Java Main Method Explained - What Does All That Stuff Mean?
Summary
TLDRThis video script demystifies the Java 'public static void main(String[] args)' method, explaining its components and purpose. It clarifies that 'public' ensures accessibility, 'static' allows the method to be called without an instance, 'void' signifies no return value, 'main' is the required method name for the Java Runtime Environment (JRE) to execute the program, and 'String[] args' is an array for passing arguments to the program. The script also demonstrates how to use 'args' to accept user input and the importance of handling these arguments to avoid errors like 'ArrayIndexOutOfBoundsException'.
Takeaways
- 😀 The 'public static void main(String[] args)' method is the entry point for a Java program, which must be present for the Java Runtime Environment (JRE) to execute the code.
- 🔓 The 'public' access modifier is necessary because the main method needs to be accessible to the JRE from outside the class.
- 📌 The 'static' keyword means the main method can be called without creating an instance of the class, which is how the JRE invokes it.
- 🛑 The 'void' return type indicates that the main method does not return any value; it simply executes and then the program ends.
- 📝 The name 'main' is not arbitrary; it is the specific name that the JRE looks for to start the program.
- 🔑 The 'String[] args' parameter is an array of strings that can hold command-line arguments passed to the program, allowing for external input.
- 💡 The 'args' parameter provides flexibility, allowing programs to utilize input provided when the program is started.
- 🛠️ IDEs like Eclipse allow for setting arguments for the main method through run configurations, facilitating easy testing with different inputs.
- 🖥️ Outside of an IDE, command-line interfaces can also be used to pass arguments to a Java program by including them after the class name in the 'java' command.
- ⚠️ If the 'args' array is used in the program, it's important to ensure that arguments are passed when running the program to avoid errors like 'ArrayIndexOutOfBoundsException'.
- 🎓 Understanding each component of the 'public static void main(String[] args)' method signature demystifies the Java programming environment and empowers programmers to write more effective code.
Q & A
What is the purpose of the 'public static void main(String[] args)' method in Java?
-The 'public static void main(String[] args)' method is the entry point for any Java application. It is the method that the Java Runtime Environment (JRE) calls to start the program execution.
Why is the 'main' method declared as 'public'?
-The 'main' method is declared 'public' to ensure it is accessible to the Java Runtime Environment, which needs to call this method to run the program.
What does 'static' mean in the context of the 'main' method?
-The 'static' keyword indicates that the method can be called without creating an instance of the class. The JRE calls the main method directly on the class, not on an instance of the class.
Why does the 'main' method have a 'void' return type?
-The 'void' return type signifies that the 'main' method does not return any value. When the main method completes execution, the program ends without returning anything.
Can the 'main' method be named something other than 'main'?
-No, the 'main' method must be named 'main' because that is the name the Java Runtime Environment looks for when starting the program.
What is the purpose of the 'String[] args' parameter in the 'main' method?
-The 'String[] args' parameter is an array of strings that can hold command-line arguments passed to the program when it is executed. It allows the program to receive input from the user or the environment.
How can you pass arguments to a Java program when running it from an IDE like Eclipse?
-In Eclipse, you can pass arguments by right-clicking on the class, going to 'Run As', then 'Run Configurations', and entering the arguments in the 'Arguments' tab.
Can you pass arguments to a Java program when running it from the command line?
-Yes, you can pass arguments to a Java program from the command line by specifying them after the class name when using the 'java' command to run the program.
What happens if the 'main' method tries to access 'args' without any arguments being passed?
-If no arguments are passed and the 'main' method tries to access 'args', it will result in an 'ArrayIndexOutOfBoundsException' because the program is attempting to access an element in an empty array.
What is an example of how the 'args' parameter can be used in a Java program?
-An example use of the 'args' parameter is to print a message passed as an argument when starting the program, such as 'System.out.println(args[0]);' to print the first argument.
How can command-line arguments be used to influence the behavior of a Java program?
-Command-line arguments can be used to customize the behavior of a Java program by providing input for configuration, setting parameters, or controlling the flow of the program based on the provided values.
Outlines
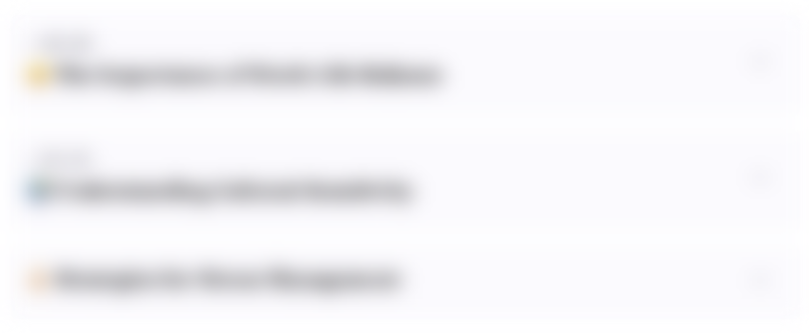
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
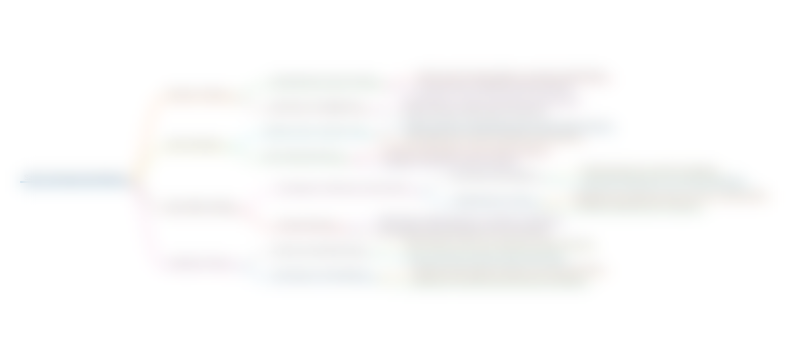
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
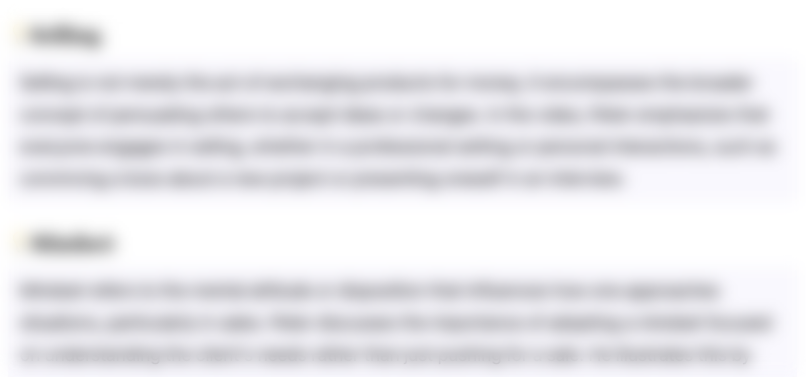
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
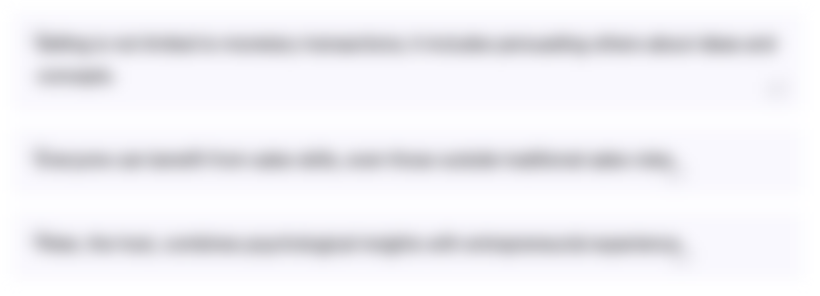
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
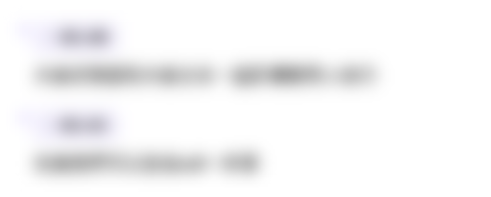
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
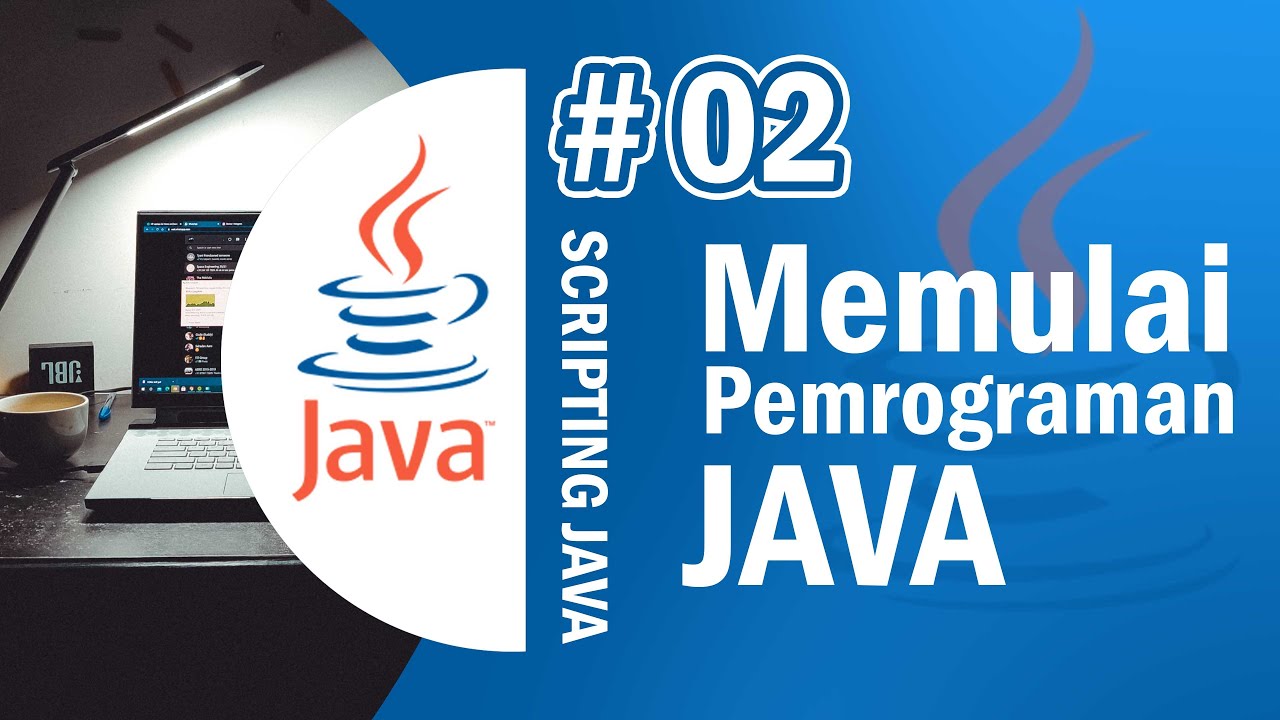
Java 02 - Memulai Pemrograman Java dengan IDE Netbeans - Tutorial Java Netbeans Indonesia
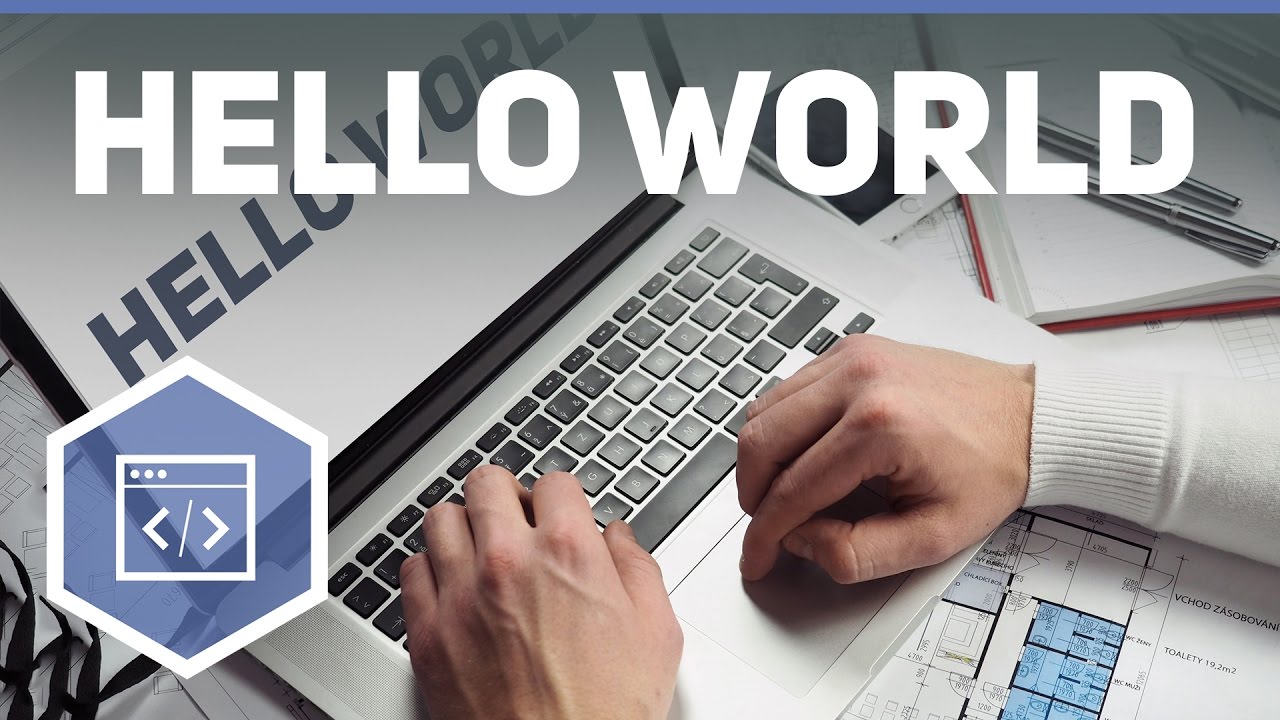
Hello World - Java Tutorial 2 Programm-Elemente Einstieg
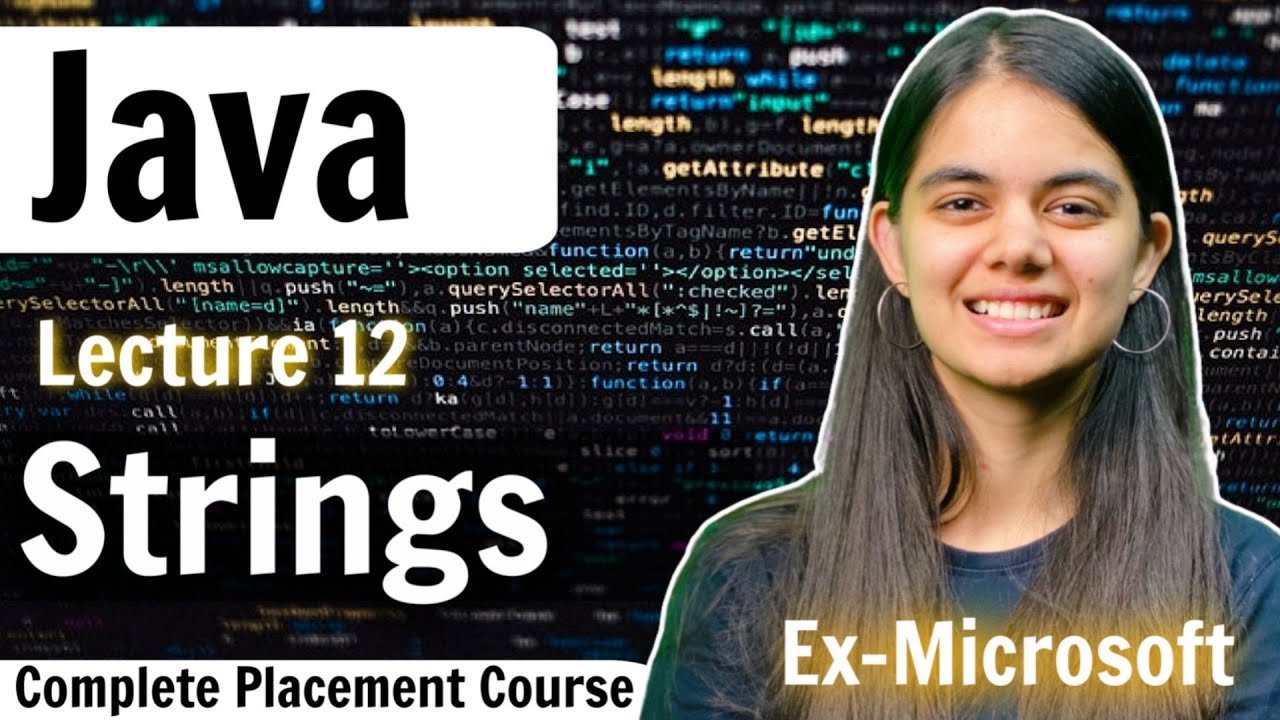
Strings | Lecture 12 | Java Placement Series
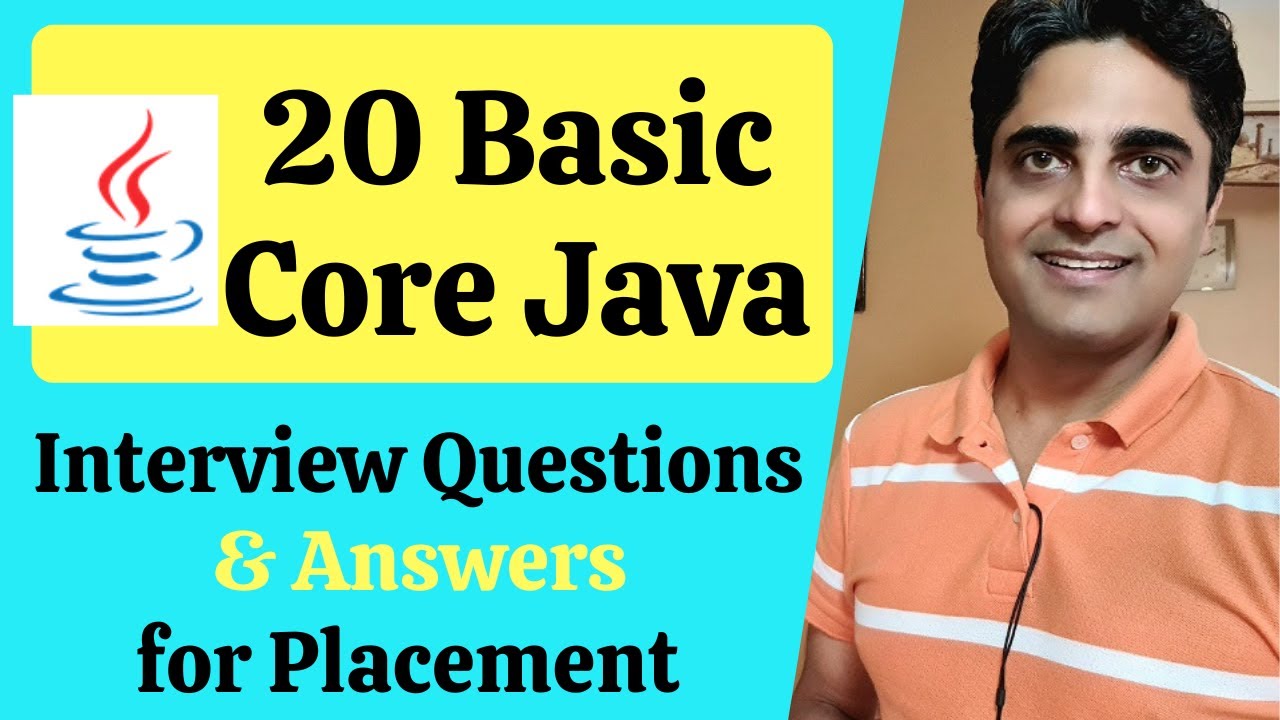
20 Basic Core Java Interview Questions & Answers- TCS, Accenture, Cognizant, Infosys, Wipro, HCL

#36 StringBuffer and StringBuilder in Java

INDIAN BUDGET EXPLAINED IN 10 MINUTES | Budget 2023 explained | Abhi and Niyu
5.0 / 5 (0 votes)