C_46 Arrays in C - part 1 | Introduction to Arrays
Summary
TLDRThis video tutorial introduces arrays in C programming, explaining why they are essential for managing large datasets. The presenter highlights the limitations of using individual variables and demonstrates how arrays can store multiple items under one variable name. Key topics covered include the need for arrays, array declaration syntax, common errors, and the benefits of using macros for defining array sizes. By practicing these concepts, viewers can gain a solid understanding of how to work with arrays in C and avoid typical mistakes. The video serves as a foundational guide for beginners in C programming.
Takeaways
- 😀 **Why Arrays are Important**: Arrays allow you to store multiple values under a single variable name, making it easier to handle large datasets (e.g., storing the roll numbers of 60 students).
- 😀 **Memory Allocation**: Arrays are stored in contiguous memory locations, which means each element occupies a consecutive block of memory. This makes accessing elements efficient.
- 😀 **Array Declaration Syntax**: The general syntax for declaring an array is: `data_type array_name[size];` where `data_type` is the type of elements (e.g., int, float), `array_name` is the name of the array, and `size` is the number of elements.
- 😀 **Type Consistency**: Arrays must store homogeneous data types, meaning all elements must be of the same type (e.g., all integers, all floats).
- 😀 **Declaration Example**: To declare an array to store 60 roll numbers of type `int`, you would write: `int rollNumbers[60];`.
- 😀 **Size of the Array**: The size of the array must be a constant value (e.g., 5, 10) and cannot be left undefined or negative. The size must be a positive integer or a constant expression.
- 😀 **Incorrect Array Declaration**: Declarations like `int numbers[];` or `int numbers[-5];` are incorrect and will result in compilation errors.
- 😀 **Array Initialization**: Arrays can be initialized at the time of declaration. For example: `int numbers[3] = {1, 2, 3};` initializes the array with the values 1, 2, and 3.
- 😀 **Macros for Array Size**: You can use macros to define the size of an array. For example: `#define SIZE 60` and then use `int rollNumbers[SIZE];`.
- 😀 **Practice is Key**: Watching the video alone isn't enough to learn arrays. It's important to practice coding on your own using an IDE to fully grasp the concepts of array declaration and initialization.
Q & A
Why are arrays needed in programming?
-Arrays are needed to store multiple data items of the same type under a single variable name. This allows for efficient management of data and avoids the need to declare many individual variables.
What is the definition of an array in C programming?
-An array in C is a collection of elements of the same type, stored in contiguous memory locations. It allows for efficient access and management of data.
What is the general syntax for declaring an array in C?
-The general syntax for declaring an array in C is: `data_type array_name[size];`, where `data_type` is the type of elements, `array_name` is the name of the array, and `size` is the number of elements the array can hold.
What happens if the array size is not specified during declaration?
-If the array size is not specified during declaration, the compiler will throw an error. The size must be provided as a constant value.
Can variables be used to specify the size of an array in C?
-No, variables cannot be used to specify the size of an array directly in C. The size must be a constant. However, macros can be used to define the size, which acts as a constant.
What is the main difference between a 1D array and a multi-dimensional array?
-A 1D array has only one row, while a multi-dimensional array (such as 2D or 3D) involves multiple rows and columns. A 2D array, for example, is like a table with rows and columns.
What are some common mistakes when declaring arrays in C?
-Common mistakes include not specifying the size, using variables instead of constants for the size, or incorrectly initializing arrays without defining their size.
How are array elements stored in memory?
-Array elements are stored in contiguous memory locations. For example, if an array starts at memory address `1000`, the next element would be at `1004` (assuming each element is 4 bytes).
What are the rules for initializing arrays in C?
-Arrays can be initialized at the time of declaration using curly braces. The values provided will be assigned to the array elements in order. If not initialized, the array contains undefined values (garbage values).
Why is it recommended to use macros for array size in C?
-Using macros for array size provides flexibility and makes the code easier to maintain. A macro allows the array size to be defined as a constant, making it easier to change the size in one place without needing to modify multiple instances in the code.
Outlines
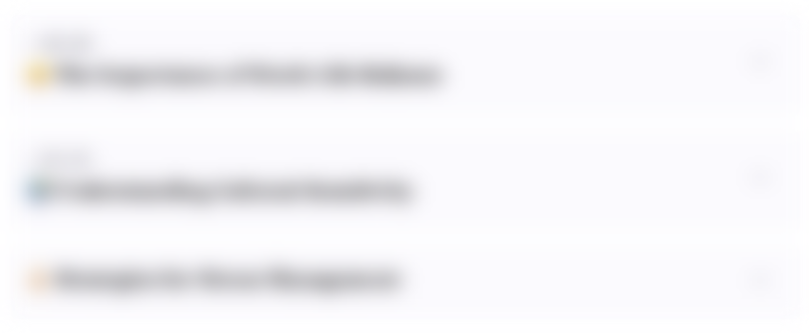
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
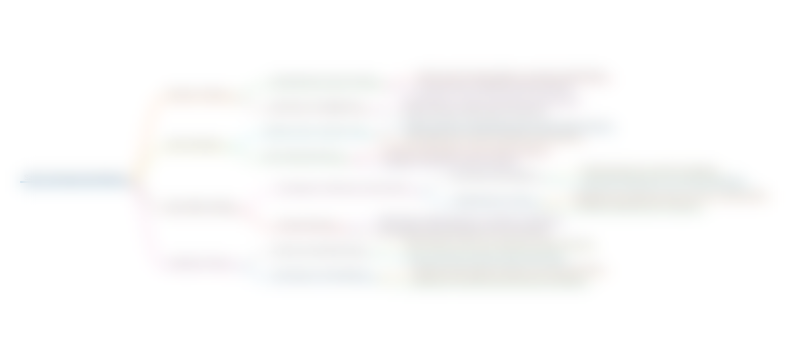
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
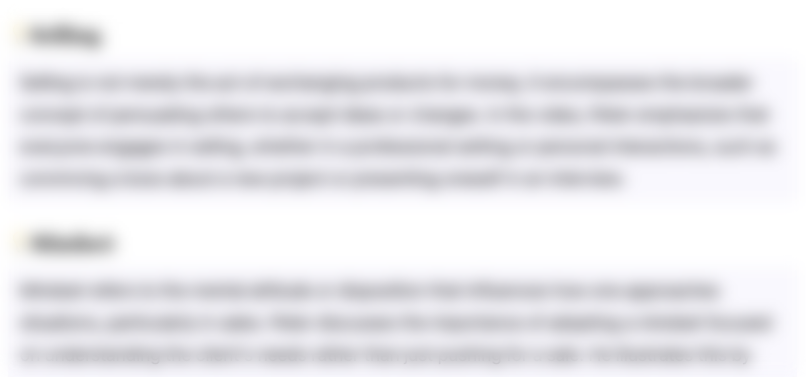
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
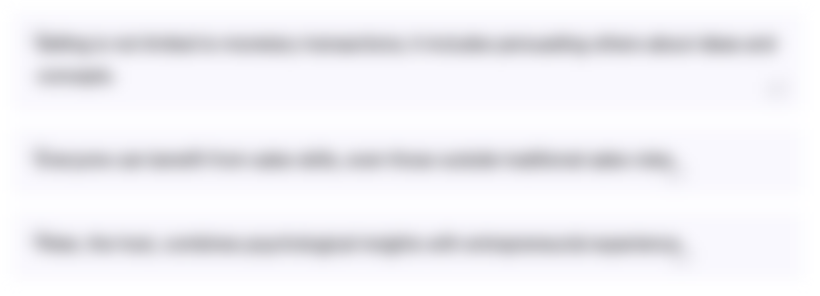
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
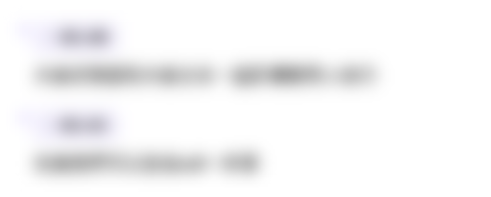
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
5.0 / 5 (0 votes)