NASM Assembly programming Tutorial 01
Summary
TLDRThis tutorial introduces viewers to writing a basic assembly program similar to those in C or C++. The script guides through creating a file with the .ASM extension, setting up a data and text section, and using a 'printf' function to display a message on the screen. It explains the importance of stack frame setup and teardown, and concludes with compiling the program using NASM and GCC. The tutorial is designed to be accessible to those familiar with C programming, aiming to transition into the world of assembly language.
Takeaways
- 📝 The tutorial is about writing a functioning assembly program similar to those in C or C++.
- 📁 The first step is to create a file with the .ASM extension for assembly language.
- 📊 A typical assembly program includes a data section for initialized data and a text section for assembly code, which may also include uninitialized variables.
- 🔧 The tutorial demonstrates how to set up basic templates for an assembly program, including sections for data and code.
- 🗑️ The script suggests removing the section for uninitialized variables if they are not needed for the program.
- 🖥️ The program aims to display a message on the screen using a C-style function called 'printf'.
- 📑 Comments and null-terminated strings are used to document and define messages in assembly language.
- 🔗 Labels in assembly are treated as memory locations, which is important for referencing in code.
- 🔝 The 'global' directive is used to make the 'main' label accessible outside the program for external calls.
- 🔄 The tutorial covers setting up and destroying a stack frame using 'push EBP' and 'mov EBP, ESP' instructions.
- 📡 The 'external printf' directive tells the assembler that the printf function is available externally and not defined within the current source code.
- 🔧 The process of compiling an assembly program is explained, including using NASM for assembly and GCC for the final program compilation.
- 🎉 The tutorial concludes with the successful display of a message in assembly, indicating a working program.
Q & A
What is the purpose of the tutorial in the transcript?
-The purpose of the tutorial is to guide the user through writing a functioning assembly program similar to those found in C or C++.
What file extension is suggested for an assembly program?
-The suggested file extension for an assembly program is .ASM.
What are the two main sections of an assembly program as described in the transcript?
-The two main sections of an assembly program are the data section for initialized data and the text section for the actual assembly code and uninitialized variables.
Why might one choose to not include an uninitialized data section in an assembly program?
-One might choose not to include an uninitialized data section if the program does not require the use of uninitialized variables.
What is the purpose of the 'printf' function in the context of this assembly program?
-The 'printf' function is used in the assembly program to display a message to the screen, similar to its use in C programming.
What does the 'global main' directive do in assembly language?
-The 'global main' directive makes the 'main' label available outside of the immediate program, allowing other programs to find and start from it.
What is the purpose of the 'push EBP' instruction in setting up a stack frame?
-The 'push EBP' instruction saves the base pointer of the caller and sets up a new stack frame by moving the stack pointer into EBP.
What does the 'external printf' declaration indicate in the assembly program?
-The 'external printf' declaration tells the assembly program that the 'printf' function is available externally and not declared within the source code itself.
What is the process for compiling an assembly program as described in the transcript?
-The process involves using an assembler like NASM to create an object file (.o), and then using a compiler like GCC to link the object file and produce the final executable program.
What command is used to assemble the .ASM file using NASM in the tutorial?
-The command used to assemble the .ASM file using NASM is 'nasm -f elf32 asm1.asm -o asm1.o'.
How does the tutorial suggest testing the compiled assembly program?
-The tutorial suggests testing the compiled assembly program by running it from the command line using './asm1' and observing if the expected message is displayed.
Outlines
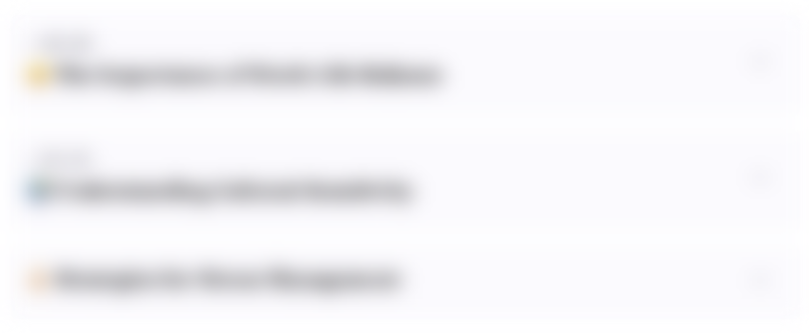
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
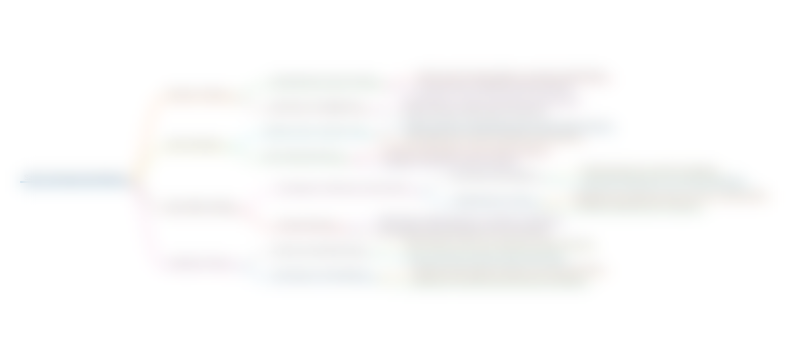
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
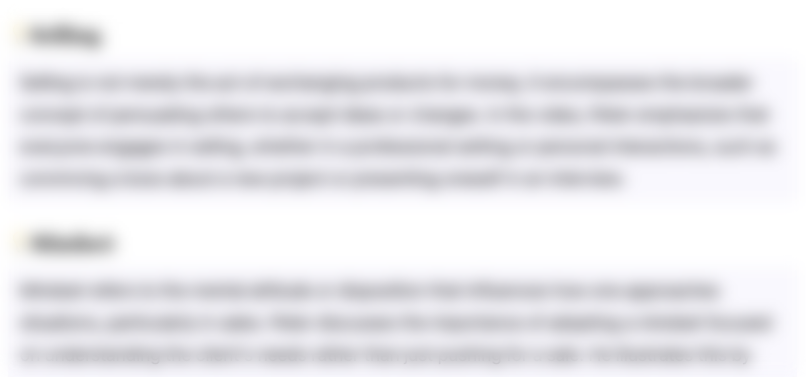
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
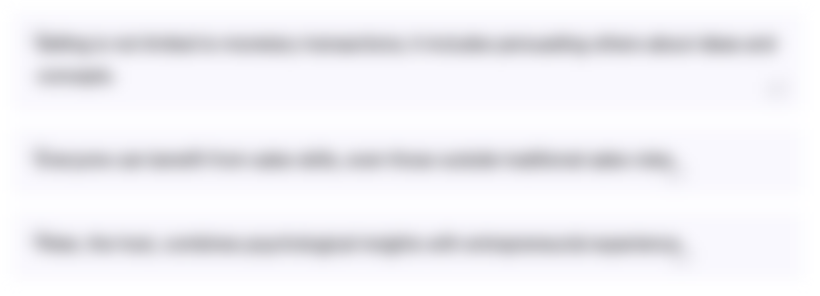
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
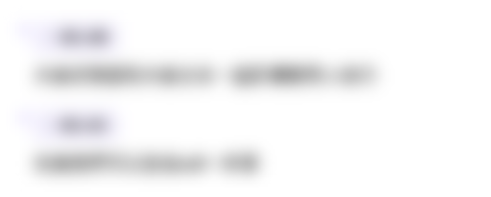
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
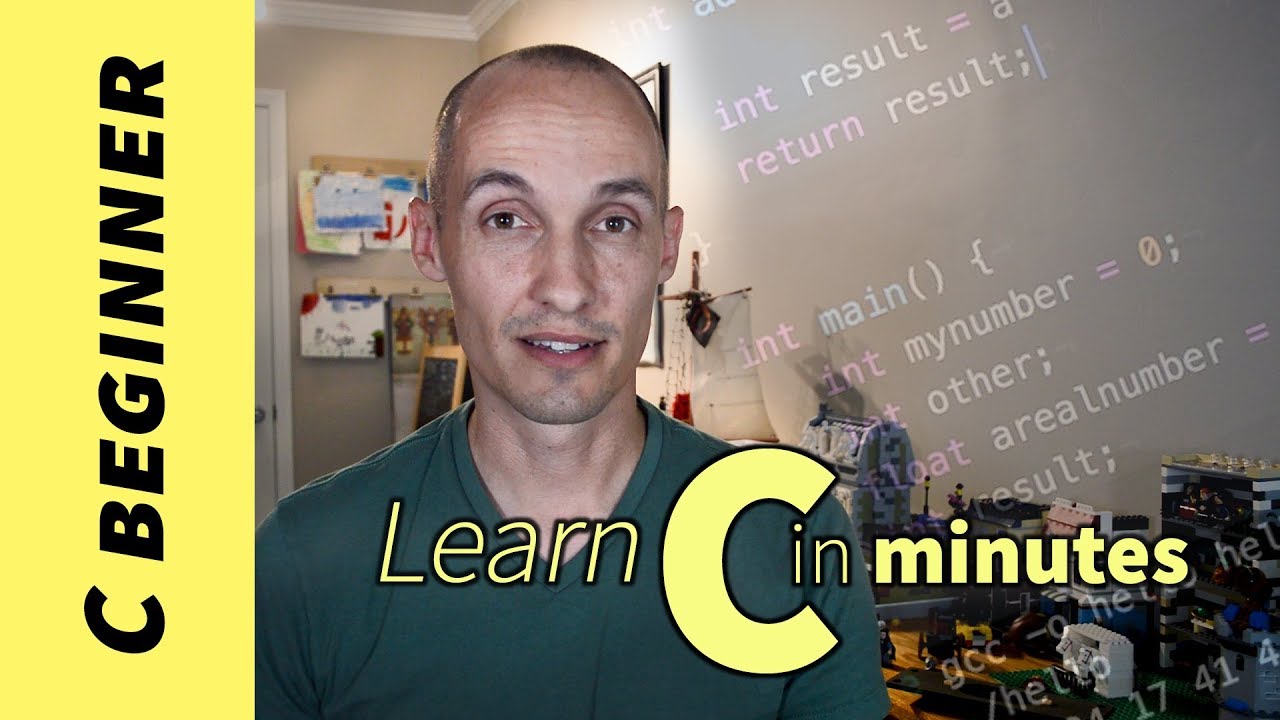
Learn C in minutes (lesson 0)
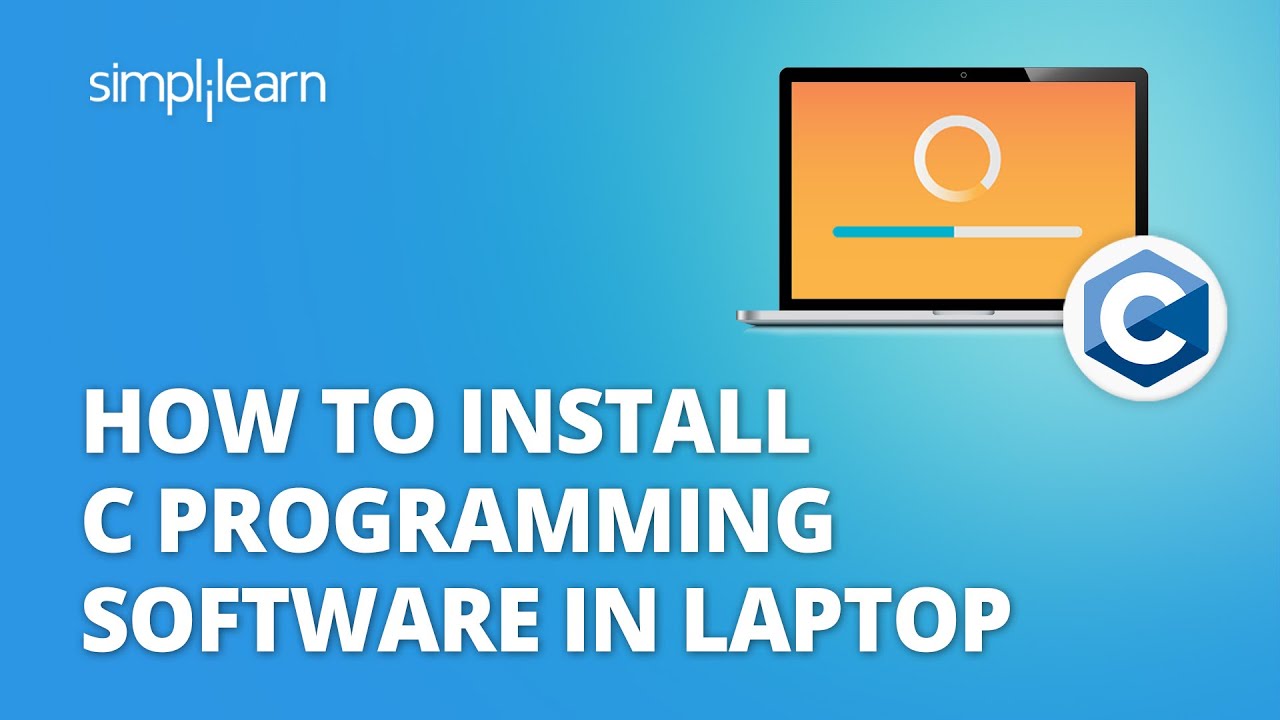
How To Install C Programming Software In Laptop | C Installation Tutorial For Beginners |Simplilearn
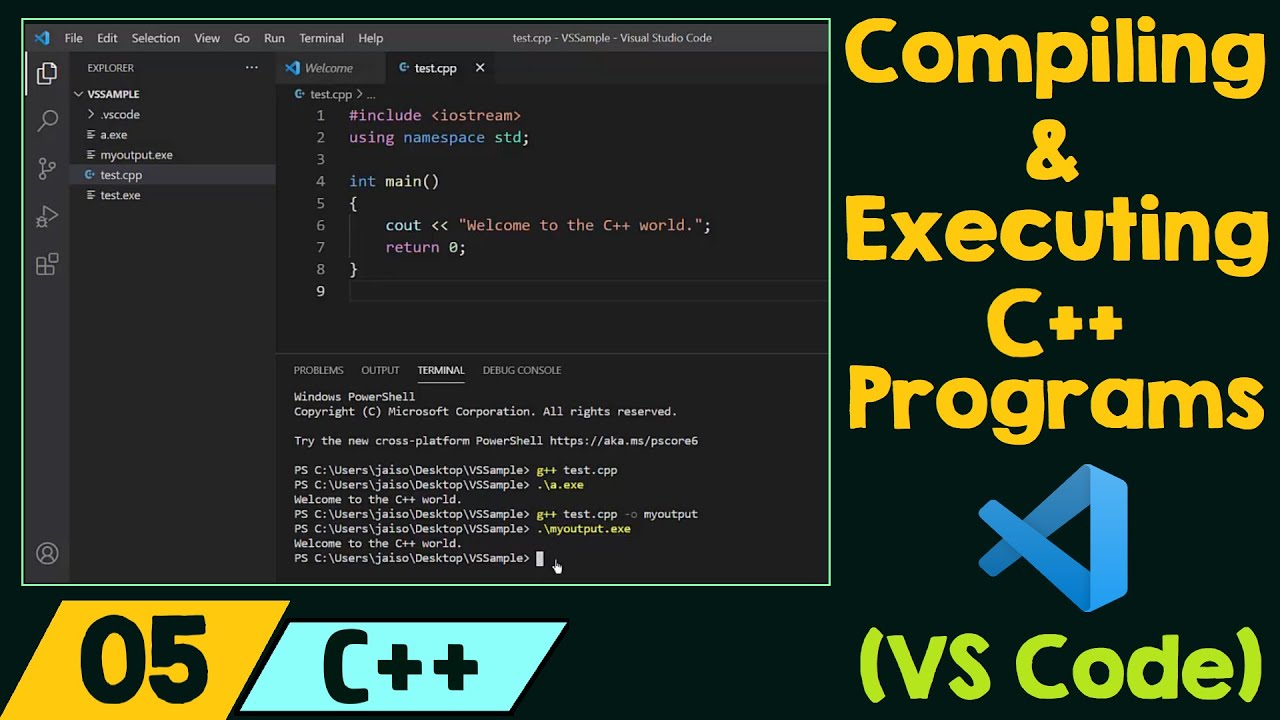
Compiling & Executing C++ Programs (VS Code)
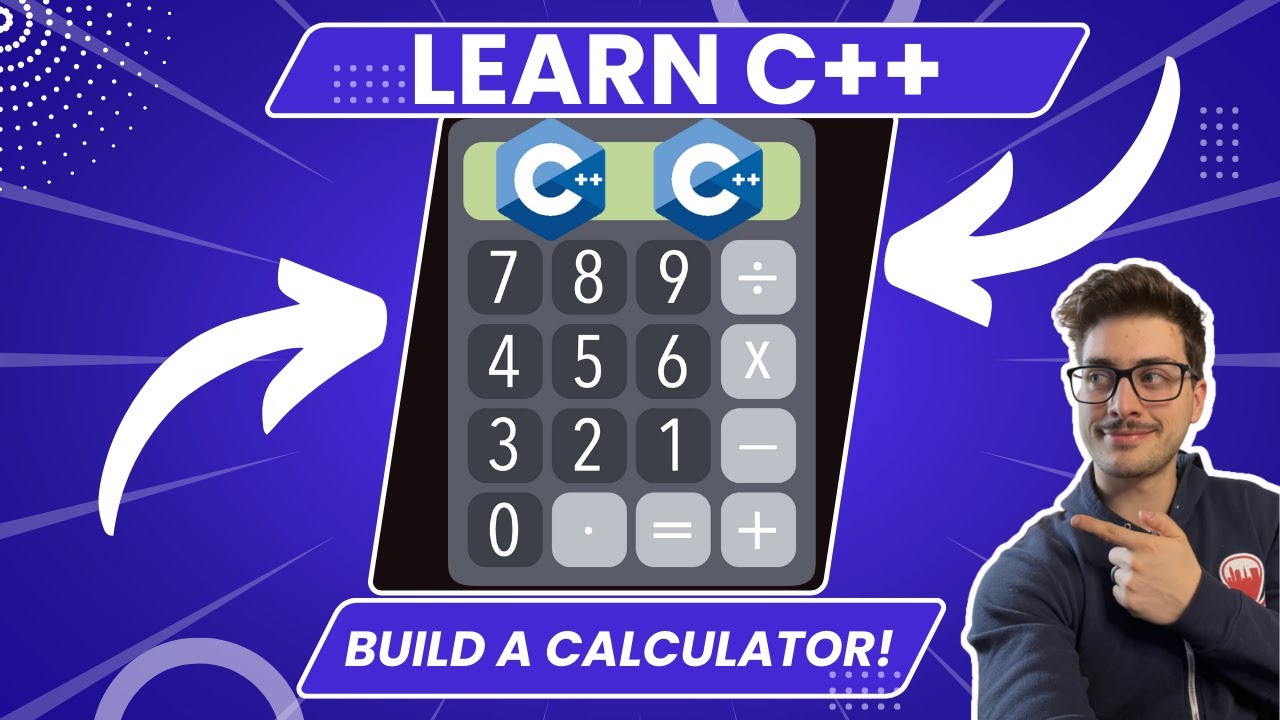
How to Program A Calculator in C++! (Great C++ Microsoft Visual Studio Beginner Project)
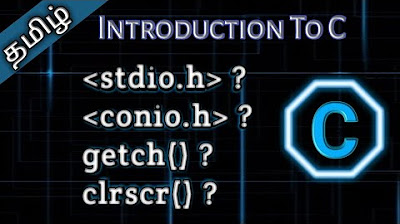
1. Introduction to C Language in Tamil || Tamil Pro Techniques ||
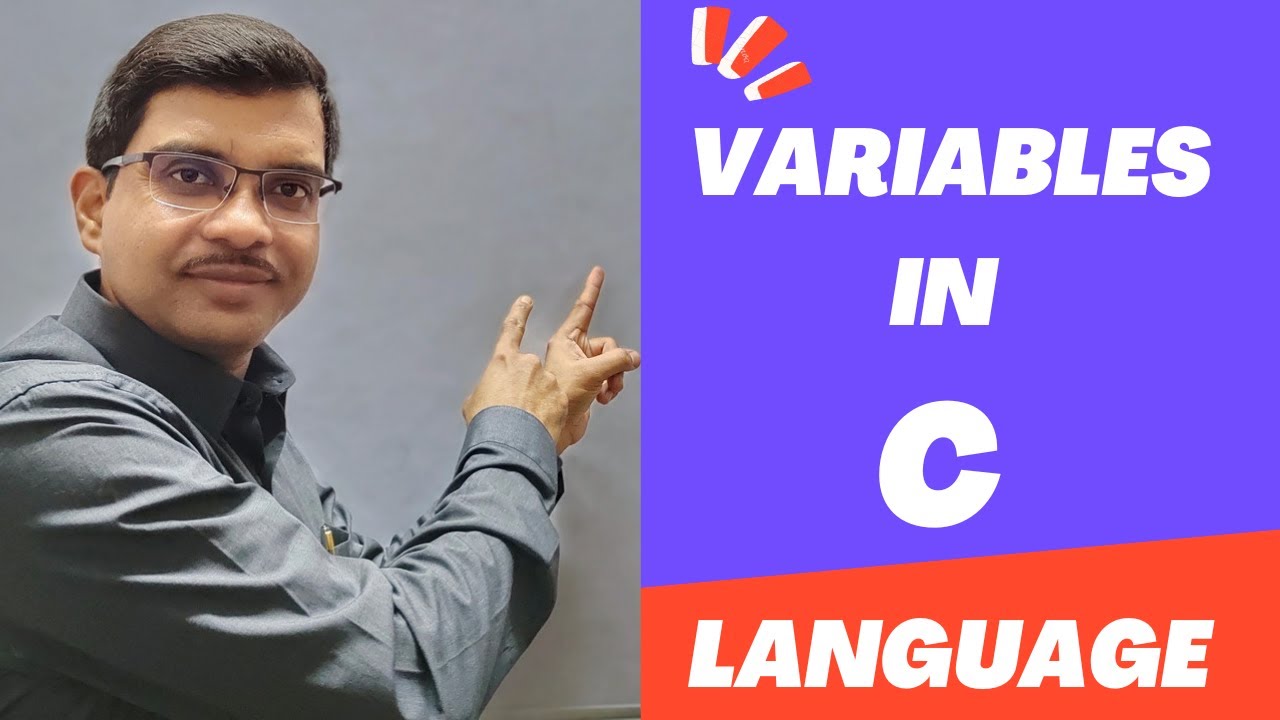
C_07 Variables in C Language | C Programming Tutorials
5.0 / 5 (0 votes)