Numpy - Part 02
Summary
TLDRThis script offers an in-depth tutorial on numpy, covering array creation, indexing, and properties like shape, ndim, size, and d-type. It explores statistical functions such as mean, median, percentile, and correlation coefficient, demonstrating how to compute these along different axes. The script also introduces concatenation, reshaping, and random number generation with numpy, providing practical examples and concluding with a teaser for upcoming pandas lectures on DataFrames.
Takeaways
- 📊 **Shape and Indexing**: Learn how to use the `shape` attribute to understand the dimensions of an array and how to index into arrays to access specific elements.
- 🔢 **Array Properties**: Discover properties like `ndim` for the number of dimensions and `size` for the total number of elements in an array.
- 📏 **Array Methods**: Understand how to use array methods such as `shape`, `ndim`, `size`, and `dtype` to inspect and manipulate array data.
- 📉 **Descriptive Statistics**: Gain insights into calculating statistics like `mean`, `median`, `min`, `max`, and `percentile` for arrays.
- 📈 **Mean Calculation**: Learn how to calculate the mean across different axes of a matrix and what it signifies.
- 📋 **Concatenation**: Explore how to concatenate arrays using functions like `np.concatenate` and `np.stack`, and the importance of matching shapes.
- 🔄 **Random Number Generation**: Understand how to generate random numbers using numpy with functions like `randint`, `random`, and `shuffle`.
- 🔗 **Correlation and Covariance**: Learn to calculate the correlation coefficient and covariance matrix to measure relationships between data sets.
- 📊 **Histograms**: Get introduced to the concept of histograms and how to use `np.histogram` to count the number of elements within certain ranges.
- 🔑 **Data Types**: Recognize the importance of knowing the data type (`dtype`) of an array and how it affects operations performed on it.
Q & A
What is the shape property used for in an array?
-The shape property is used to determine the dimensions of an array. It returns a tuple representing the array's shape, showing the number of rows and columns.
How can you access the number of rows and columns in an array?
-You can access the number of rows using `shape[0]` and the number of columns using `shape[1]` on the array. Alternatively, you can use `ndim` to get the number of dimensions and `size` to get the total number of elements.
What does `ndim` represent in an array?
-`ndim` stands for the number of array dimensions. For example, a one-dimensional array has `ndim` equal to 1, while a two-dimensional array has `ndim` equal to 2.
How can you calculate the mean and median of an array in numpy?
-You can calculate the mean using `np.mean()` and the median using `np.median()`. These functions can also be applied along a specific axis by setting the `axis` parameter.
What is the difference between `np.mean()` and accessing the mean as an attribute of an array?
-`np.mean()` is a function that computes the mean across the array or along a specified axis, while accessing the mean as an attribute would imply a property like `array.mean()` which is not standard in numpy. The correct method is to use the function form.
How do you calculate the percentile of an array's values in numpy?
-You can calculate the percentile of an array's values using `np.percentile()`. You specify the array and the percentile value (between 0 and 100) to get the value below which the given percentage of the data falls.
What is the correlation coefficient and how do you calculate it between two vectors?
-The correlation coefficient is a measure of the linear correlation (dependence) between two variables. In numpy, you can calculate it using `np.corrcoef()` by passing the two vectors as arguments.
What does `dtype` indicate for an array?
-`dtype` indicates the data type of the elements in the array. It tells you whether the array elements are integers, floats, strings, etc.
How can you concatenate two arrays along a specific axis in numpy?
-You can concatenate two arrays along a specific axis using `np.concatenate()` with the `axis` parameter set to the desired axis number. By default, it concatenates along axis 0.
What is the difference between `np.concatenate()` and `np.stack()`?
-`np.concatenate()` joins arrays along an existing axis, while `np.stack()` creates a new axis, stacking arrays on top of each other.
How can you generate random numbers using numpy?
-You can generate random numbers using functions in numpy's random module such as `np.random.rand()` for uniform distribution, `np.random.randn()` for normal distribution, and `np.random.randint()` for random integers within a specified range.
What does `np.random.shuffle()` do?
-`np.random.shuffle()` is used to shuffle the elements of an array in place, rearranging the elements randomly.
Outlines
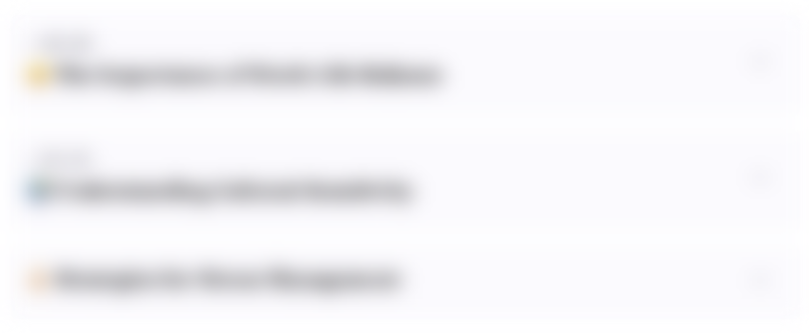
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
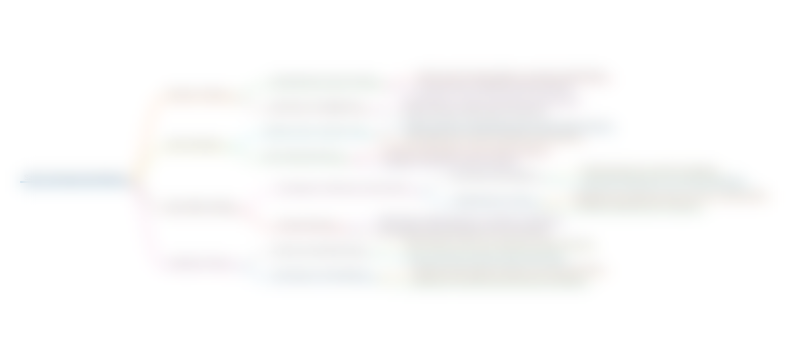
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
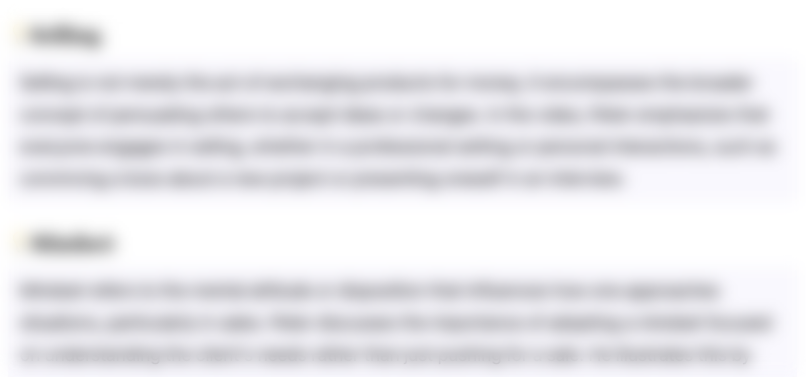
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
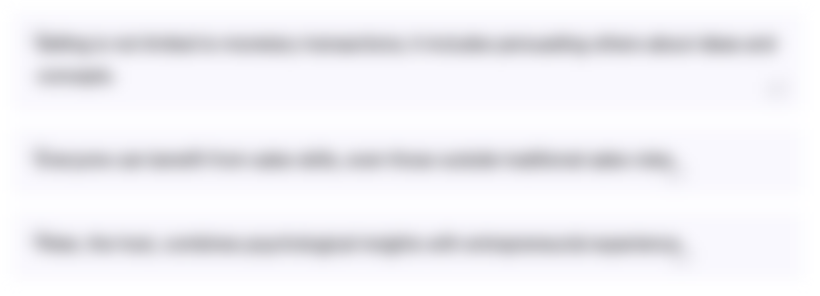
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
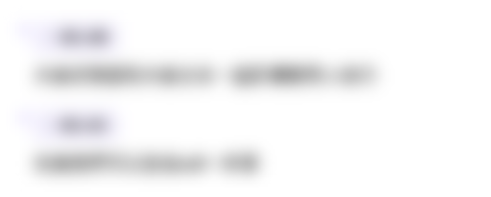
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
5.0 / 5 (0 votes)