Why is every React site so slow?
Summary
TLDRThis video explores a significant performance issue in React: unnecessary re-renders that cause visual flickers on websites like GitHub. The speaker highlights how React’s virtual DOM checks can slow down apps and demonstrates how memoization can help improve performance. However, manually optimizing renders can be tedious, so the React compiler is introduced as a powerful tool that automatically handles memoization, speeding up React apps with minimal effort. The video also presents tools like React Scan and the Million project to aid developers in optimizing their React apps. Overall, the React compiler is shown as a game-changer for performance optimization.
Takeaways
- 😀 React has a render problem, where unnecessary re-renders can cause performance issues, such as flickering and slow page loads.
- 😀 The React virtual DOM helps optimize performance by checking what needs to be updated, but unnecessary checks still happen, leading to performance degradation.
- 😀 Components re-render even when there are no actual changes in state, which can be inefficient and cause lag.
- 😀 React uses diffing to compare the current virtual DOM with the new one, updating only the parts of the real DOM that have changed.
- 😀 React.memo and useMemo can help optimize performance by preventing unnecessary re-renders of components, but they require careful implementation.
- 😀 The React compiler can automatically handle memoization, improving performance without needing manual code adjustments.
- 😀 React's default behavior assumes that everything might change, causing re-renders even for unchanged props or state, which can lead to inefficiencies.
- 😀 React memoization can be tedious and error-prone, especially when passing inline objects or functions that are redefined on every render.
- 😀 Real-world applications like GitHub and Pinterest often experience performance issues due to inefficient rendering and lack of proper optimizations.
- 😀 Tools like React Scan can help developers visualize unnecessary re-renders and identify performance bottlenecks in their React apps.
- 😀 New tools and projects like Million are being developed to optimize React performance further, making apps run faster without needing major code changes.
Q & A
What is the primary issue discussed in the video?
-The primary issue discussed is the render problem in React applications, where unnecessary re-renders occur, leading to poor performance and visual flickers, especially on complex sites like GitHub.
How does React's virtual DOM help with performance?
-React's virtual DOM helps by providing an in-memory representation of the real DOM. It compares the previous state of the UI with the new state and updates only the parts of the DOM that have changed, reducing unnecessary updates.
What is the 'render problem' mentioned in the video?
-The render problem refers to unnecessary re-renders and checks occurring in React applications, which negatively impact performance. These are especially noticeable when the state changes high up in the component tree, causing lower-level components to re-render unnecessarily.
What role does memoization play in addressing the render problem?
-Memoization helps optimize rendering by ensuring that components only re-render when their props change. React's `React.memo` or `useMemo` hooks can be used to avoid unnecessary re-renders, though it's not always straightforward to implement.
Why is memoization not always used in practice, according to the video?
-Memoization is often avoided because it can be tedious to implement correctly and requires careful management of props and state. The speaker mentions that they don't typically use memoization unless absolutely necessary.
What is the React compiler, and how does it solve the render problem?
-The React compiler automatically optimizes re-renders by adding the right memoization and performance optimizations without requiring manual intervention. It detects and eliminates unnecessary re-renders, improving app performance significantly.
How does the React compiler differ from traditional memoization methods like `React.memo`?
-Unlike traditional memoization methods, the React compiler automatically applies the necessary optimizations without requiring manual wrapping of components with `React.memo` or managing `useCallback`. It handles performance optimizations more seamlessly.
What specific example does the speaker use to demonstrate the render problem?
-The speaker uses a demo app with a Color Picker, a counter, and a slow component that renders a large array. When the counter button is clicked, the slow component unnecessarily re-renders, causing lag. Enabling the React compiler fixes this issue by preventing unnecessary renders.
What happens when an inline object is passed as a prop in React?
-When an inline object is passed as a prop, React treats it as a new object on each render. This leads to unnecessary re-renders, as React assumes the object has changed, even if it hasn't.
How does the video suggest you can test render optimization on your React app?
-The video suggests using tools like React Scan and the React DevTools extension to visualize and analyze re-renders in your app. These tools allow you to easily see what is re-rendering and help identify performance bottlenecks.
Outlines
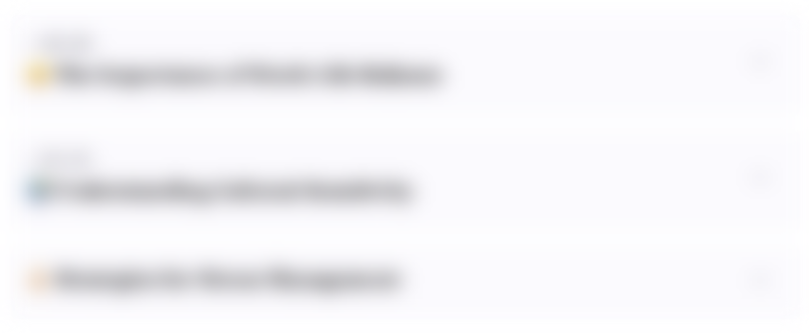
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
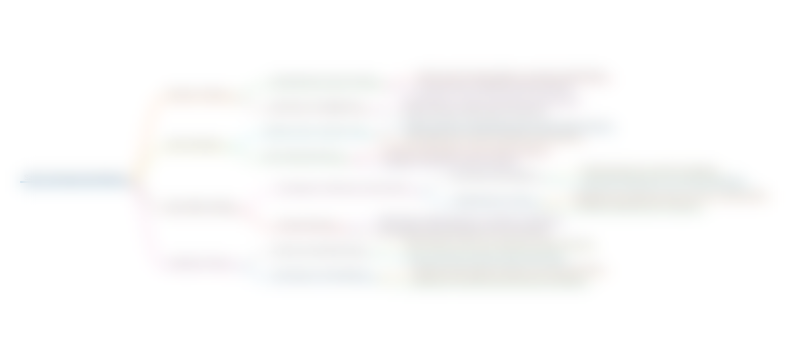
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
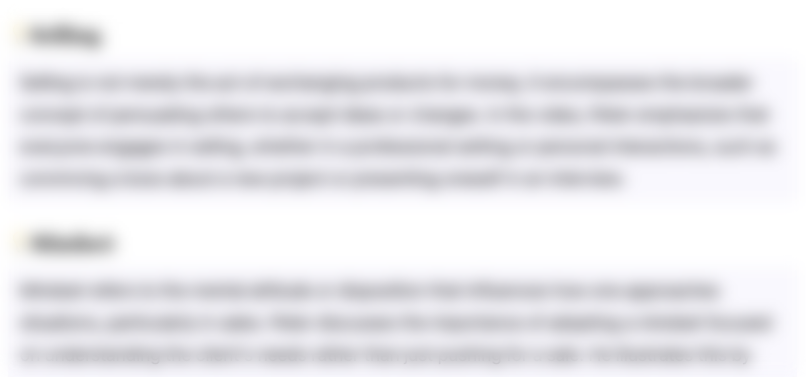
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
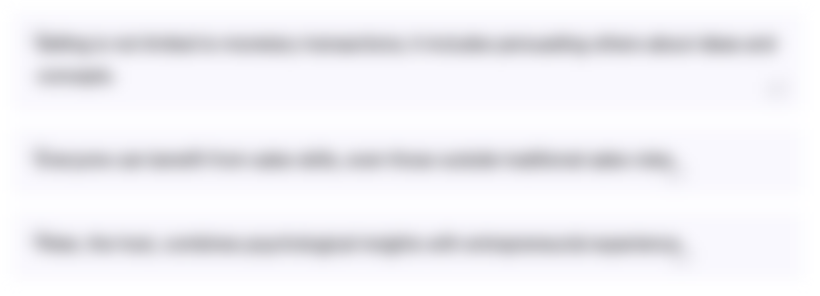
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
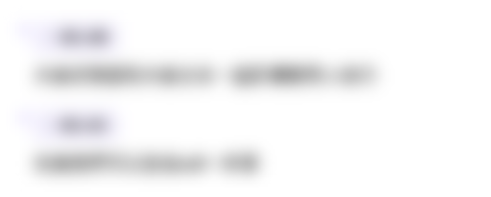
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
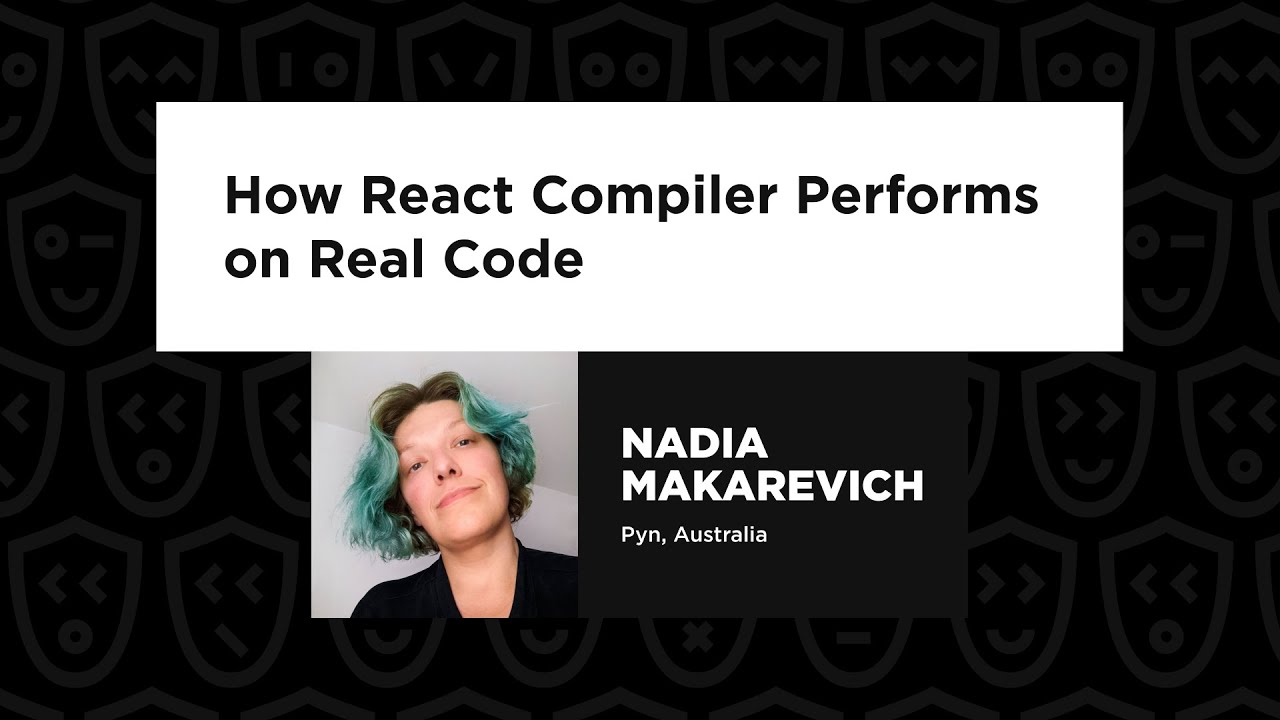
Nadia Makarevich – How React Compiler Performs on Real Code, React Advanced 2024
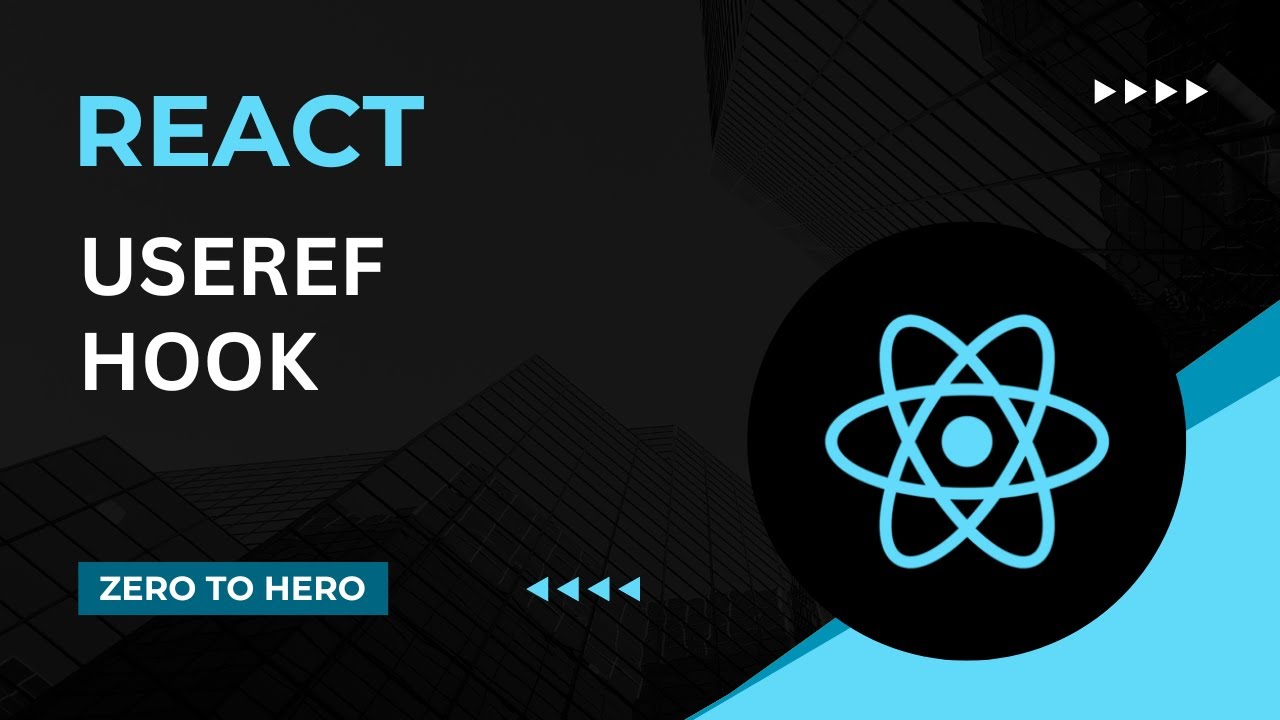
useRef Hook | Mastering React: An In-Depth Zero to Hero Video Series

Learn useRef in 11 Minutes

Elements, Children and Re-renders - Advanced React course, Episode 2

Performance Optimization and Wasted Renders | Lecture 239 | React.JS 🔥

States | Mastering React: An In-Depth Zero to Hero Video Series
5.0 / 5 (0 votes)