C# Classes Tutorial | Mosh
Summary
TLDRIn this lecture, the fundamentals of classes in C# are explored, highlighting their role as building blocks of software applications. Real-world examples, such as a blog engine, illustrate how various classes operate within different layers, including presentation, business logic, and data access. The distinction between classes and objects is clarified, emphasizing that an object is an instance of a class. Key concepts such as instance members and static members are introduced, alongside practical coding demonstrations. By the end, viewers are equipped with a foundational understanding of how to declare classes and utilize their members effectively in C#.
Takeaways
- 😀 A class is a fundamental building block of a software application, serving as a blueprint for creating objects.
- 📚 Real-world applications consist of multiple classes, each responsible for specific behaviors, enhancing maintainability and organization.
- 🧩 The distinction between classes and objects is crucial: a class defines a type, while an object is an instance of that class in memory.
- 🔍 In UML (Unified Modeling Language), a class is represented with its name, attributes (fields), and methods (functions) for clear communication.
- 🖊️ Class names in C# follow Pascal Case conventions, while method parameters use Camel Case for naming consistency.
- 🚀 Instantiating a class in C# requires using the 'new' operator, allowing you to create and interact with objects.
- 🔑 Class members in C# are categorized into instance members (accessible via objects) and static members (accessible via the class itself).
- 🗝️ Static members represent singleton concepts, like current date and time, ensuring only one instance exists in memory.
- 💡 The lecture introduces methods for converting data types, such as a static 'Parse' method for creating a 'Person' object from a string.
- 📈 Understanding classes and object-oriented programming is essential for building robust, extensible applications in C#.
Q & A
What is the purpose of a class in programming?
-A class serves as a building block of a software application, encapsulating data and behavior related to a specific concept or functionality.
How is a class structured in UML?
-In UML, a class consists of three parts: the class name, its fields (or attributes), and its methods (or functions) which define its behavior.
What are the three layers typically found in a layered architecture of a software application?
-The three layers are the presentation layer, business logic layer (or domain layer), and data access layer (or persistence layer).
What distinguishes an object from a class?
-An object is an instance of a class that exists in memory, while a class is a blueprint from which objects are created.
What naming convention is used for classes in C#?
-Classes in C# are named using Pascal case, where the first letter of each word is capitalized.
What is the significance of the 'static' keyword in C#?
-The 'static' keyword indicates that a member belongs to the class itself rather than to any specific instance of the class, allowing access without needing to create an object.
How do you declare a method in a C# class?
-A method is declared by specifying an access modifier, a return type, the method name, and any parameters in parentheses, followed by the method body enclosed in curly braces.
Why might you prefer using the 'var' keyword when declaring variables in C#?
-Using 'var' allows the compiler to infer the type of the variable from the assigned value, reducing redundancy and making the code cleaner.
What is an instance member and how is it accessed?
-An instance member is associated with a specific object of a class and is accessed through that object. For example, you might create a person object and then access its fields or methods.
What is a practical example of a static member?
-A practical example of a static member is the Console.WriteLine method in C#, which can be called directly from the Console class without needing to instantiate an object.
Outlines
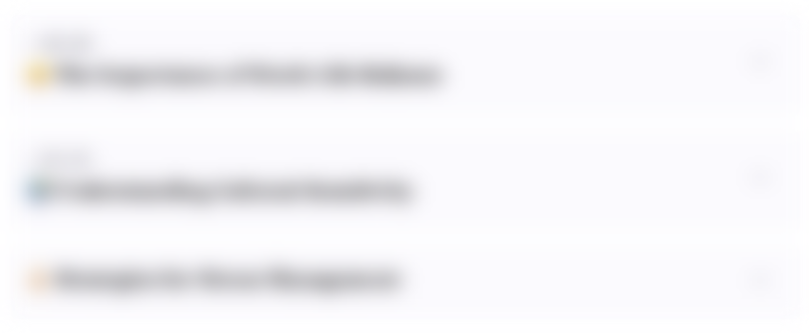
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
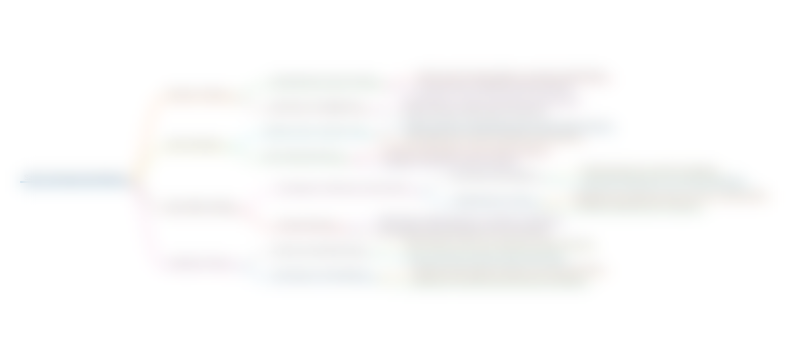
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
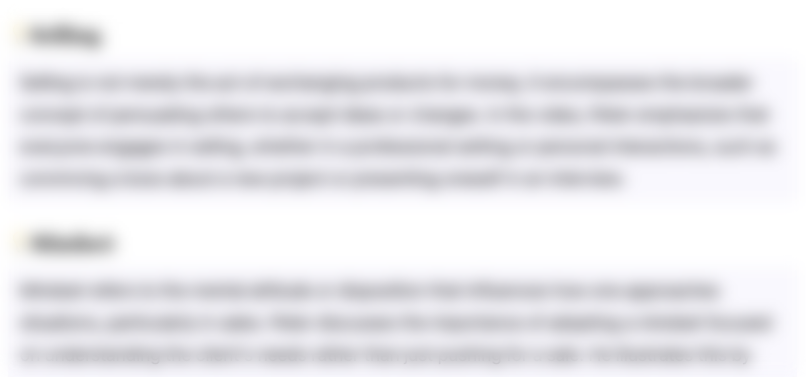
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
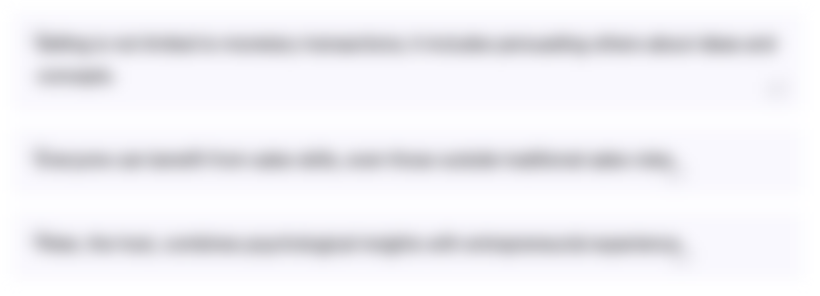
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
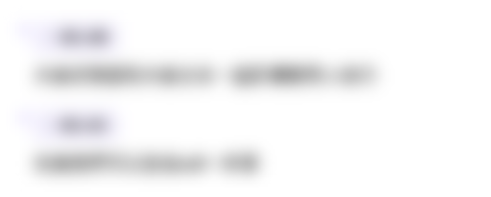
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة

Pengantar Rekayasa Perangkat Lunak (Software Engineering)
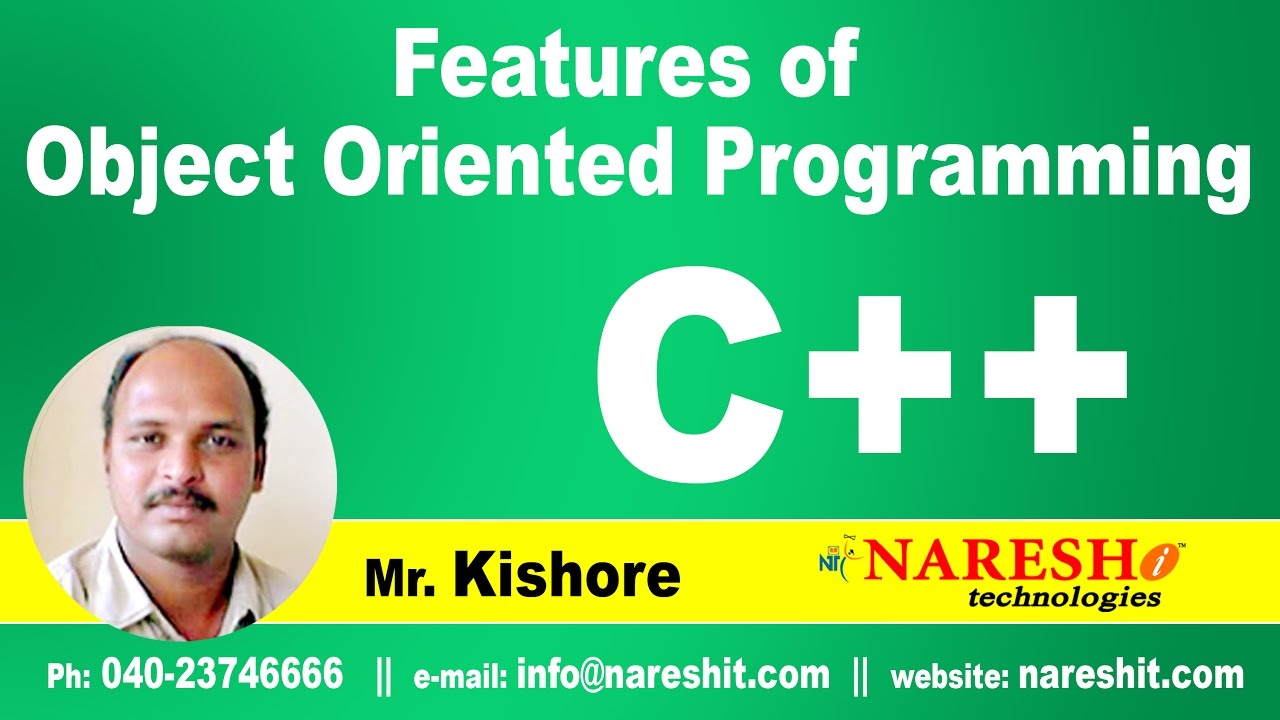
Features of Object Oriented Programming Part 2 | C ++ Tutorial | Mr. Kishore
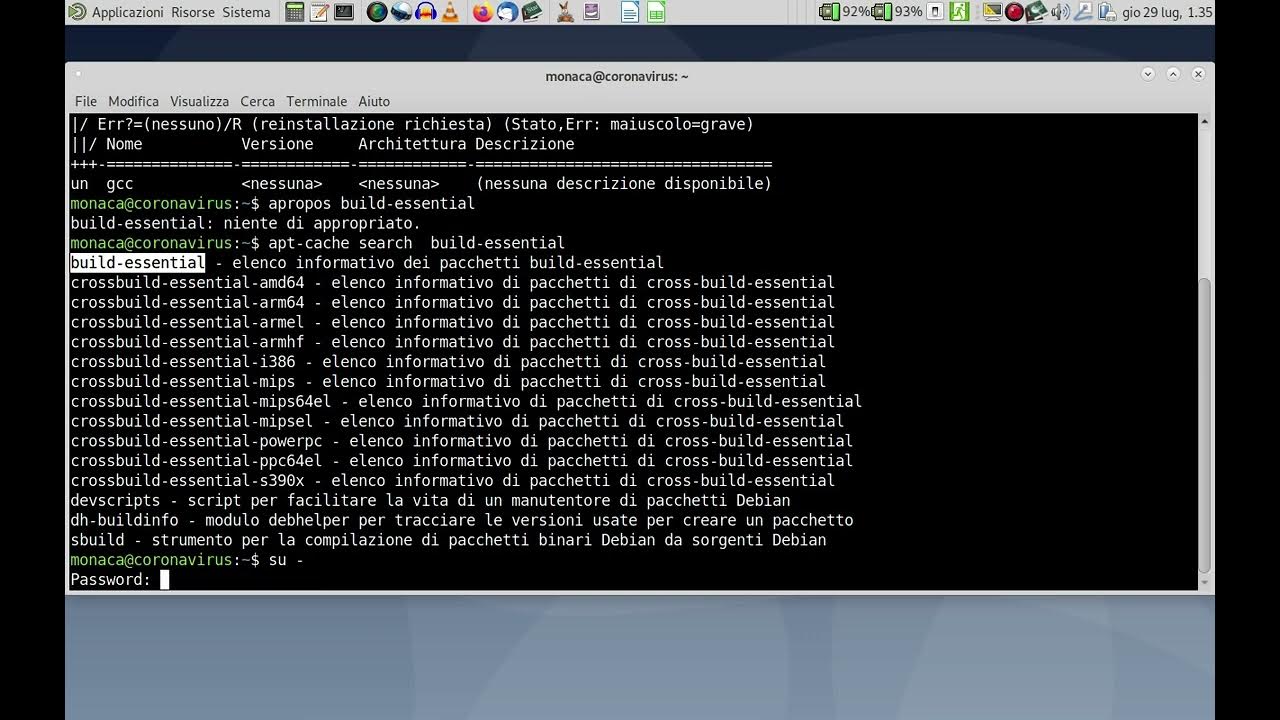
Programmazione di Sistema Gnu Linux 01
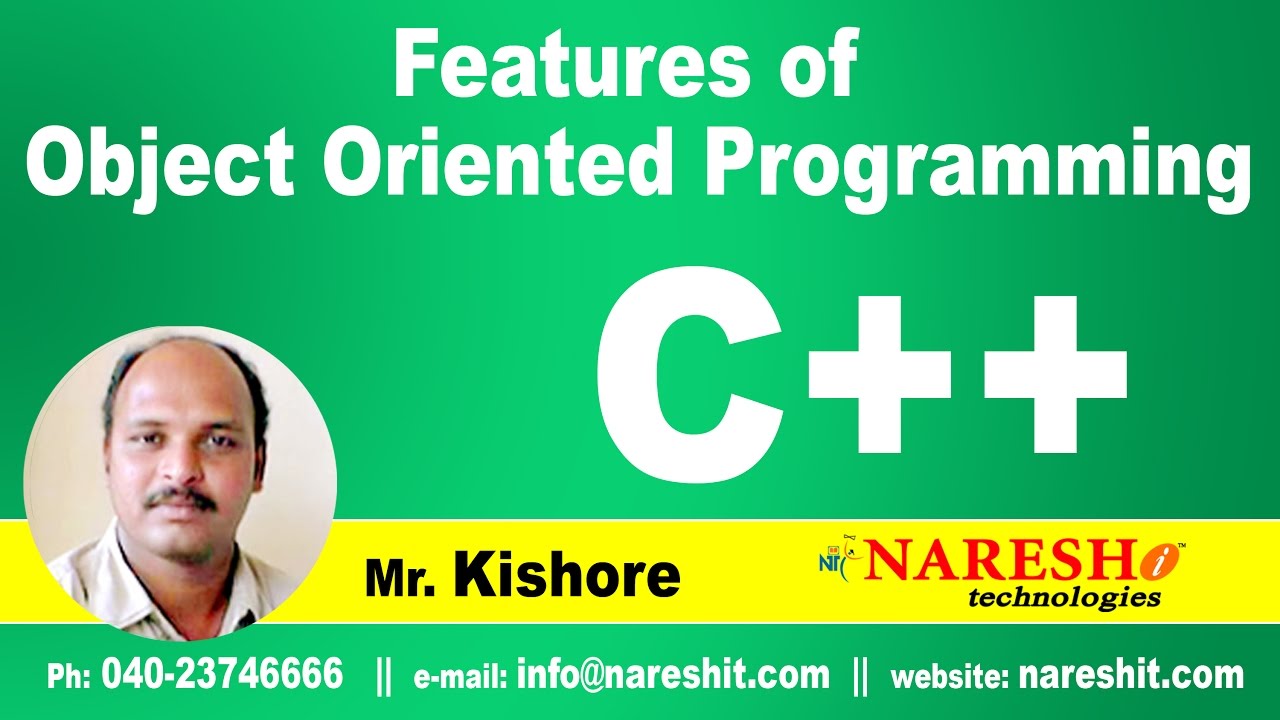
Object Oriented Programming Features Part 3 | C ++ Tutorial | Mr. Kishore

COS 333: Chapter 11, Part 2
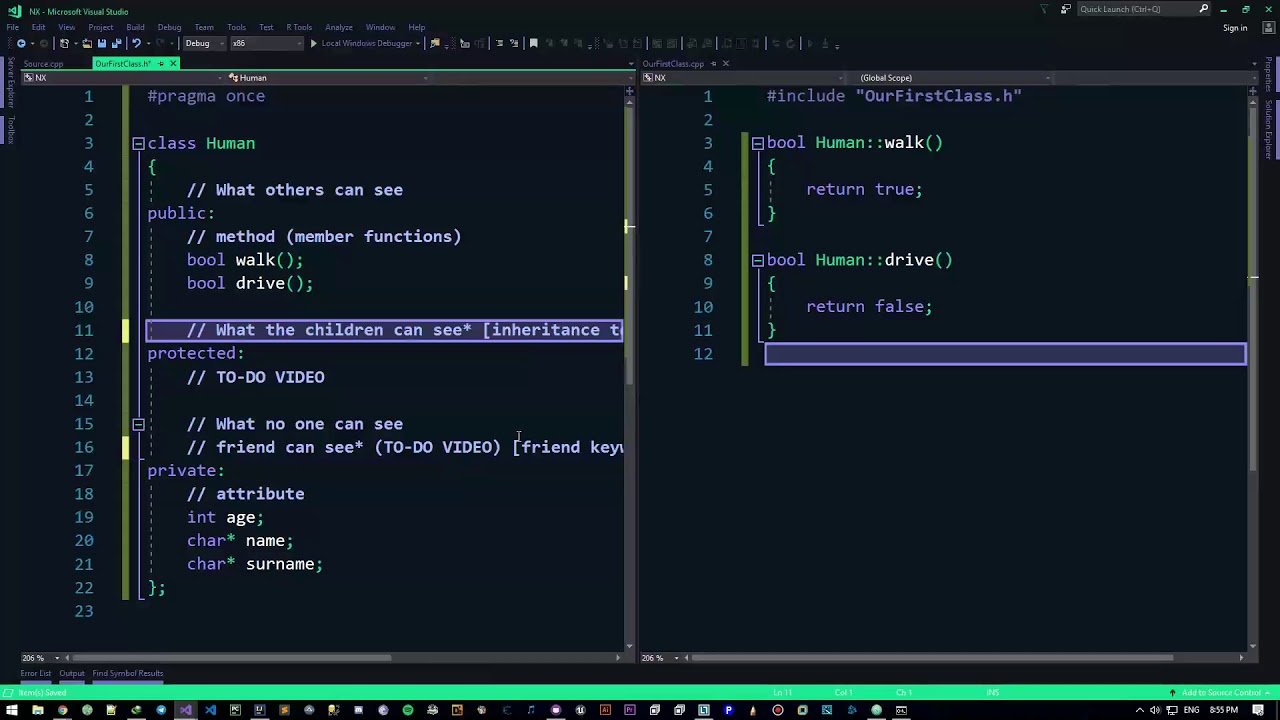
01 - [Classes] C++ Intermediate Programming Tutorial
5.0 / 5 (0 votes)