C_16 Operators in C - Part 4 | C Programming Tutorials
Summary
TLDRThis tutorial explains the use of relational operators in C programming, which are essential for comparing values, expressions, or variables. It covers various relational operators such as less than, greater than, equal to, and not equal to, providing practical examples. The video also explores how relational operators are used in decision-making structures like if-else statements. Key points include the distinction between assignment and comparison operators, and considerations when comparing characters, strings, and floating-point numbers. The video concludes with a sample program demonstrating the practical use of these operators.
Takeaways
- 😀 Relational operators in C compare two values and return either true (1) or false (0), which are stored as integers in memory.
- 😀 The main relational operators in C are: `==`, `!=`, `>`, `<`, `>=`, and `<=`.
- 😀 Relational operators are commonly used in decision-making structures like `if-else` statements to evaluate conditions.
- 😀 `==` is an equality operator (not to be confused with the assignment operator `=`).
- 😀 When comparing integers, floats, and characters, relational operators help determine their relationships based on their values or ASCII codes.
- 😀 Boolean logic in C treats `true` as 1 and `false` as 0, which are the output of relational comparisons.
- 😀 C allows comparisons between constants, variables, and even expressions involving arithmetic operations.
- 😀 The comparison of characters in C is based on their ASCII values (e.g., 'a' = 97, 'b' = 98), and relational operators work with those values.
- 😀 Relational operators cannot be directly used to compare strings in C. They compare memory addresses instead of actual string content, which leads to undefined behavior.
- 😀 When dealing with floating-point numbers, it's important to avoid using relational operators like `==` or `!=` due to precision issues, which can lead to unexpected results.
- 😀 Operator precedence plays a critical role in evaluating complex expressions. Arithmetic operators have higher precedence than relational operators, which affects the order of evaluation in expressions.
Q & A
What are relational operators in C programming?
-Relational operators in C are used to compare two values or expressions and determine their relationship. They include operators such as less than (`<`), greater than (`>`), less than or equal to (`<=`), greater than or equal to (`>=`), equal to (`==`), and not equal to (`!=`).
What type of values do relational operators return?
-Relational operators return a boolean value, represented as `1` for true and `0` for false in C programming. These are stored in memory as integer values.
Can relational operators be used to compare variables and expressions?
-Yes, relational operators can compare constants, variables, and expressions. For example, expressions like `a + b < c + d` or `x - y > 10` can also be compared using relational operators.
How do relational operators work with characters in C?
-Relational operators work with characters by comparing their ASCII values. For example, the character `'a'` has an ASCII value of 97, and `'b'` has 98. So `'a' < 'b'` is true because 97 is less than 98.
Why can't strings be compared using relational operators in C?
-Strings cannot be compared directly using relational operators in C because they are stored in memory as sequences of characters, and relational operators compare the memory addresses rather than the content. This can lead to undefined behavior.
What is the difference between `=` and `==` in C programming?
-`=` is the assignment operator used to assign a value to a variable, whereas `==` is the equality operator used to check if two values are equal. For example, `a = 5` assigns 5 to `a`, while `a == 5` checks if `a` is equal to 5.
How do relational operators behave when used with floating-point numbers?
-Relational operators can be used with floating-point numbers, but comparisons may not always be accurate due to precision issues. It's generally advised to avoid using `==` and `!=` for floating-point numbers unless high precision is not required.
What is the associativity of relational operators in C?
-Relational operators in C have left-to-right associativity. This means that when multiple relational operators are used in a single expression, they are evaluated from left to right.
What would happen if we compare the strings 'Jenny' and 'Lectures' using relational operators?
-Comparing strings like `'Jenny' < 'Lectures'` using relational operators in C would not compare their contents. Instead, it would compare the memory addresses where the strings are stored, leading to undefined behavior.
What is the output of the relational expression `a < b < c` where `a = 18`, `b = 9`, and `c = 10`?
-The expression `a < b < c` would be evaluated in steps. First, `a < b` is false (`0`), so the expression becomes `0 < c`. Since `0 < 10` is true, the final output is `1`.
Outlines
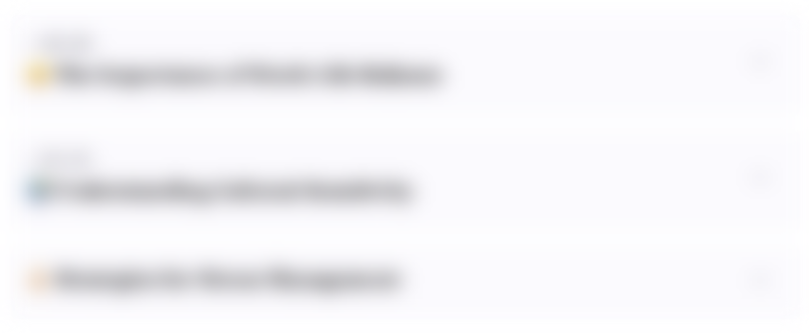
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
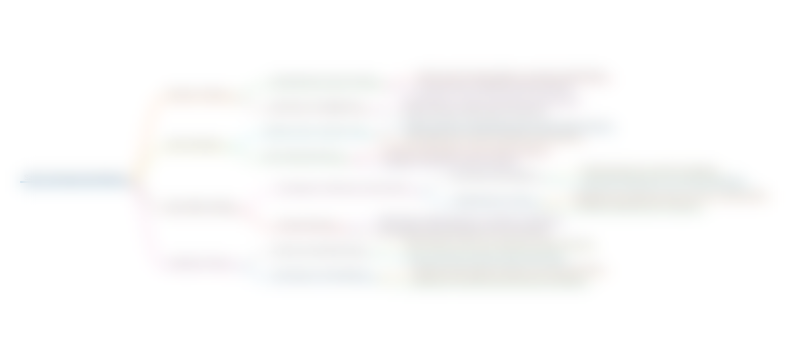
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
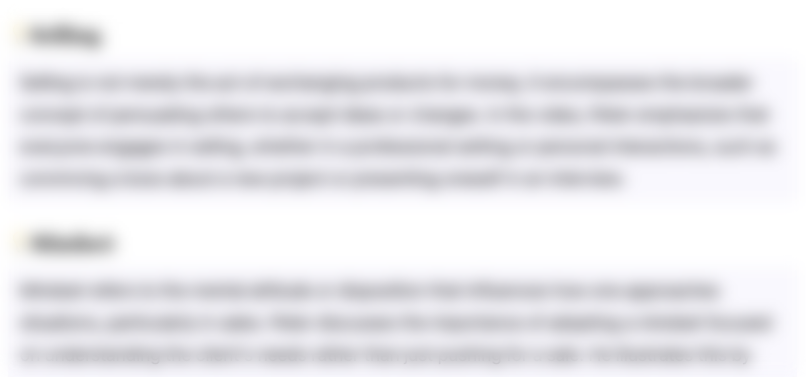
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
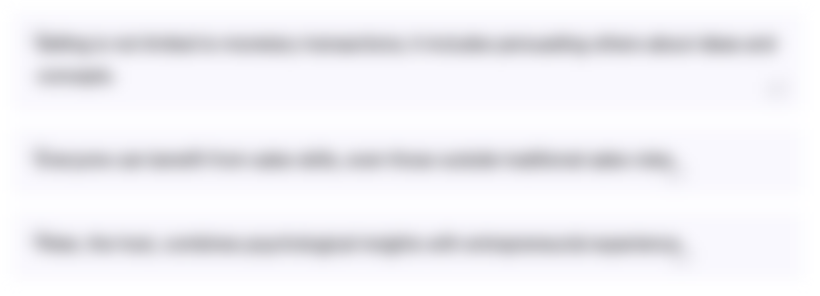
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
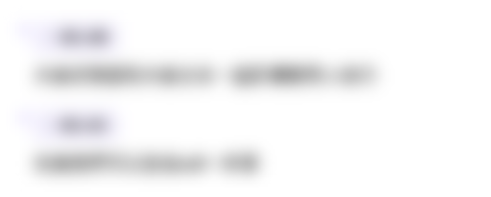
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة

#11 Python Tutorial for Beginners | Operators in Python
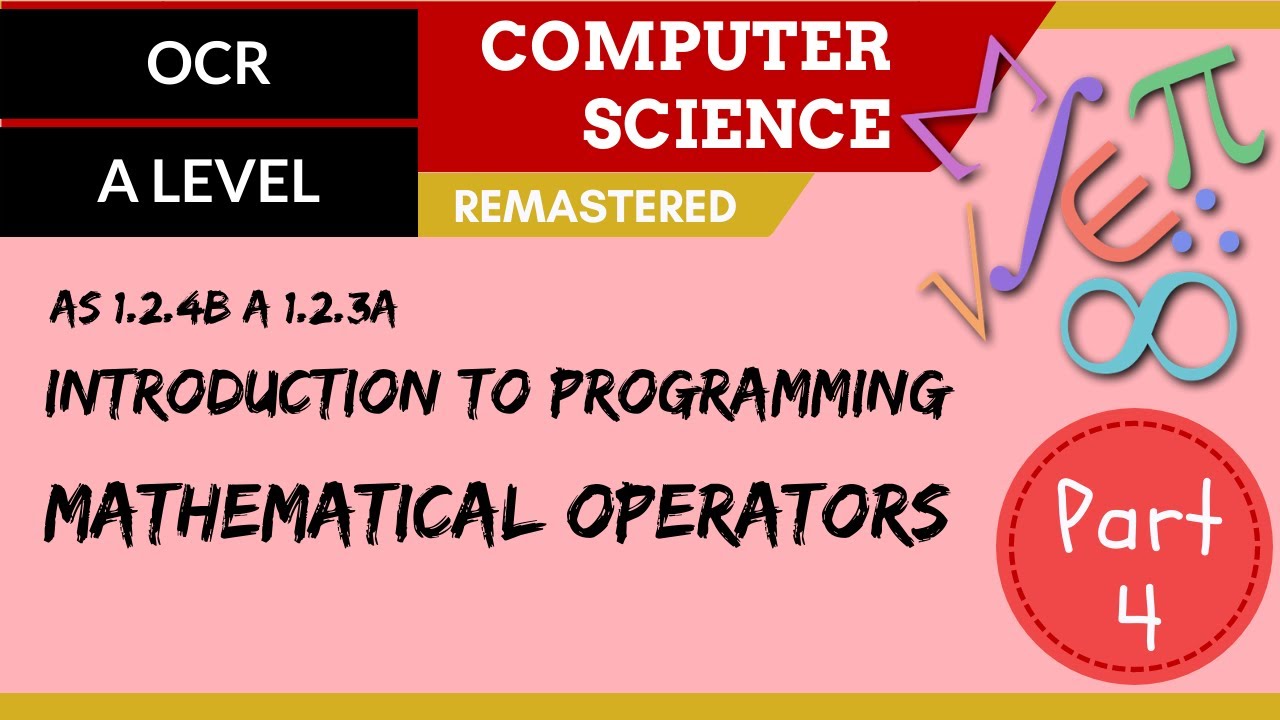
43. OCR A Level (H046-H446) SLR8 - 1.2 Introduction to programming part 4 mathematical operators
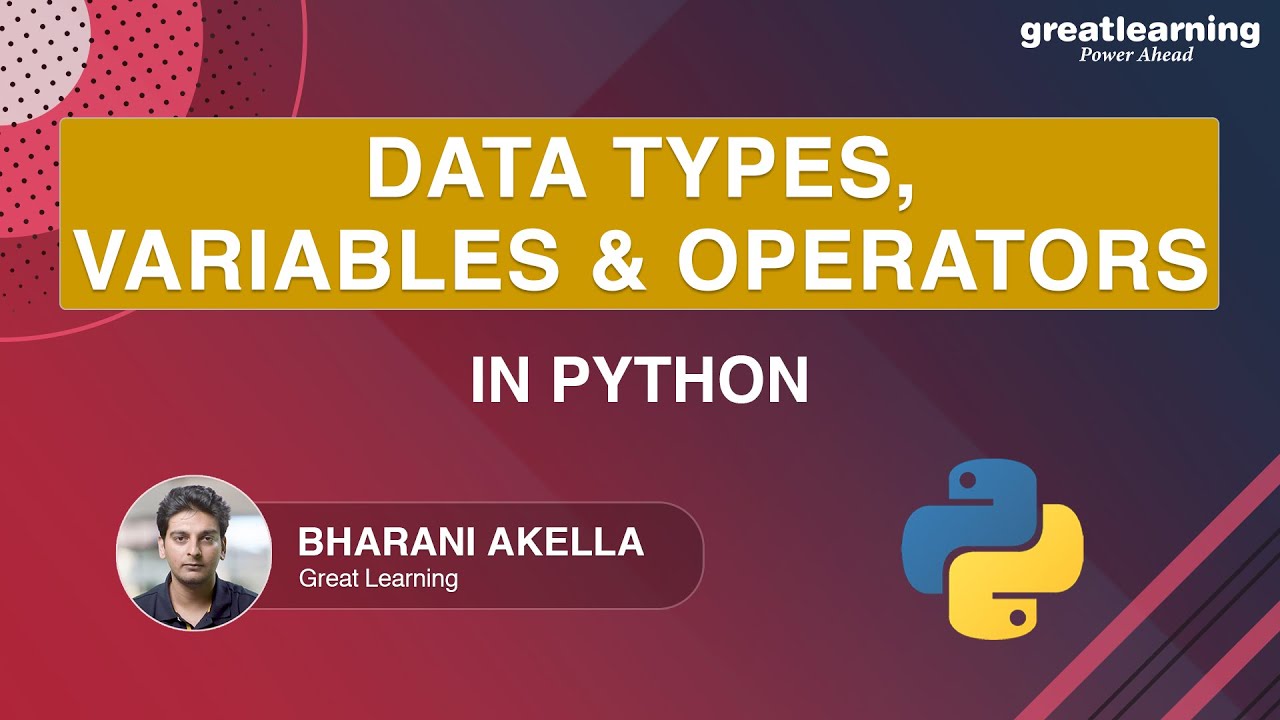
Data Types Variables And Operators In Python | Python Fundamentals | Great Learning
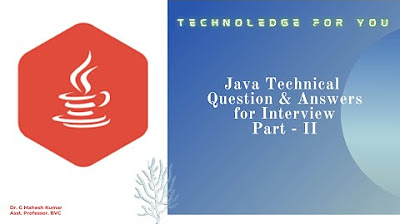
JAVA TECHNICAL QUESTION & ANSWERS FOR INTERVIEW PART - II
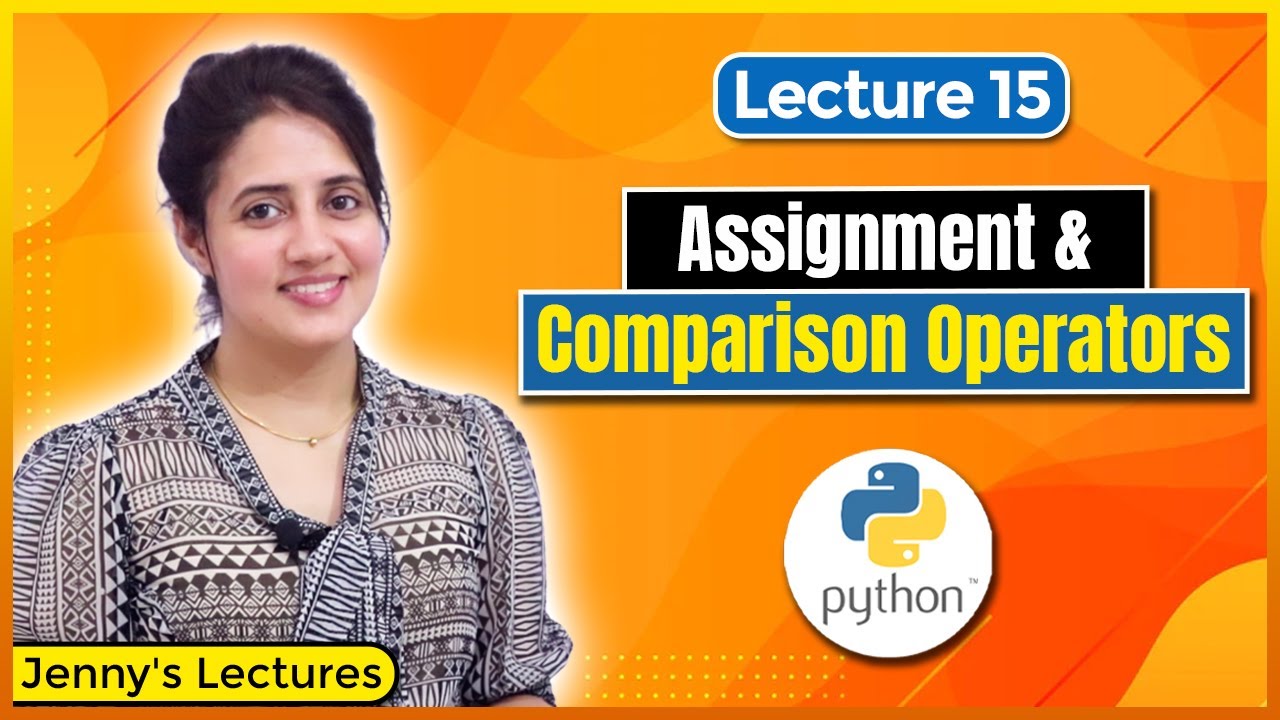
P_15 Operators in Python | Assignment and Comparison Operators | Python Tutorials for Beginners
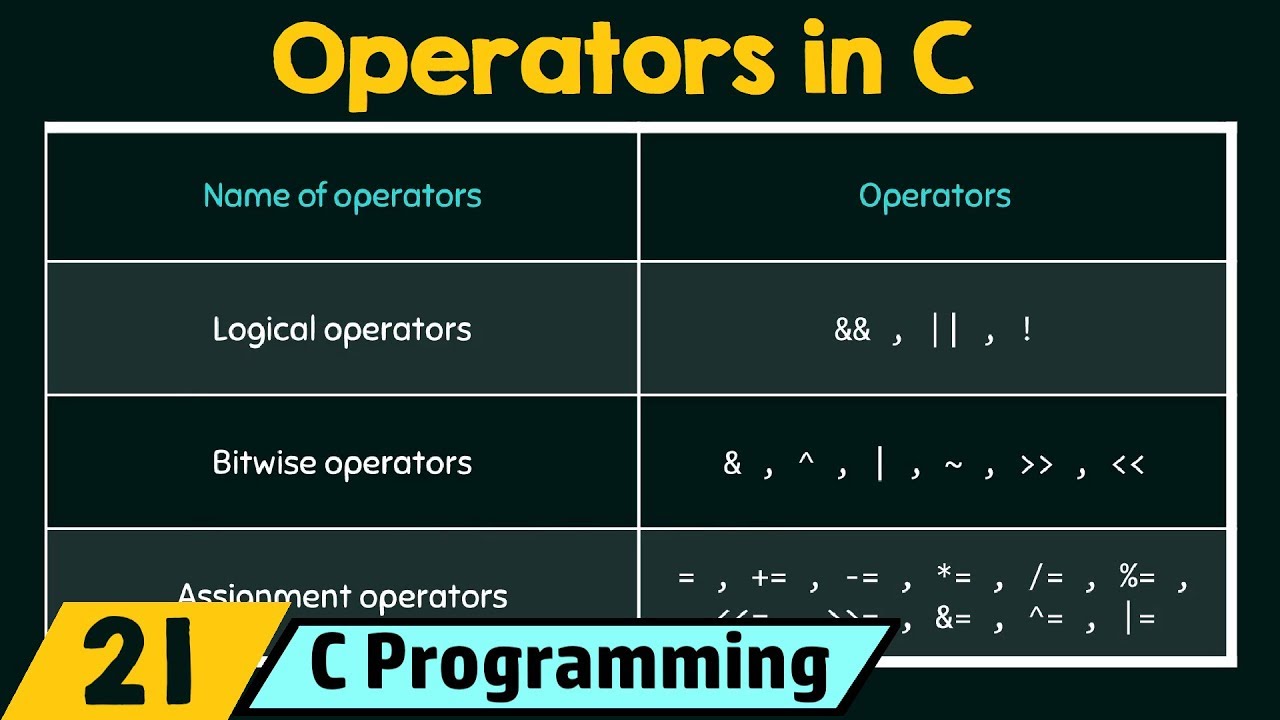
Introduction to Operators in C
5.0 / 5 (0 votes)