All Python Syntax in 25 Minutes – Tutorial
Summary
TLDRThis video provides a comprehensive introduction to Python programming, covering fundamental concepts such as data types, control structures, functions, and error handling. It explains string manipulation techniques, the use of F-strings for formatting, and the importance of assertions and logging in debugging. Additionally, the video introduces lambda functions for concise coding and demonstrates how to manage variable-length arguments with *args and **kwargs. It concludes with best practices for running Python scripts, emphasizing the __name__ variable for controlling execution context. This engaging overview equips viewers with essential knowledge to enhance their Python coding skills.
Takeaways
- 😀 Use `strip()`, `lstrip()`, and `rstrip()` methods to manipulate whitespace in strings effectively.
- 📝 String formatting can be done using `format()` and F-Strings for improved readability and functionality.
- 🚫 Employ try/except blocks to handle exceptions gracefully and provide custom error messages using `raise`.
- 🔍 Assertions with `assert` statements are crucial for sanity checks to catch errors early in the code.
- 📜 Implement logging in your Python programs to monitor execution flow and aid in debugging.
- ⚡ Lambda functions allow for creating anonymous functions in a compact form, enhancing code conciseness.
- 🔄 Ternary operations provide a one-liner alternative to traditional if-else statements, simplifying conditional logic.
- 🍏 Use `*args` and `**kwargs` in function definitions to accept an arbitrary number of positional and keyword arguments.
- 🛡️ The `if __name__ == '__main__':` guard allows code to run only when the script is executed directly, preventing unwanted execution during imports.
- 📚 For deeper understanding, explore Python classes and objects for advanced programming techniques.
Q & A
What is the purpose of the 'strip()' method in Python?
-'strip()' removes whitespace from both the beginning and the end of a string.
How do f-strings improve string formatting in Python?
-F-strings allow for embedding expressions directly within string literals by prefixing the string with 'f' and using curly braces to include variables.
What is the role of 'raise' in exception handling?
-'raise' is used to trigger an exception manually, which can then be caught and handled using 'try' and 'except' blocks.
What are assertions, and how are they used?
-Assertions are sanity checks in the code that verify certain conditions. If the condition is false, an 'AssertionError' is raised.
How can the logging module enhance a Python program?
-The logging module provides a way to track events and messages during program execution, helping with debugging and monitoring.
What is a lambda function, and when would you use it?
-A lambda function is a small, anonymous function defined with the 'lambda' keyword, often used for short operations or to create functions on the fly.
Can you explain the ternary operation syntax in Python?
-The ternary operation allows for a compact form of an 'if-else' statement in a single line: 'result = value_if_true if condition else value_if_false'.
What do *args and **kwargs represent in function definitions?
-*args allows a function to accept any number of positional arguments as a tuple, while **kwargs allows for any number of keyword arguments as a dictionary.
Why is 'if __name__ == "__main__":' important in Python scripts?
-This condition ensures that certain code blocks are executed only when the script is run directly, preventing them from running when the script is imported as a module.
How do you raise custom exceptions in Python?
-You can raise custom exceptions using 'raise' followed by the exception type and a message, allowing you to define specific error handling in your code.
Outlines
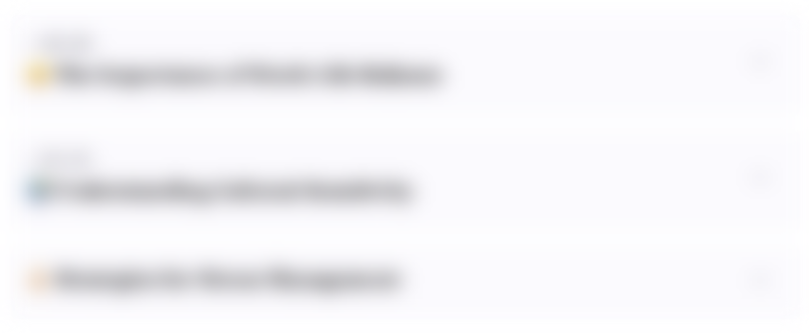
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
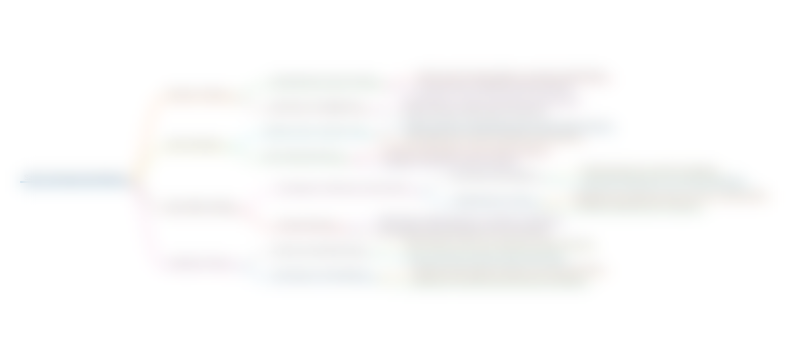
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
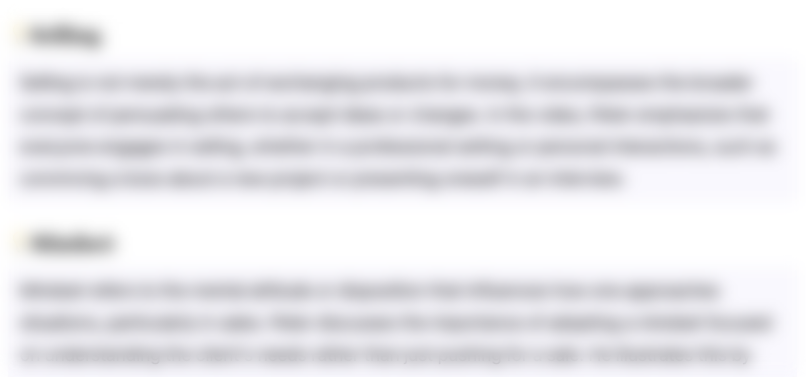
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
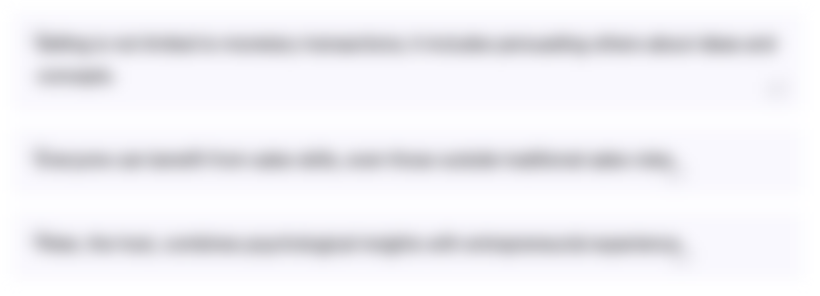
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
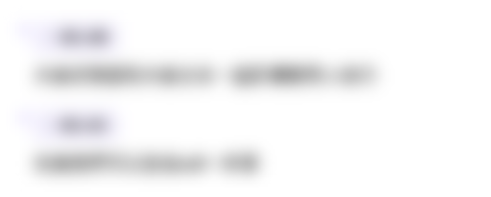
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآن5.0 / 5 (0 votes)