C Programming Tutorial 9 - C Basics Part 1 - Variables, Expressions, Statements
Summary
TLDRIn this introductory tutorial on C programming, Caleb emphasizes the importance of understanding the basic structure of a C program, including spacing and formatting for clarity. He explains variables, their declaration and initialization, and the significance of syntax in writing code. The video illustrates how to perform mathematical operations using variables, highlighting the benefits of using variables over hard-coded values. Caleb concludes by encouraging viewers to practice these concepts and engage with questions, setting the stage for future lessons on printing output to the console.
Takeaways
- 😀 Understanding the general structure of a C program is essential for beginners.
- 😀 C is a space-insensitive language, allowing flexibility in spacing but emphasizing clarity through formatting.
- 😀 Proper indentation and spacing help improve the readability of code blocks.
- 😀 Variables are fundamental in programming, allowing for data storage and reuse throughout the code.
- 😀 A variable is declared with a type (e.g., int) and initialized with a value (e.g., x = 5).
- 😀 Syntax refers to the rules governing the structure of C code, including where to place symbols like semicolons.
- 😀 Using variables instead of hardcoding values simplifies code maintenance and improves flexibility.
- 😀 Expressions in C are evaluated to yield a single value, making calculations straightforward.
- 😀 Both variable declaration and initialization can be performed in one line for efficiency.
- 😀 Future lessons will cover how to output results to the console using the printf function.
Q & A
What is the main focus of this C programming tutorial?
-The tutorial aims to teach the basics of C programming, specifically how to write programs and understand their structure, starting with the 'Hello World' program.
Why is it important to understand spacing in C programming?
-While C is a space-insensitive language and spacing does not affect functionality, proper spacing and indentation improve code clarity and make it easier to read.
What does it mean that C is a 'space-insensitive language'?
-Being space-insensitive means that the placement of spaces and tabs in the code does not affect its execution; the code will function the same regardless of how it is spaced.
How do you declare a variable in C?
-You declare a variable by specifying its type followed by its name, such as 'int x;'. This tells the compiler that 'x' will be an integer variable.
What is the difference between variable declaration and initialization?
-Variable declaration introduces the variable to the program, while initialization assigns a value to that variable. For example, 'int x;' is a declaration, and 'x = 5;' is initialization.
What conventions should be followed when naming variables in C?
-A common convention is to start variable names with a lowercase letter and use camel case for multiple words (e.g., 'myVariable'). For single variables, lowercase is typically used (e.g., 'x').
What is an expression in programming?
-An expression is a combination of variables, constants, and operators that can be evaluated to yield a single value, such as 'x / 2' which evaluates to a number based on the value of 'x'.
Why is it beneficial to use variables instead of hard-coded values?
-Using variables allows for code flexibility, as variables can store values that may change or be input by users, avoiding the need to repeatedly change hard-coded values throughout the code.
What are the key terms introduced in the tutorial?
-The key terms include declaration, initialization, expression, and syntax, which are essential for understanding how to write and structure C programs.
What will be covered in the next video of the tutorial series?
-The next video will focus on how to output data to the console using the 'printf' function.
Outlines
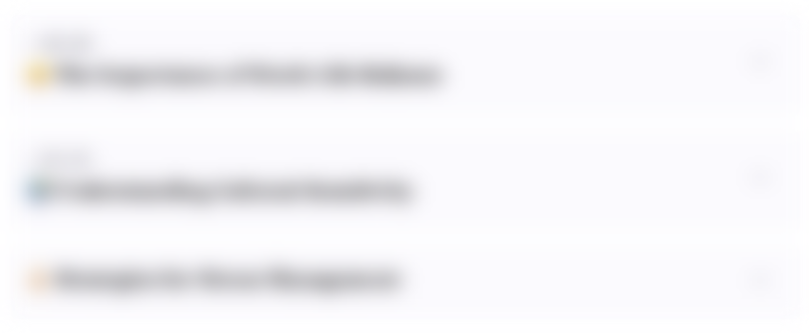
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
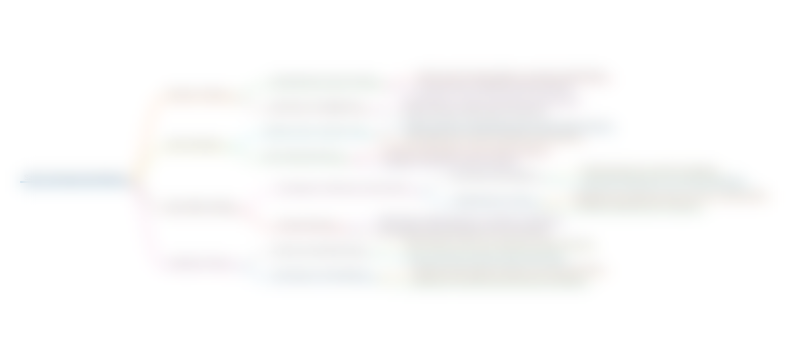
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
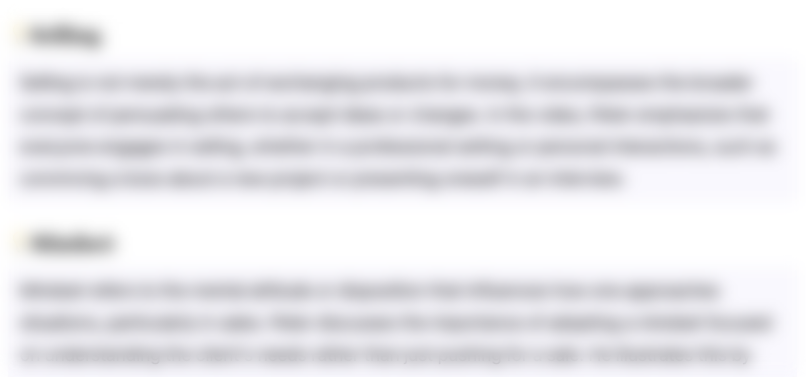
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
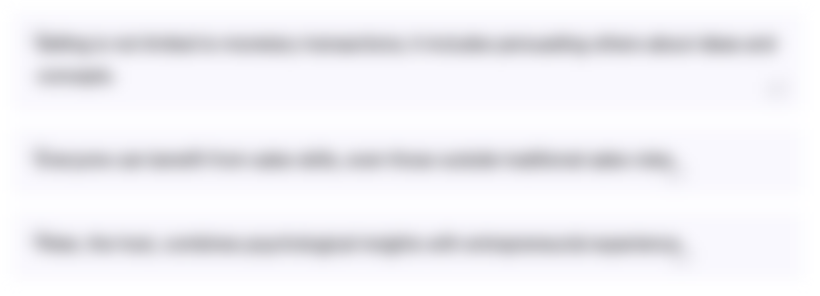
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
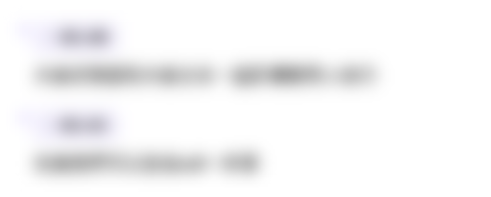
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
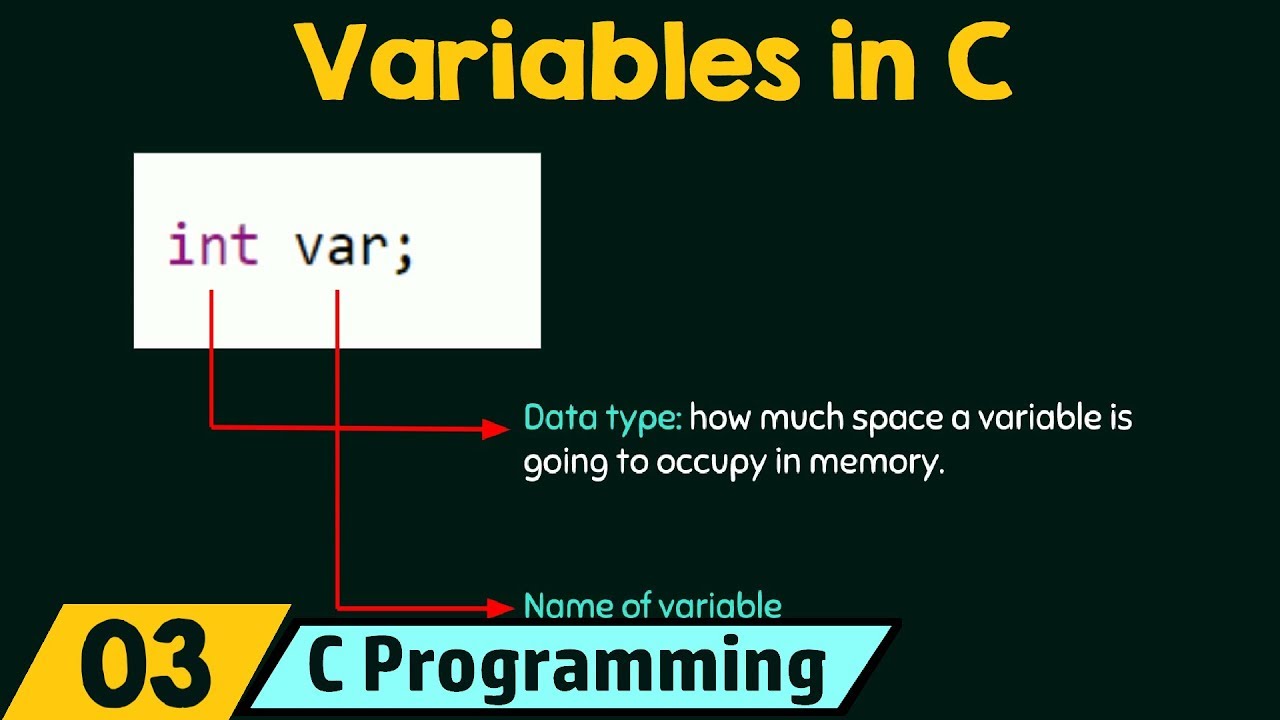
Introduction to Variables
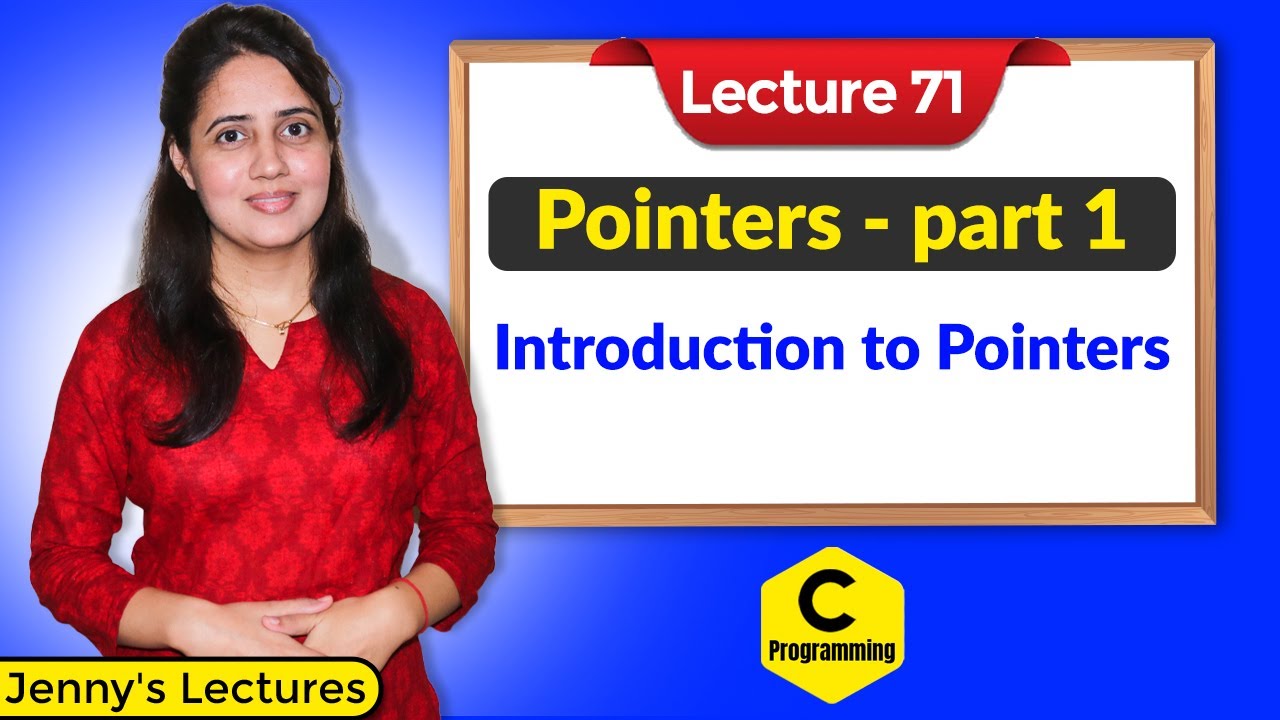
C_71 Pointers in C - part 1| Introduction to pointers in C | C Programming Tutorials
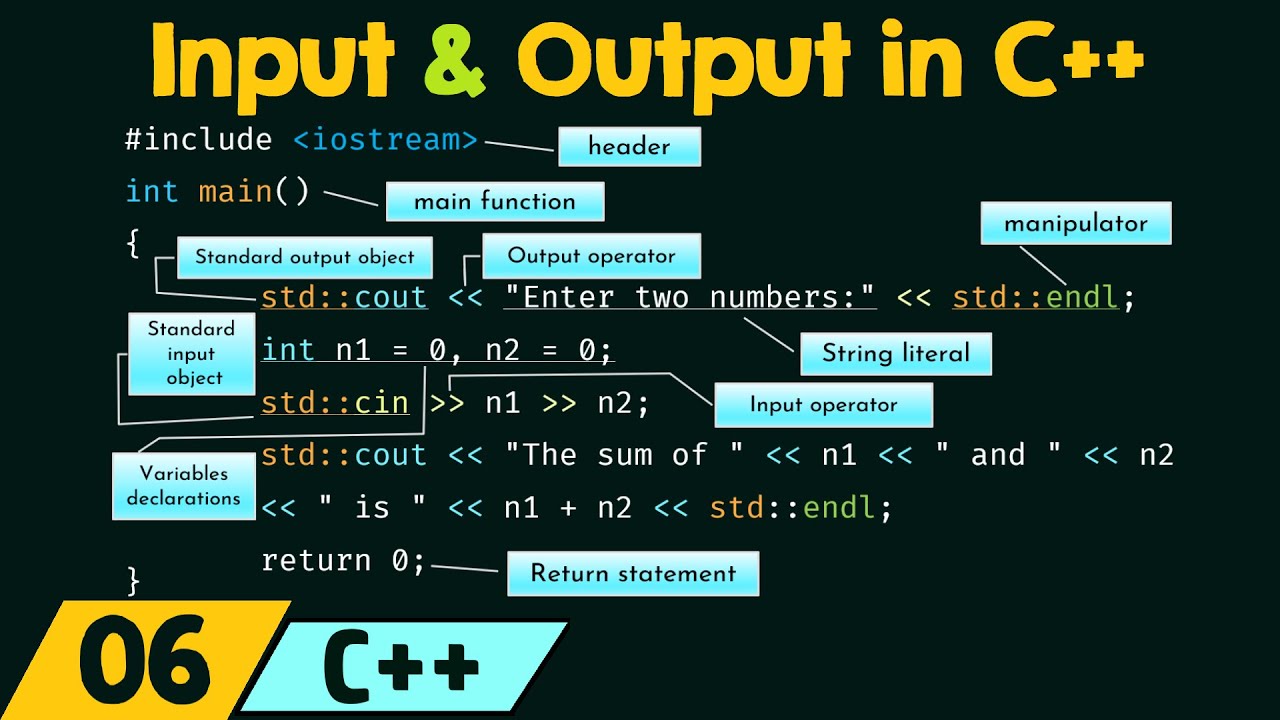
Input and Output in C++
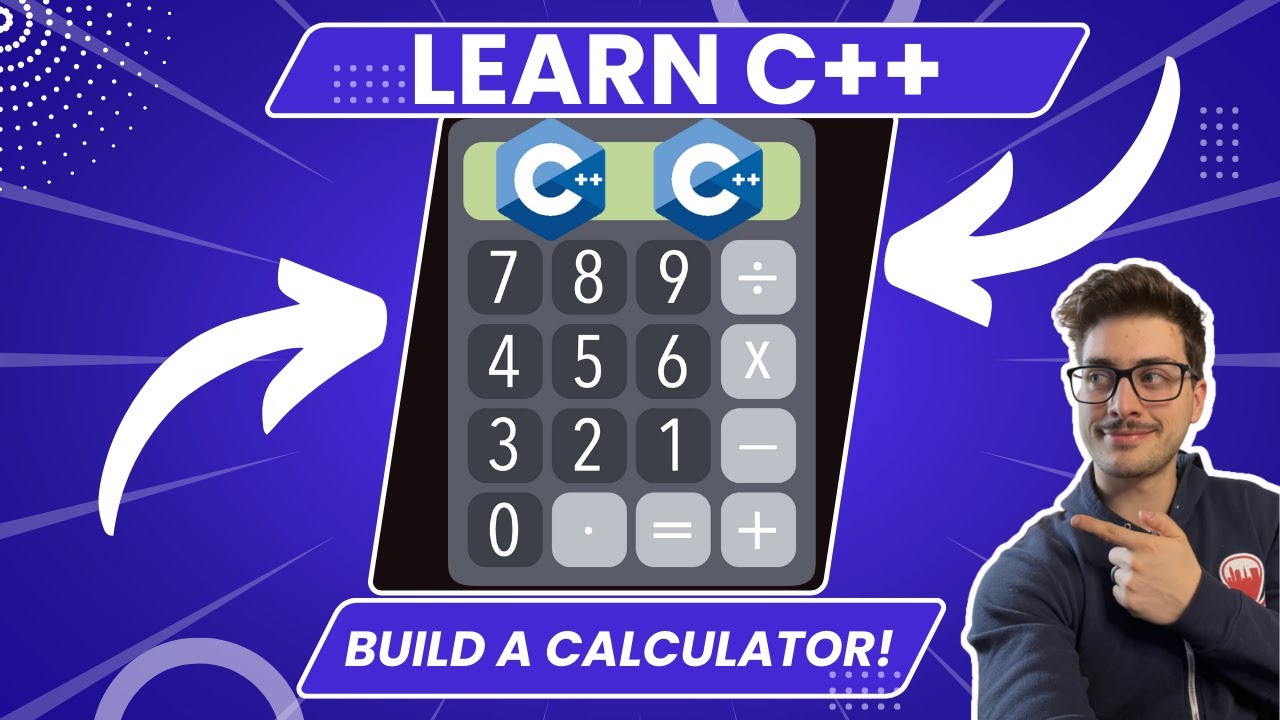
How to Program A Calculator in C++! (Great C++ Microsoft Visual Studio Beginner Project)
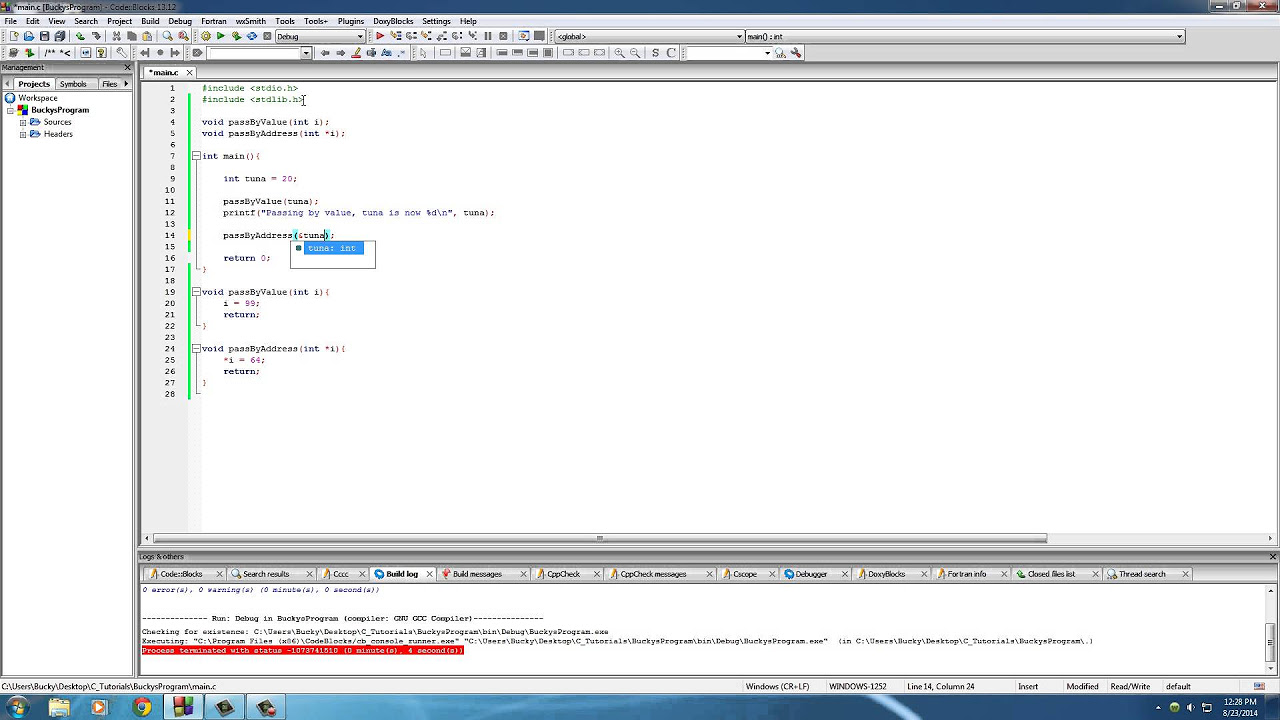
C Programming Tutorial - 58 - Pass by Reference vs Pass by Value
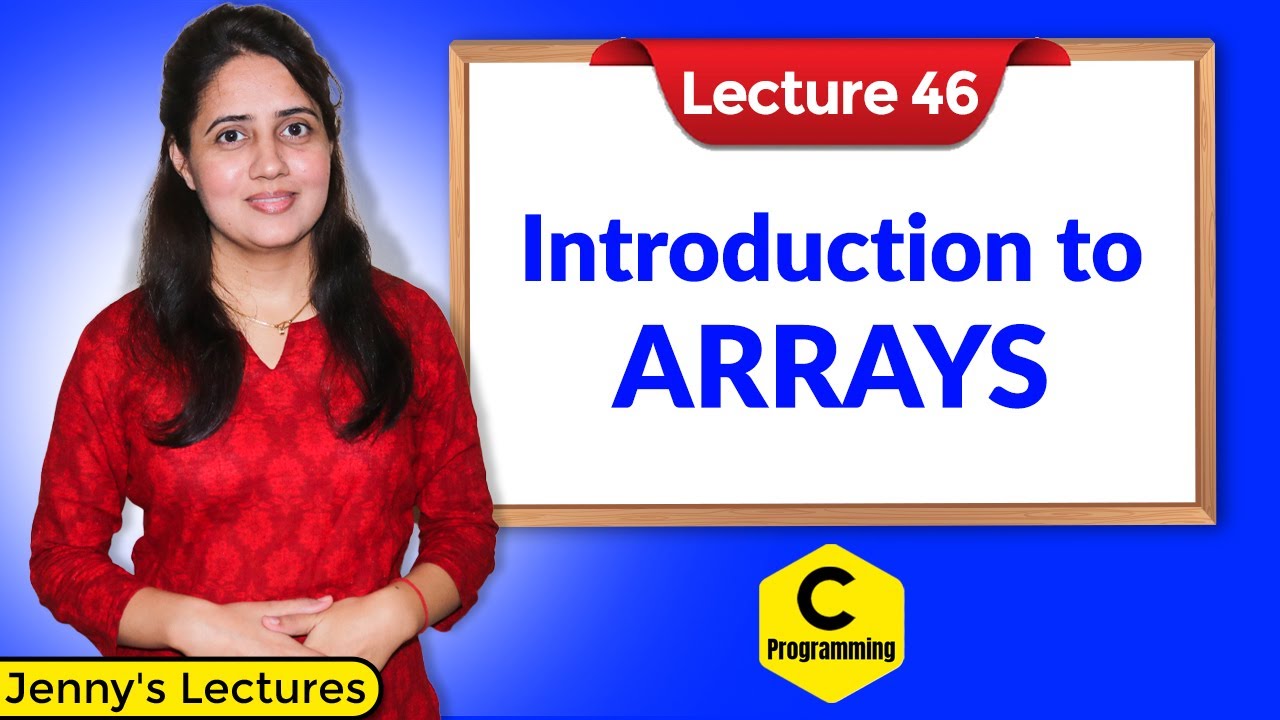
C_46 Arrays in C - part 1 | Introduction to Arrays
5.0 / 5 (0 votes)