Topic 3 Lesson 9
Summary
TLDRThis lesson introduces the Bisection method for approximating square roots of numbers between 0 and 1, avoiding the power operator due to floating-point inaccuracies. The method efficiently narrows the search interval by halving it with each iteration, aiming for an approximation error less than a given tolerance, epsilon. The script explains the iterative process, provides a Python code example, and compares the Bisection method's efficiency to Heron's method, highlighting its straightforward convergence analysis.
Takeaways
- 📚 The lesson focuses on the Bisection method, a numerical technique used to approximate the square root of a given number.
- 🔢 It is assumed that the number \( x \) is non-negative and less than or equal to 1, ensuring the square root is between \( x \) and 1.
- 📉 The method avoids using the power operator and instead relies on the properties of the square root function within the given interval.
- 🔍 The goal is to find an approximation \( p \) such that \( |p^2 - x| < \epsilon \), where \( \epsilon \) is a given tolerance (e.g., \( 10^{-6} \)).
- 🚫 A brute force approach would involve checking many points, which is inefficient and slow.
- 🔄 The Bisection method improves efficiency by examining the midpoint of the interval and narrowing the search based on whether the midpoint squared is less than or greater than \( x \).
- 🔢 The midpoint is calculated as \( \text{mid} = \frac{\text{low} + \text{high}}{2} \), where \( \text{low} \) and \( \text{high} \) are the current bounds of the search interval.
- 🔎 The method iteratively halves the search interval, significantly reducing the number of steps needed to achieve the desired accuracy.
- 📉 The error approximation decreases exponentially with each iteration, making the Bisection method highly efficient for finding square roots.
- 📚 The lesson also mentions Heron's method, which has a faster convergence rate but is less straightforward to analyze compared to the Bisection method.
Q & A
What is the main topic of the lesson?
-The main topic of the lesson is the Bisection method, specifically its application in approximating the square root of a given number.
Why is it assumed that the number x is less than or equal to 1?
-It is assumed that x is less than or equal to 1 for simplicity, ensuring that the square root of x is between x and 1, which makes the problem more manageable.
What is the significance of the intersection point between y=sqrt(x) and y=x?
-The intersection point between y=sqrt(x) and y=x is significant because it indicates that for x values between 0 and 1, the square root of x will also be between x and 1.
Why is the brute force iterative approach considered slow?
-The brute force iterative approach is considered slow because it involves dividing the interval between x and 1 into many points and traversing them one by one, potentially requiring a large number of steps.
What is the main idea behind the Bisection method?
-The main idea behind the Bisection method is to examine the middle element of the interval between x and 1, compare it with the square root of x, and then narrow down the search interval based on the comparison, effectively bisecting the search space at each iteration.
How does the Bisection method improve efficiency compared to the brute force approach?
-The Bisection method improves efficiency by reducing the search interval length by half at each iteration, thus quickly narrowing down the possible values for the square root.
What is the stopping condition for the while loop in the Bisection method algorithm?
-The stopping condition for the while loop is when the absolute value of (mid*mid - x) is less than or equal to epsilon, indicating that the approximation is within the desired tolerance.
How does the Bisection method handle the case when mid*mid is less than x?
-When mid*mid is less than x, the method updates the lower bound of the search interval (low) to mid, effectively narrowing the search to the interval between mid and high.
How does the Bisection method handle the case when mid*mid is greater than x?
-When mid*mid is greater than x, the method updates the upper bound of the search interval (high) to mid, effectively narrowing the search to the interval between low and mid.
What is the relationship between the number of iterations and the approximation error in the Bisection method?
-The approximation error decreases exponentially with the number of iterations. After k iterations, the error is at most (1/2^(k+1)), which shows that the method can quickly reduce the error to a very small value.
How does the Bisection method compare to Heron's method in terms of convergence rate?
-While Heron's method has a faster convergence rate, its convergence analysis is less trivial than that of the Bisection method, which is more straightforward and easier to understand.
Outlines
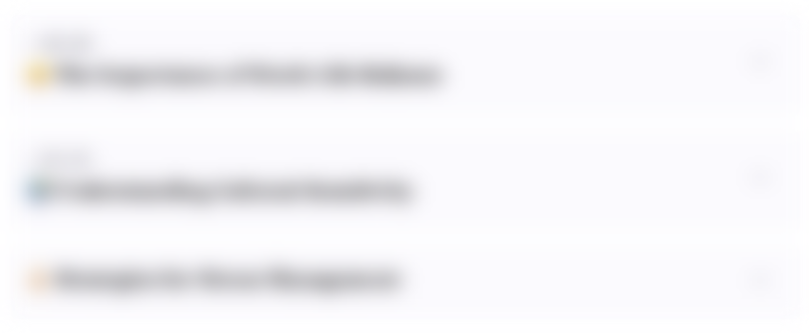
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
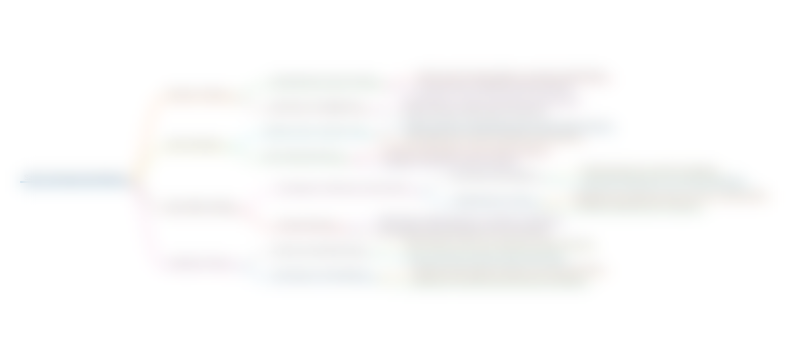
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
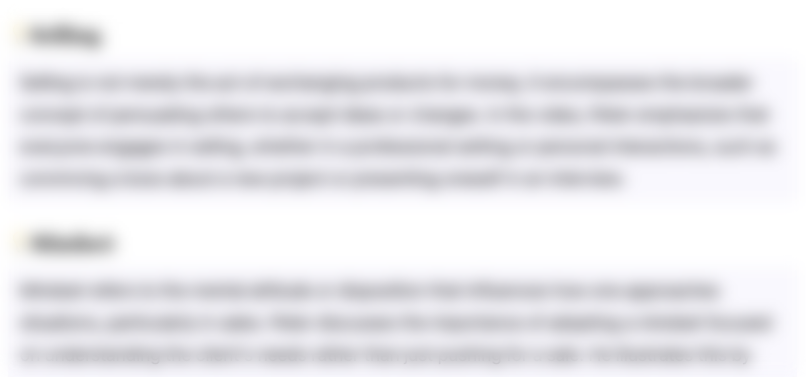
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
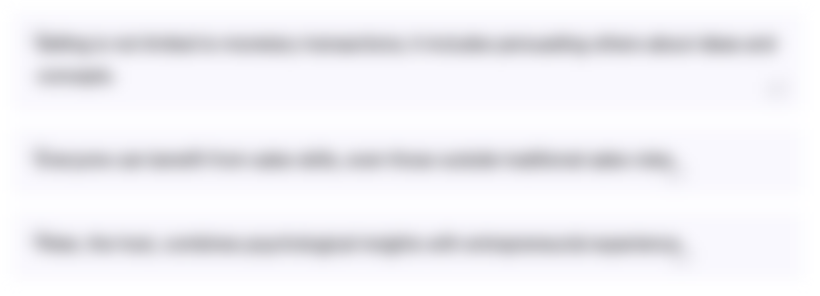
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
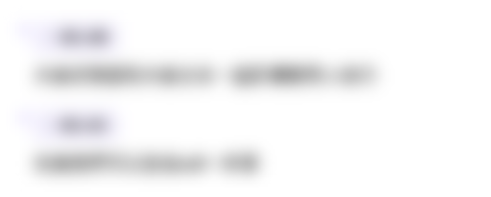
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآن5.0 / 5 (0 votes)