jQuery Crash Course [2] - Events
Summary
TLDRThis tutorial delves into event handling in JavaScript using jQuery, focusing on how to manage user interactions with buttons, paragraphs, and forms. It covers setting up buttons, using the document ready function, and attaching click events. Various event types such as double click, hover, and mouse events are explored, demonstrating how to toggle visibility and retrieve event information. Additionally, the tutorial introduces form events like focus, blur, key events, and form submission, showcasing how to capture and manipulate user input effectively. This foundational knowledge sets the stage for more advanced DOM manipulation techniques.
Takeaways
- 😀 Always ensure your JavaScript runs after the DOM has fully loaded to avoid issues with element selection.
- 🖱️ Use jQuery's `.on()` method for handling events for better performance and flexibility.
- 🔄 The `.toggle()` method allows for easily toggling visibility of elements with a single button.
- 📥 Events like `focus` and `blur` can enhance user interaction with form inputs by changing styles.
- 🔑 Key events such as `keyup` and `keydown` allow tracking user input in real-time.
- 👆 `Mouse up` and `mouse down` events enable more interactive button behaviors beyond simple clicks.
- 🎛️ You can access detailed information about events through event parameters, which provide useful data like target IDs and values.
- 🎉 The `hover` event in jQuery combines `mouseenter` and `mouseleave` for smoother interaction effects.
- 🔄 For form handling, always use `e.preventDefault()` to stop the default submission behavior and manage data manually.
- 👤 By using the event object, you can easily retrieve and manipulate input values and attributes dynamically.
Q & A
What is the primary purpose of the 'document.ready' function in jQuery?
-The 'document.ready' function ensures that the JavaScript code runs only after the entire document is fully loaded, preventing issues with elements not being available for manipulation.
How can you bind a click event to a button using jQuery?
-You can bind a click event to a button by using the syntax: $('#buttonID').on('click', function() { /* your code */ }); where 'buttonID' is the ID of the button.
What does the 'toggle' method do in jQuery?
-The 'toggle' method in jQuery alternates the visibility of an element, showing it if it's hidden and hiding it if it's visible when called.
What are mouse events mentioned in the script, and how do they function?
-Mouse events mentioned include 'mousedown', 'mouseup', 'mouseenter', 'mouseleave', 'mousemove', and 'dblclick'. They trigger specific actions based on mouse interactions with elements.
What is the difference between the 'hover' method and using 'mouseenter' and 'mouseleave' events?
-The 'hover' method is a shorthand for applying both 'mouseenter' and 'mouseleave' events together, allowing for a more concise way to handle mouse entering and leaving an element.
How can you use jQuery to change the background color of an input field when focused?
-You can change the background color of an input field by binding a focus event and setting the CSS property, like so: $(inputSelector).focus(function() { $(this).css('background', 'pink'); });.
What is the purpose of 'preventDefault()' in form submission?
-'preventDefault()' is used to stop the default action of an event, such as form submission, allowing you to perform custom actions like validation or logging without reloading the page.
What is the significance of passing an event object as a parameter in event handlers?
-Passing an event object provides access to properties and methods related to the event, allowing you to get information like the target element, mouse coordinates, and more.
How do you handle input changes in a select dropdown using jQuery?
-You handle input changes in a select dropdown by using the 'change' event, like so: $('#selectID').change(function() { /* your code */ }); where 'selectID' is the ID of the dropdown.
What can you retrieve from the event object during mouse events?
-From the event object during mouse events, you can retrieve properties like 'clientX' and 'clientY' for mouse coordinates, and access the target element's attributes such as 'id' or 'class'.
Outlines
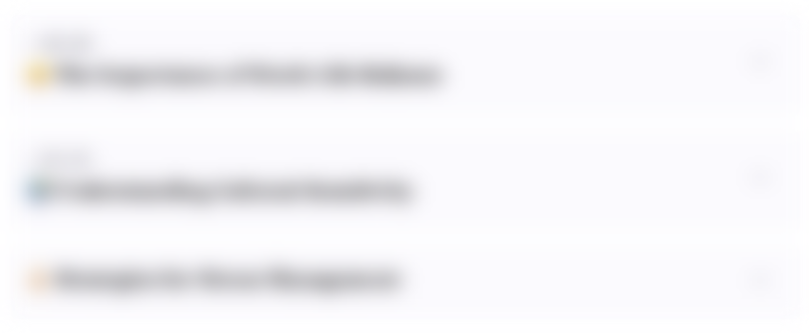
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
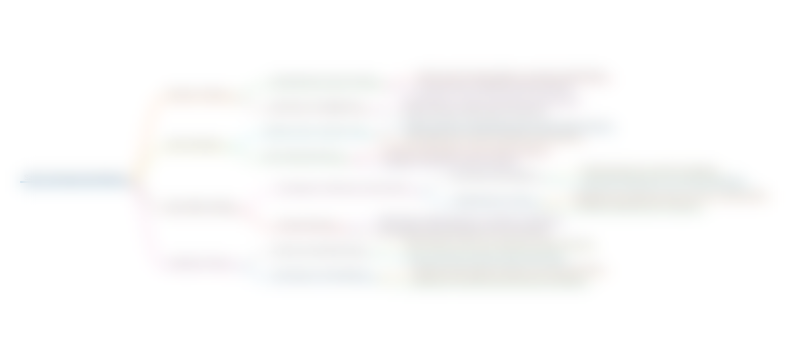
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
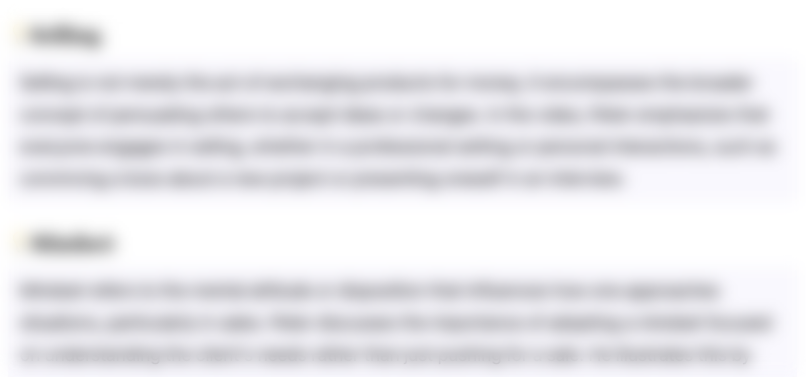
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
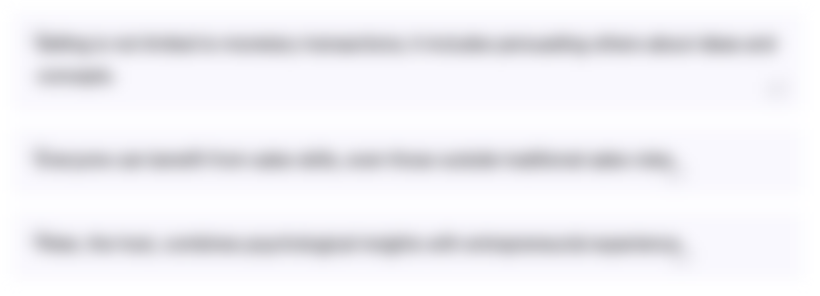
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
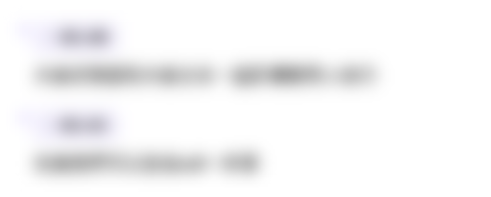
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
5.0 / 5 (0 votes)