Javascript Interview Questions ( Var, Let and Const ) - Hoisting, Scoping, Shadowing and more
Summary
TLDRThis video script discusses the intricacies of variable declaration in JavaScript, focusing on 'var', 'let', and 'const'. It explains scope, shadowing, and hoisting, highlighting how 'var' is function-scoped and can be redeclared, while 'let' and 'const' are block-scoped and cannot. The script delves into the temporal dead zone and the importance of understanding execution context. Aimed at interview preparation, it provides insights into common interview questions and the impact of variable declaration on JavaScript's behavior.
Takeaways
- 📚 JavaScript has three ways to declare variables: `var`, `let`, and `const`, with `var` being the oldest and `let` and `const` introduced in ES6 to overcome some limitations of `var`.
- 🔍 Scope is a critical concept in JavaScript, defining regions where variables can be recognized; it can be global, functional, or block scope.
- 🚫 The `var` keyword declares variables with function scope or global scope, allowing for redeclaration without error, unlike `let` and `const` which are block-scoped and do not allow redeclaration in the same scope.
- 🔑 Variable shadowing occurs when a variable declared with the same name in a inner scope takes precedence over an outer scope variable, which is allowed with `var` but not with `let` or `const` in the opposite scenario.
- 🚫 `let` and `const` must be initialized at the time of declaration, unlike `var` which can be declared without initialization.
- 🔄 Variables declared with `var` and `let` can be updated or reassigned, but `const` variables cannot be updated once initialized, attempting to do so results in an error.
- 💡 Hoisting is a JavaScript mechanism where variable and function declarations are moved to the top of their containing scope during the creation phase, but `let` and `const` are in the 'Temporal Dead Zone' until they are initialized.
- 🛑 The 'Temporal Dead Zone' (TDZ) is a period between declaration and initialization where `let` and `const` variables are in scope but cannot be accessed, leading to ReferenceError if attempted to be used before initialization.
- 🤔 Interviewers often use questions about variable declaration, scope, hoisting, and TDZ to evaluate a candidate's understanding of JavaScript fundamentals.
- 📈 The order of execution in JavaScript involves a creation phase where the context is set up, including hoisting, followed by an execution phase where the code is executed line by line.
- 🔍 Understanding the nuances between `var`, `let`, and `const` is essential for effective JavaScript coding and is a common topic in technical interviews.
Q & A
What are the three ways to declare variables in JavaScript?
-The three ways to declare variables in JavaScript are using 'var', 'let', and 'const'. 'var' has been in JavaScript since its inception, while 'let' and 'const' were introduced in ES6 to overcome some limitations of 'var'.
What is the concept of scope in JavaScript?
-Scope in JavaScript refers to the region of a program where a variable exists and can be accessed. Beyond this region, the variable cannot be recognized. There are different types of scopes such as global scope, block scope, and function scope.
How does 'var' differ from 'let' and 'const' in terms of scoping?
-'var' is function-scoped or globally-scoped if not declared within a function, whereas 'let' and 'const' are block-scoped, meaning they are only accessible within the block in which they are declared.
What is variable shadowing in JavaScript?
-Variable shadowing occurs when a local variable with the same name as a variable in an outer scope is declared, effectively 'shadowing' the outer variable within the inner scope.
Why is it called 'illegal shadowing' when trying to shadow a 'let' variable with a 'var'?
-It is called 'illegal shadowing' because you cannot redeclare a 'let' variable with a 'var' in the same scope, as 'let' is block-scoped and 'var' is function-scoped or globally-scoped, leading to a 'variable already declared' error.
Can 'var', 'let', and 'const' be redeclared in the same scope?
-Yes, 'var' can be redeclared multiple times in the same scope without error. However, 'let' and 'const' cannot be redeclared in the same scope due to their block-scoping rules.
Why must a 'const' variable be initialized at the time of declaration?
-A 'const' variable must be initialized at the time of declaration because it represents a constant value that cannot be reassigned. If not initialized, it would lead to an error stating 'missing initializer in const declaration'.
What is the difference between updating 'var' and 'let' variables compared to 'const' variables?
-Both 'var' and 'let' variables can be updated after their initial assignment, meaning their values can be changed. In contrast, 'const' variables cannot be updated or reassigned once declared, as they are constants.
What is hoisting in JavaScript and how does it relate to 'var', 'let', and 'const'?
-Hoisting is a JavaScript mechanism where variable and function declarations are moved to the top of their containing scope during the compilation phase. 'var' variables are hoisted to the top of their function or global scope, while 'let' and 'const' are placed in a 'temporal dead zone' until they are initialized.
What is the 'temporal dead zone' and how does it differ from hoisting?
-The 'temporal dead zone' is the period between the declaration and initialization of 'let' and 'const' variables. During this time, the variables are in scope but have not yet been initialized, leading to a reference error if accessed before the initialization. This differs from hoisting, where 'var' variables are hoisted with an initial value of 'undefined'.
What is the significance of understanding hoisting and the 'temporal dead zone' in JavaScript interviews?
-Understanding hoisting and the 'temporal dead zone' is significant because it demonstrates a deeper knowledge of JavaScript's execution context and variable scoping, which are common topics in technical interviews.
Outlines
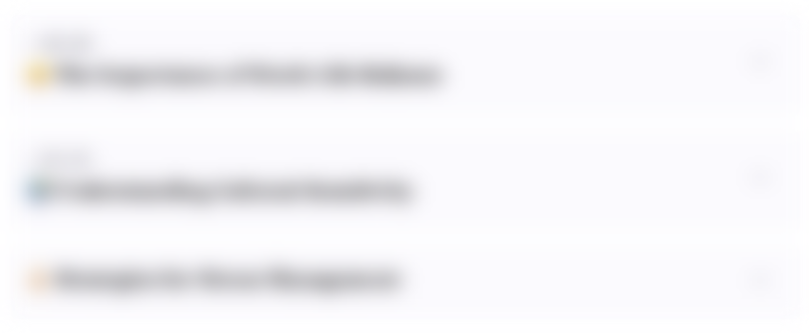
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
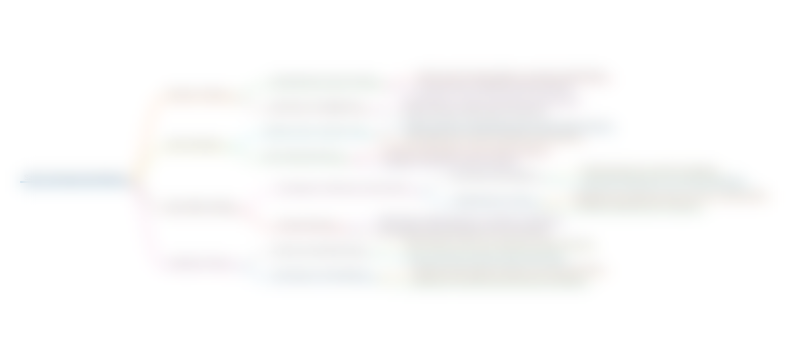
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
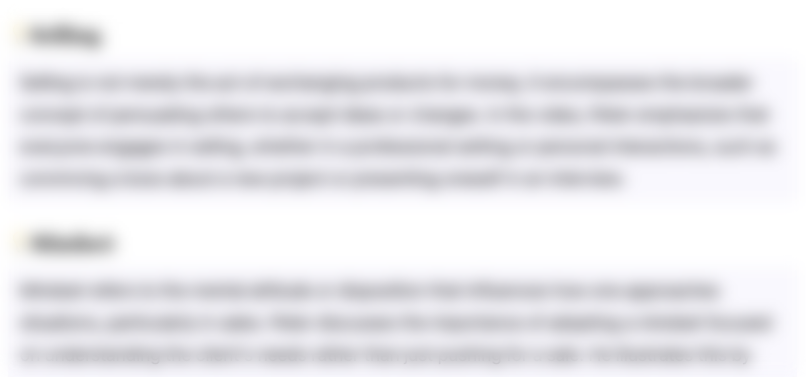
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
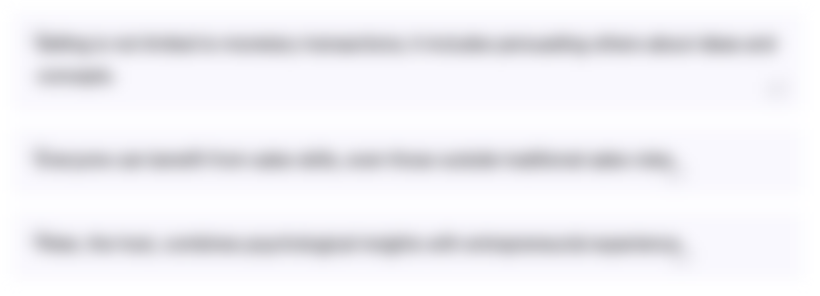
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
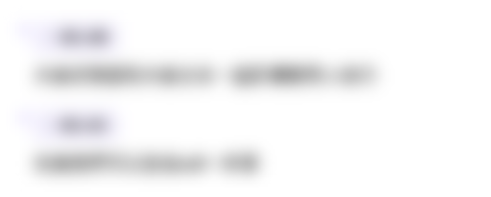
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة

Declaring Variables without Var, Let, Const - What Would Happen?

Top 10 JavaScript Interview Questions EXPLAINED! | Tanay Pratap Hindi

Codecademy: "Learn Javascript" Walkthrough | Variables: 1 - 10

Hoisting in JavaScript 🔥(variables & functions) | Namaste JavaScript Ep. 3
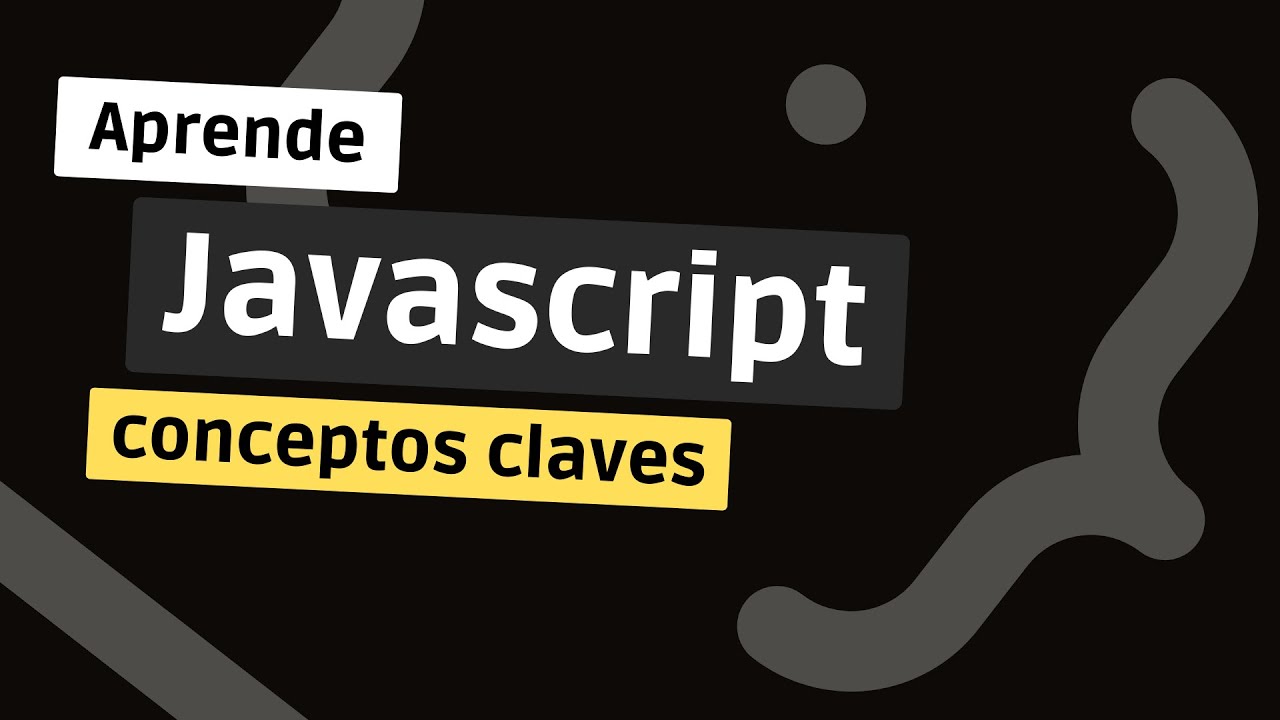
Curso Javascript - #03 const vs let vs var
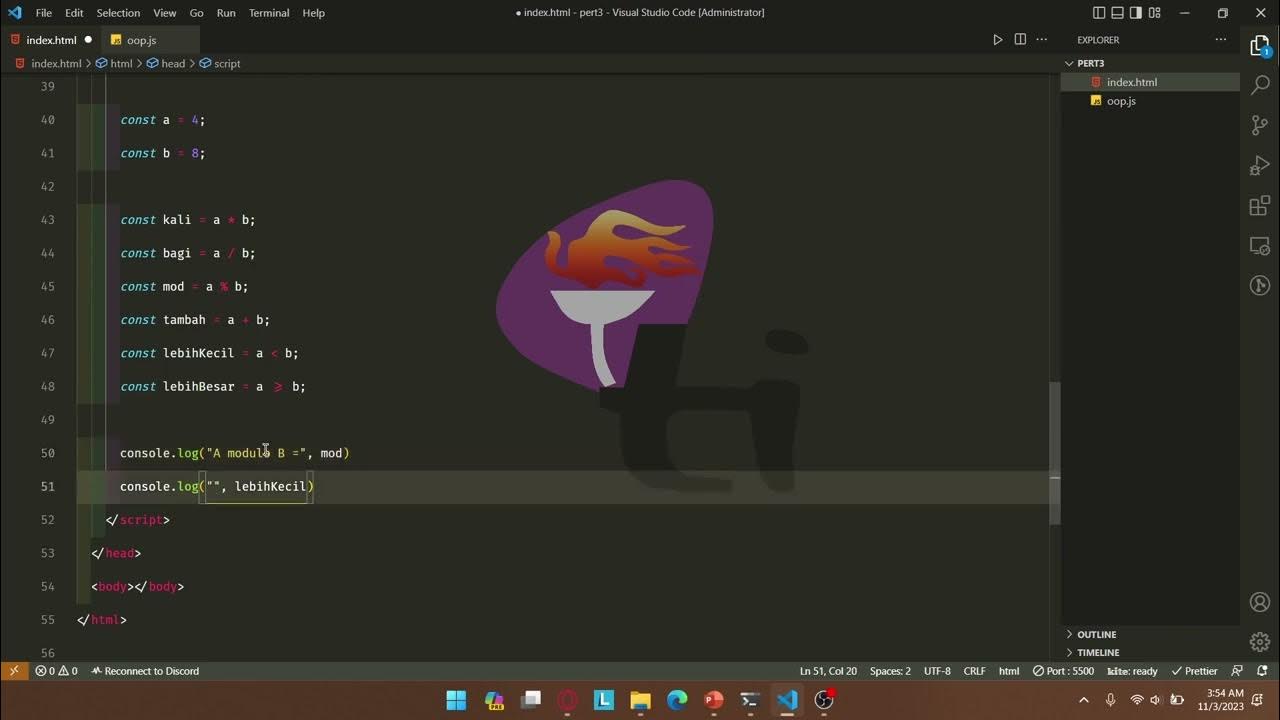
PWEB 3IA01 3IA02 | Pertemuan 3 | PJ Fierza
5.0 / 5 (0 votes)