C++ Validating Input with a while Loop
Summary
TLDRThis tutorial demonstrates how to implement input validation using a while loop structure in a program. The program prompts users for their age, validates the input to ensure it's an integer, and provides a custom message based on the age. If the input is invalid, the program enters a loop, prompting the user to enter a valid number. The tutorial also addresses handling type mismatches and clearing the input stream to prevent endless loops, ensuring the program only continues with valid data.
Takeaways
- 🔁 The tutorial demonstrates using a while loop to validate user input in a program that asks for the user's age.
- 📢 The program provides a custom message based on the age input and asks if the user wants to run the program again.
- ❌ If non-integer data is entered, the program enters an endless loop due to a type mismatch.
- 🔍 To handle this, an inner while loop is introduced to ensure the input is an integer before proceeding.
- 💬 The program displays a 'must be a number' message if the input is not valid, prompting the user to try again.
- 🔄 The input stream is cleared and restored to a working state when an input failure occurs.
- 🗑️ The program uses 'cin.ignore' to discard the invalid input, either up to 100 characters or until a newline character is encountered.
- 🔒 The tutorial emphasizes the importance of input validation to prevent the program from entering an endless loop with incorrect data types.
- 📝 The script illustrates how to manage user input and error handling in programming, ensuring the program only processes valid data.
- 🔁 The tutorial concludes with the program successfully validating the user's input and continuing with its logic once valid data is entered.
Q & A
What is the main focus of the tutorial?
-The tutorial focuses on input validation using a while loop structure in a program that asks for the user's age.
What happens when the user enters a valid age?
-When the user enters a valid age, the program provides a custom message based on the age and asks if the user wants to run the program again.
What is the purpose of the inner while loop?
-The inner while loop is used to ensure that the input is an integer. It continues to prompt the user until a valid integer is entered.
Why does the program go into an endless loop when non-integer data is entered?
-The program goes into an endless loop because the input is not of the expected integer type, causing a type mismatch and failing to exit the loop.
How does the program handle input failure?
-Upon input failure, the program uses a while loop to keep prompting the user until a valid number is entered. It also clears the input stream and discards the invalid input.
What is the significance of the 'C in clear' statement?
-The 'C in clear' statement is used to clear the input stream and restore it to a working state after an input failure.
Why is it necessary to discard the invalid input?
-Discarding the invalid input is necessary to prevent the program from continuously processing the wrong data type and to allow for new, valid input.
What is the role of the 'C and ignore' statement in the program?
-The 'C and ignore' statement is used to ignore up to 100 characters or until a newline character is encountered, effectively discarding the invalid input.
How does the program ensure that the user is prompted again after an invalid input?
-The program ensures that the user is prompted again by displaying a 'must be a number' message and then clearing the input stream before resuming the input prompt.
What is the final check the program performs after the user enters a number?
-After the user enters a number, the program checks if it is a valid integer. If valid, the loop exits, and the program continues with the rest of the logic using the correct data type.
Outlines
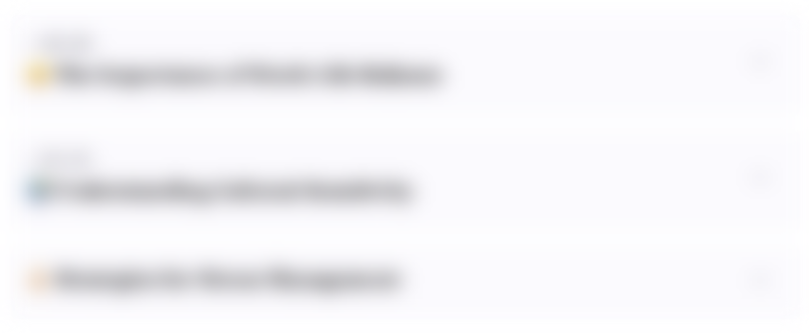
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
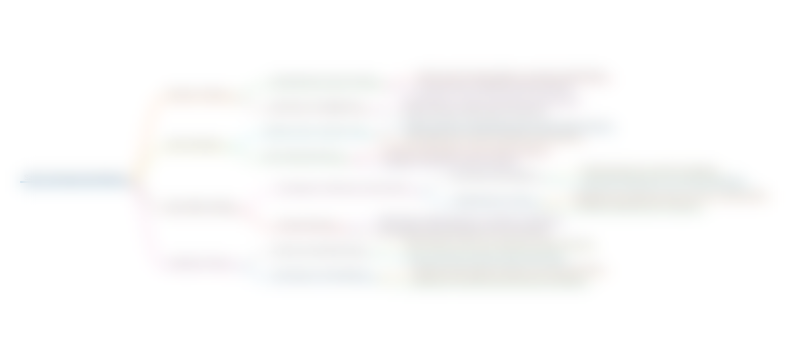
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
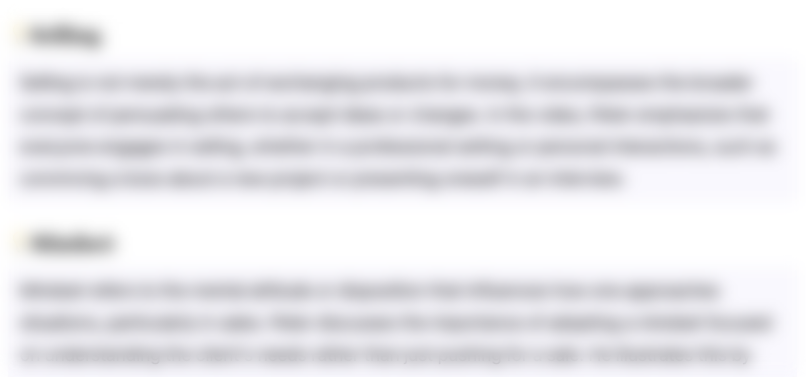
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
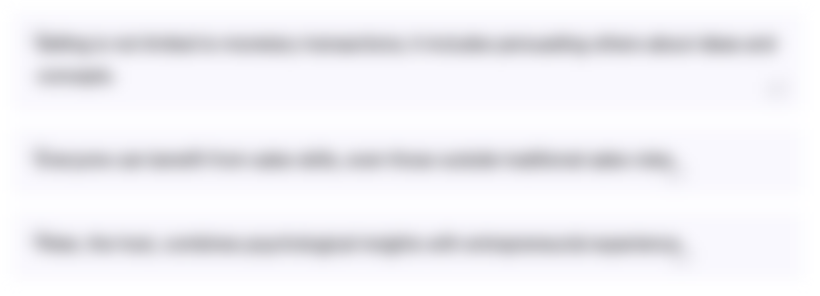
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
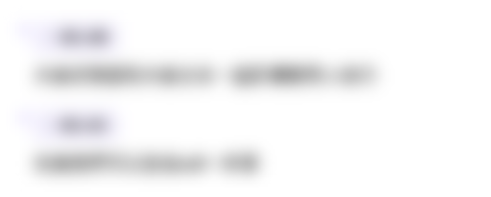
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة

Python Programming Tutorial: Python while loop input validation
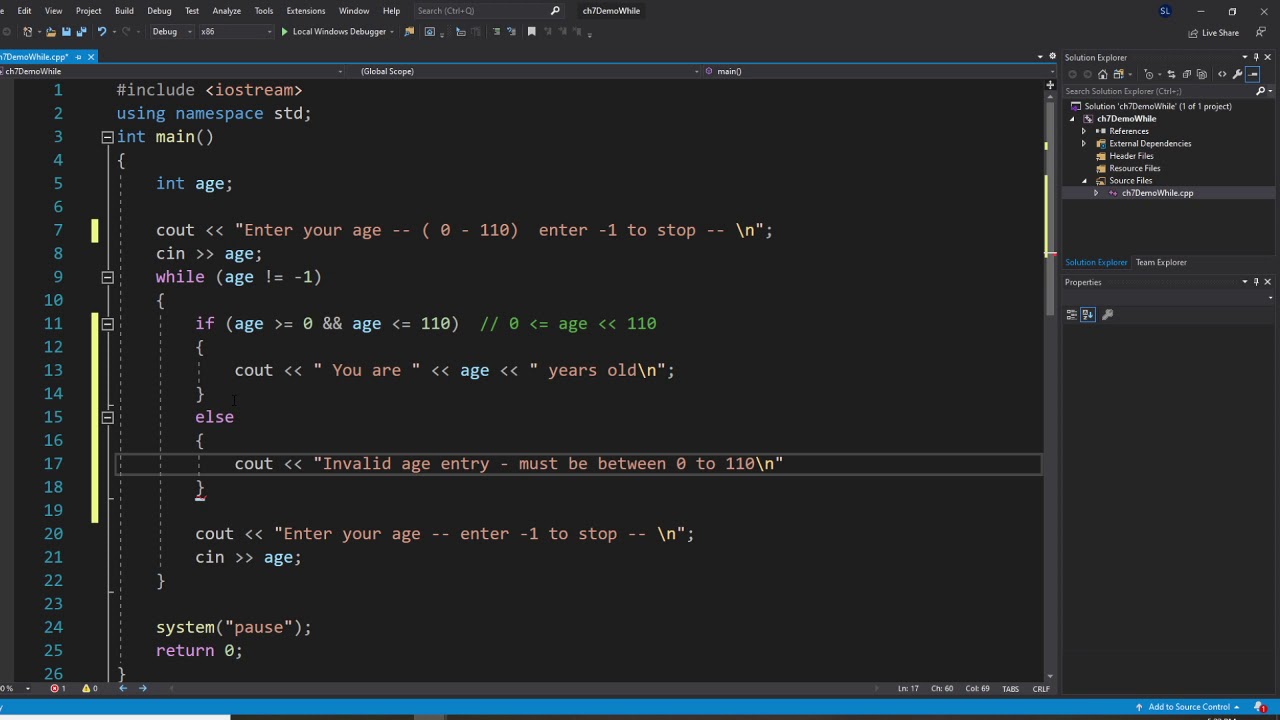
C++ read and display the age, use if else to prevent user enter invalid age. error handling
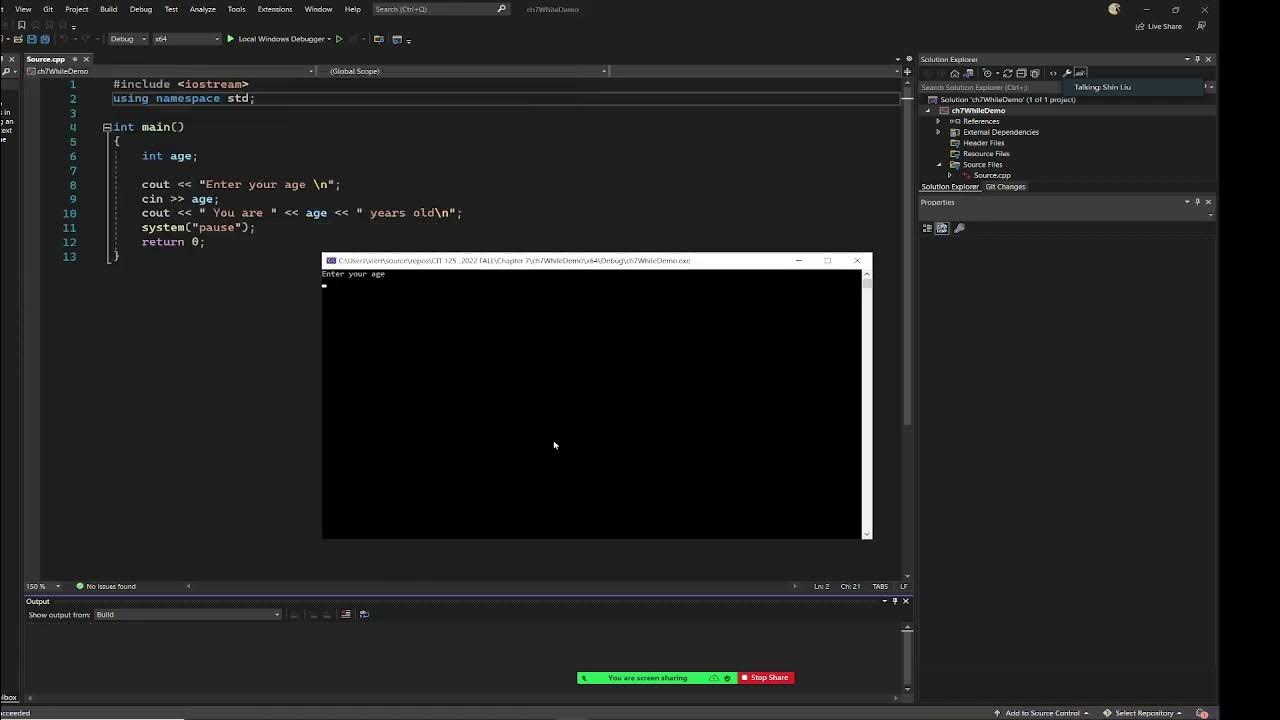
C++ programming , while loop introduction
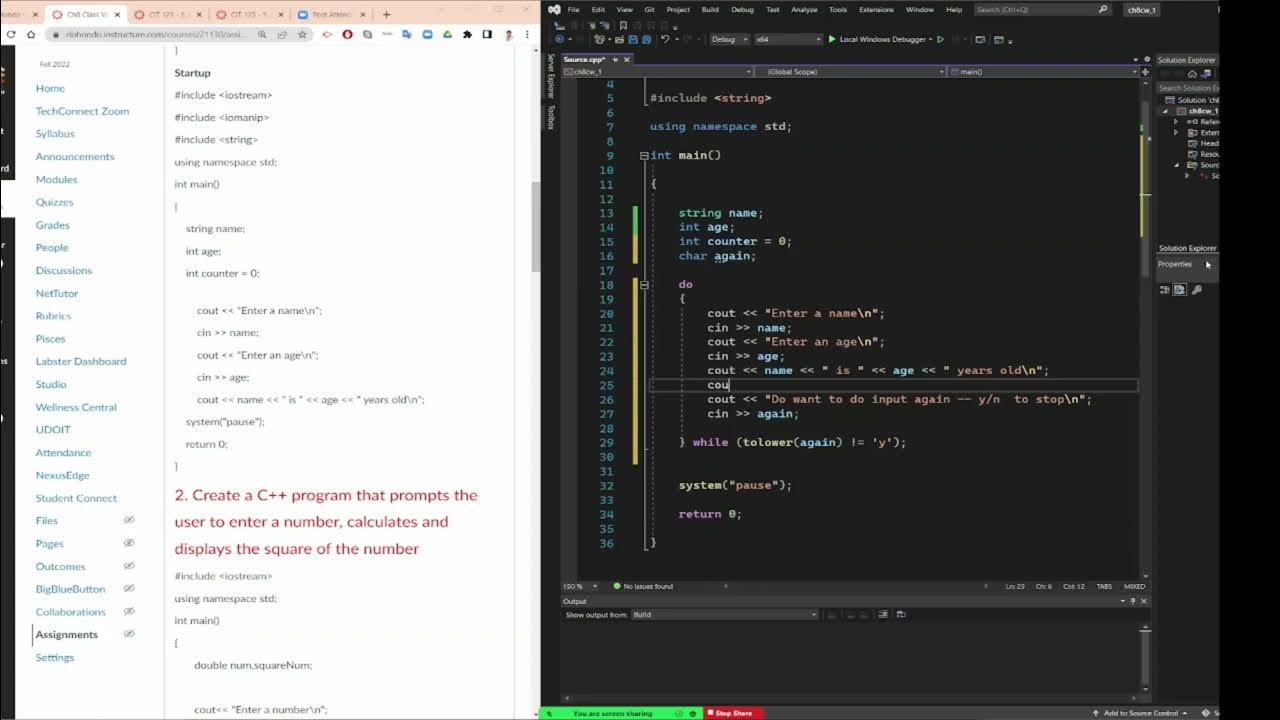
C++ programming , read a name and an age repeatedly with do while loop
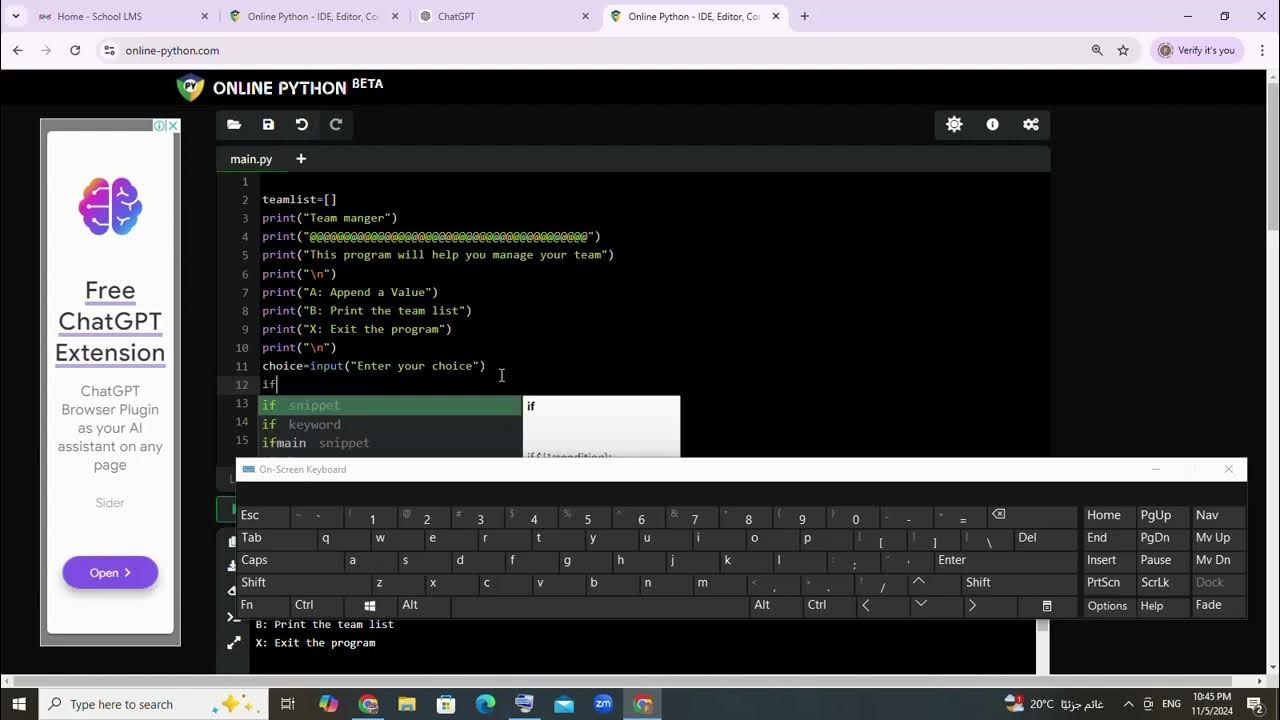
Team Manager Interface Program-Lists in Python
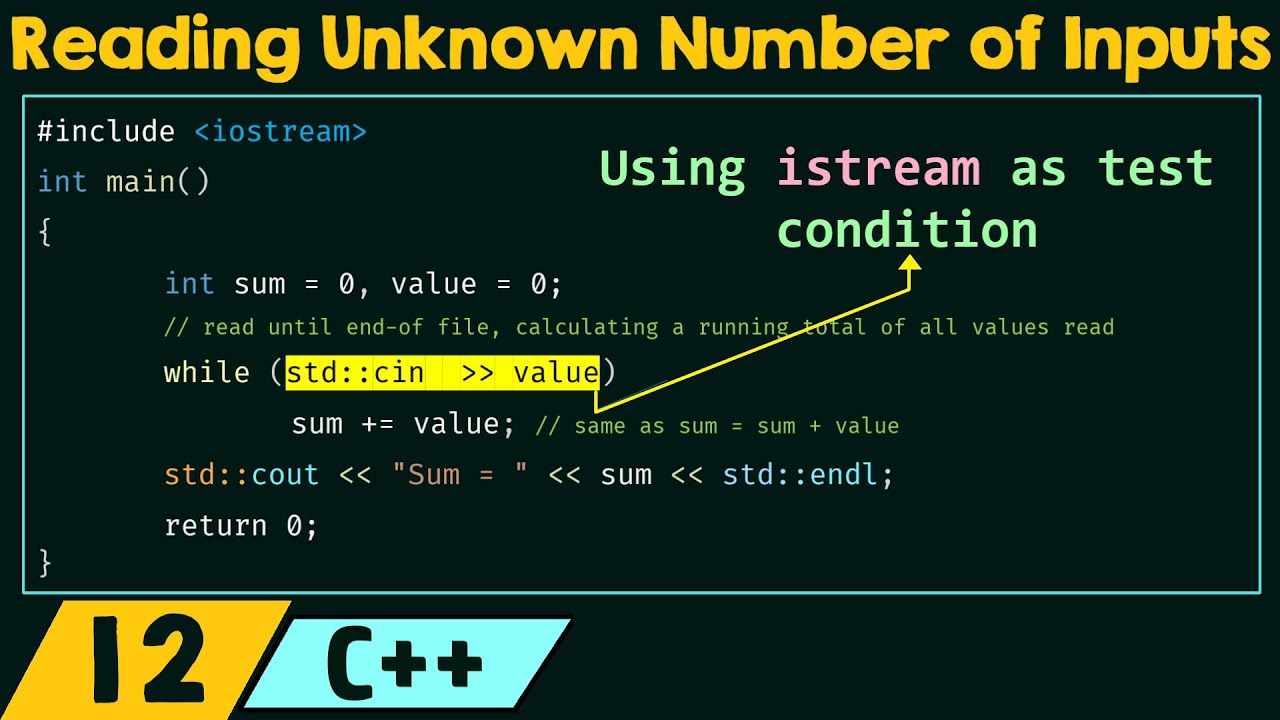
Reading an Unknown Number of Inputs in C++
5.0 / 5 (0 votes)