File Handling in Java | Java program to create a File
Summary
TLDRThis video introduces file handling in Java, explaining how to create and manage files effectively. The tutorial covers the basics of file creation, reading, writing, and handling exceptions using methods like 'canWrite', 'createNewFile', and 'delete'. Viewers will learn to handle various file operations, such as checking if a file exists or handling I/O exceptions with 'try-catch' blocks. The video also demonstrates practical applications, showing how to create, read, and update files using Java classes and methods. It's an essential guide for anyone looking to work with files in Java programming.
Takeaways
- 😀 File handling in Java refers to managing file creation, reading, writing, and exception handling.
- 😀 The script explains how to handle files using Java's built-in classes and methods, such as 'createNewFile', 'read', and 'write'.
- 😀 A major focus is on handling exceptions during file operations, such as 'FileNotFoundException'.
- 😀 File handling allows the storage of data in files, which is essential for managing large datasets or persistent information.
- 😀 The process of handling files involves using various methods, including 'canRead', 'canWrite', and 'getName', to manipulate file properties.
- 😀 Java provides different input/output stream classes, categorized into 'byte streams' and 'character streams', for managing file data.
- 😀 The script demonstrates how to create and manage files, including handling cases where a file already exists.
- 😀 It covers the importance of exception handling and how 'try-catch' blocks can be used to manage runtime errors.
- 😀 The program creates a file and checks if it exists using methods like 'createNewFile' and 'exists'.
- 😀 Key methods like 'File.createNewFile()', 'File.delete()', and 'File.length()' are highlighted for their utility in managing files.
- 😀 The script also teaches how to handle file input/output operations by using streams for reading and writing data to a file.
Q & A
What is file handling in Java?
-File handling in Java refers to the process of working with files, such as creating, reading, writing, and managing data stored in files. It involves using specific classes and methods provided by Java to interact with files on the system.
Why is file handling important in modern programming?
-File handling is crucial because it allows programmers to store and manage data outside the program, ensuring data persistence. Files are used to store a wide range of information, such as user data, configuration settings, and logs.
What are some common exceptions encountered during file handling?
-Some common exceptions include `FileNotFoundException`, which occurs when trying to access a file that does not exist, and `IOException`, which handles errors during input or output operations, such as failure to read from or write to a file.
What is the role of the `createNewFile()` method in file handling?
-`createNewFile()` is used to create a new file in the specified location. If the file already exists, it returns false; otherwise, it creates the file and returns true.
How can you check if a file is already available before creating it?
-Before creating a file, you can use the `exists()` method from the `File` class to check if a file already exists at the specified location.
What are input and output streams in Java file handling?
-Input and output streams in Java are used for reading and writing data from or to a file. An input stream reads data from the file, while an output stream writes data to the file.
How does the `canWrite()` method work in file handling?
-`canWrite()` checks whether the file is writable or not. It returns true if the file can be written to, and false if it cannot be written to due to permissions or other restrictions.
What is the significance of using `try-catch` blocks in file handling?
-A `try-catch` block is used to handle exceptions that might occur during file operations, such as file not found or errors during reading and writing. It ensures that the program can continue executing even if an exception occurs.
What does the `length()` method do in file handling?
-`length()` returns the size of a file in bytes. It helps in determining the amount of data stored in a file, which can be useful for validating file content or checking the file's size before performing operations.
What should be done if a `FileNotFoundException` occurs in file handling?
-If a `FileNotFoundException` occurs, it can be handled by either creating the file if it doesn't exist, displaying an appropriate error message, or ensuring that the correct file path is provided.
Outlines
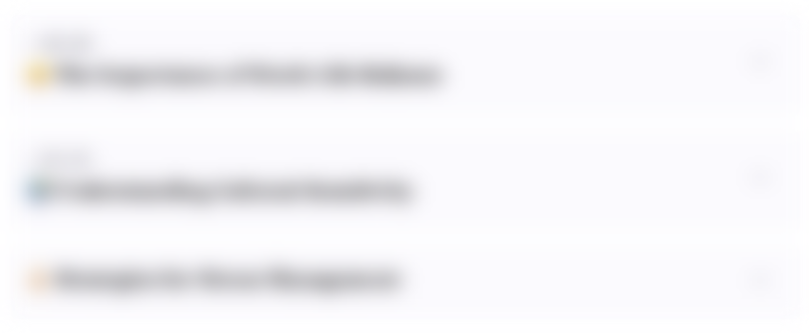
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
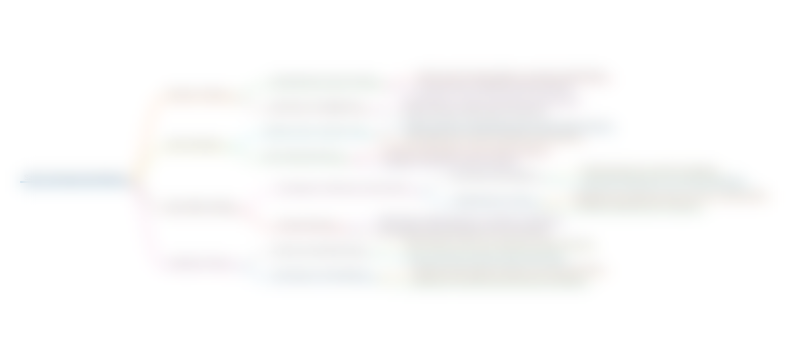
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
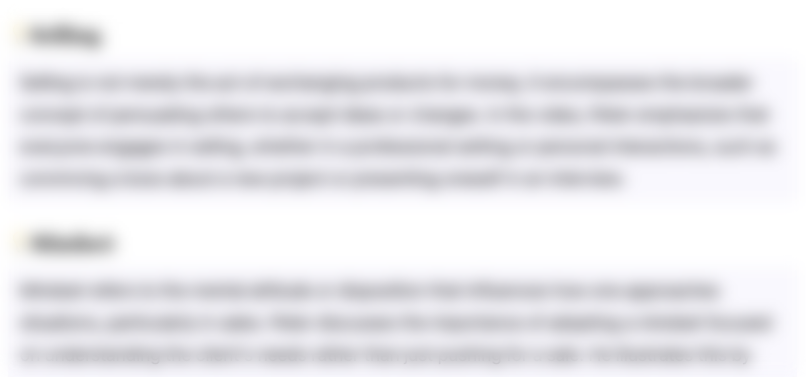
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
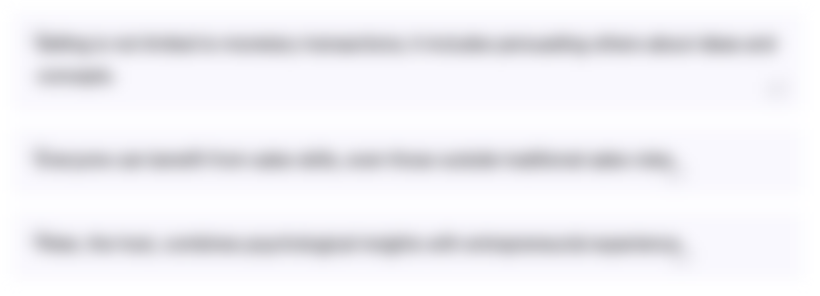
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
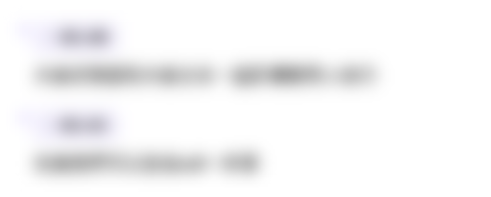
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
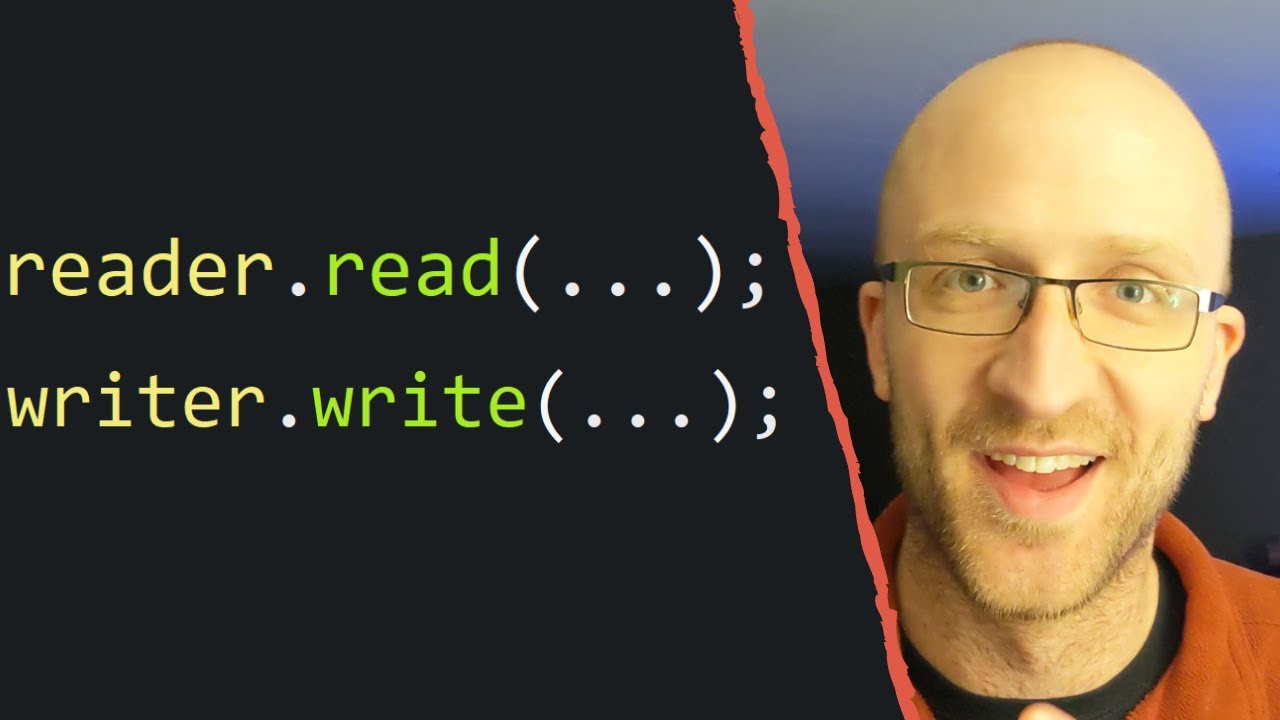
Java File Input/Output - It's Way Easier Than You Think
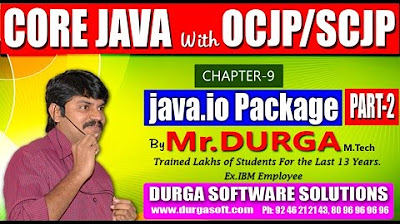
Core Java With OCJP/SCJP-java IO Package-Part 2 || File I/O

C_119 File Handling in C - part 1 | Introduction to Files

C++ File Handling | Learn Coding
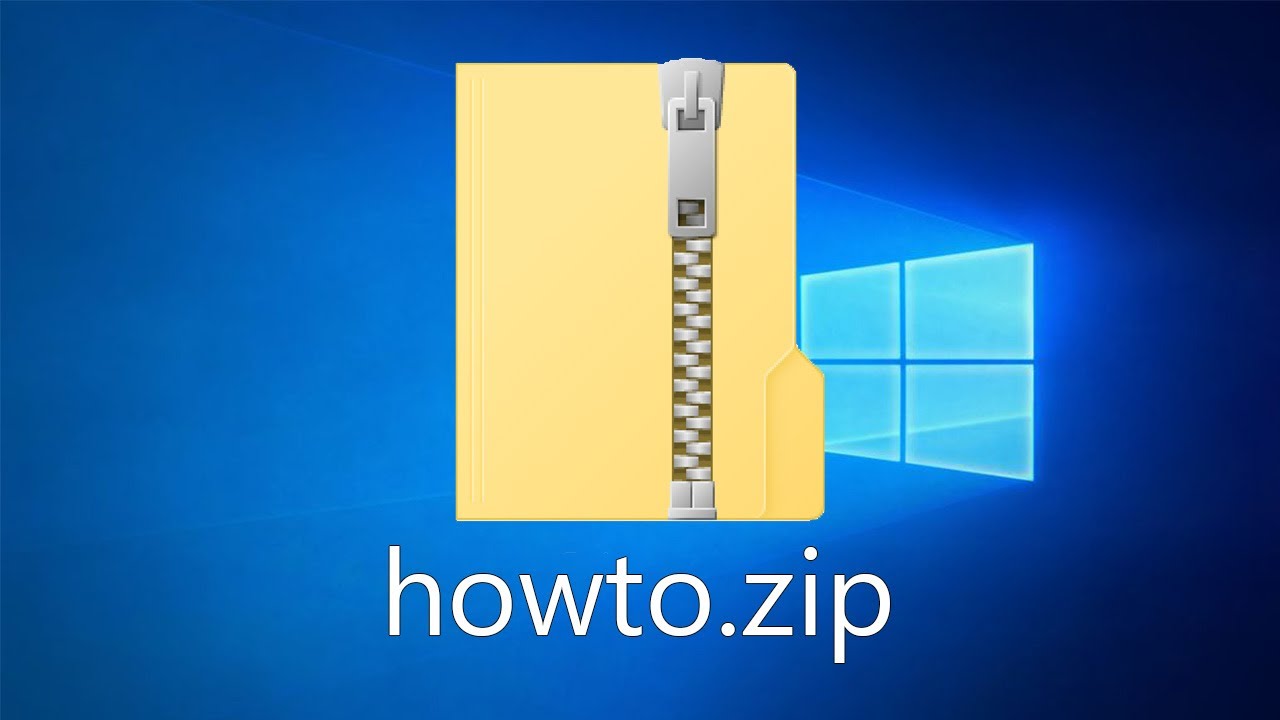
How to make a ZIP Files in Windows
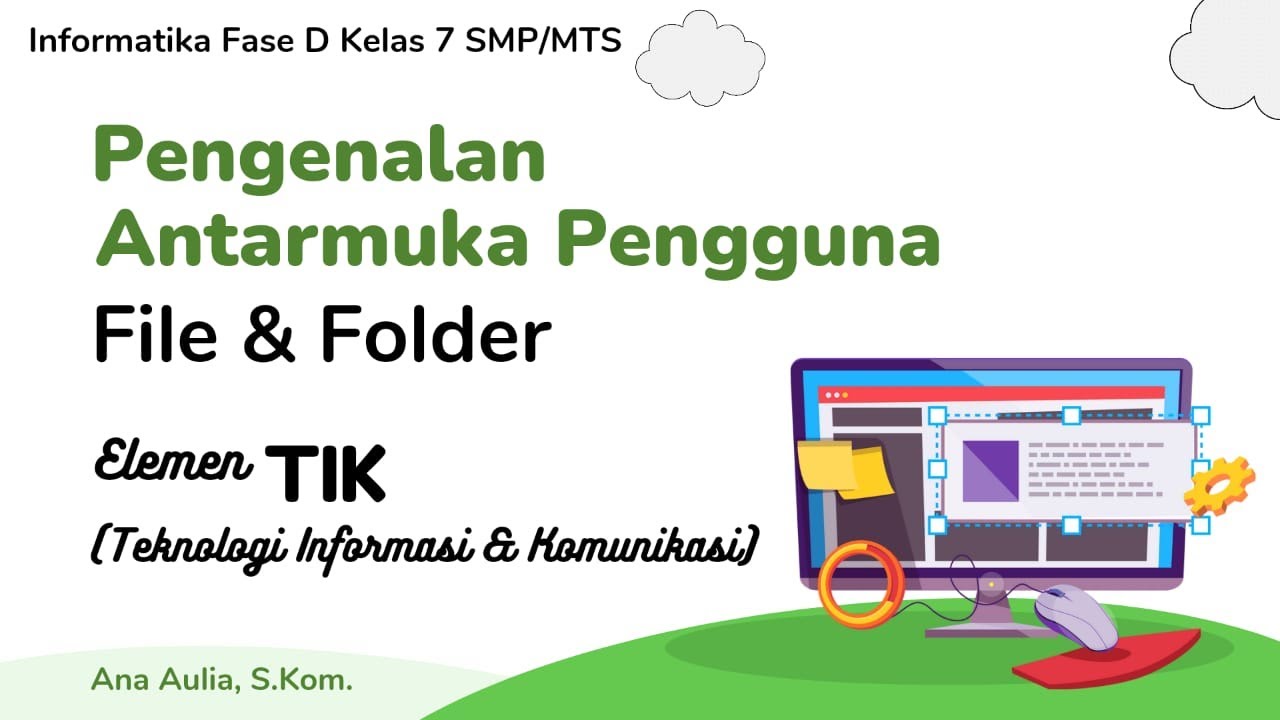
#1 Pengenalan Antarmuka Pengguna - File & Folder - Elemen TIK | Informatika Kelas 7 - Pelajar Hebat
5.0 / 5 (0 votes)