
Takeaways
- 🔒 The video demonstrates the process of creating and using a JWT (JSON Web Token) for authentication in a Spring Security context.
- 📝 It explains that after successful login with username and password, a JWT is generated and sent to the client for subsequent requests.
- 🔄 The client must send the JWT in the Authorization header without the username and password for accessing secured resources.
- 🛠️ The video outlines the need to create a custom JWT filter that precedes the default username and password authentication filter in the security filter chain.
- 👤 The JWT filter is responsible for extracting the token from the Authorization header, validating it, and creating an authentication object if the token is valid.
- 🔑 The process of validating the token involves decoding it, checking for expiration, and ensuring it corresponds to a valid user in the database.
- 🛑 The video emphasizes the importance of checking if the security context is already authenticated to avoid re-authentication of the user.
- 📚 It mentions the creation of methods in the JWT service for extracting the username from the token and validating the token's authenticity and expiration.
- 🔄 The video script includes code snippets and explanations for implementing the JWT filter and service, including handling changes in library versions.
- 📈 The presenter guides the viewer through the entire flow from token generation to validation, emphasizing the steps involved in securing an application with JWT.
- 💻 The video concludes with a successful demonstration of the JWT authentication flow in a Postman request, showing that the system is working as expected.
Q & A
What is the purpose of JWT in the context of the script?
-JWT (JSON Web Tokens) are used for authentication and authorization in the script. They allow the server to generate a token after a successful login, which can then be used to access secured resources without the need to send username and password with every request.
How does the server verify the username and password in the script?
-The server verifies the username and password by checking them against the credentials stored in the database. If they match, the server proceeds to generate a JWT for the user.
What issue is being addressed with the token in the script?
-The issue being addressed is that while the token is being generated correctly, the application is not able to use it to access resources like fetching students' data. The token needs to be validated on the server side to make this work.
What is the role of the 'OncePerRequestFilter' in the script?
-'OncePerRequestFilter' is an abstract class that ensures the filter logic is executed only once per request in the Spring Security filter chain. It is used as a base for creating custom filters like the JWT filter.
How does the script handle the order of filters in the Spring Security configuration?
-The script modifies the filter chain order by adding a custom JWT filter before the UsernamePasswordAuthenticationFilter. This ensures that the JWT filter is checked first for authentication before falling back to username and password authentication.
What is the significance of the 'doFilterInternal' method in the script?
-The 'doFilterInternal' method is an abstract method that must be implemented in any filter extending 'OncePerRequestFilter'. It contains the core logic for processing the incoming HTTP request and is where the JWT validation takes place.
How does the script extract the JWT from the HTTP request header?
-The script extracts the JWT from the 'Authorization' header of the HTTP request. It checks if the header starts with 'Bearer ', and if so, it extracts the substring that represents the actual token.
What is the process for validating the JWT in the script?
-The validation process involves extracting the username from the JWT using a service method, checking if the token is not expired, and ensuring that the username exists in the database. If these conditions are met, the token is considered valid.
How does the script create an authentication object after validating the JWT?
-After validating the JWT, the script creates a 'UsernamePasswordAuthenticationToken' with the username as the principal, null as the credentials, and authorities fetched from the user details.
What changes are required in the 'JWTService' class to support the new version of the JWT library?
-In the new version of the JWT library, changes include using 'verifyWith' instead of 'setSigningKey', and updating method calls to reflect changes in the library's API, such as using 'signedClaims' instead of 'claims' and 'payload' instead of 'body'.
How does the script ensure that the authentication process is secure?
-The script ensures security by validating the JWT for each request, checking for token expiration, and ensuring that the username mentioned in the token exists in the database. It also sets the authentication object in the security context to bypass the username and password authentication filter for subsequent requests.
Outlines
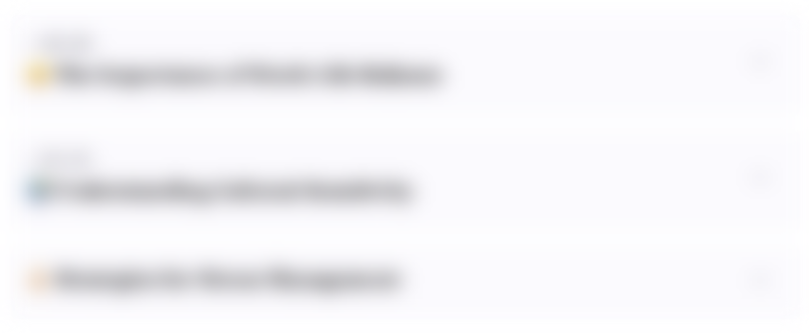
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
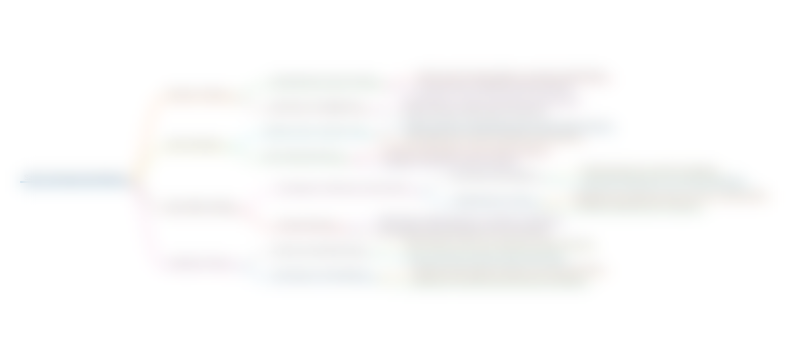
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
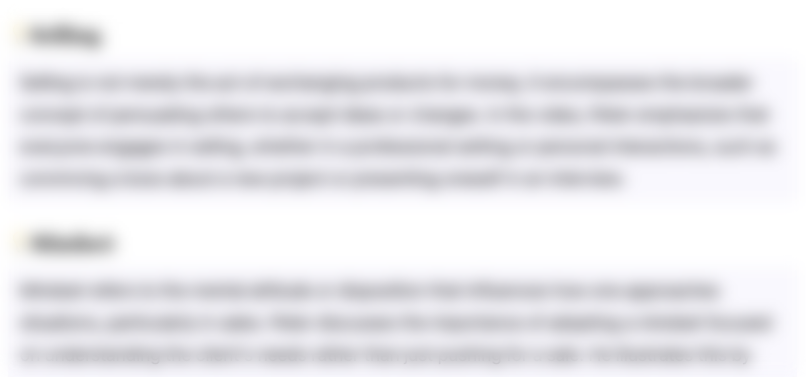
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
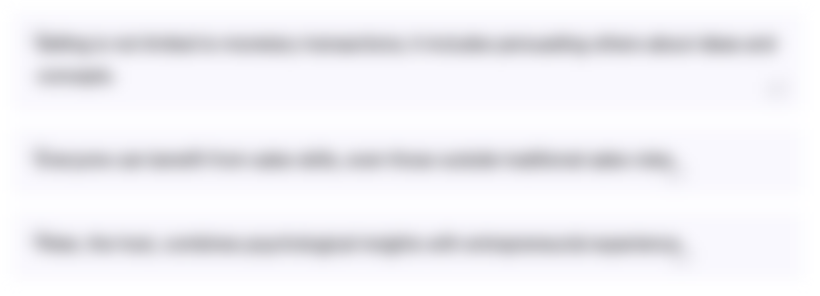
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
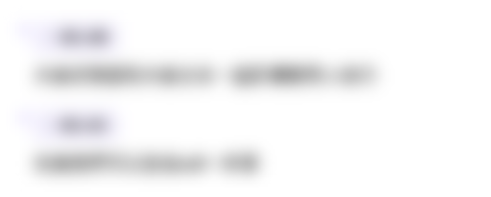
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة

Learn JWT in 10 Minutes with Express, Node, and Cookie Parser

Adding JWT Authentication & Authorization in ASP.NET Core
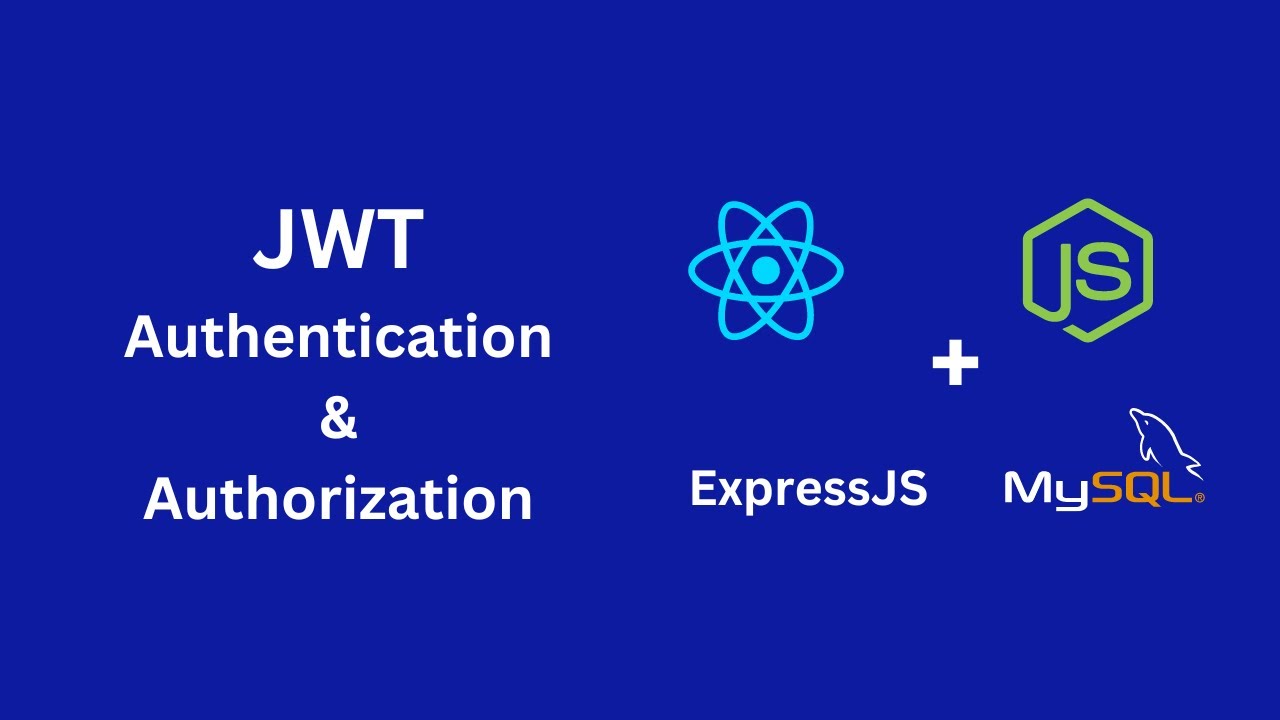
JWT Authentication with Node.js, React, MySQL | Node JS Authentication With JSON Web Token
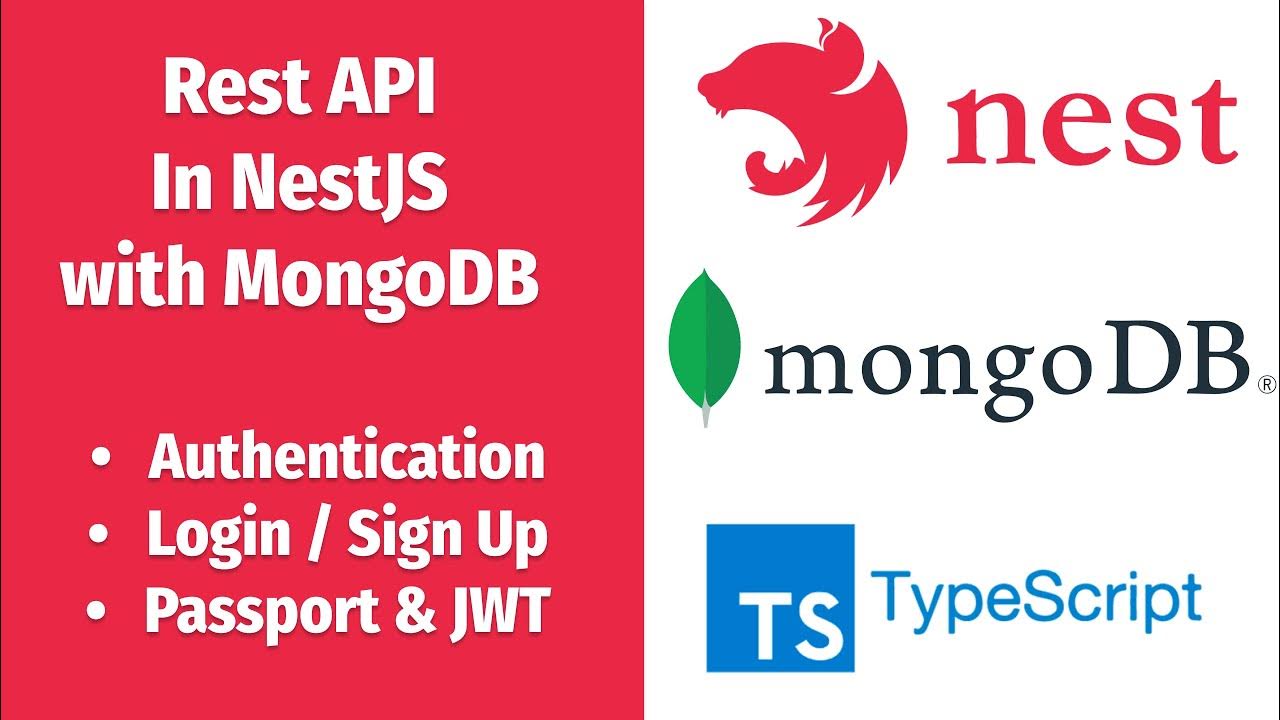
NestJs REST API with MongoDB #4 - Authentication, Login/Sign Up, assign JWT and more
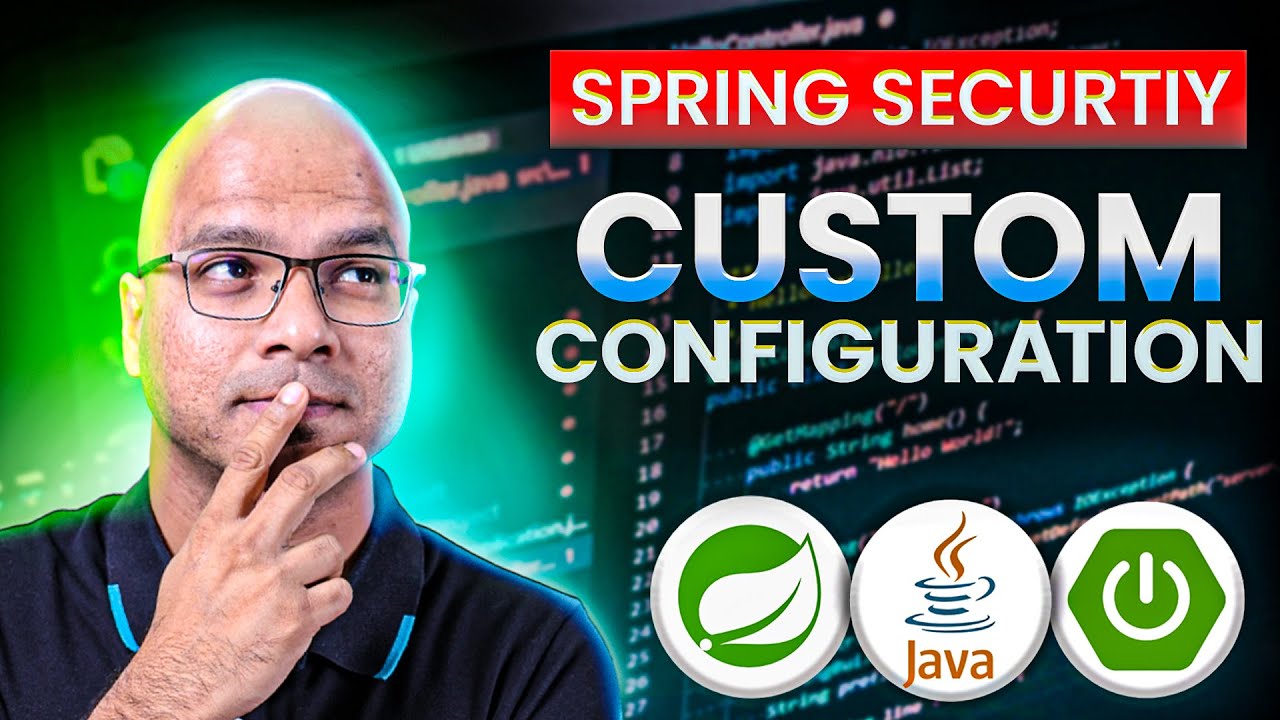
#32 Spring Security | Custom Configuration
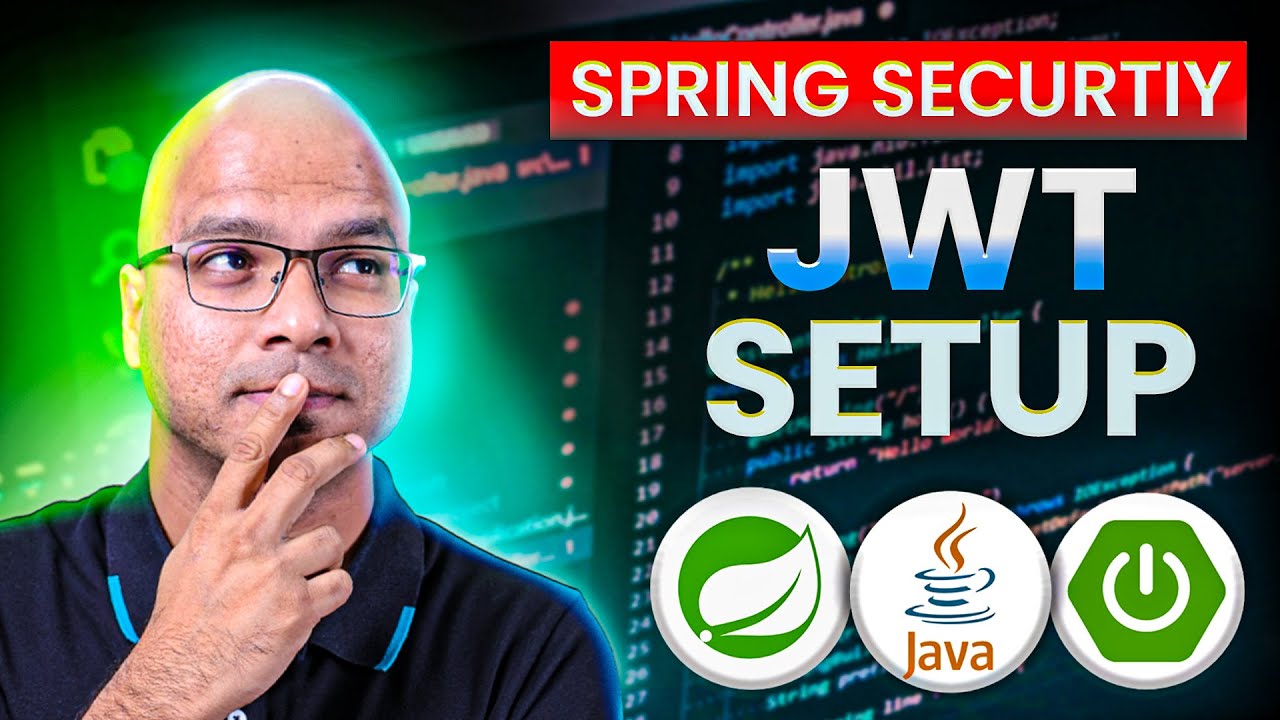
#36 Spring Security Project Setup for JWT
5.0 / 5 (0 votes)