Python Pandas Tutorial 2: Dataframe Basics
Summary
TLDRIn this tutorial, you'll learn the basics of working with DataFrames in pandas, a powerful library for data analysis in Python. Key topics include creating DataFrames from CSV files or Python dictionaries, inspecting and manipulating data, performing basic statistical operations (like max, min, and mean), and selecting data conditionally. You'll also discover how to modify the index of your DataFrame and reset it. Whether you're new to pandas or looking to solidify your understanding, this tutorial provides a clear and hands-on introduction to pandas' core features, with examples and practical tips for analyzing tabular data.
Takeaways
- 😀 DataFrame is the core object in pandas for representing tabular data like rows and columns.
- 😀 You can create a DataFrame using a CSV file (read_csv) or by manually passing a Python dictionary.
- 😀 Jupyter Notebook is recommended for data visualization and working with pandas, but other IDEs like PyCharm or Notepad++ can also be used.
- 😀 You can use the df.shape attribute to check the number of rows and columns in your DataFrame.
- 😀 The df.head() function allows you to preview the first few rows of a DataFrame, while df.tail() shows the last few rows.
- 😀 DataFrame columns can be accessed directly using df.column_name or through bracket notation, like df['column_name'].
- 😀 To view summary statistics, you can use functions like min(), max(), mean(), and describe() on your DataFrame columns.
- 😀 You can filter rows based on conditions, similar to SQL queries, using logical expressions like df[df['column'] > value].
- 😀 DataFrame columns are of type pandas Series, which also support various statistical and arithmetic operations.
- 😀 You can change the index of a DataFrame with the set_index() method and use it for efficient lookups with loc.
- 😀 You can reset the DataFrame index using the reset_index() function and change the index to any column of your choice.
Q & A
What is the main object in the Pandas framework that is used for representing tabular data?
-The main object in Pandas for representing tabular data is the DataFrame. It is a data structure that organizes data into rows and columns, similar to an Excel sheet.
What is the purpose of the `pd.read_csv()` function in Pandas?
-The `pd.read_csv()` function is used to read a comma-separated values (CSV) file and convert it into a Pandas DataFrame, allowing easy manipulation and analysis of the data.
How can you create a DataFrame using a Python dictionary?
-You can create a DataFrame from a Python dictionary by using `pd.DataFrame()`. The dictionary keys represent column names, and the values are the data for each column.
What does the `df.shape` attribute return in Pandas?
-The `df.shape` attribute returns a tuple representing the dimensions of the DataFrame: the number of rows and columns. For example, `(6, 4)` means the DataFrame has 6 rows and 4 columns.
What is the difference between `df.head()` and `df.tail()`?
-The `df.head()` function returns the first 5 rows of the DataFrame by default, while `df.tail()` returns the last 5 rows. Both can be customized to show a specific number of rows by passing an argument like `df.head(2)`.
How can you access a specific column in a Pandas DataFrame?
-You can access a specific column by using the syntax `df['column_name']`, where `column_name` is the name of the column you want to access.
What is the purpose of the `df.describe()` method in Pandas?
-The `df.describe()` method provides a quick statistical summary of the numerical columns in a DataFrame, including count, mean, standard deviation, and percentiles.
How do you filter rows in a DataFrame based on a condition?
-You can filter rows using boolean indexing. For example, `df[df['temperature'] >= 32]` filters the rows where the temperature is greater than or equal to 32.
What happens when you use `df.set_index('column_name')` in Pandas?
-The `df.set_index('column_name')` method changes the DataFrame's index to the values from the specified column. This can make it easier to reference rows based on that column.
How can you reset the index of a DataFrame to the default integer-based index?
-You can reset the index to the default integer-based index by using `df.reset_index(inplace=True)`. This will remove any custom index and revert to a range starting from 0.
Outlines
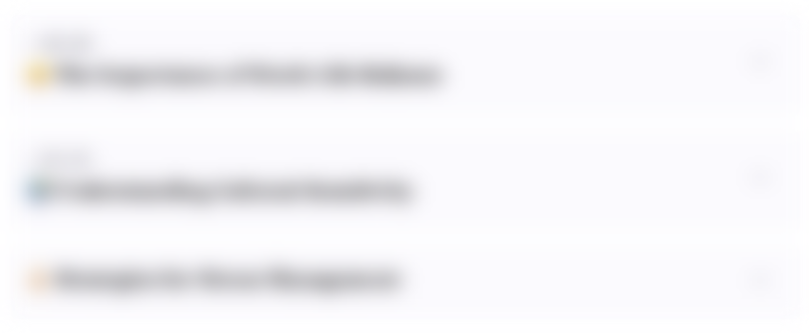
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
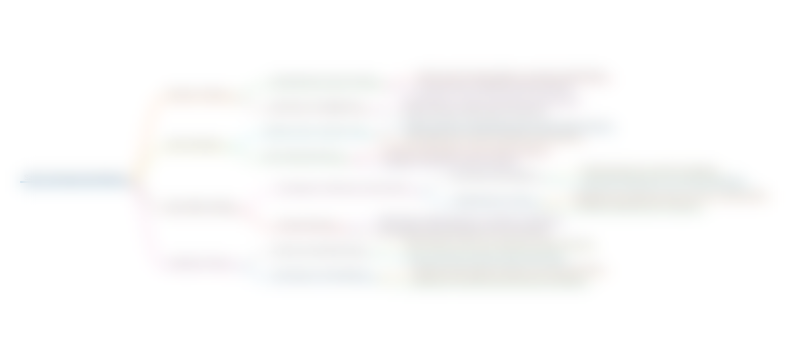
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
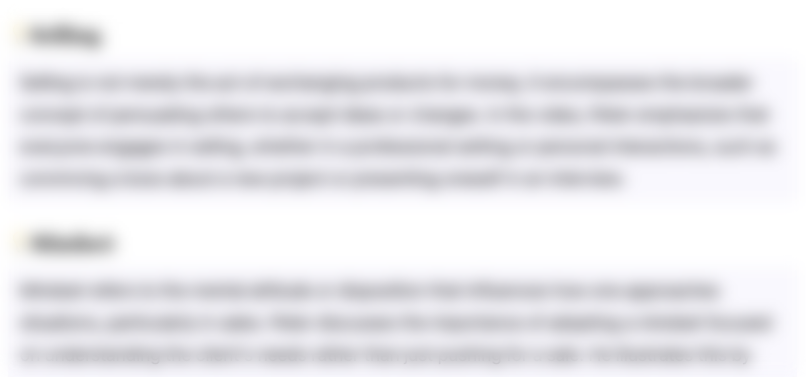
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
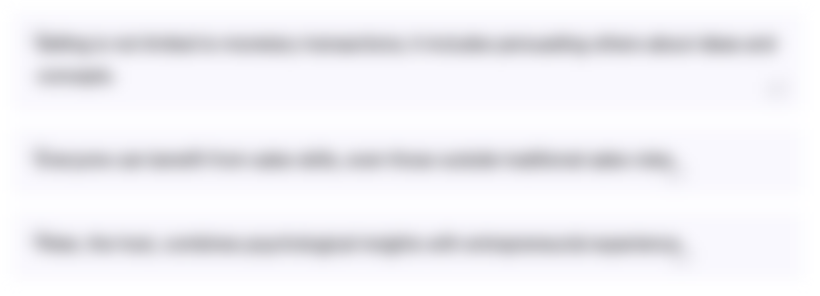
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
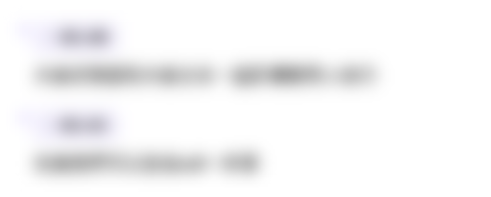
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频

Dataframes - Part 01
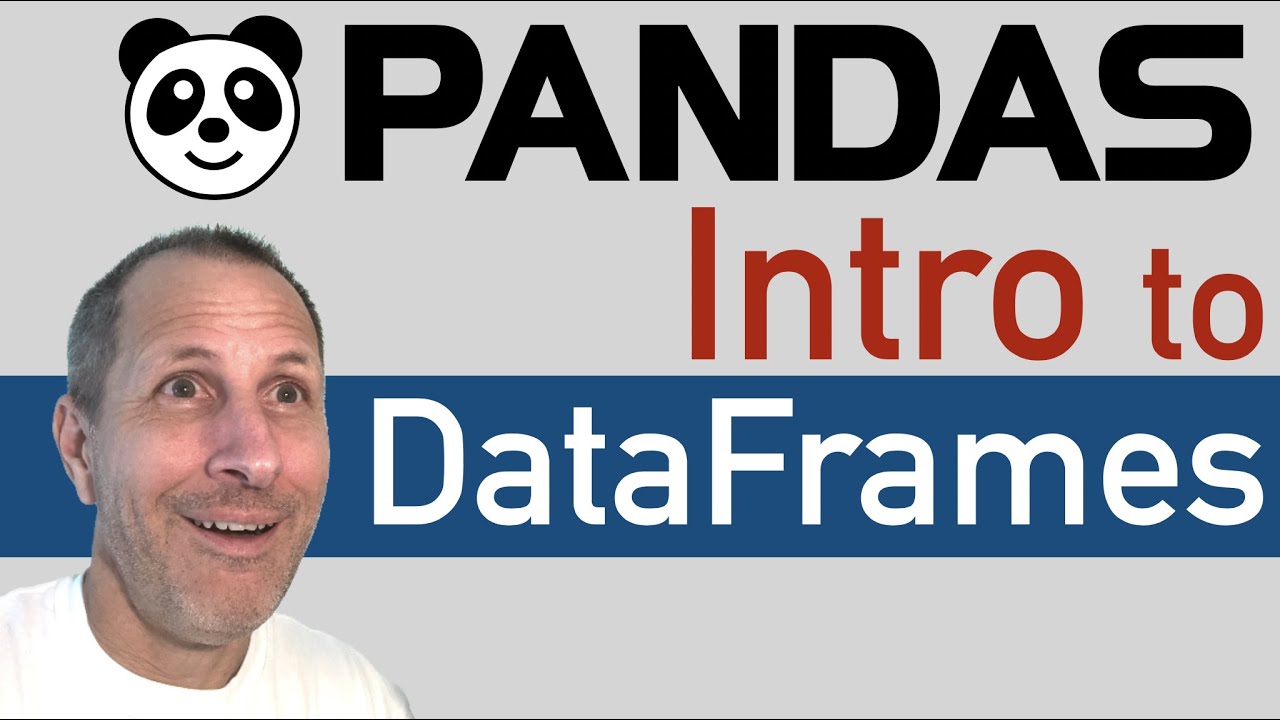
Python: Pandas Tutorial | Intro to DataFrames
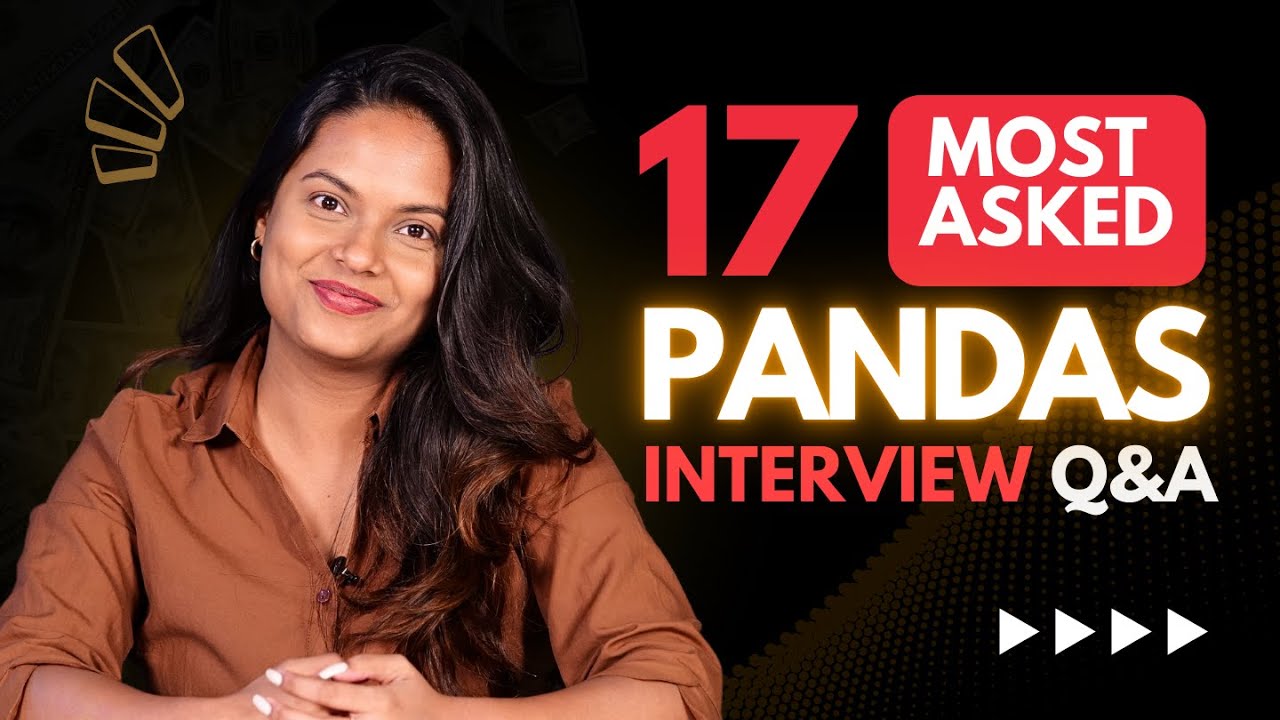
17 Most Asked Pandas Interview Questions & Answers | Python Pandas Interview Questions 2024
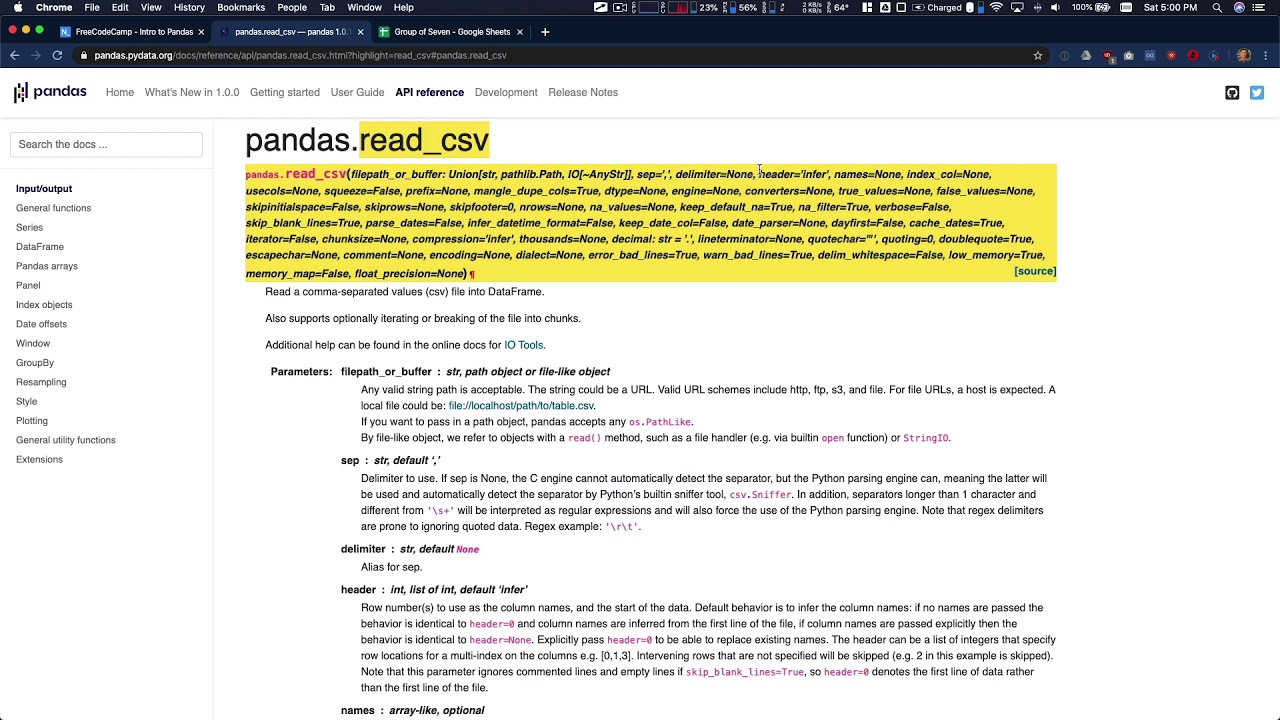
Pandas Creating Columns - Data Analysis with Python Course
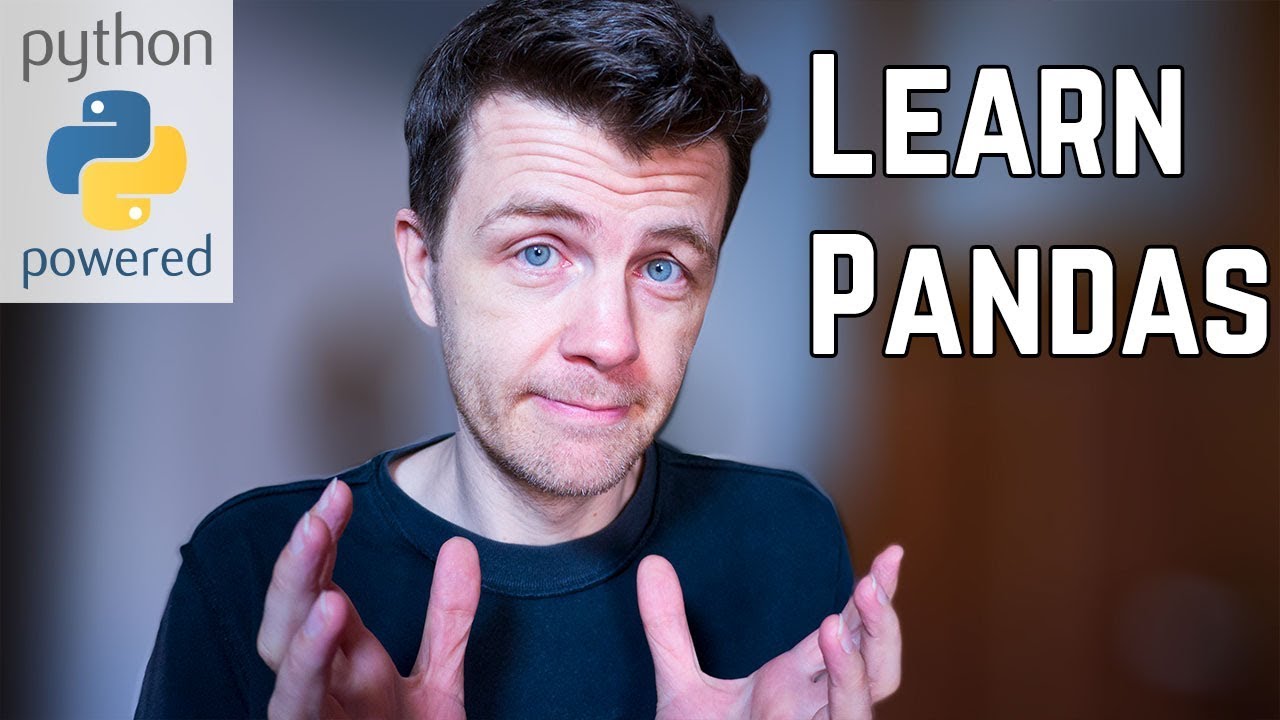
What is Pandas? Why and How to Use Pandas in Python
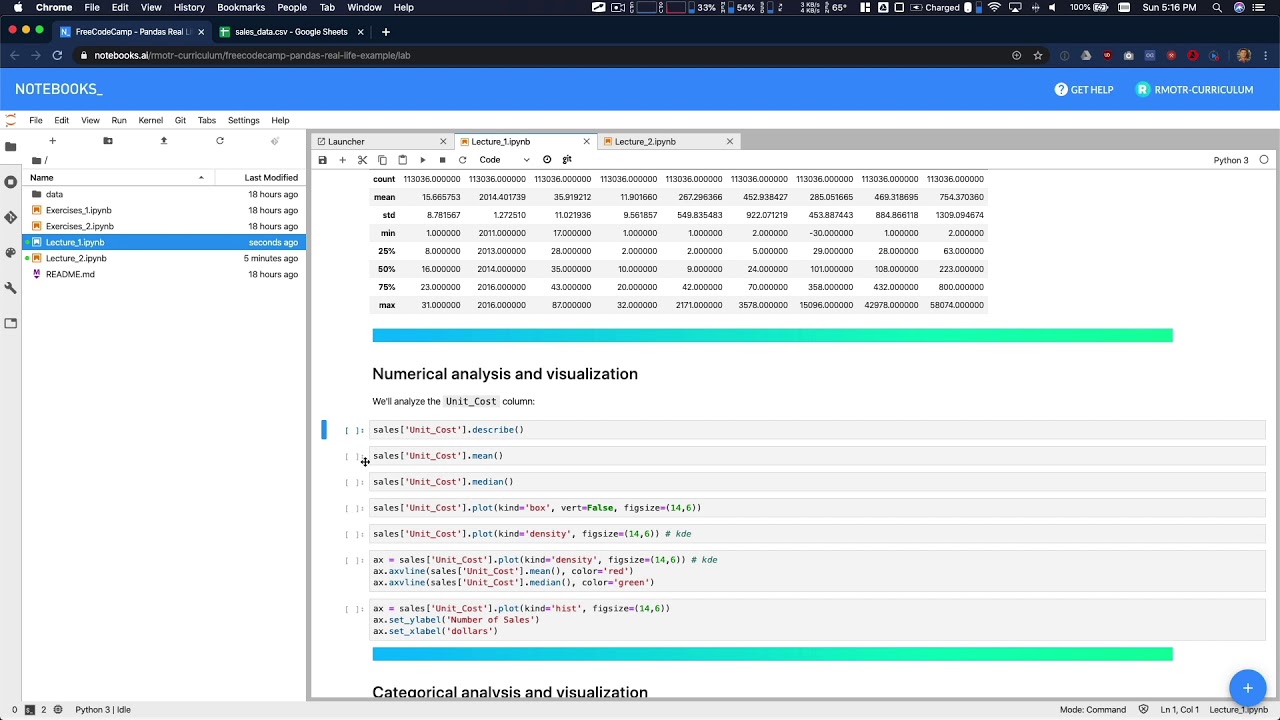
Data Analysis Example A - Data Analysis with Python
5.0 / 5 (0 votes)