Node.js Tutorial - 24 - Streams and Buffers
Summary
TLDRThis video delves into the concepts of streams and buffers in computing. Streams are sequences of data transferred over time, like internet video streams or file transfers within a computer, which are processed in chunks as they arrive. Buffers, likened to a roller coaster's waiting area, are small storage areas in Node.js that hold incoming data until it's ready for processing. The video also connects these concepts to binary data, character sets, and encoding, illustrating how Node.js uses buffers to handle raw binary data, and demonstrates basic buffer operations in JavaScript.
Takeaways
- 🌐 Streams are sequences of data that move from one point to another over time, such as data over the Internet or between files within a computer.
- 🔁 Node.js processes data in chunks as it arrives in streams, rather than waiting for the entire data to be available, which is efficient for streaming services like YouTube.
- 📦 Buffers in Node.js are like a small waiting area for data that can't be processed immediately, similar to how people wait in line for a roller coaster ride.
- 🚀 Buffers help manage the flow of data by storing incoming data until it's ready to be processed, improving efficiency and preventing unnecessary memory usage.
- 🔢 A buffer in Node.js is represented by an array of numbers, each corresponding to the Unicode character code of the string being stored.
- 💾 Node.js provides the Buffer feature globally, so it can be used without importing it, making it a fundamental part of Node.js's data handling.
- 🔡 The string 'vishwas' can be converted into a buffer, which shows the hexadecimal representation of the binary data when logged to the console.
- 🔄 Buffers can be written to, but they have limited memory, so writing more data than the buffer can hold will overwrite existing data.
- 🔤 The `toString` method can be used to get the string representation of the binary data stored in a buffer.
- 🔄 Understanding the foundational concepts of binary data, character sets, and encoding is crucial for a deep understanding of Node.js's buffer operations.
- 📚 While not always necessary to work with buffers directly, having a solid grasp of these concepts helps in forming a comprehensive mental model of Node.js and its capabilities.
Q & A
What is a stream in the context of data processing?
-A stream is a sequence of data that is being moved from one point to another over time, such as data transferred over the Internet or within a computer.
Why is it beneficial to process data in chunks as it arrives, rather than waiting for the entire data?
-Processing data in chunks as it arrives prevents unnecessary data downloads and memory usage, allowing for efficient handling of data without delays.
What is the role of buffers in handling data streams?
-Buffers act as a temporary storage area where data is held before it is processed. They help manage data flow by storing incoming data until it can be processed.
How does the analogy of a roller coaster explain the concept of a buffer?
-The roller coaster analogy demonstrates how buffers manage data flow by comparing it to people waiting in line for a ride. Just as people wait in a queue, data waits in a buffer until it's ready to be processed.
What is the relationship between character encoding and buffers?
-Character encoding determines how numbers representing characters are converted into binary data, which is then stored in buffers. Buffers hold the raw binary data that corresponds to characters.
How does Node.js represent the binary data in a buffer when logged to the console?
-Node.js represents the binary data in a buffer as hexadecimal or base 16 notation when logged to the console, which is more manageable than printing 8-bit binary for every character.
What happens when you write to a buffer in Node.js?
-Writing to a buffer in Node.js replaces the existing data with new data up to the buffer's capacity. If the new data exceeds the buffer's size, it overwrites the existing data partially or completely.
Why is it important to understand buffers when learning about Node.js?
-Understanding buffers is crucial as they are a fundamental part of Node.js's internal workings. Even though you might not interact with them directly, they are key to forming a comprehensive mental model of Node.js.
What is the default character encoding used by Node.js when creating a buffer from a string?
-The default character encoding used by Node.js when creating a buffer from a string is UTF-8.
How can you convert a buffer back to a string representation in Node.js?
-You can convert a buffer back to a string representation in Node.js using the `toString` method on the buffer object.
Outlines
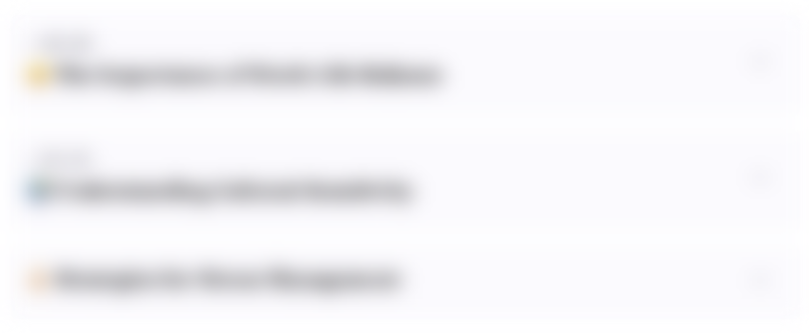
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
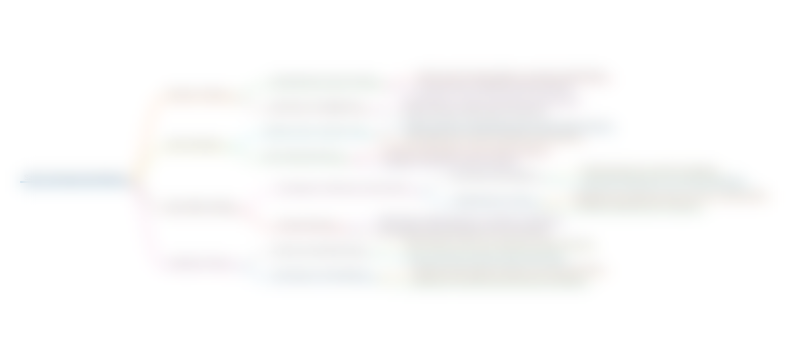
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
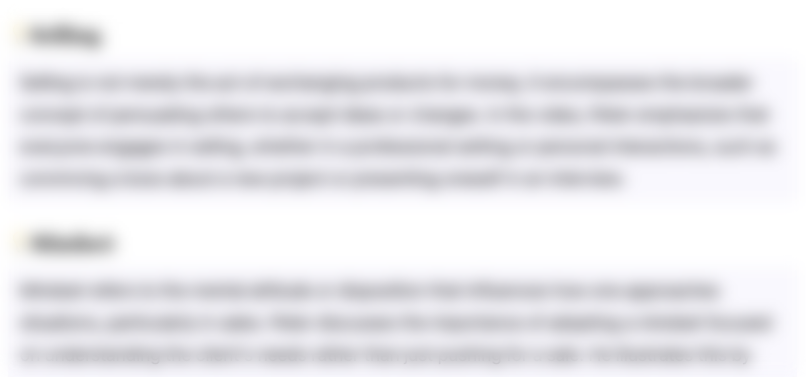
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
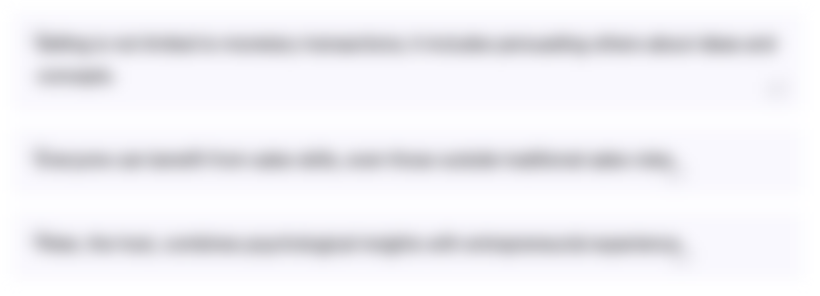
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
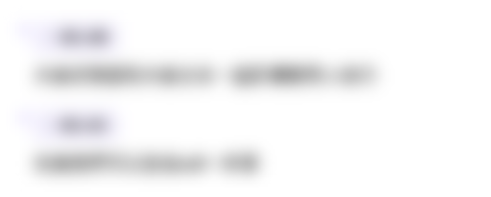
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
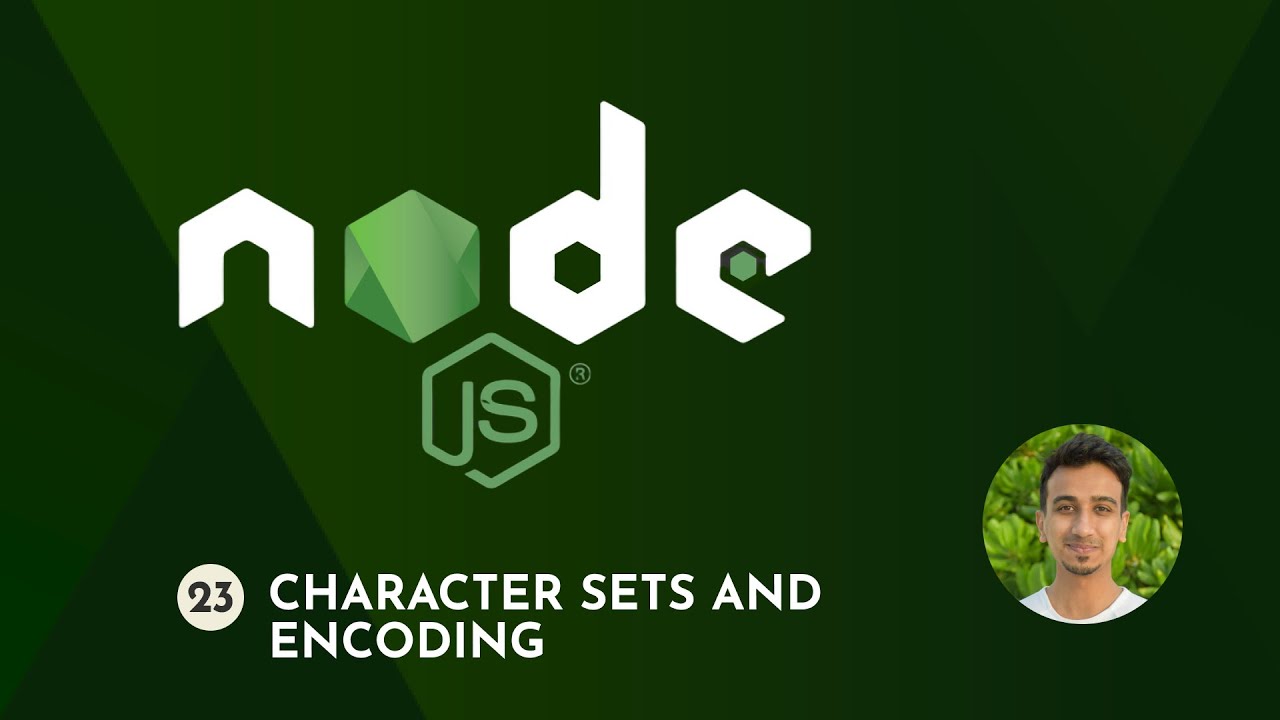
Node.js Tutorial - 23 - Character Sets and Encoding
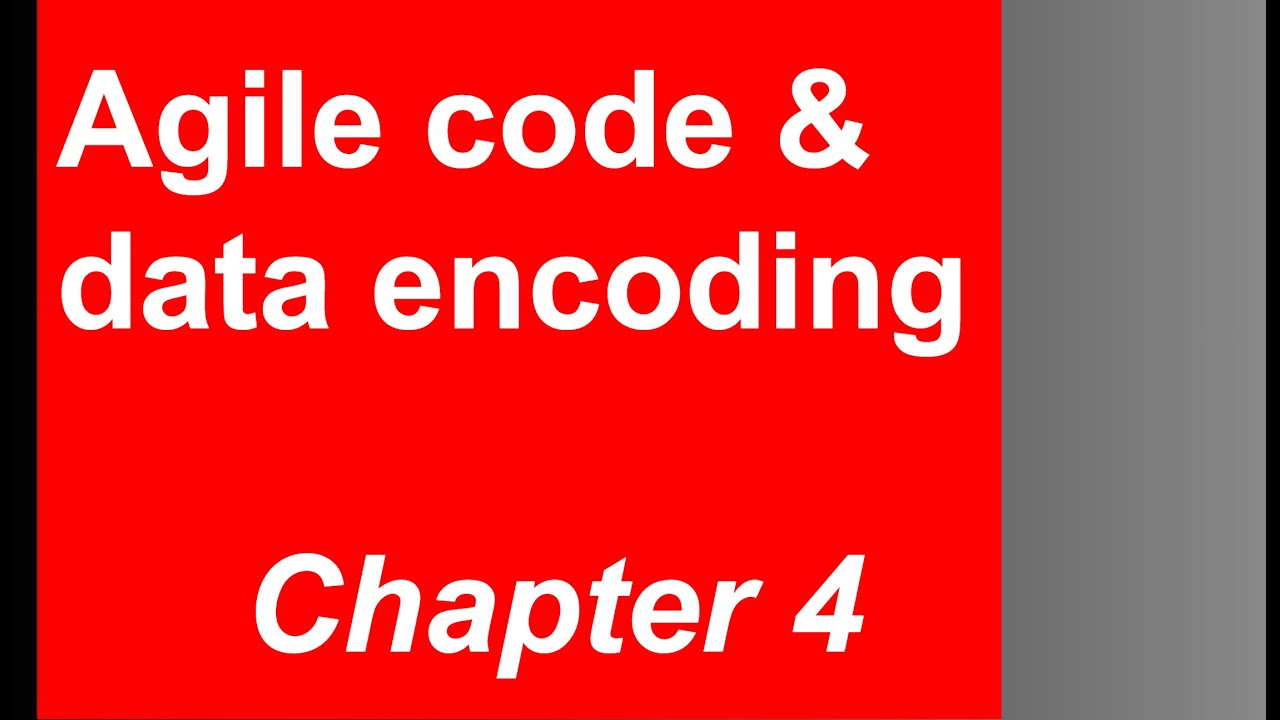
Chapter 4 - Agile code evolution, data encoding - Designing Data Intensive applications book review

Google SWE teaches systems design | EP28: Time Series Databases
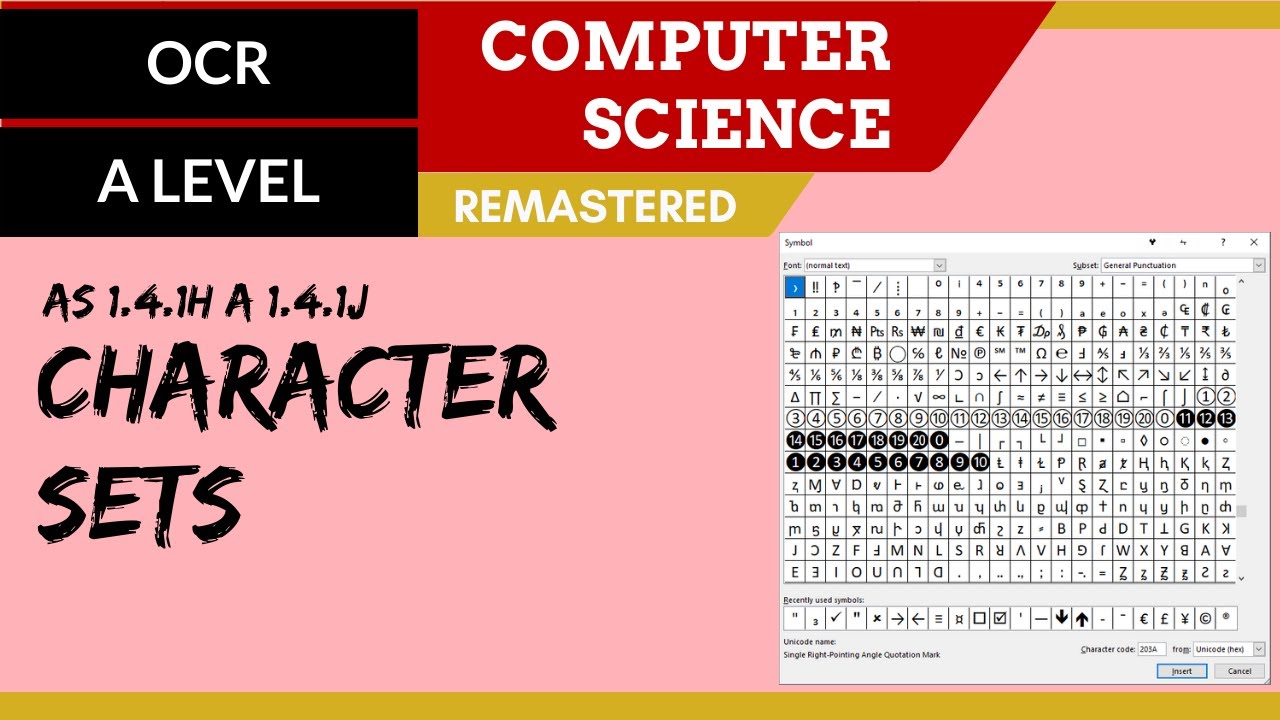
84. OCR A Level (H046-H446) SLR13 - 1.4 Character sets
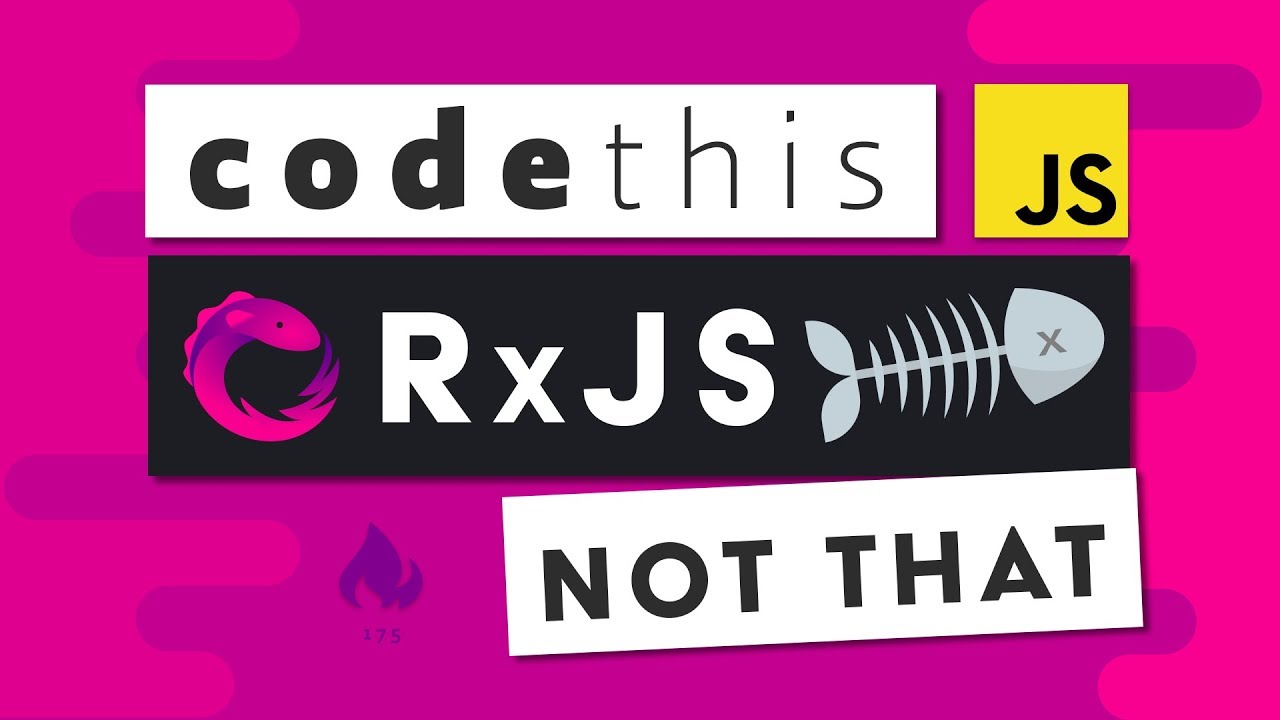
RxJS Top Ten - Code This, Not That
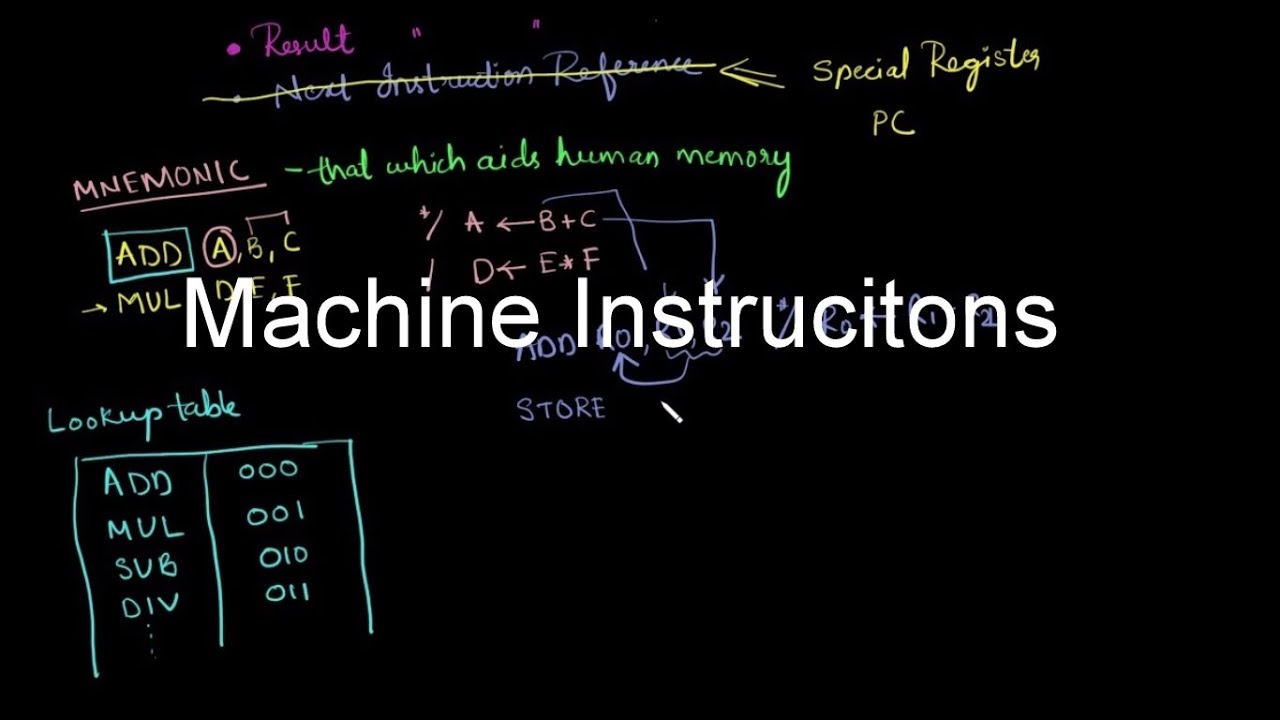
Gate Computer Organization-14 | Machine Instructions
5.0 / 5 (0 votes)