58. OCR GCSE (J277) 2.1 Linear search
Summary
TLDRThis video explores the linear search algorithm, a fundamental concept in computer science. It's a straightforward method for locating an item in a list or array without requiring any order. Despite its simplicity and efficiency for small datasets, it can be inefficient for larger ones. The video provides a practical example with a word search and a supermarket scenario, illustrating how a linear search can be applied in various contexts. It also discusses the algorithm's implementation in Python, emphasizing understanding over memorization. The video concludes with a reference to a book, 'Essential Algorithms for A Level Computer Science,' which covers algorithms required for GCSE and A Level studies, offering a structured approach to learning through high-level introductions, structured English, diagrams, and code examples in Python and Visual Basic.
Takeaways
- 🔍 **Linear Search Defined**: The script introduces the linear search algorithm, which involves checking each item in a dataset sequentially until the target is found.
- 📚 **Applicability**: Linear search can be applied to data stored in memory like arrays or lists, and also in files, making it versatile for different storage types.
- 🚫 **No Order Required**: Unlike binary search, linear search does not require the data to be sorted, which simplifies its application.
- 📉 **Efficiency Consideration**: While efficient for small datasets, linear search becomes inefficient for larger ones due to its sequential nature.
- 📚 **Practical Example**: The script uses a word search puzzle as an example to illustrate how linear search works in a real-world context.
- 🛒 **Everyday Analogy**: It compares linear search to finding a specific box of cereal on a supermarket shelf, emphasizing its practicality.
- 💡 **Algorithmic Thinking**: The video encourages viewers to think algorithmically, showing how to apply linear search to a product database lookup scenario.
- 📝 **Pseudocode Explanation**: It outlines a simple pseudocode for linear search, explaining the use of a Boolean variable and an index to track the search process.
- 💻 **Python Code Example**: The script provides a Python code example that demonstrates how to implement linear search in a two-dimensional list for product data.
- 📖 **Educational Resources**: The video mentions a book, 'Essential Algorithms for A Level Computer Science', which covers algorithms including linear search, suitable for GCSE and A Level students.
- 🎓 **GCSE Specification**: It highlights the GCSE requirements for understanding algorithms, emphasizing the need to understand the steps and prerequisites without memorizing the code.
Q & A
What is the linear search algorithm?
-The linear search algorithm is a method for finding a particular item in a list or array by checking each element in sequence until the desired item is found or the list ends.
What are the key characteristics of the linear search algorithm?
-The linear search algorithm is straightforward, works on unsorted data, and does not require the data to be in any order. It is efficient for small data sets but inefficient for large ones.
How is linear search different from binary search?
-Linear search differs from binary search in that it does not require the data to be sorted and checks each item in sequence, whereas binary search operates on sorted data and eliminates half of the remaining elements with each comparison.
In what type of storage devices can linear search be performed?
-Linear search can be performed on data stored in memory, such as arrays or lists, as well as data stored in files.
Can you provide an example of a linear search from the script?
-An example from the script is searching for a word in a word search puzzle, where each letter is stored in a grid, and each letter is checked in turn to match the first letter of the word being searched for.
How is a linear search applied in a supermarket scenario as described in the script?
-In a supermarket scenario, a linear search can be applied by looking for a particular box of cereal on a shelf by going through each box one at a time until the desired one is found.
What is the role of the 'found' variable in the linear search algorithm?
-The 'found' variable in the linear search algorithm is a Boolean variable that is initially set to false. It is used to keep track of whether the item being searched for has been found within the data set.
What is the significance of setting the 'found' variable to true when the item is found?
-Setting the 'found' variable to true signifies that the search is complete, and there is no need to continue checking the remaining items, thus making the algorithm more efficient.
What does the GCSE specification require students to understand about algorithms?
-The GCSE specification requires students to understand the main steps of each algorithm, any prerequisites, how to apply the algorithm to a data set, and how to identify an algorithm if given its code.
What is the purpose of the book 'Essential Algorithms for A Level Computer Science' mentioned in the script?
-The book 'Essential Algorithms for A Level Computer Science' is designed to help students understand algorithms required for the GCSE and A Level computer science exams. It provides a structured approach to learning algorithms, including high-level introductions, structured English, diagrams, pseudocode, and actual code examples in Python and Visual Basic.
Outlines
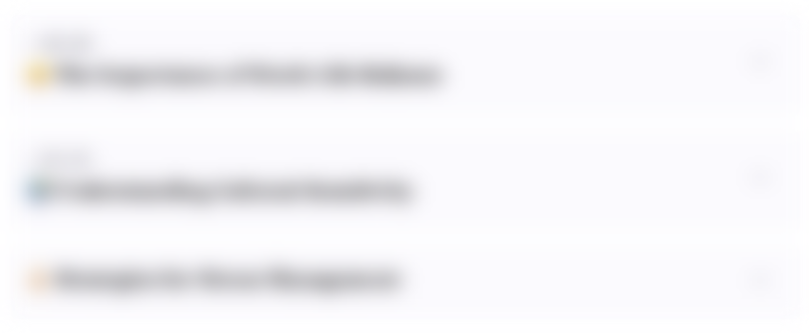
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
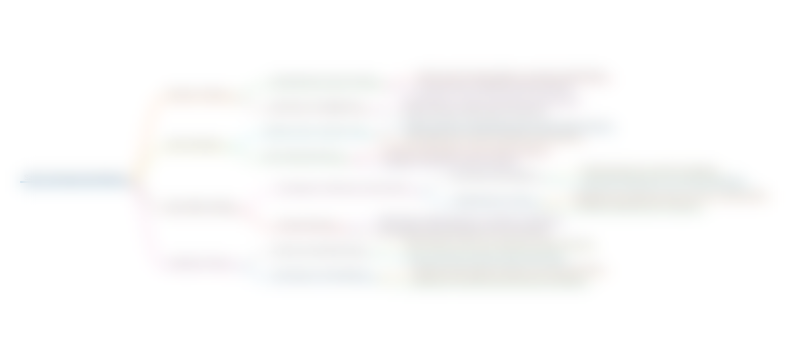
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
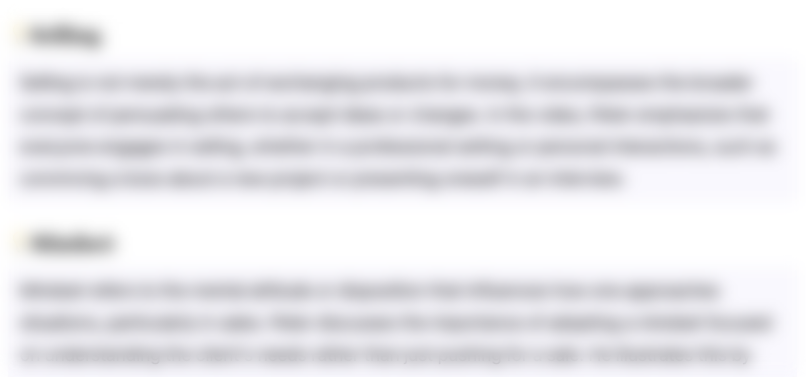
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
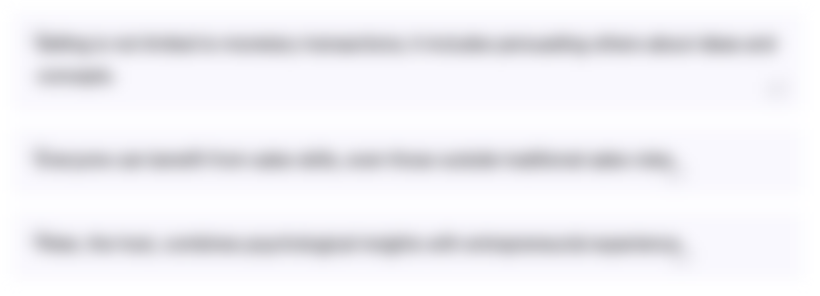
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
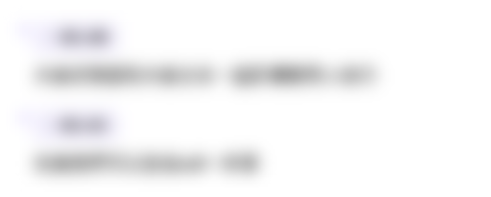
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
5.0 / 5 (0 votes)