Methods in Java Tutorial #26
Summary
TLDRIn this Java tutorial, Alex introduces the concept of methods, which allow you to organize and reuse code efficiently. He explains how to create and call methods to perform tasks such as multiplication and division, reducing repetitive code. Through examples, Alex demonstrates how methods simplify the process of writing reusable and maintainable code, making programming easier. He also covers the use of parameters in methods and how to call methods with different arguments. The tutorial emphasizes the importance of methods in keeping code clean and manageable, encouraging viewers to practice and subscribe for more content.
Takeaways
- 😀 Methods in Java allow you to reuse code efficiently by grouping related instructions into a single callable block.
- 😀 To define a method, use the syntax 'public static void methodName()' and include the code to be executed within curly braces.
- 😀 A method call replaces multiple lines of code, making programs cleaner and easier to maintain.
- 😀 Methods can take input through parameters, allowing you to pass values into the method when you call it.
- 😀 You can call methods multiple times with different arguments, avoiding repetitive code.
- 😀 The 'public static void main' method is the entry point of a Java program, where execution starts.
- 😀 To create a method, specify a return type (like 'void' if no value is returned) and a name that describes its purpose.
- 😀 Using methods in your program makes it easier to modify values or logic, as you only need to change it in one place.
- 😀 A method can be used to perform specific tasks, such as printing output or performing calculations, like multiplication and division.
- 😀 By practicing methods, you learn how to break complex tasks into smaller, manageable chunks, improving code organization and readability.
Q & A
What is a method in Java?
-A method in Java is a block of code that performs a specific task and can be called to execute that task using just one line of code.
How do you define a method in Java?
-To define a method in Java, you use the following syntax: `public static void methodName() { // code }`, where `public static void` are modifiers and `methodName` is the name of your method.
Why are methods important in Java programming?
-Methods are important because they allow you to reuse code, reduce redundancy, make your program more readable, and maintainable.
What is the purpose of the 'public static void' keywords in a method definition?
-'Public' makes the method accessible from other classes, 'static' means the method can be called without creating an instance of the class, and 'void' indicates the method does not return a value.
What is the difference between calling a method and writing the code directly in the main method?
-Calling a method reduces redundancy by allowing you to use the same block of code multiple times with different inputs, whereas writing the code directly would require repeating the same block each time.
Can you pass parameters to a method in Java? If so, how?
-Yes, you can pass parameters to a method by including them in the parentheses after the method name, like `methodName(int a, int b)`, and then using those parameters inside the method.
How does the 'multiply' method in the script work?
-The 'multiply' method takes two integers as parameters, multiplies them, and prints the result. It's called by passing two numbers as arguments, like `multiply(5, 10)`.
What are the advantages of using methods like 'multiply', 'welcome', and 'divide' in the script?
-These methods improve code readability, reusability, and maintainability by avoiding redundant code and grouping related functionality into a single, callable block.
How would you modify the 'multiply' method to return the result instead of printing it?
-To modify the 'multiply' method to return the result, you would change the return type from 'void' to 'int' and use `return a * b;` instead of `System.out.println(a * b);`.
What is the significance of calling methods like 'welcome()' in the script?
-The 'welcome()' method is used to provide a reusable welcome message, demonstrating how methods can encapsulate specific tasks and make the code more organized.
Outlines
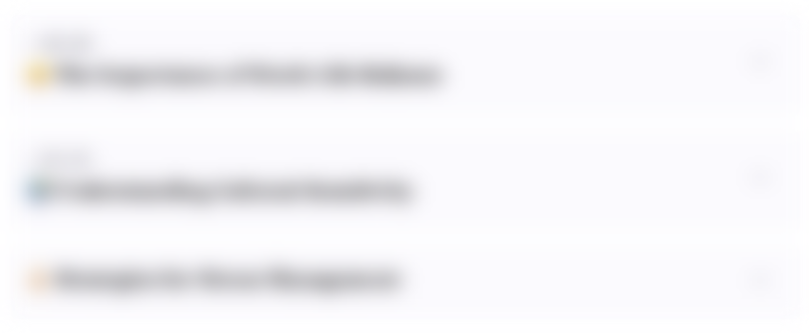
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
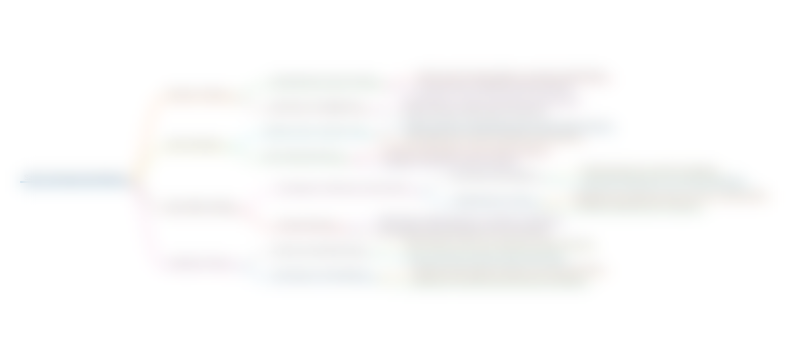
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
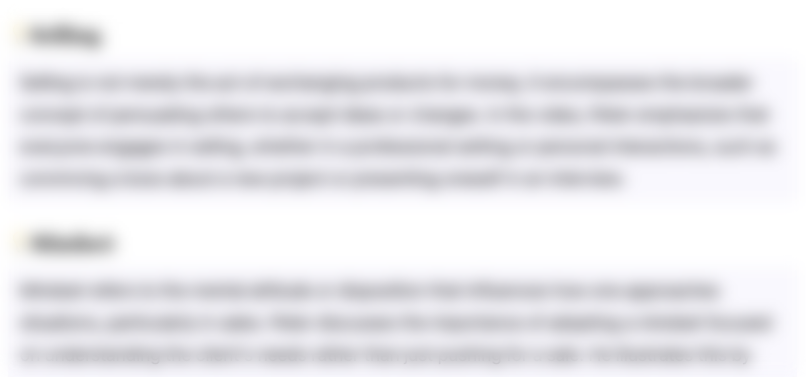
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
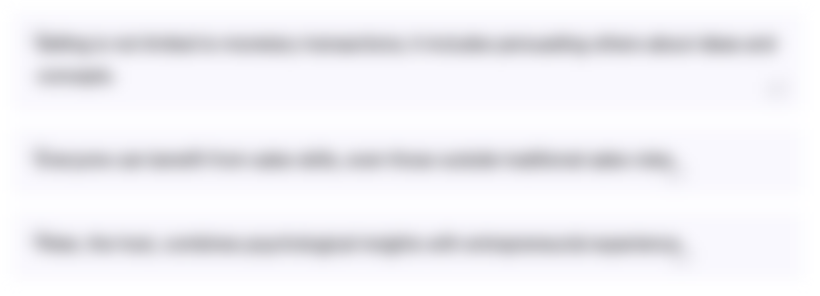
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
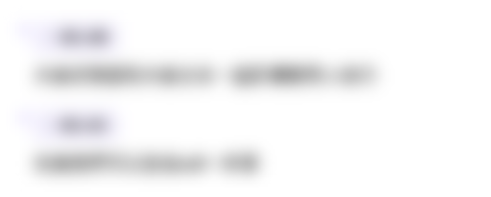
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة

Java Inheritance | Java Inheritance Program Example | Java Inheritance Tutorial | Simplilearn
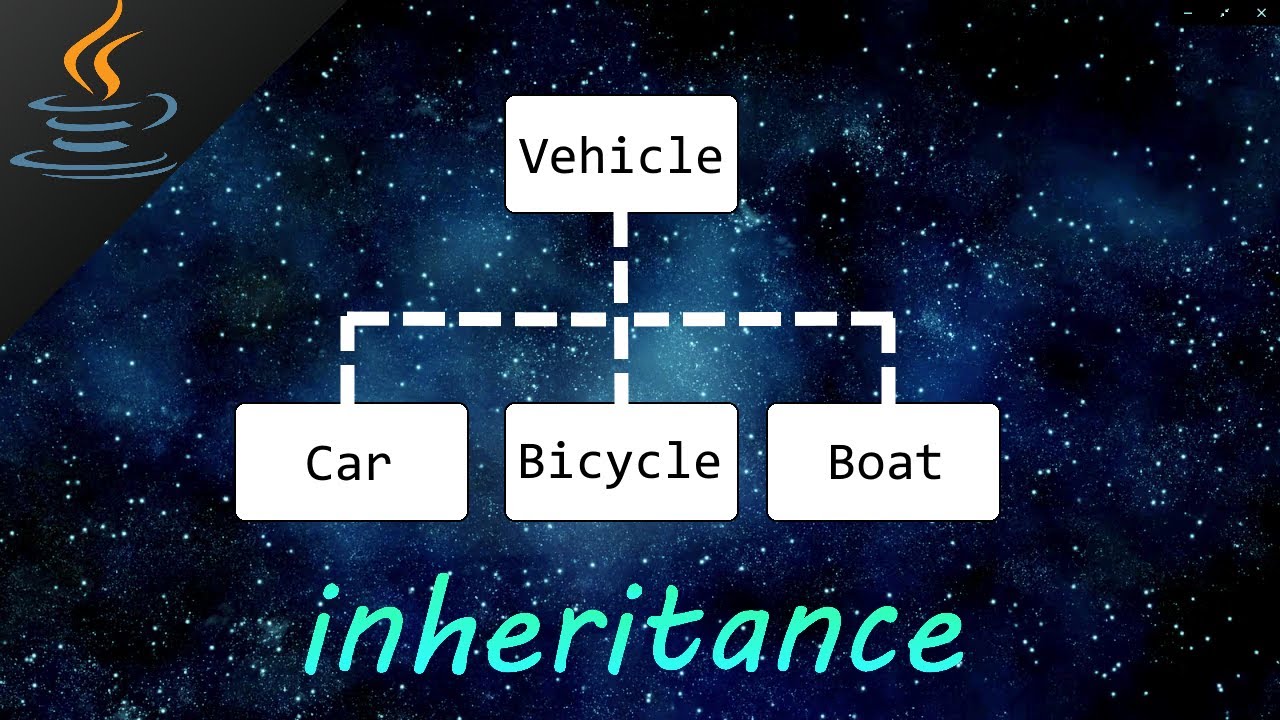
Java inheritance 👪
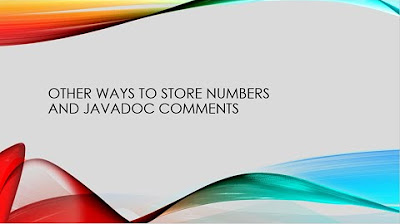
Stage 5 #4 Number Classes like BigInteger And Javadoc Comments
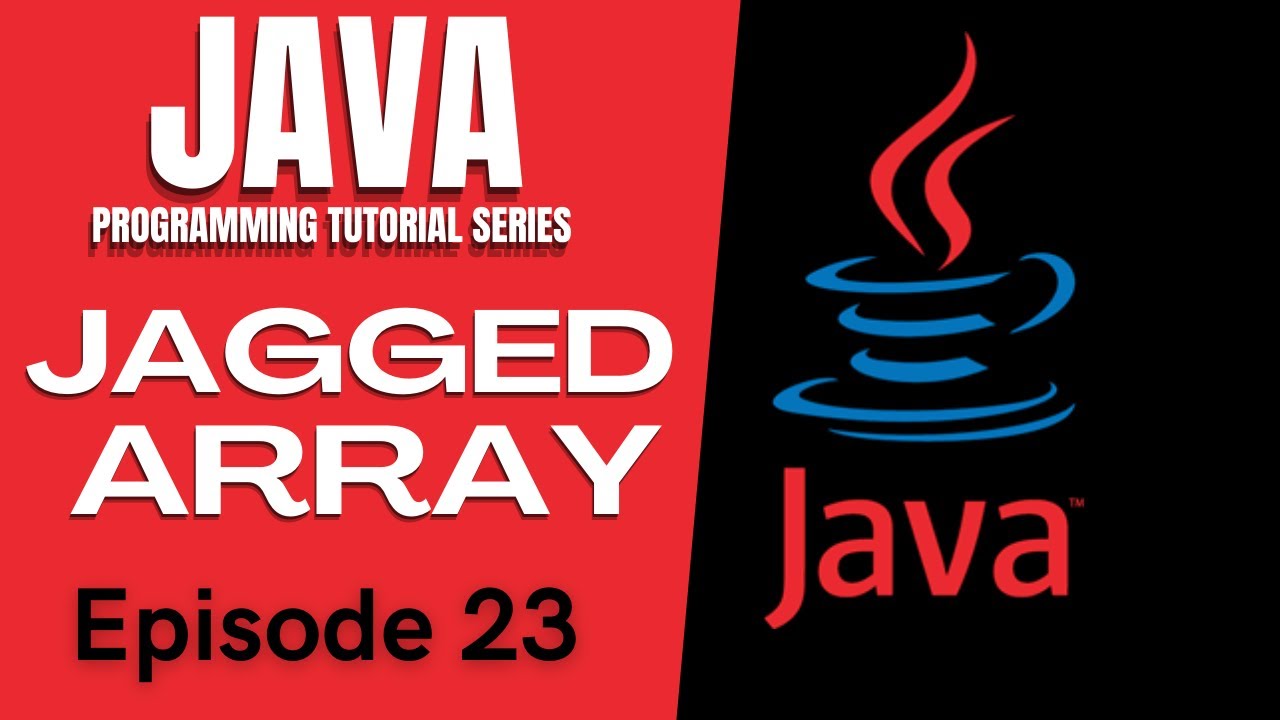
Java Tutorial #23 | 2D JAGGED ARRAYS IN JAVA | RAGGED |Tagalog | English | Filipino | 2021
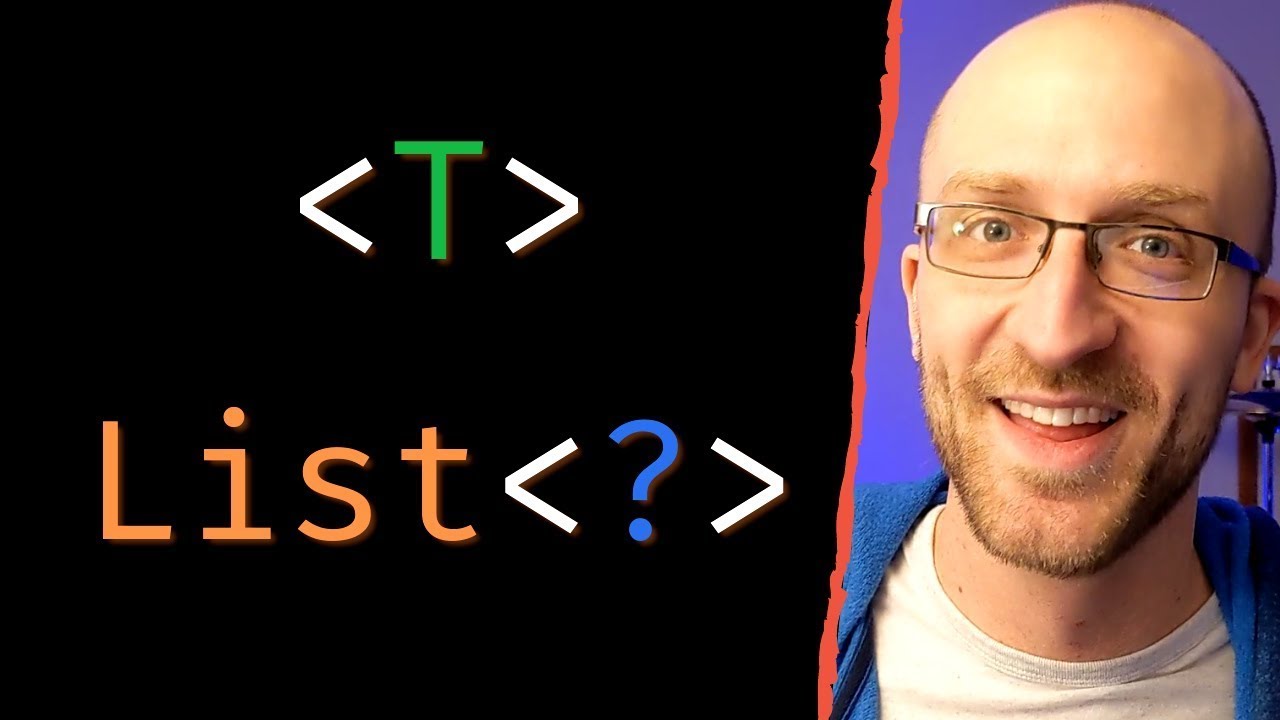
Generics In Java - Full Simple Tutorial
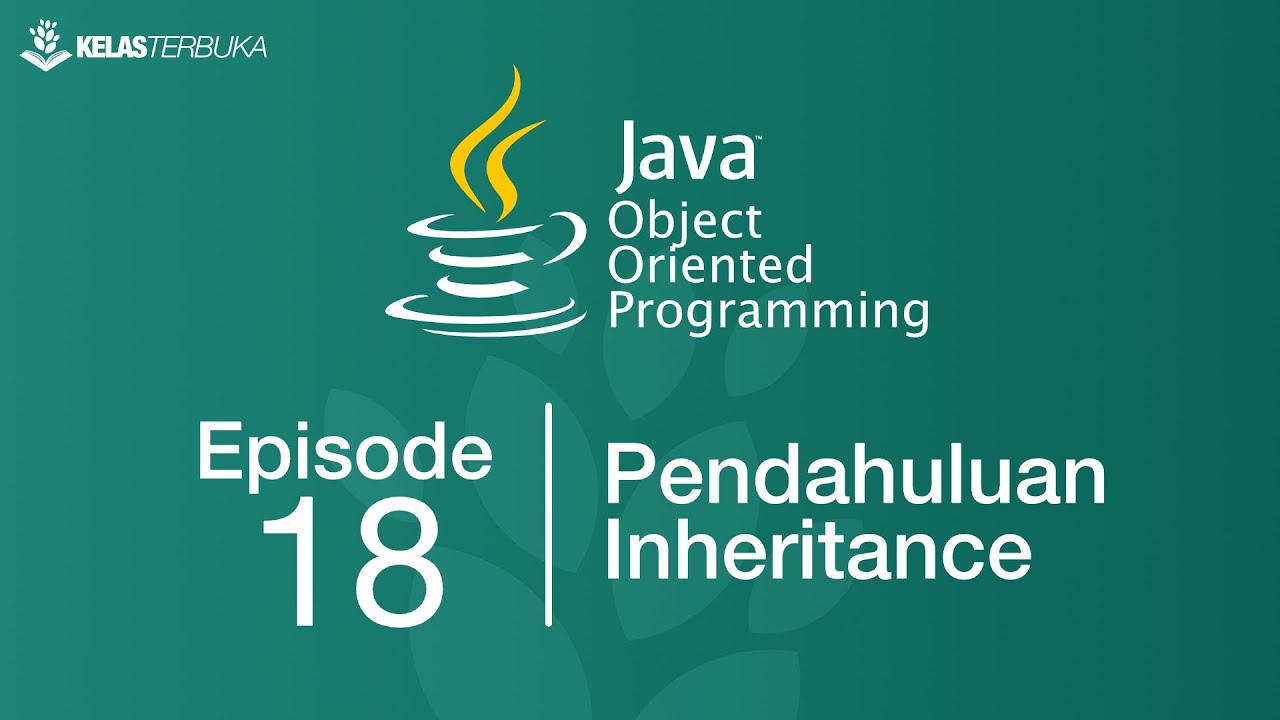
Belajar Java [OOP] - 18 - Pengenalan Inheritance
5.0 / 5 (0 votes)