Go (Golang) Tutorial #4 - Printing & Formatting Strings
Summary
TLDRThis tutorial introduces key methods from the fmt package in Go for formatting and printing strings. It explains the difference between Print and Println functions, including how to use escape characters like newline. It then covers Printf, demonstrating how to embed variables within formatted strings using format specifiers. Additionally, it explores saving formatted strings with Sprintf and outputs them for later use. The lesson also showcases working with variables, formatting floats, and determining variable types, with examples that are practical for Go programming learners.
Takeaways
- 🖨️ The `fmt` package is used to format strings and print them to the console.
- 🔤 The `fmt.Print` function prints text without adding a new line at the end, unlike `Println`.
- ✍️ To manually add a new line in `Print`, use the escape sequence `\n`.
- 👥 Variables can be printed in strings using multiple arguments separated by commas.
- 💡 `Printf` is used for formatted strings, where variables can be embedded directly into the string using format specifiers.
- 🔢 The format specifier `%v` outputs the default format of variables in `Printf`.
- 🔠 The format specifier `%q` adds quotes around string variables in formatted strings.
- 📏 The format specifier `%T` is used to print the type of a variable in `Printf`.
- 🔢 To format floats in `Printf`, use `%f` with optional precision control (e.g., `%.2f` for two decimal places).
- 💾 `Sprintf` works like `Printf` but returns the formatted string for later use, instead of printing it directly.
Q & A
What is the purpose of the `fmt` package in Go?
-The `fmt` package in Go is used to format and print strings to the console. It provides various methods like `Print`, `Println`, `Printf`, and `Sprintf` for string formatting and output.
What is the key difference between the `Print` and `Println` functions?
-The key difference is that `Print` does not add a newline at the end of the output, while `Println` automatically adds a newline after printing the content.
How do you manually add a newline in the `Print` function?
-You can manually add a newline in the `Print` function by using the escape sequence `\n` at the desired location in the string.
How can you print multiple values using the `Println` function?
-You can print multiple values using `Println` by separating them with commas inside the parentheses. Each value will be printed with a space separating them.
What is a format specifier in Go, and how is it used in `Printf`?
-A format specifier is a placeholder in a string that is replaced by a value when the string is printed. In `Printf`, it is represented by `%` followed by a letter, like `%v` for the default format of variables.
What does the `%v` format specifier do in `Printf`?
-The `%v` format specifier in `Printf` is the default format for variables and outputs them in their default string representation.
What happens if you use the `%q` format specifier for an integer?
-The `%q` format specifier is intended for strings and will enclose the string in quotes. If used for an integer, it may not work correctly and can produce unexpected output like a hash symbol.
How can you use `Printf` to output the type of a variable in Go?
-You can use the `%T` format specifier in `Printf` to output the type of a variable. For example, `fmt.Printf("%T", age)` would output the type of the `age` variable.
How can you control the number of decimal places when printing a float in Go?
-To control the number of decimal places when printing a float in Go, you can use a format like `%.2f` where the number between `.` and `f` specifies the number of decimal places (e.g., `%.2f` for two decimal places).
What is the difference between `Printf` and `Sprintf` in Go?
-The difference is that `Printf` prints the formatted string directly to the console, while `Sprintf` formats the string and returns it, allowing you to store it in a variable for later use.
Outlines
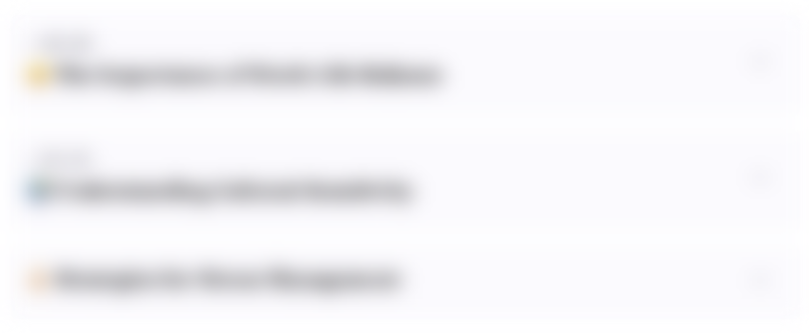
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
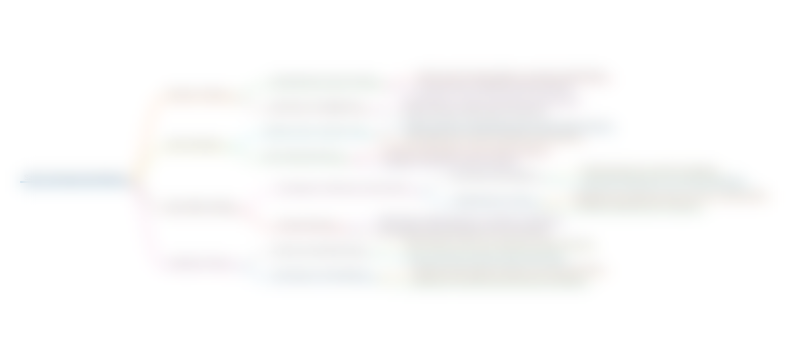
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
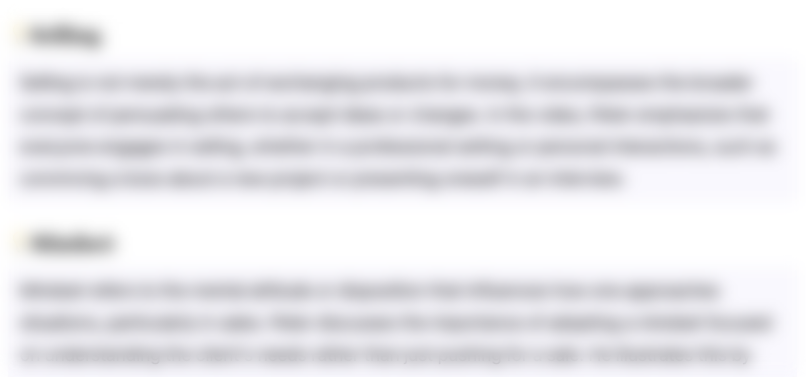
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
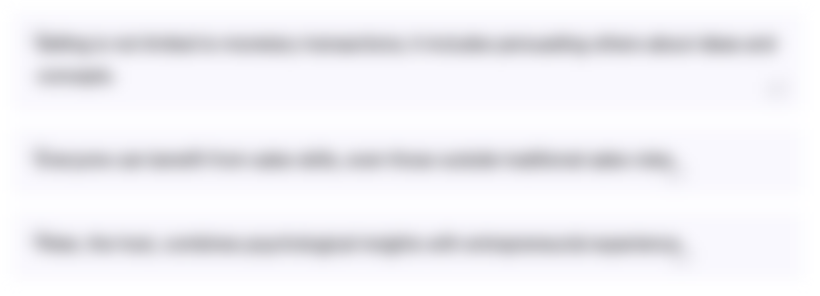
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
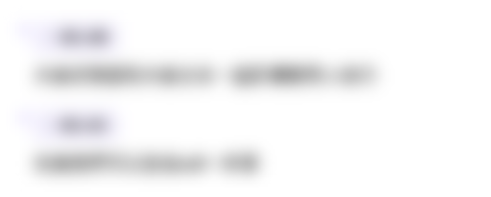
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
5.0 / 5 (0 votes)