AP CS A - 7.3 Traversing ArrayLists
Summary
TLDRIn this lesson, we explore how to traverse ArrayLists, highlighting the key differences from arrays. We discuss using loops, such as while and enhanced for loops, to access elements with proper index handling to avoid common pitfalls like IndexOutOfBoundsException. A practical example illustrates the challenges of removing items, specifically when modifying the list during iteration, which can lead to skipping elements or throwing ConcurrentModificationException. By incorporating techniques to manage index adjustments and removal logic, learners gain crucial skills for effectively manipulating ArrayLists in Java.
Takeaways
- 😀 Traversing ArrayLists involves moving through all items, similar to arrays but with different methods.
- 😀 Use `size()` and `get(index)` for ArrayLists instead of `length` and brackets used in arrays.
- 😀 When traversing, ensure index values remain within the range of 0 to size - 1 to avoid errors.
- 😀 In for loops, an index variable `i` starts at 0 and increments through the ArrayList size.
- 😀 Removing elements while traversing can cause skipped values; use `i--` after a remove to fix this.
- 😀 While loops require careful index management, incrementing only when an element isn't removed.
- 😀 Enhanced for loops should be avoided when modifying the ArrayList to prevent `ConcurrentModificationException`.
- 😀 Arrays can have fixed sizes, while ArrayLists can change, affecting how they are traversed.
- 😀 Always initialize loop counters properly to prevent off-by-one errors.
- 😀 Practicing ArrayList traversal in environments like CodeHS can help reinforce these concepts.
Q & A
What is the main focus of the lesson?
-The lesson focuses on how to traverse ArrayLists in Java, highlighting the differences between ArrayLists and arrays.
How do you access elements in an ArrayList?
-Elements in an ArrayList are accessed using the `size()` method and the `get(index)` method instead of using `length` and brackets like in arrays.
What indexing range do ArrayLists use?
-ArrayList indices range from zero to `size - 1`, meaning the last index is always one less than the size of the ArrayList.
What happens if you try to access an index greater than `size - 1`?
-Attempting to access an index greater than `size - 1` results in an `IndexOutOfBoundsException`.
What types of loops can be used to traverse an ArrayList?
-You can use for loops, while loops, and enhanced for loops to traverse an ArrayList.
What issue arises when removing items from an ArrayList while iterating with a for loop?
-Removing an item shifts subsequent elements down, which can cause the loop to skip the next element because the index increments after a removal.
How can you prevent skipping elements during removal in a for loop?
-You can prevent skipping by decrementing the index variable (`i--`) after a removal to recheck the current index in the next iteration.
What is a concurrent modification exception?
-A concurrent modification exception occurs when an ArrayList is modified while it is being iterated over, especially when using enhanced for loops.
When is it not advisable to use enhanced for loops with ArrayLists?
-It is not advisable to use enhanced for loops when adding or removing items from an ArrayList, as this can lead to concurrent modification exceptions.
What is the significance of properly managing index values when traversing ArrayLists?
-Properly managing index values is crucial to ensure all elements are accessed correctly and to avoid errors such as skipping elements or running into exceptions.
Outlines
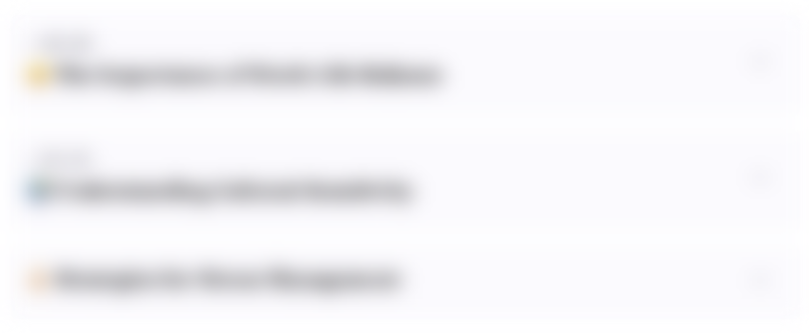
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
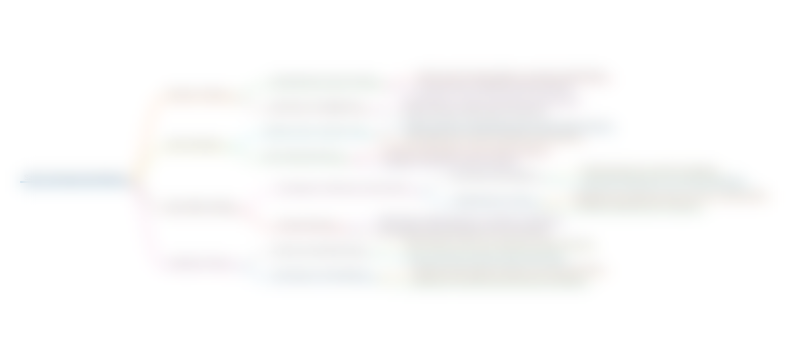
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
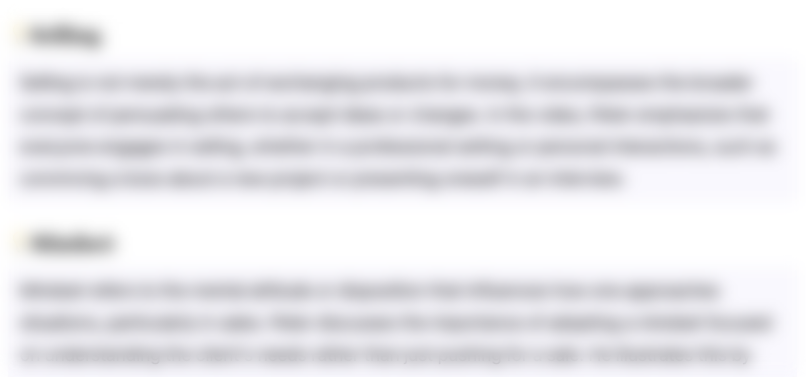
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
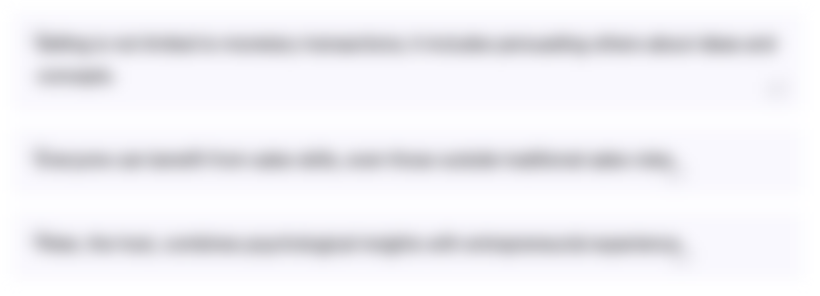
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
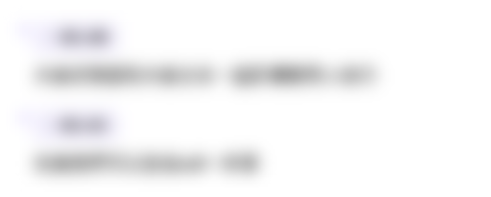
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآن5.0 / 5 (0 votes)