How To Create Variables That Don't Suck - Writing Clean Java Code
Summary
TLDRThis video script emphasizes the importance of using meaningful and descriptive names in programming to enhance code readability and maintainability. It discusses best practices for naming variables, classes, methods, and packages, drawing inspiration from 'Clean Code' by Robert C. Martin. Key takeaways include using context to inform variable names, keeping names concise yet meaningful, and adhering to naming conventions like camelCase for variables and classes. The script also stresses the value of pronounceable names for team communication and the significance of method names that clearly convey their purpose.
Takeaways
- 🔠 **Meaningful Names**: Use variable names that clearly indicate what is stored and the purpose behind it.
- 📏 **Context Matters**: The context in which a variable is used affects its naming; for instance, 'catWeightInKilograms' makes sense within a Cat class but not in isolation.
- 🔑 **Keep It Short**: Variable names should be as short as possible while remaining meaningful and clear.
- 🗣️ **Pronounceability**: Names should be easy to pronounce to facilitate communication among team members.
- 🚫 **Avoid Type Information**: Generally, don't include type information in variable names unless necessary for clarity.
- 🔤 **Naming Conventions**: Follow Java naming conventions such as camelCase for variables and classes, and UPPERCASE with underscores for constants.
- 📚 **Constants**: Use all caps with underscores for constants, following the convention for enums as well.
- 📖 **Readability**: Good method names should be verbs or verb phrases that clearly describe what the method does without needing to look into the code.
- 🛠️ **Single Responsibility**: Methods should do one thing, making naming straightforward and reducing the chance of miscommunication.
- ⏱️ **Time Investment**: Investing time in choosing good names pays off in the long run by making code more maintainable and understandable.
Q & A
Why are good names important in programming?
-Good names are crucial in programming because they make the code more readable and maintainable, reducing the time and effort required for other programmers or future self to understand the code.
What does a meaningful variable name convey?
-A meaningful variable name conveys both what is being stored in the variable and the purpose behind that variable, making it clear and understandable without needing to look at the code.
Why is it important to include context in variable names?
-Including context in variable names is important because it clarifies what the variable represents, especially when it's not immediately clear from the surrounding code, such as when the variable is not part of a class or a well-defined scope.
What is the recommended length for variable names?
-Variable names should be kept as short as possible while still being meaningful, avoiding overly long or verbose names that do not add clarity.
Why should variable names be pronounceable?
-Variable names should be pronounceable to facilitate communication among team members during discussions, code reviews, or when explaining the code to others.
Why should type information generally be avoided in variable names?
-Type information should generally be avoided in variable names because it can be redundant, as the programming language itself already defines the type, and it can clutter the name, making it less readable.
What is the naming convention for variables in Java?
-In Java, variables should use camelCase, with the first letter of each word capitalized except for the first letter of the variable name, which should be lowercase.
How should constants be named in Java?
-Constants in Java should be named using all uppercase letters with words separated by underscores, following the convention for enums and other constants.
What is the difference between class names and variable names in terms of naming conventions?
-Class names should be nouns and use camelCase with the first letter capitalized, while variable names should also use camelCase but start with a lowercase letter.
Why should method names be verbs or verb phrases?
-Method names should be verbs or verb phrases because they describe actions, which is what methods typically perform, making it clear what the method does when called.
How can you tell if a method name is good?
-A good method name is one that clearly describes what the method does without needing to look inside the code, and it matches the functionality when you do look at the code.
Outlines
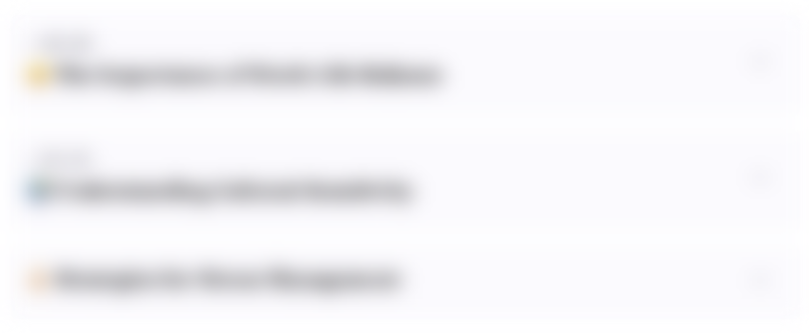
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
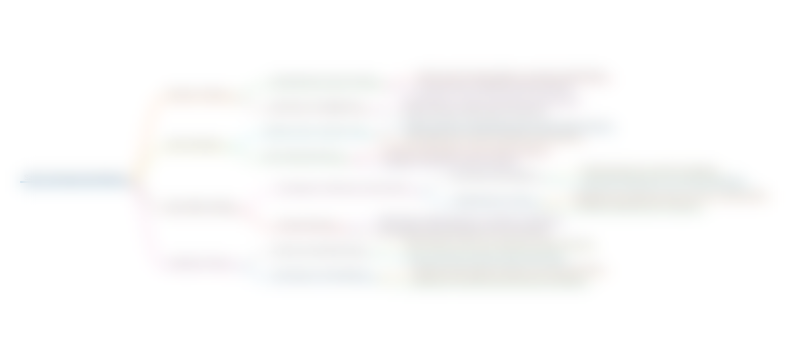
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
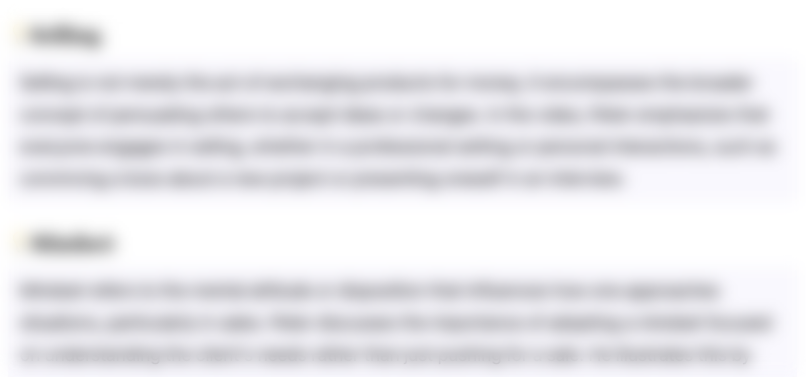
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
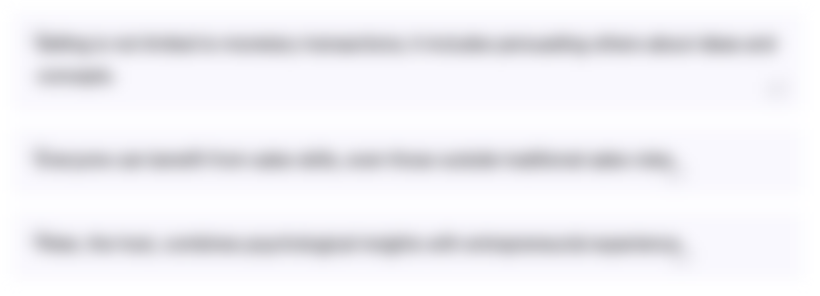
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
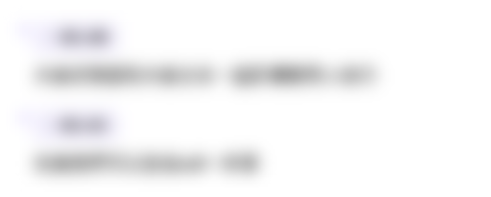
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
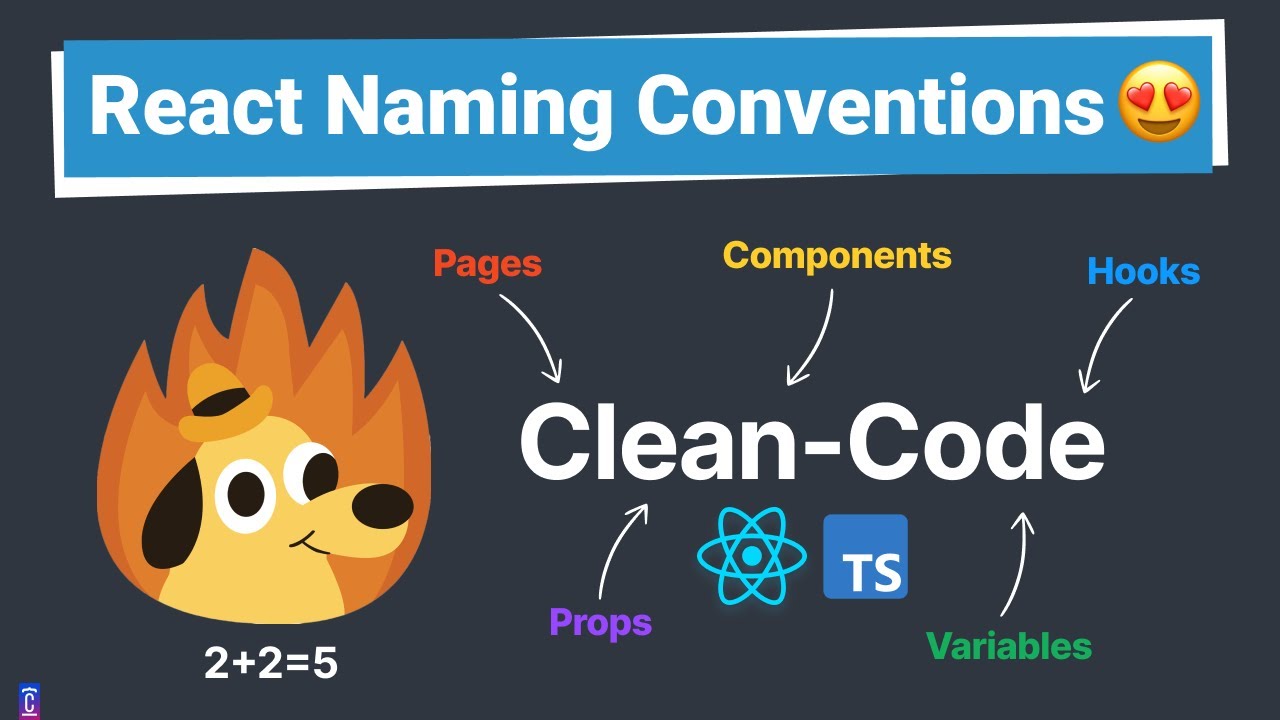
React Naming Conventions You should follow as a Junior Developer - clean-code
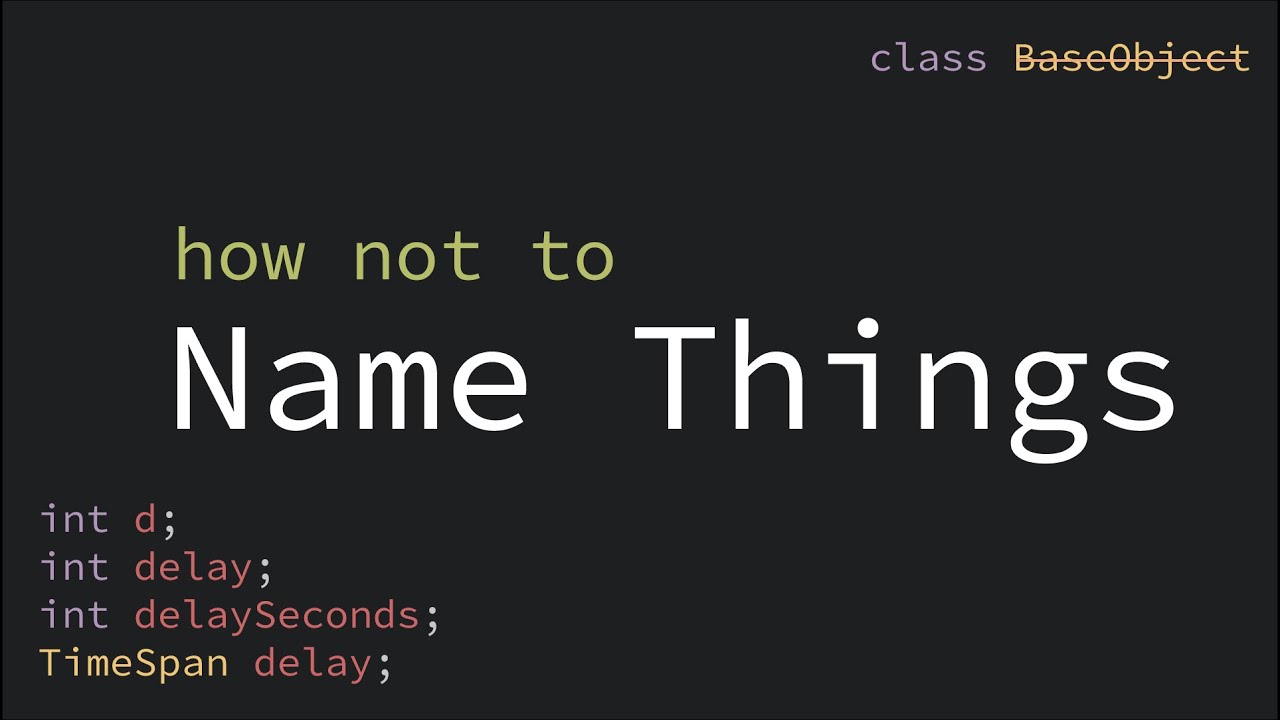
Naming Things in Code
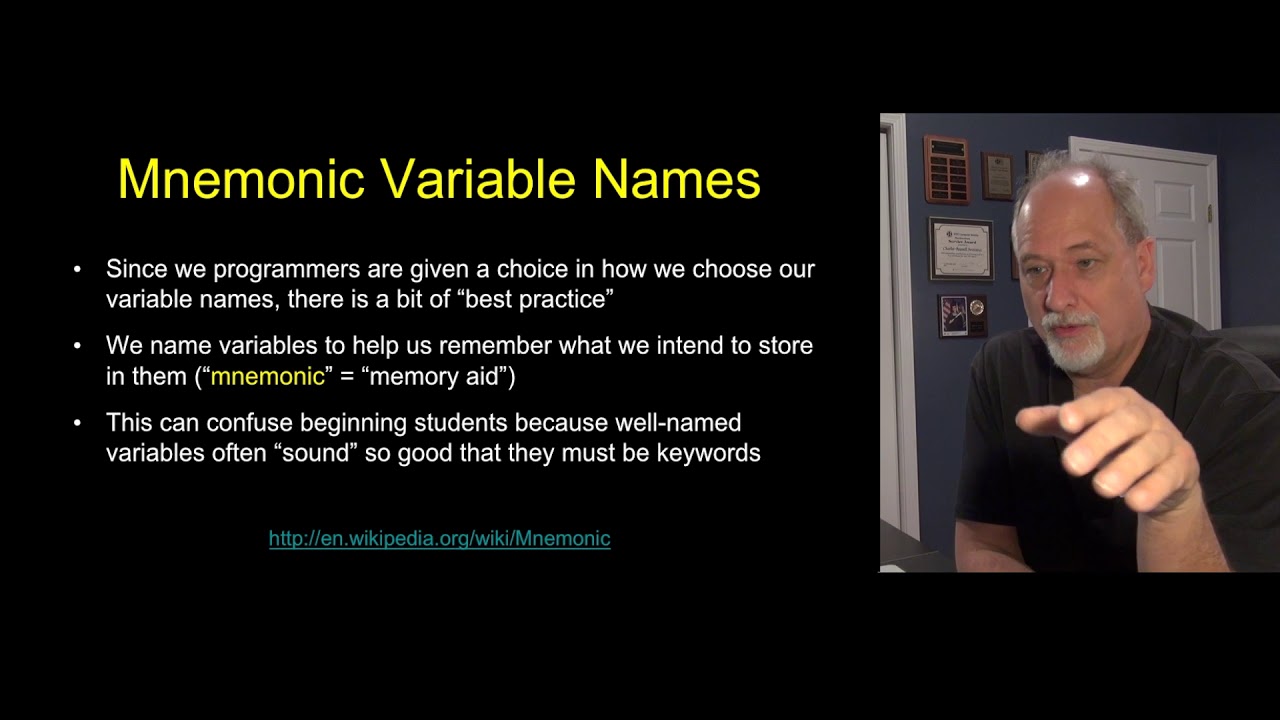
02 - Expressions A - Python for Everybody Course
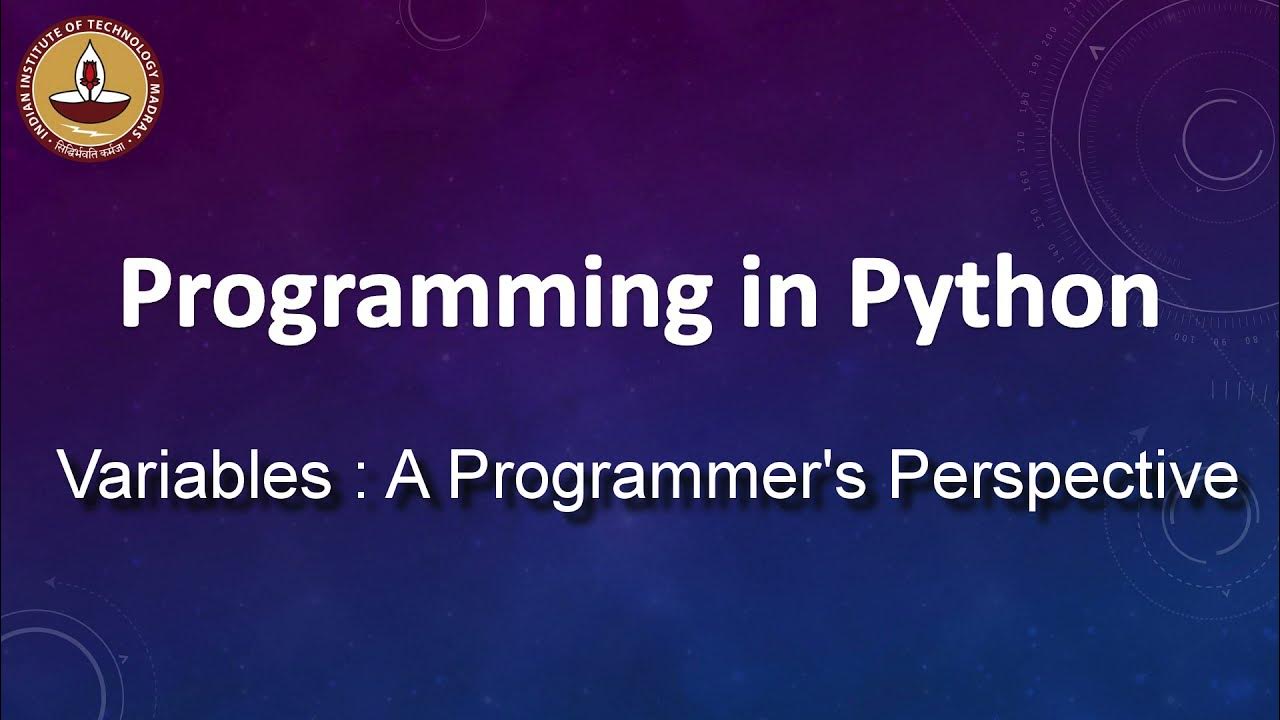
Variables : A Programmer's Perspective

10 Tips Menulis Code JavaScript yang Clean
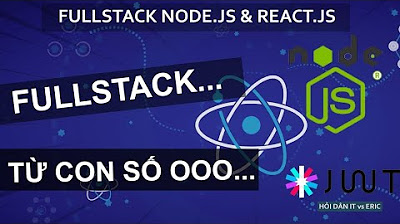
#6.2 Đặt Tên Số Ít/Số Nhiều Cho Table Database | SERIES FULLSTACK - JWT, Node.JS & React
5.0 / 5 (0 votes)