Functions in Karel - Python
Summary
TLDRThis lesson delves into the importance and mechanics of functions in programming with Carol. Functions are essential for breaking down complex problems, enhancing code readability, and avoiding code repetition. The video script teaches how to define and name functions effectively, using 'def' and following naming conventions like snake case. It distinguishes between defining a function and calling it to execute commands, illustrating the process with a practical example of teaching Carol to 'turn around'. The lesson emphasizes the significance of functions in programming and computer science, encouraging learners to practice defining and calling their own functions.
Takeaways
- 📘 Functions are a way to teach Carol (presumably a programming environment or robot) new commands or actions.
- 🔍 Functions help break down problems into smaller, more manageable parts, making problem-solving easier.
- 📝 Using functions can make code more understandable, which is crucial when working as part of a team.
- 🔁 Functions prevent the need for repeating code, promoting efficiency and reducing errors.
- 🛠 The purpose of programming is to teach computers new things, and functions are a fundamental part of this process.
- 📝 The general format for writing a function in Carol includes using the 'def' keyword, followed by a descriptive name.
- 🔑 Function names should start with a letter, be in lowercase, and use underscores to separate words (snake case).
- 🎯 Function names should be descriptive, starting with an action verb to indicate what the function does.
- 🤖 Defining a function is like teaching Carol a new word, but calling the function is what makes Carol execute the action.
- 📑 In Python, the function definition must appear before the call statement in the code.
- 🔄 Once a function is defined, it can be called multiple times without needing to redefine it, allowing for repeated actions.
Q & A
What is the primary purpose of using functions in programming?
-The primary purpose of using functions in programming is to break a problem into smaller parts, making it easier to solve, and to avoid repeating code, which simplifies problem-solving and makes the code easier to understand and maintain.
Why is it important to use functions when working as part of a team on a coding project?
-Using functions is important in a team setting because it helps ensure that the code is readable and understandable by others, which is crucial for collaboration and maintenance of the codebase.
What is the general format for writing a function in Carol?
-The general format for writing a function in Carol starts with the keyword 'def' for define, followed by the function name, which should be descriptive and follow naming conventions such as snake case, and then a colon. The function's body, which contains the commands, is indented.
What are the basic rules for naming functions in Carol?
-The basic rules for naming functions in Carol include starting the name with a letter, using all lowercase letters, separating words with underscores (snake case), and starting with an action verb that describes what the function does.
What is the difference between defining a function and calling a function in Carol?
-Defining a function in Carol is like teaching it a new word or command, but it doesn't execute the command. Calling a function is the act of instructing Carol to execute the command associated with the function.
Why is it necessary to define a function before calling it in Python?
-In Python, a function must be defined before it can be called because the interpreter needs to know about the function's existence and structure before it can execute its commands.
What does the keyword 'def' stand for in the context of defining a function in Carol?
-The keyword 'def' stands for 'define', which is used to declare the start of a function definition in Carol.
How can you make a function name in Carol more descriptive?
-A function name in Carol can be made more descriptive by starting with an action verb, using lowercase letters, separating words with underscores, and ensuring the name clearly communicates the function's purpose.
What is the significance of using snake case for function names in Carol?
-Using snake case for function names in Carol improves readability by clearly separating words within the function name, making it easier to understand the function's purpose at a glance.
Can you provide an example of a poorly named function and how it could be improved based on the script?
-An example of a poorly named function from the script is 'blahblah', which is not descriptive. It could be improved to something like 'take_four_balls', which clearly communicates the action the function is meant to perform.
How does the script demonstrate the process of teaching Carol a new command and then executing it?
-The script demonstrates this process by first defining a function called 'turnaround' with the necessary commands for Carol to turn around. Then, it shows how to call this function multiple times in the code to execute the turn around action without needing to redefine the function.
Outlines
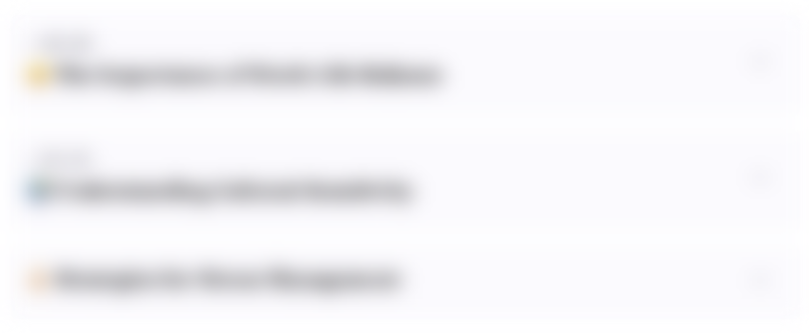
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
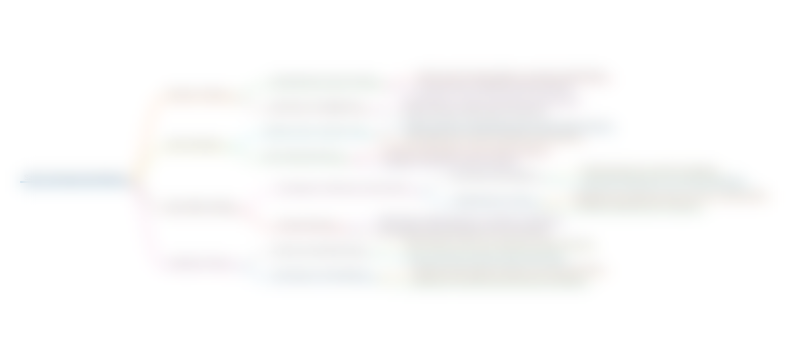
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
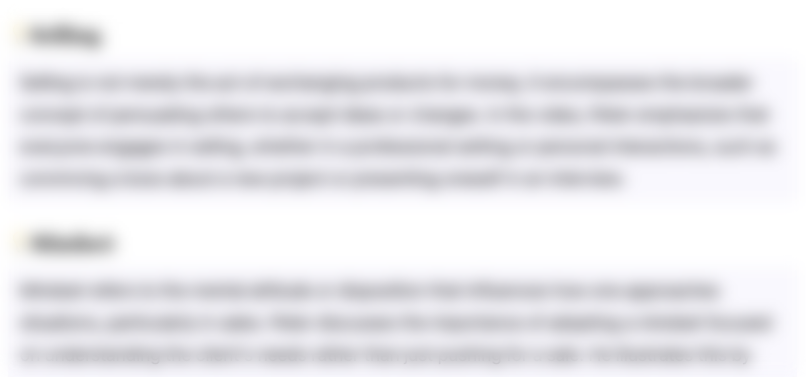
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
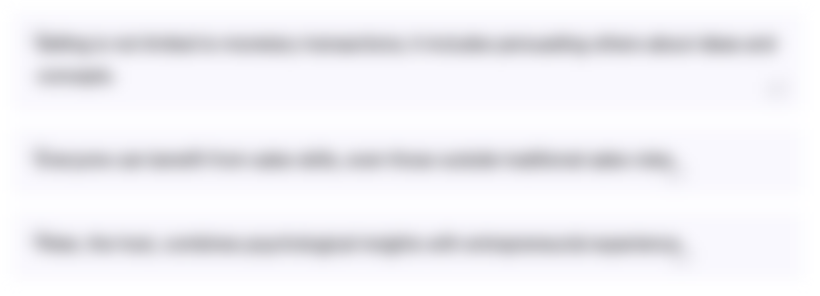
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
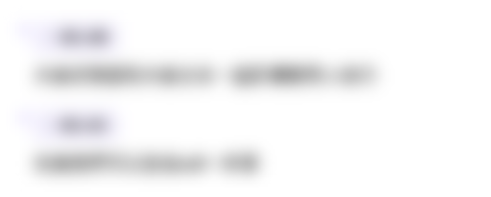
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآن5.0 / 5 (0 votes)