#68 What is an Observable | Understanding Observables & RxJS | A Complete Angular Course
Summary
TLDRThis section of the Angular course delves into the concept of observables, essential for handling asynchronous data in JavaScript. It contrasts observables with promises, highlighting their ability to manage data streams, unlike promises which offer a single resolution. The script explains that observables, provided by the RxJS library, use the Observer pattern to emit events that subscribers can react to. The lecture promises a practical demonstration of creating and utilizing observables in Angular applications, emphasizing their non-blocking nature and suitability for tasks like streaming large data sets.
Takeaways
- 📚 Observables are used in Angular to handle asynchronous data, similar to how promises are used in JavaScript.
- 🔁 JavaScript is a single-threaded language, meaning it executes code line by line, and long tasks can block subsequent code execution.
- 🌐 Asynchronous operations, like HTTP requests, can take time and are non-blocking, allowing the main thread to continue executing other code.
- 🚀 Promises can handle asynchronous data but are limited to returning a single value or error, unlike observables.
- 📡 Observables can handle a stream of data, emitting multiple values over time, which is useful for applications like video streaming.
- 🔄 The difference between promises and observables lies in their ability to handle data streaming, with observables being more suited for this purpose.
- 🛑 Promises will always return data or an error, regardless of whether it is used, while observables will only emit data if there is a subscriber.
- 📚 Observables are not a native feature of JavaScript or Angular but are provided by the RxJS library, which uses the Observer pattern.
- 🔄 The Observer pattern involves an observable that emits events and an observer that listens and reacts to these events.
- 🛠 RxJS is a library for working with asynchronous data streams, providing methods to manipulate and work with observables.
- 🔍 The script promises to cover the creation and usage of observables in Angular applications in the next lecture.
Q & A
What is an observable in the context of Angular?
-An observable is a mechanism used to handle asynchronous data in Angular. It is a way to represent a stream of data that can be observed and processed over time.
Why do we need observables in JavaScript, considering it already has promises?
-While promises are useful for handling asynchronous data, observables offer more flexibility, especially when dealing with streams of data where multiple values are emitted over time, unlike promises which resolve with a single value or error.
How does JavaScript's single-threaded nature affect the execution of asynchronous operations?
-JavaScript's single-threaded nature means that long-running tasks can block the execution of subsequent code. Asynchronous operations, like HTTP requests, prevent this blocking by running in the background, allowing the main thread to continue executing other code.
What is the difference between synchronous and asynchronous code in terms of their effect on the main thread?
-Synchronous code is blocking; it must complete before the next line of code can execute. Asynchronous code, on the other hand, is non-blocking, allowing other operations to proceed on the main thread while waiting for the asynchronous operation to complete.
Can you explain the concept of streaming data as mentioned in the script?
-Streaming data refers to the process of sending large amounts of data in small chunks or packets over time, rather than all at once. This is useful for applications like video streaming, where users can start consuming the content before the entire file has been downloaded.
How does a promise handle a stream of data?
-A promise is not designed to handle a stream of data. It resolves or rejects with a single value or error when the asynchronous operation completes, making it unsuitable for scenarios where multiple data chunks are expected.
What is the main advantage of using observables over promises when dealing with streams of data?
-Observables can emit multiple values over time, allowing them to handle streams of data effectively. They can emit new data as it arrives, making them ideal for applications that require real-time data updates or processing.
Why does an observable only provide data if there is someone to use it?
-Observables are designed to be lazy, meaning they will only emit data when there is an observer (subscriber) listening to it. This helps in optimizing performance and resource usage by avoiding unnecessary data processing.
What is the relationship between observables and the RxJS library?
-Observables are not a native feature of JavaScript or Angular; they are provided by the RxJS library, which stands for 'Reactive Extensions for JavaScript'. RxJS offers a suite of tools and methods for working with observables and asynchronous data streams.
Can you briefly describe the Observer pattern as it relates to observables?
-The Observer pattern involves an observable (or subject) that emits events and one or more observers (subscribers) that listen for these events. When an event is emitted, the observers can react to it by executing specific logic or functions, such as handling data with the 'next' callback, or responding to errors or completion with their respective callbacks.
What are the types of events that an observable can emit in RxJS?
-In RxJS, an observable can emit three types of events: 'next' for emitting data, 'error' for signaling an error condition, and 'completion' to indicate that the observable has finished emitting data.
Outlines
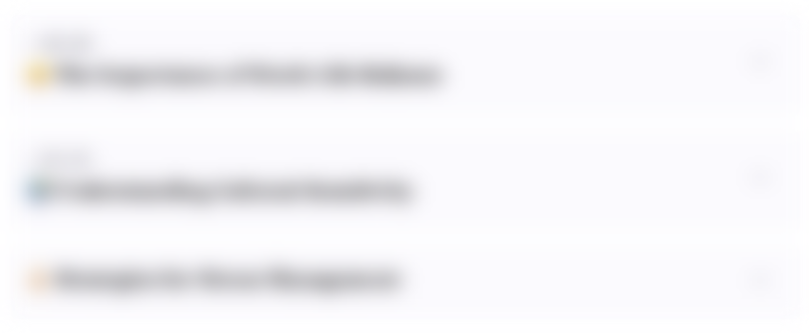
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
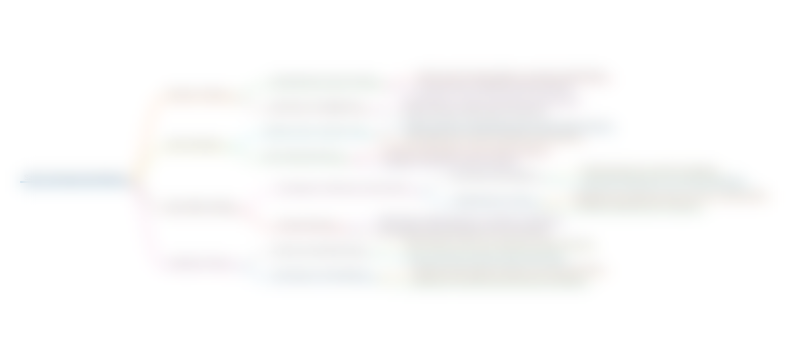
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
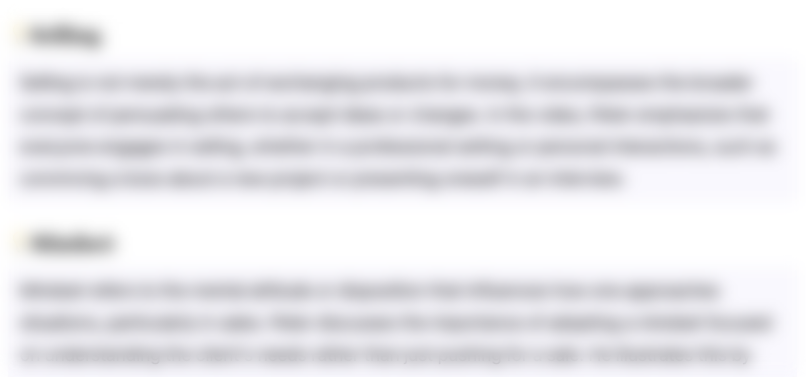
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
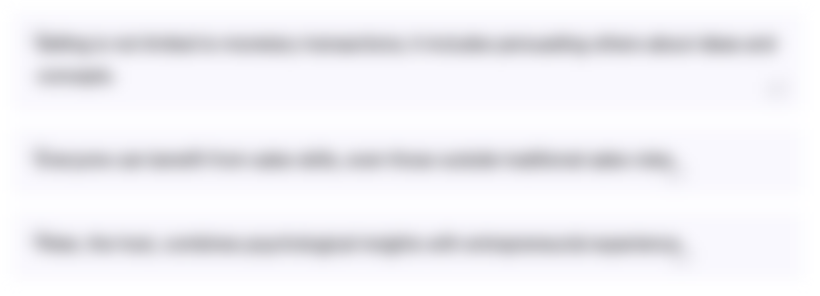
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
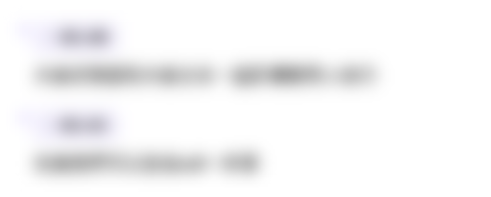
此内容仅限付费用户访问。 请升级后访问。
立即升级5.0 / 5 (0 votes)