AP CS A - 2.8 Wrapper Classes
Summary
TLDRThis lesson introduces Java's wrapper classes, essential for storing primitive data types in collections like ArrayLists, which only accept objects. It explains the relationship between primitive types (e.g., int, double) and their corresponding wrapper classes (Integer, Double). Key concepts include instantiation, utility methods for conversion, and the processes of autoboxing and unboxing, which allow seamless interaction between primitives and wrapper objects. By the end of the lesson, learners gain insight into how these concepts enhance code readability and usability in Java.
Takeaways
- 😀 Wrapper classes are essential for converting primitive types to object types in Java.
- 😀 Each primitive type (e.g., int, double) has a corresponding wrapper class (e.g., Integer, Double).
- 😀 ArrayLists can only store object types, making wrapper classes necessary for storing primitive values.
- 😀 Wrapper classes have built-in methods for converting between primitive and object types, like intValue() and doubleValue().
- 😀 Autoboxing allows automatic conversion from a primitive type to its corresponding wrapper class.
- 😀 Unboxing is the reverse process, converting a wrapper class back to its primitive type.
- 😀 Java's compiler automatically handles autoboxing and unboxing, simplifying code for programmers.
- 😀 Wrapper classes are part of the java.lang package, meaning they are built into the Java platform and don't require additional imports.
- 😀 Integer.MIN_VALUE and Integer.MAX_VALUE can be used to set variable bounds for arrays.
- 😀 Understanding wrapper classes and their methods is crucial for writing clean and efficient Java code.
Q & A
What are wrapper classes in Java?
-Wrapper classes in Java are classes that encapsulate primitive data types in an object, allowing primitives to be treated as objects.
Why are wrapper classes necessary in Java?
-Wrapper classes are necessary because certain data structures, like ArrayLists, can only store object types and not primitive values. They enable the storage and manipulation of primitive values in these object-only contexts.
What is the difference between primitive types and wrapper classes in Java?
-Primitive types are built-in data types in Java, such as int and double, while wrapper classes are object representations of these primitive types, such as Integer and Double.
How do you instantiate a wrapper class object?
-To instantiate a wrapper class object, you use the 'new' operator followed by the class name and provide the corresponding primitive value as an argument. For example, 'Integer myInteger = new Integer(5);'.
What methods do the Integer and Double wrapper classes provide?
-The Integer and Double wrapper classes provide methods like intValue() and doubleValue(), which convert the wrapper objects back into their respective primitive types.
What is autoboxing in Java?
-Autoboxing is the automatic conversion of a primitive type into its corresponding wrapper class. For example, assigning an int to an Integer variable is done automatically by the Java compiler.
What is unboxing in Java?
-Unboxing is the reverse process of autoboxing, where the Java compiler automatically converts a wrapper class object back into its corresponding primitive type.
How do autoboxing and unboxing improve code readability?
-Autoboxing and unboxing allow programmers to use primitive types and wrapper objects interchangeably without explicit casting, resulting in cleaner and more readable code.
What package do the Integer and Double classes belong to?
-The Integer and Double classes are part of the java.lang package, which is built into the Java platform and does not require explicit importation.
What are Integer.MIN and Integer.MAX used for?
-Integer.MIN and Integer.MAX are constants that represent the minimum and maximum possible values for an Integer object, which can be useful for defining upper and lower bounds in programming.
Outlines
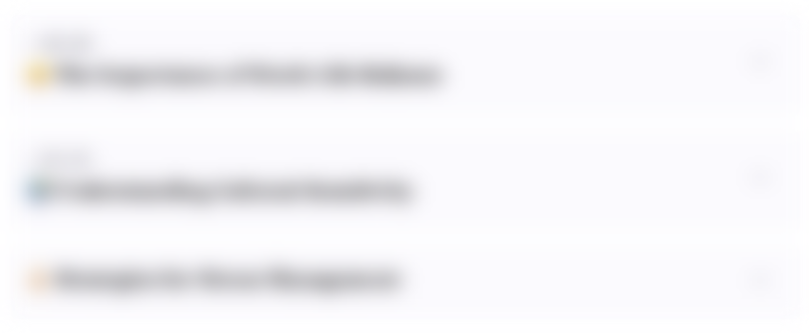
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
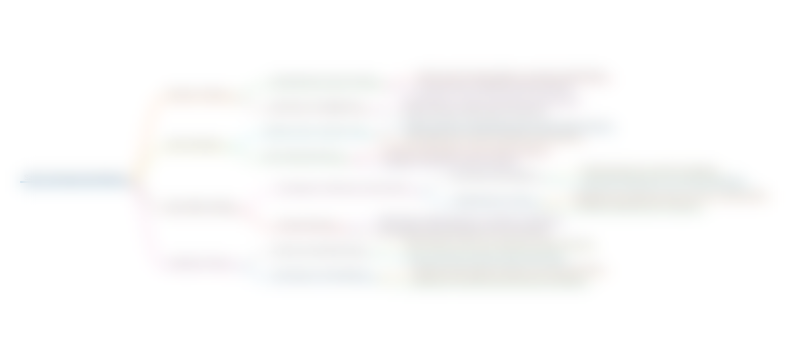
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
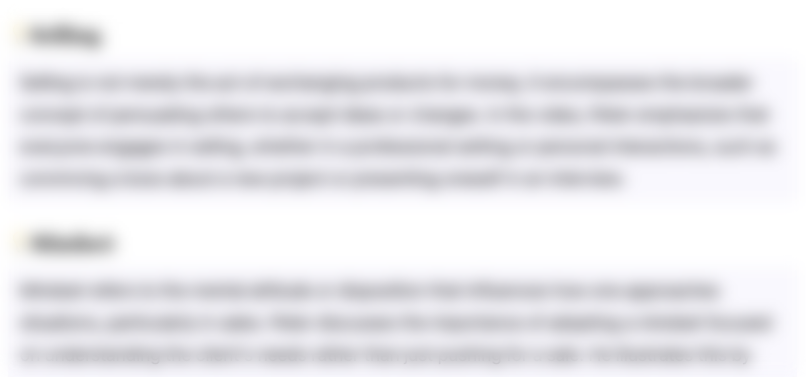
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
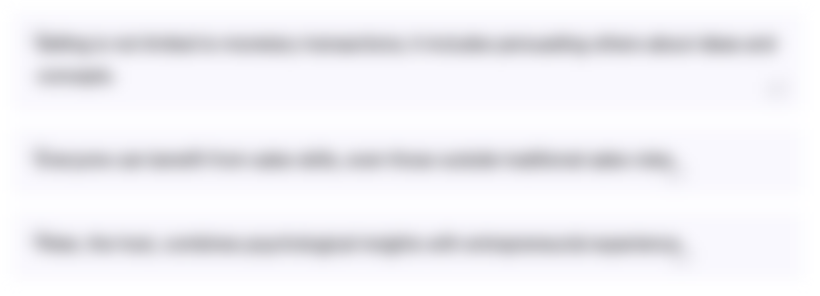
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
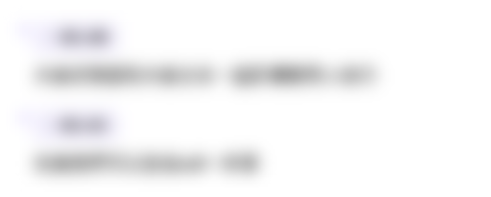
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
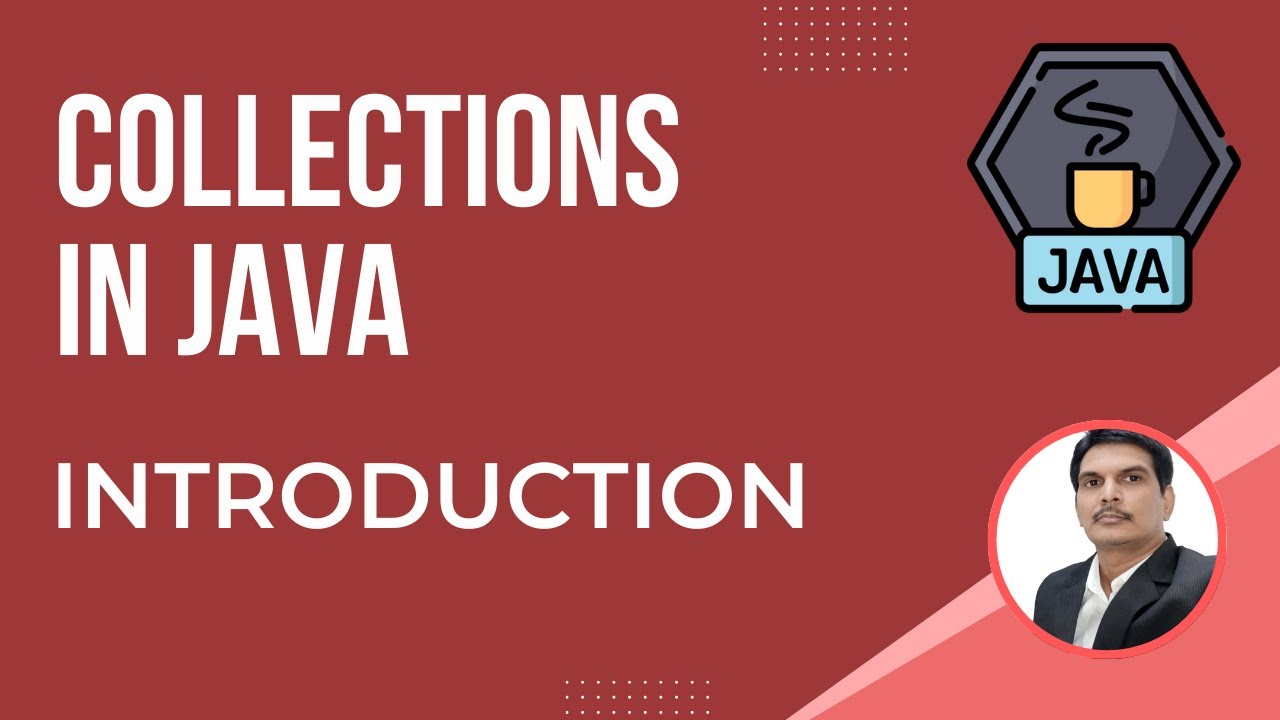
Java Collections Framework-Part1 | Collection Vs Collection Framework

AP CS A - 2.1 Instances of Classes

Data Types in Java | Master DSA in Java
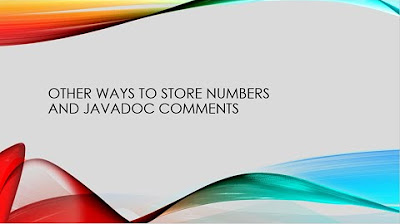
Stage 5 #4 Number Classes like BigInteger And Javadoc Comments
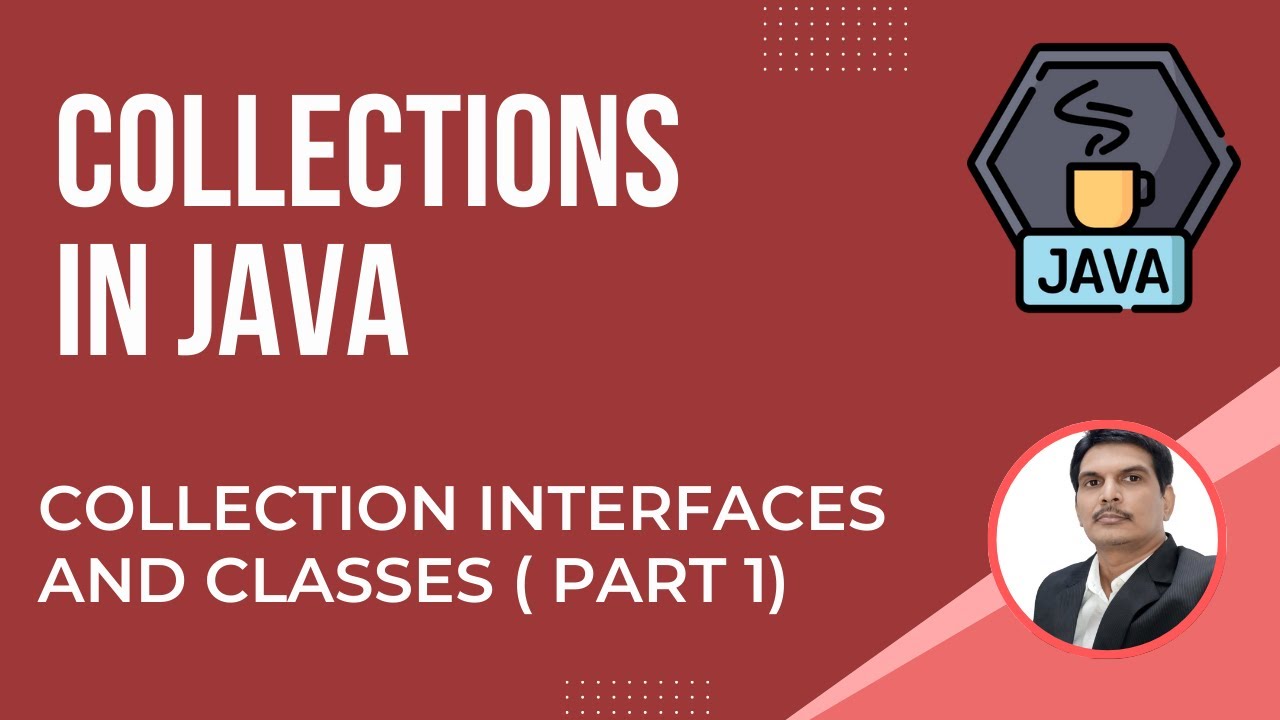
Java Collections Framework-Part2 | Interfaces And Classes
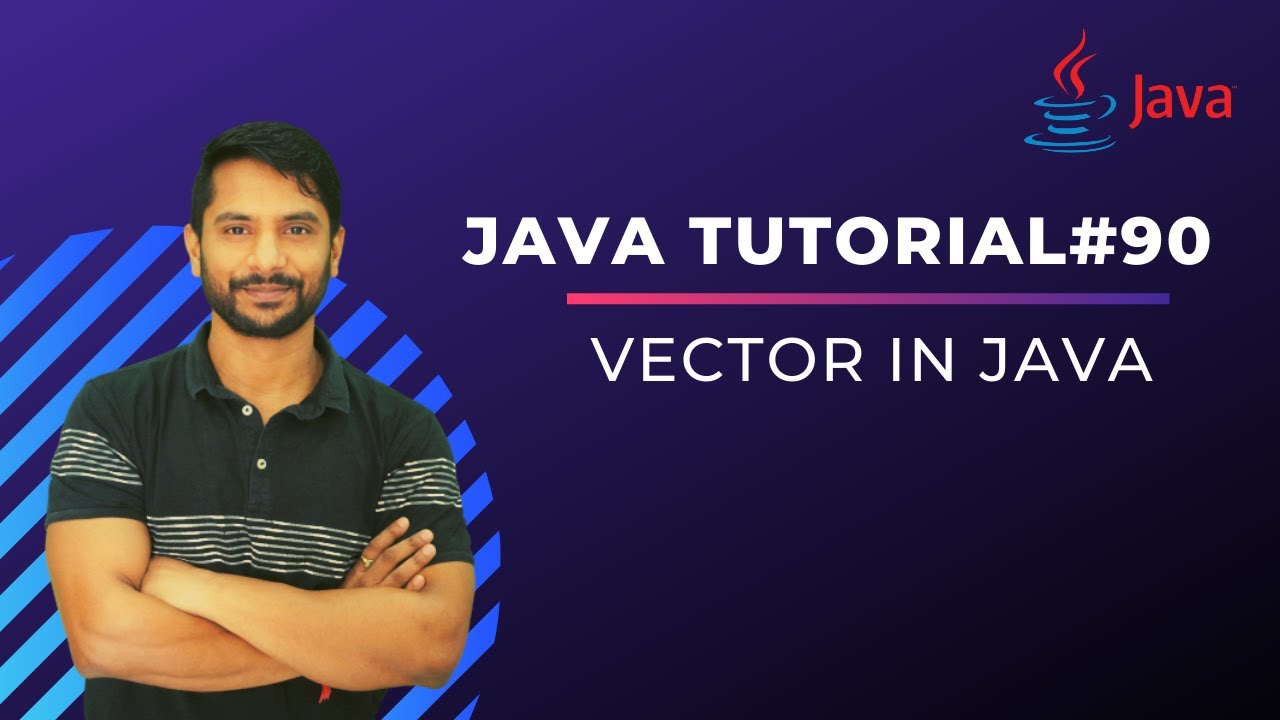
Introduction to Vector | Java Programming | In Hindi
5.0 / 5 (0 votes)