Please Master These 10 Python Functions…
Summary
TLDRThis script offers an in-depth exploration of ten essential Python functions that every programmer should master. Starting with the versatile 'print' function, it covers its advanced parameters like 'Sep' and 'end'. The script then introduces the 'help' function for quick access to documentation, followed by 'range', 'map', 'filter', 'sum', 'sorted', 'enumerate', 'zip', and the file handling 'open' function. Each function is explained with examples and use cases, emphasizing their efficiency and utility in coding, concluding with best practices for file operations using context managers.
Takeaways
- 📝 The `print` function in Python is versatile, allowing for customization of separators and end characters to control the output format.
- 🔍 The `help` function serves as an in-built documentation tool, providing quick access to a function's docstrings without needing to search online.
- 🔢 The `range` function is essential for generating sequences of numbers with customizable start, stop, and step values, and can be converted to a list for direct usage.
- 🗺️ The `map` function applies a given function to every item in an iterable, streamlining the process of performing operations across collections.
- 📝 The `filter` function selectively filters items in an iterable based on a provided function that returns a boolean value, deciding whether to include each item.
- 🌐 The `sum` function quickly calculates the total of numbers in an iterable, with the option to set a custom starting value for the summation.
- 🔄 The `sorted` function sorts iterables in ascending or descending order, with the ability to define custom sort keys through a function.
- 🔑 The `enumerate` function pairs each item in an iterable with its index, simplifying loops that require both index and value access.
- 🔗 The `zip` function combines multiple iterables into a single iterable of tuples, efficiently merging corresponding elements from each iterable.
- 📖 The `open` function is crucial for file operations, offering different modes for reading, writing, and appending, with the emphasis on using context managers for proper resource management.
Q & A
What is the primary purpose of the 'print' function in Python?
-The 'print' function in Python is used to display output to the console or terminal. It can handle a mix of strings and variables, and allows for customization of the separator and end parameters to control the format of the output.
How can the 'print' function's default behavior of separating arguments with a space be changed?
-The default behavior of the 'print' function can be changed by passing a different value to the 'sep' argument. For example, setting 'sep' to a pipe character '|' will replace the space with a pipe between arguments.
What does the 'end' argument in the 'print' function do?
-The 'end' argument in the 'print' function determines what is printed at the end of the line. By default, it adds a newline character (' '), but it can be changed to any string, allowing for printing on the same line consecutively.
What is the 'help' function in Python and how is it used?
-The 'help' function in Python is used to display the documentation of a given function or module. It reads the docstrings to provide information on usage and parameters, which is useful for quickly accessing documentation without needing to search online.
Can the 'help' function be used with user-defined functions?
-Yes, the 'help' function can be used with user-defined functions as well. It prints the docstring associated with the function, which should be provided when the function is defined to describe its purpose and usage.
What is the 'range' function in Python and how does it work?
-The 'range' function in Python generates a sequence of numbers. It can take one, two, or three arguments for start, stop, and step values, respectively. By default, it starts at 0 and steps by 1, going up to but not including the stop value.
How can the 'map' function be used to apply a function to every item in an iterable?
-The 'map' function takes a function and an iterable as arguments, then applies the function to each item in the iterable. The results are returned as a map object, which can be converted to a list to view all the results at once.
What is the purpose of the 'filter' function in Python?
-The 'filter' function is used to filter items in an iterable based on a given function. If the function returns True for an item, it is included in the result; otherwise, it is excluded.
What does the 'sum' function do and can it accept a starting value?
-The 'sum' function calculates the total sum of all numeric items in an iterable. It can accept an optional 'start' parameter, which allows the summation to begin from a value other than zero.
How does the 'sorted' function work and what arguments can be used to modify its behavior?
-The 'sorted' function sorts an iterable in ascending order by default. It can be modified by passing the 'reverse' argument set to True for descending order, or by using the 'key' argument with a function to determine the sorting criteria.
What is the 'enumerate' function used for and how does it simplify loops?
-The 'enumerate' function is used to iterate over an iterable while having access to both the index and the value of each item. It simplifies loops by returning a tuple containing the index and value for each iteration, eliminating the need for manual index tracking.
Can the 'zip' function be used to combine multiple lists and how does it handle lists of different lengths?
-Yes, the 'zip' function combines multiple iterables into a single iterable of tuples. It stops at the shortest iterable, thus handling lists of different lengths by not causing an index out of bounds exception.
What is the 'open' function used for and what are the common modes for file operations?
-The 'open' function is used to open a file for various operations like reading, writing, and appending. The common modes are 'r' for reading, 'w' for writing (which truncates the file), and 'a' for appending to the end of the file.
Why is it recommended to use a context manager with the 'open' function?
-Using a context manager with the 'open' function ensures that the file is properly closed after its operations are completed, even if an error occurs during the file manipulation process. It prevents memory leaks and ensures the file is not left open unintentionally.
Outlines
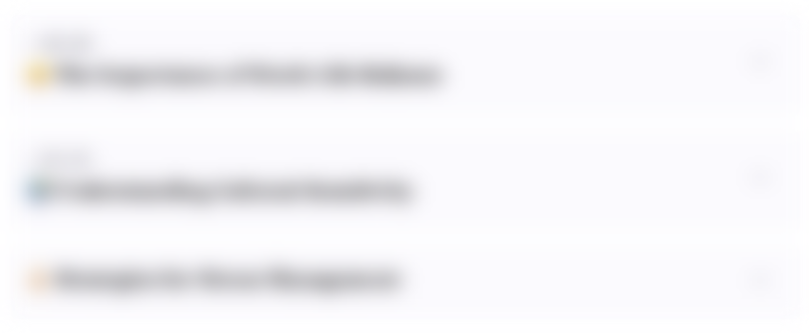
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
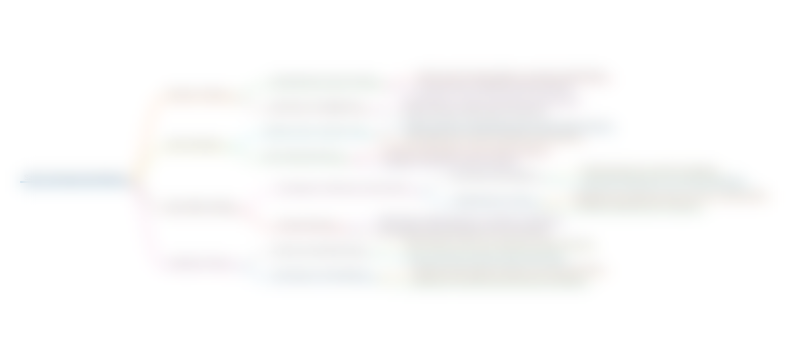
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
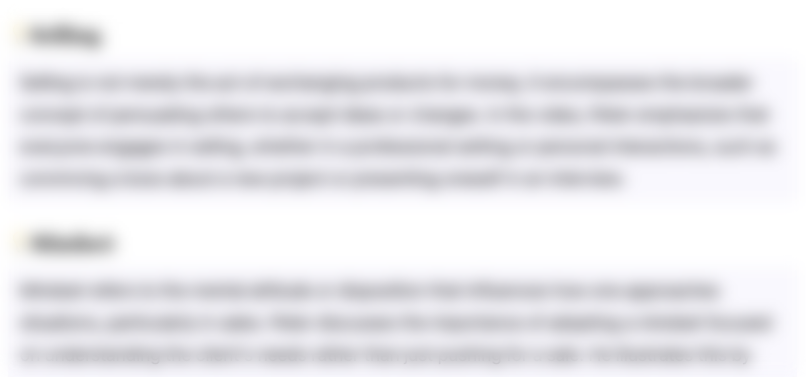
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
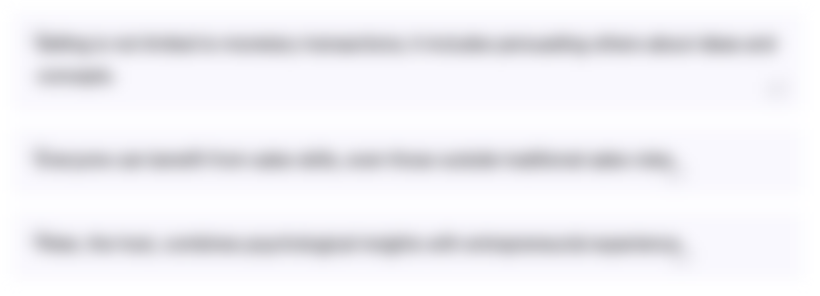
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
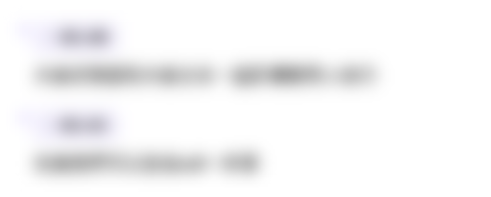
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
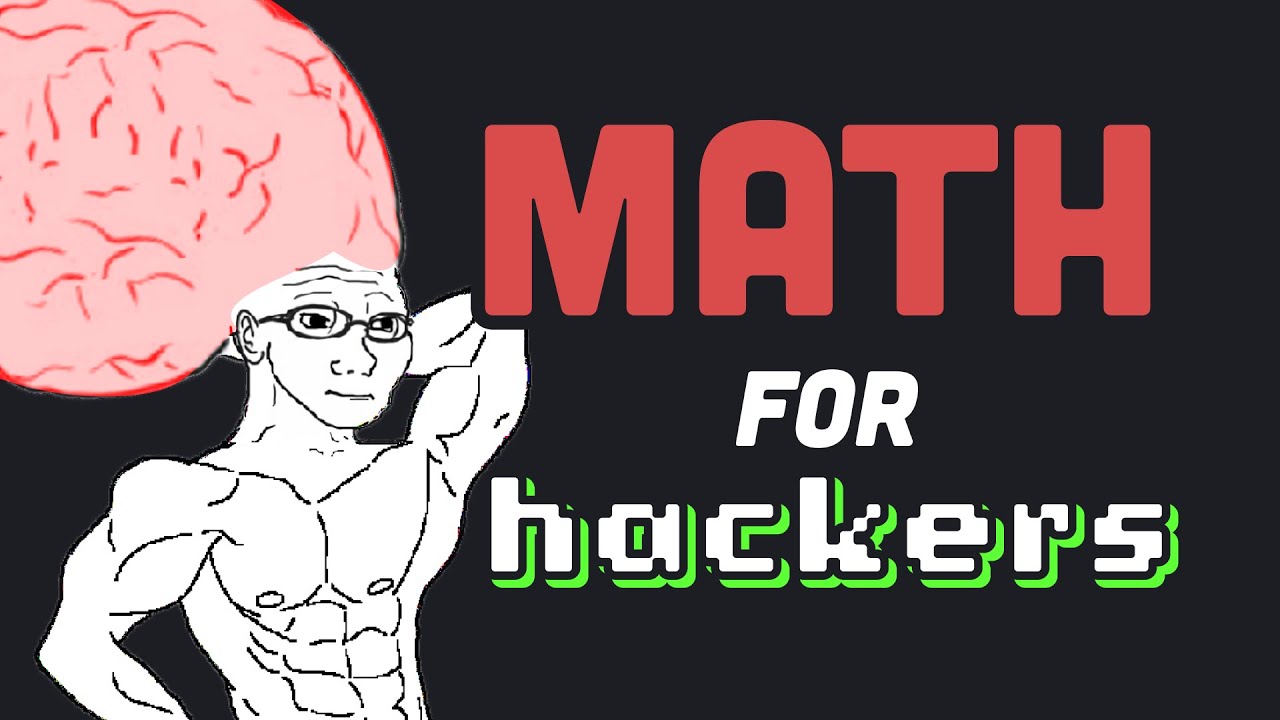
10 Math Concepts for Programmers
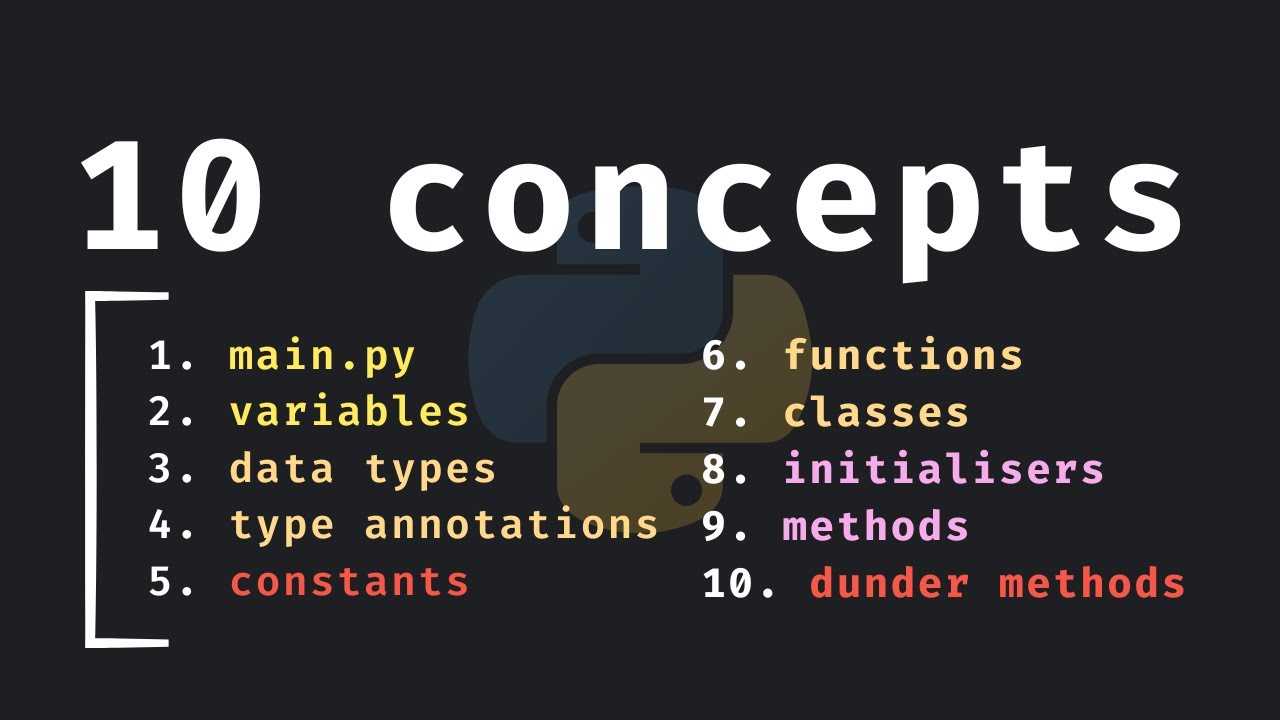
10 Important Python Concepts In 20 Minutes

How To Be A Great Facilitator - The 8 Facilitation Skills You Need (With Tips To Improve Them)

#48 parse and diff and check date
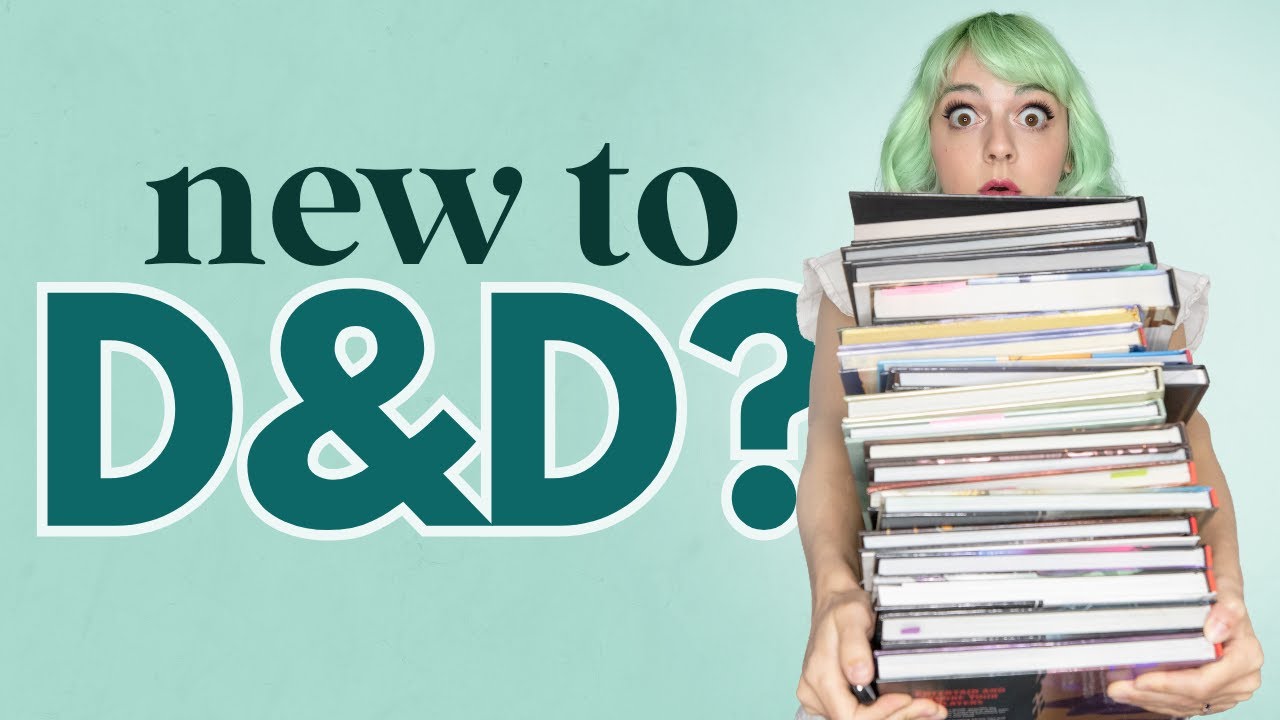
7 things ALL first-time D&D players need to know
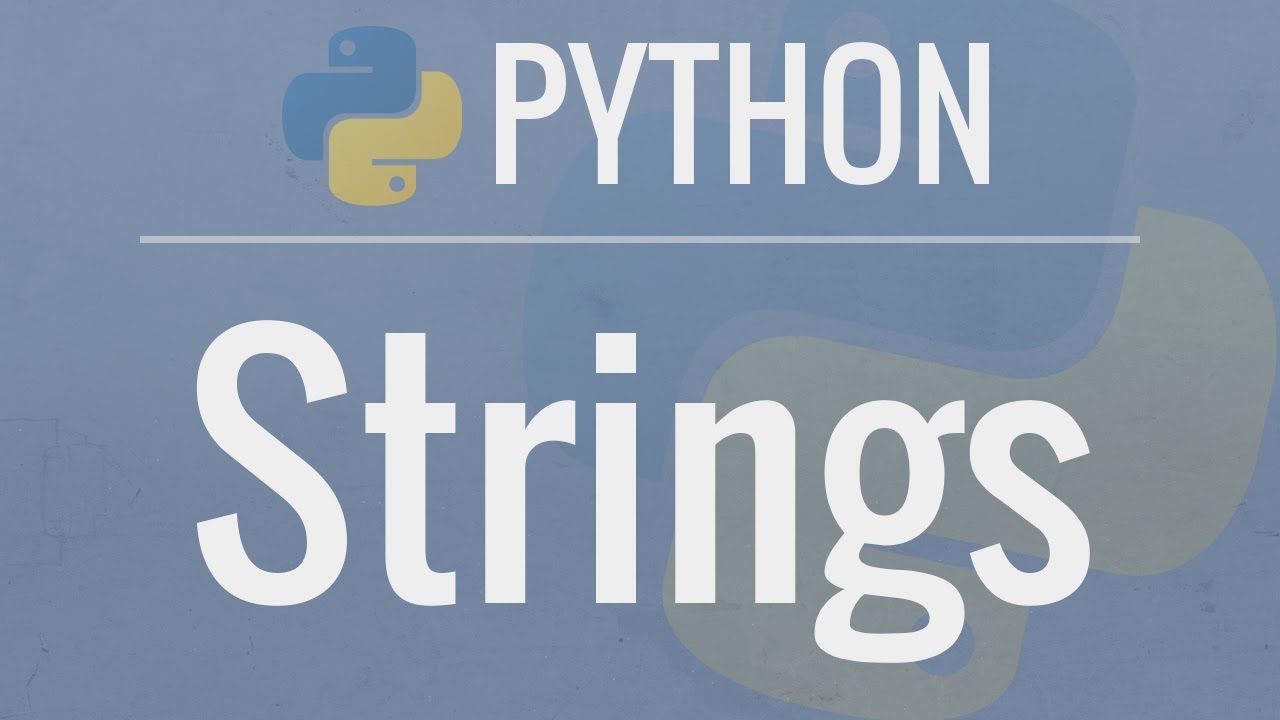
Python Tutorial for Beginners 2: Strings - Working with Textual Data
5.0 / 5 (0 votes)