La diferencia de usar forEach() y map() en javascript #es6
Summary
TLDRThis Javascript tutorial highlights the differences between 'forEach' and 'map', two array iteration functions. It emphasizes their role in array manipulation, with 'forEach' executing a provided function for each array element and 'map' returning a new array with the results of calling a provided function on every element. The tutorial aims to clarify their distinct uses, helping developers choose the appropriate function for their coding needs.
Takeaways
- 📚 Both `forEach` and `map` are array methods in JavaScript.
- 🔄 They are used for iterating over arrays, but they serve different purposes.
- 🛠 `forEach` executes a provided function once for each array element, without returning a new array.
- 🗺️ `map` creates a new array with the results of calling a provided function on every element in the calling array.
- 🔑 `forEach` is typically used when the main goal is to perform an action for each element, without needing to create a new array.
- 🌟 `map` is ideal when you need to transform each element of the array and want to get a new array with these transformations.
- 📈 The `map` function is often used for creating new arrays based on the original array's elements, such as in data processing or transformation tasks.
- 🔄 The `forEach` method does not support breaking out of the loop or skipping elements, unlike some other iteration methods.
- 🔧 Both methods can be used with various types of iterable objects, not just arrays.
- 📝 Understanding the differences between `forEach` and `map` is crucial for choosing the right method for specific tasks in JavaScript programming.
- 💡 Mastery of array methods like `forEach` and `map` can lead to more efficient and readable code.
Q & A
What are the two array functions discussed in the Javascript tutorial?
-The two array functions discussed in the tutorial are forEach and map.
What is the commonality between forEach and map functions?
-Both forEach and map functions are part of the set of array functions in Javascript and are used to iterate over arrays.
Can you use forEach and map to transform an array into a new one?
-While forEach is used to execute a function for each item in an array without creating a new array, map is used to transform each item of the original array into a new array.
Is forEach suitable for array manipulation tasks?
-No, forEach is not suitable for array manipulation tasks as it does not return a new array; it is used for executing a function on each item.
What is the primary purpose of the map function?
-The primary purpose of the map function is to create a new array by transforming each item of the original array according to a provided function.
Can forEach be used to perform conditional operations on array elements?
-Yes, forEach can be used to perform conditional operations on array elements by including conditional statements within the function passed to forEach.
Is it possible to use map to apply a function that does not return a value?
-No, the map function is specifically designed to apply a function that returns a value to each item in the array, creating a new array.
What happens to the original array when you use forEach or map?
-The original array remains unchanged when using either forEach or map, as both functions do not modify the original array but work with its elements.
How can you determine which function to use, forEach or map, when iterating over an array?
-You should use forEach when you need to perform operations on each element without creating a new array, and use map when you need to create a new array with the results of the operations performed on each element.
Can forEach and map be used with any type of iterable, not just arrays?
-While forEach and map are commonly used with arrays, they can also be used with other iterable objects, such as NodeLists or strings.
Outlines
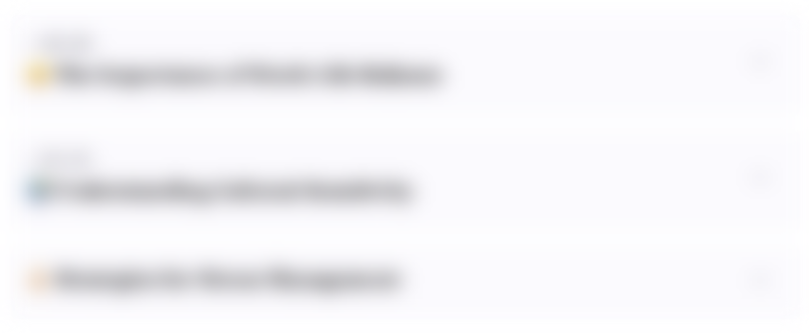
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
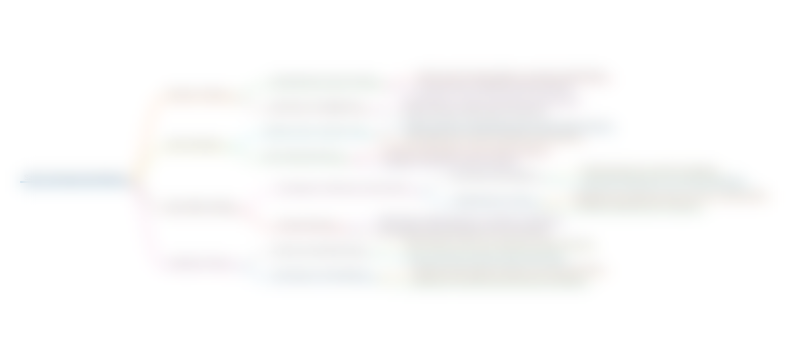
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
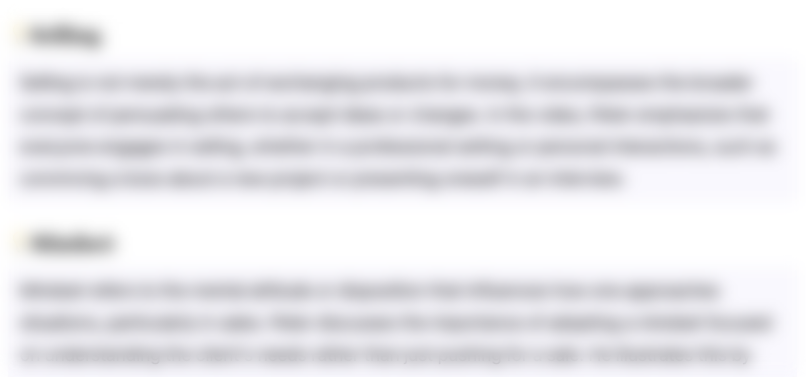
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
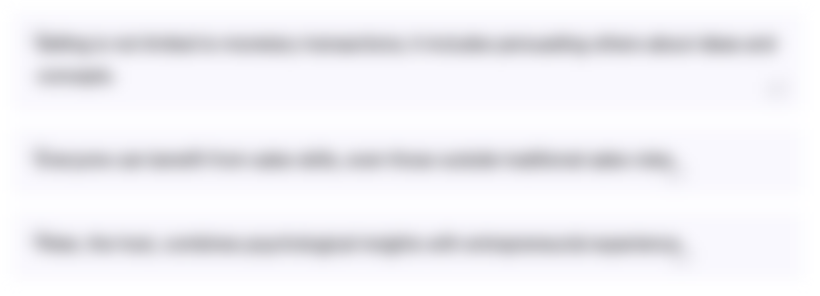
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
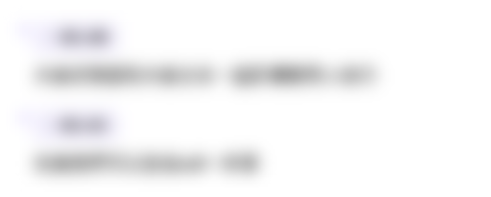
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频

JavaScript forEach vs. map — When To Use Each and Why
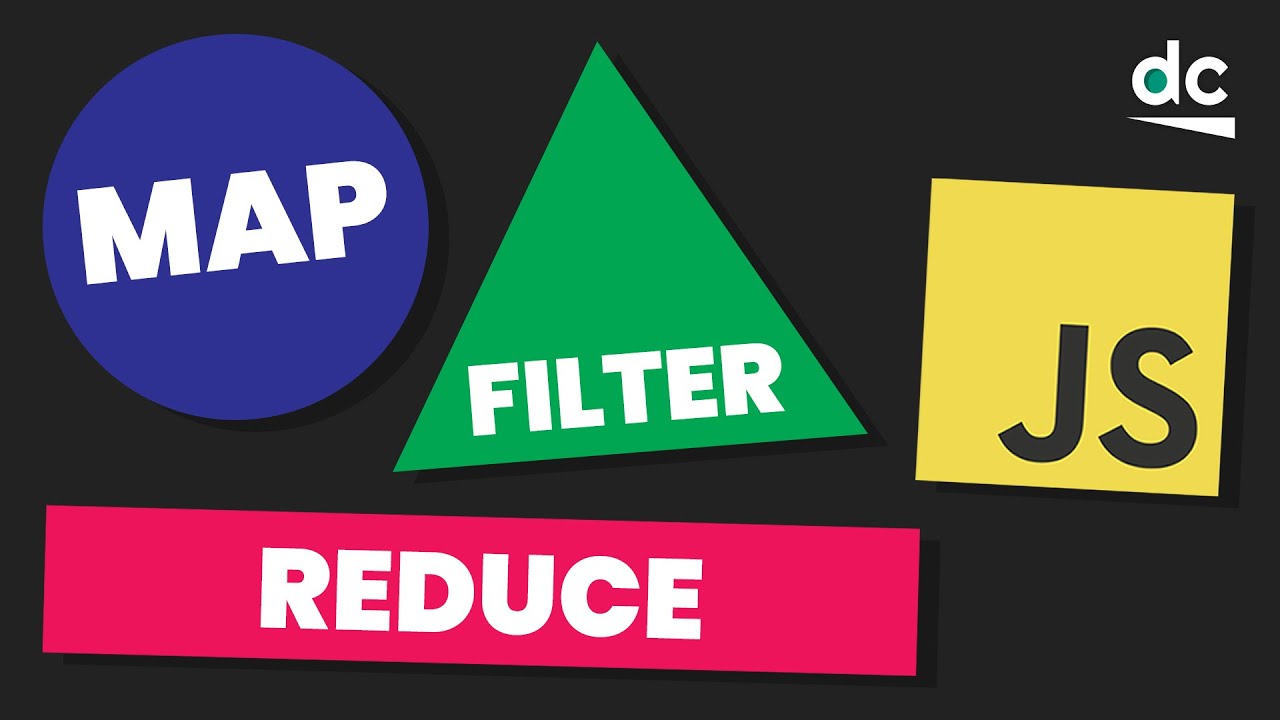
Map, Filter & Reduce EXPLAINED in JavaScript - It's EASY!

How to Design 4 elements (1×4) Patch Antenna Array Using CST Studio?|Quarter Wave Transformer Method
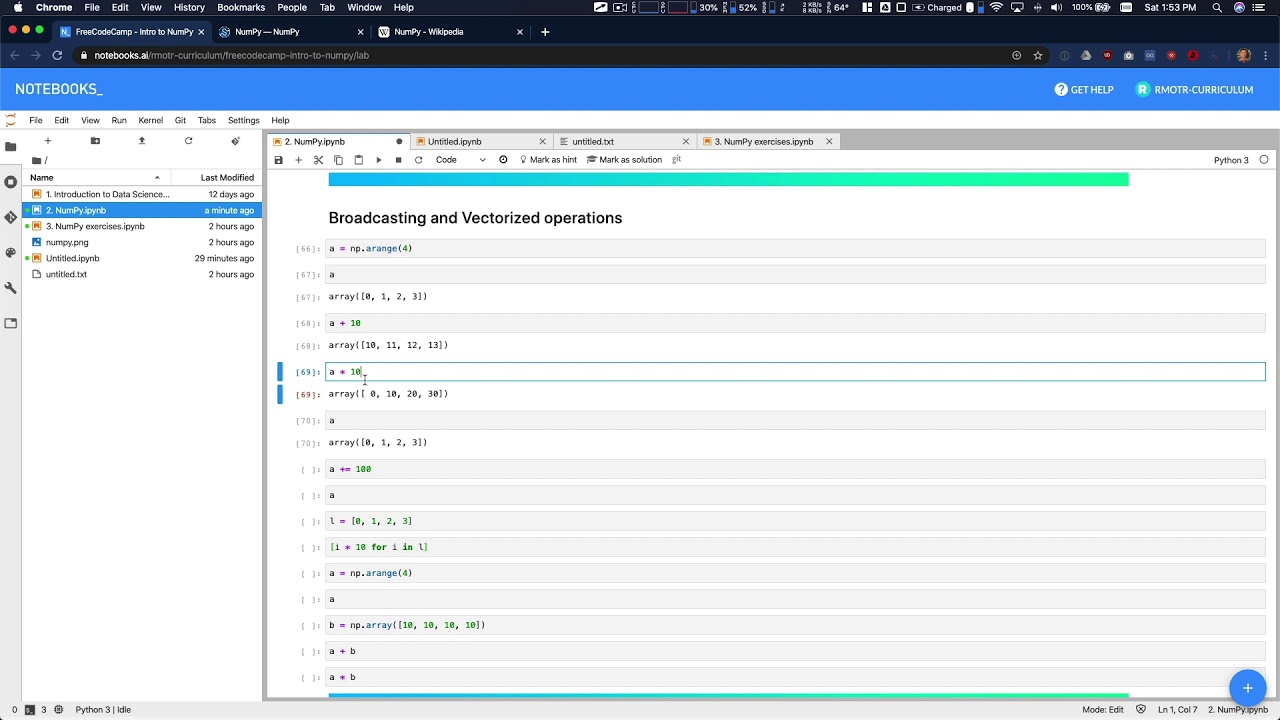
Numpy Operations - Data Analysis with Python Course
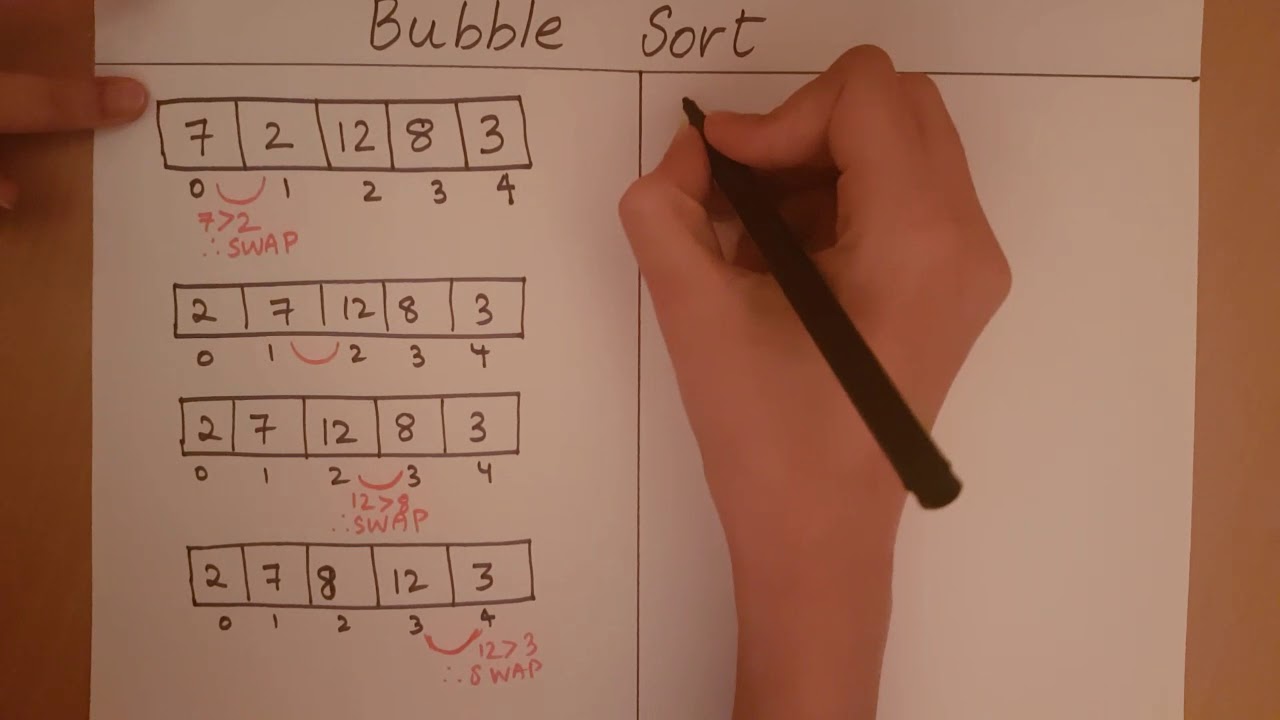
Introduction to Bubble Sort
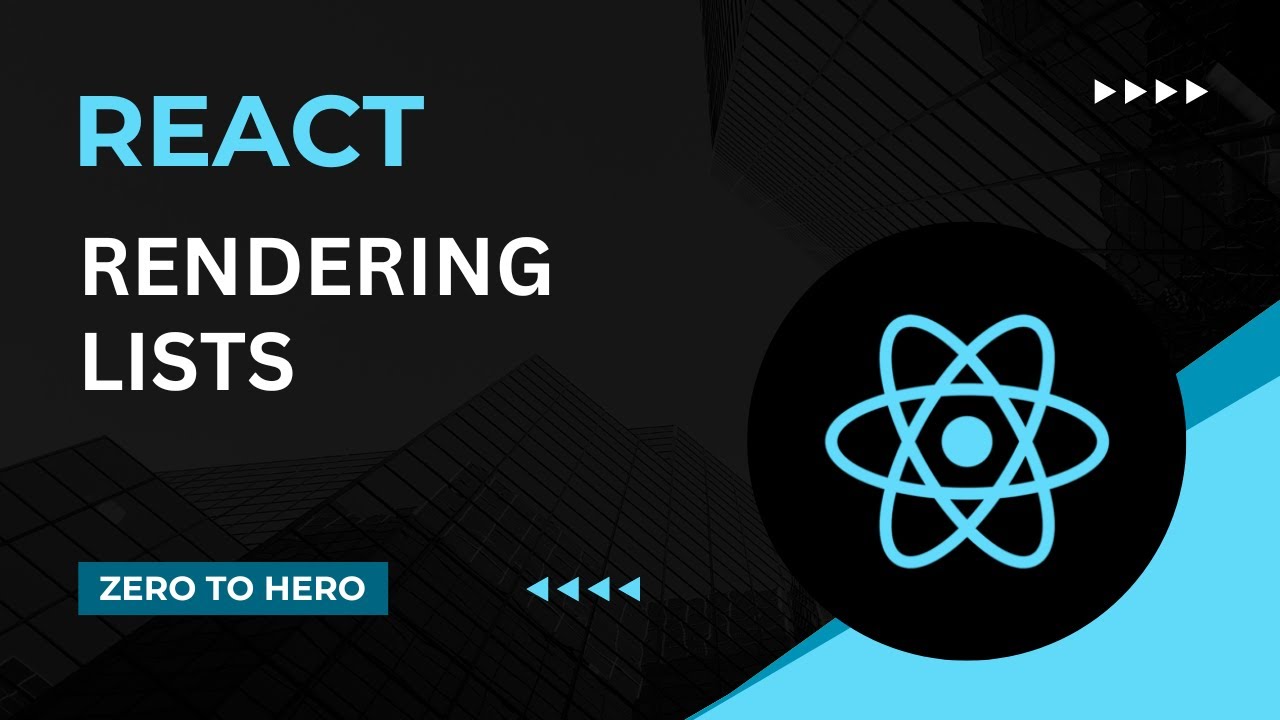
How to render lists | Mastering React: An In-Depth Zero to Hero Video Series
5.0 / 5 (0 votes)