Introduction to Redux | Lecture 257 | React.JS 🔥
Summary
TLDRThe video script provides an insightful overview of Redux, a popular third-party library used for managing global state in web applications. Redux can be used with any framework or even vanilla JavaScript, but it is closely associated with React. The script explains Redux's core concept of a single, globally accessible store that is updated via actions, which is similar to the useReducer hook in React. The Redux cycle is described as starting with an action creator in a component, dispatching the action to the store, and then having the appropriate reducer update the state. This leads to a re-render of the UI. The script also touches on Redux's history, its current standing in the development community, and when it is appropriate to use Redux. It concludes by emphasizing Redux's goal of separating state update logic from the rest of the application, making it easier to manage complex state in large applications.
Takeaways
- 📚 Redux is a third-party library used to manage global state in web applications, and it's not limited to React but can be used with any framework or even Vanilla JavaScript.
- 🔄 Redux operates on the principle of a single, globally accessible store that holds the application's state, which is updated through actions, similar to the useReducer hook in React.
- 🤝 Redux is often used in conjunction with React through the react-redux library, which facilitates the connection between the two.
- 📈 The Redux store can contain one or multiple reducers, each responsible for a specific feature or data domain within the application, ensuring a clear separation of concerns.
- ⚙️ Action creators in Redux are functions that automate the creation of action objects, centralizing actions and reducing the likelihood of errors.
- 🔧 Redux is historically significant and was once a staple in React applications for state management, but the landscape has evolved with the introduction of alternative tools.
- 🚫 Redux is not necessary for every application; it's recommended for use when managing a significant amount of global UI state that requires frequent updates.
- 📈 Redux Toolkit is a modern approach to writing Redux applications, offering a simpler and more streamlined way to work with Redux compared to classic Redux.
- 📱 The Context API is sometimes considered a replacement for Redux, but the two serve different purposes and the choice between them depends on the specific needs of the application.
- 🛠️ Redux is particularly useful for managing non-remote state within an application, while tools like React Query or SWR are better suited for managing remote state.
- 🏛️ The Redux cycle involves dispatching actions from components to the store, where reducers calculate the new state, leading to a re-render of the UI, encapsulating the state update logic separately from the application.
- 🏦 Redux can be analogized to a bank system where action creators provide instructions (actions), the dispatcher (store) instructs the vault (state) to update, and the process is managed without direct access to the vault.
Q & A
What is Redux and how does it relate to managing global state in a web application?
-Redux is a third-party library used to manage global state in a web application. It allows for the storage of all global state within a single, globally accessible store, which can then be updated using actions. Redux is not framework-specific and can be used with any framework or even Vanilla JavaScript, but it is commonly associated with React.
How is Redux connected with React and what role does react-redux library play in this?
-Redux has been tightly linked to React, and they can be easily connected using the react-redux library. This library facilitates the integration of Redux with React applications, allowing developers to manage the global state in a structured and predictable manner.
What is the significance of actions in Redux and how are they used to update the state?
-In Redux, actions are used to represent information about how the state should be updated. They are dispatched to the store, which then triggers the appropriate reducer to calculate the next state based on the action type and payload. This process is central to updating the global state in a Redux application.
How is the useReducer hook similar to Redux in terms of state management?
-The useReducer hook in React follows a similar pattern to Redux for state management. It involves creating actions and dispatching them to a reducer function, which calculates the next state. The main difference is that useReducer is used for managing local state within a component, whereas Redux handles global state across the entire application.
Why is it recommended to use Redux only when managing a significant amount of global UI state?
-Redux is recommended for managing a lot of global UI state that needs frequent updates because it provides a centralized store for all global state, making it easier to manage and update. However, for applications with less global state or more remote state, there are often better alternatives available, such as React Query, SWR, or Redux Toolkit Query.
What are the two versions of Redux and how do they differ?
-There are two versions of Redux: classic Redux and the more modern Redux with Redux Toolkit. Classic Redux is the original implementation, while Redux Toolkit is a newer approach that simplifies common Redux patterns and reduces boilerplate code. Both versions are compatible with each other.
Why is it important to learn Redux even if it's not used in every React application today?
-Redux is important to learn for several reasons: it has a reputation for being complex, making it a frequently requested topic; working on a team may involve encountering older code bases that use Redux; and some applications genuinely require a global state management solution like Redux.
How does the Redux store function as a centralized container for the application's global state?
-The Redux store serves as a single source of truth for all global state in an application. It holds the current state tree and allows access to state updates through actions. The store can also contain multiple reducers, each responsible for a different slice of the state, ensuring that the state management remains organized and modular.
What is the role of action creators in Redux and how do they simplify the process of creating actions?
-Action creators in Redux are functions that automatically generate action objects, simplifying the process of creating actions. They centralize action definitions, reducing the likelihood of errors such as typos in action type strings. This approach is optional but is a common practice in building real-world Redux applications.
How does the dispatching of actions in Redux relate to the re-rendering of React components?
-When an action is dispatched to the Redux store, it triggers the reducers to calculate the new state. Once the state is updated, any React components that subscribe to data from the store will re-render to reflect the new state. This ensures that the UI remains in sync with the latest state data.
What is the purpose of separating the state update logic from the rest of the application in Redux?
-Separating the state update logic in Redux allows for a clear distinction between the business logic that manages state transitions and the presentational components that display the UI. This separation makes the application easier to maintain, debug, and reason about, as the state management becomes more predictable and centralized.
Can you provide an analogy to explain the flow of updating state with Redux?
-The process of updating state with Redux can be compared to a bank transaction. The developer acts as the action creator, providing instructions (the action) to the dispatcher, who is like the bank teller. The dispatcher then instructs the store (the bank's vault) to update the state. This analogy emphasizes the indirect nature of state updates in Redux, which must go through a series of defined steps.
Outlines
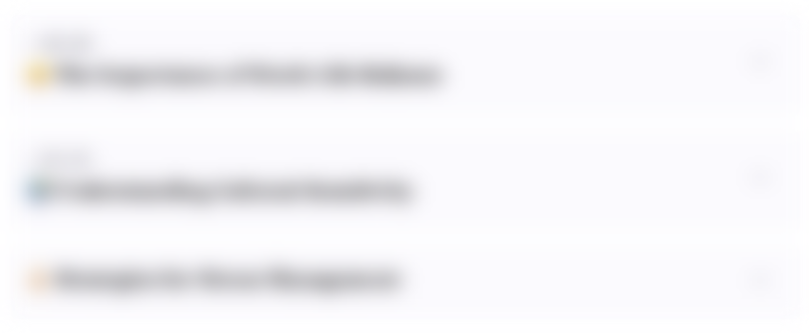
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
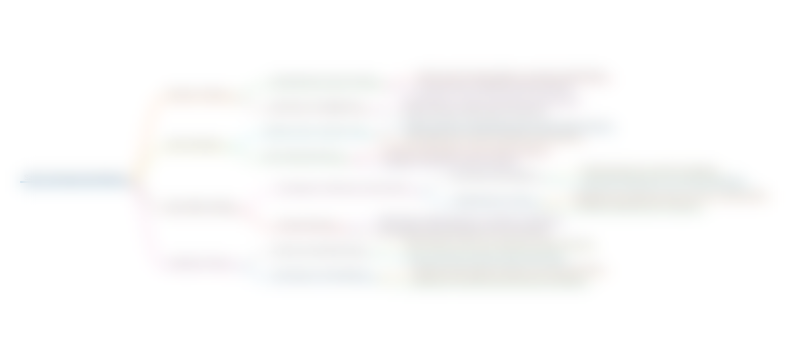
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
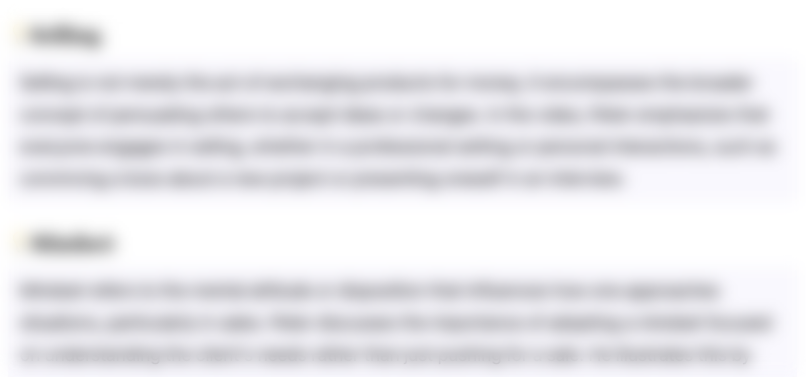
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
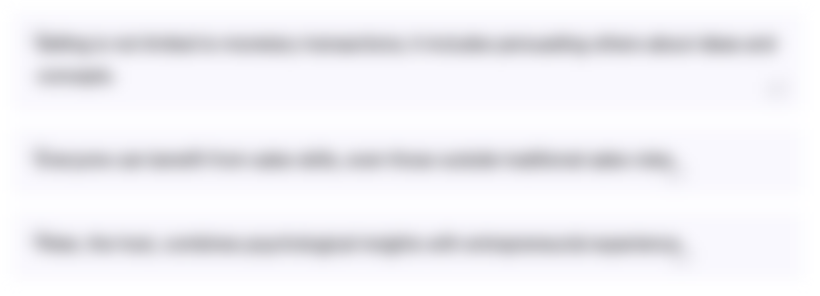
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
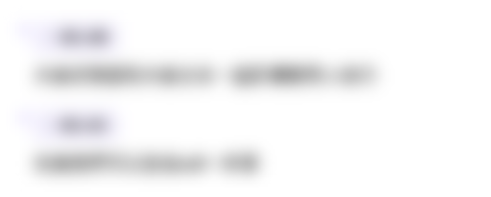
此内容仅限付费用户访问。 请升级后访问。
立即升级5.0 / 5 (0 votes)