Websockets in NestJs (Real-Time Chat App)
Summary
TLDRThis video tutorial provides a comprehensive guide to implementing WebSocket communication, detailing both backend and frontend aspects. It covers how to create a WebSocket gateway, handle messages with decorators, and broadcast messages to all connected clients. Viewers learn about detecting user connections and disconnections, as well as how clients can emit messages to the server. The tutorial emphasizes the bidirectional nature of WebSocket, demonstrating practical code examples to build a chat application that seamlessly updates user interfaces in real time. Overall, it offers valuable insights for developers looking to enhance their applications with WebSocket technology.
Takeaways
- 😀 WebSockets enable real-time communication between a client and server, allowing for efficient message exchange.
- 📡 The WebSocket server can handle various types of messages, including strings, numbers, and objects.
- 🔄 Broadcasting messages to all connected clients is straightforward using the server's `emit` method with specific event names.
- 👥 Detecting user connections and disconnections is essential for managing user sessions, accomplished through dedicated methods in the backend.
- 💬 The client can emit messages to the server, triggering responses that are sent back to all clients.
- 📲 When a user joins or leaves, events such as 'user joined' and 'user left' are emitted to keep all clients informed.
- 📈 Frontend code connects to the WebSocket server, allowing it to listen for incoming messages in real time.
- 🖱️ User actions, like button clicks, can trigger messages to be sent via WebSocket, enhancing interactivity.
- 🛠️ Utilizing decorators simplifies the process of handling different types of messages on the server.
- 📜 Effective logging during connection and disconnection events aids in tracking user activity and debugging.
Q & A
What is the purpose of using WebSockets in a chat application?
-WebSockets enable real-time, bi-directional communication between clients and servers, allowing instant message delivery without the need for constant polling.
How do you set up a WebSocket gateway in a NestJS application?
-To set up a WebSocket gateway in NestJS, you create a new class and decorate it with `@WebSocketGateway()`. This class will handle the WebSocket connections and events.
What is the role of the `@SubscribeMessage` decorator in NestJS?
-The `@SubscribeMessage` decorator is used to listen for specific events emitted by clients. It allows the server to define methods that handle these incoming messages.
How can messages be broadcasted to all connected clients?
-Messages can be broadcasted using the `emit` method on the WebSocket server instance, allowing all connected clients to receive the same message on a specified event.
What is the significance of the `@WebSocketServer()` decorator?
-The `@WebSocketServer()` decorator is used to inject the WebSocket server instance into the gateway class, enabling access to server methods like `emit` for broadcasting messages.
What methods are used to handle client connections and disconnections?
-The methods `handleConnection` and `handleDisconnect` can be implemented to log when a user connects or disconnects, as well as to notify other clients of these events.
How can clients send messages to the server?
-Clients can send messages to the server using the `emit` method, specifying the event name and the message payload, which the server then processes.
What is the importance of CORS in a WebSocket application?
-CORS (Cross-Origin Resource Sharing) is important in WebSocket applications to allow clients from different origins to connect to the WebSocket server, ensuring secure and accessible communication.
How can a client respond to specific events sent by the server?
-Clients can listen for events emitted by the server using `socket.on(eventName)`, which enables them to handle and display messages or updates in real-time.
What should developers consider when designing a WebSocket-based chat application?
-Developers should consider user authentication, connection management, message handling, error handling, and ensuring scalability for a smooth and secure chat experience.
Outlines
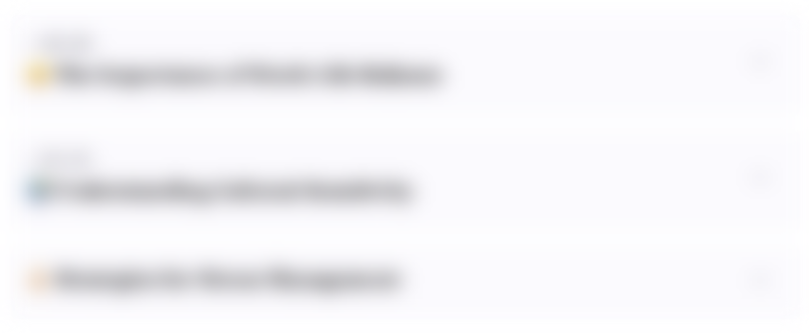
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
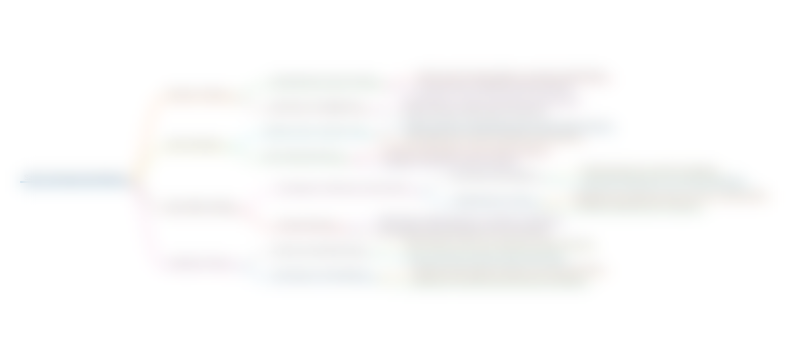
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
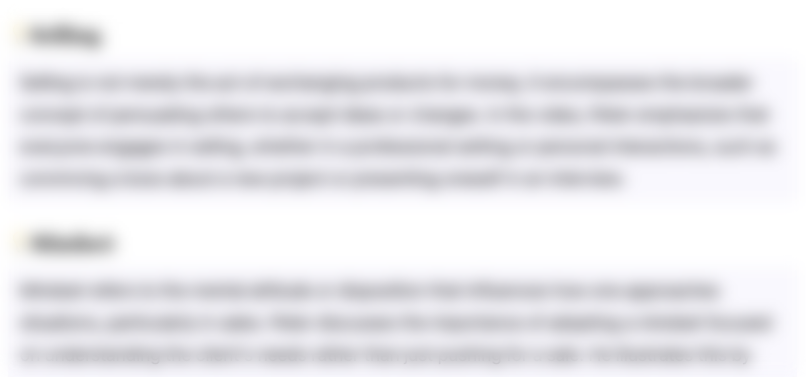
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
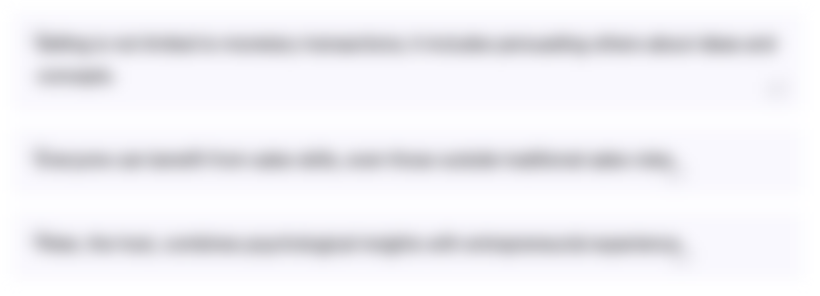
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
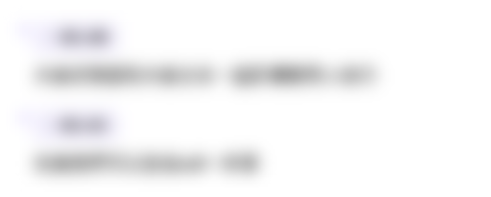
此内容仅限付费用户访问。 请升级后访问。
立即升级5.0 / 5 (0 votes)