#09 Styling View Template | Angular Components & Directives| A Complete Angular Course
Summary
TLDRThis lecture explains how to style the HTML of a view template in Angular. The speaker demonstrates styling elements using the 'Styles' property by applying CSS directly in the component's metadata. Additionally, they cover styling through external CSS files using the 'styleUrls' property for better maintainability. The differences between these methods are discussed, highlighting the drawbacks of inline styles and emphasizing the importance of component-specific CSS. Finally, the lecture clarifies that styles applied to a parent component do not affect child components, unlike in React.
Takeaways
- 💻 The view template of a component is the HTML structure associated with it, and it can be styled in multiple ways.
- 🔗 The 'Styles' property in the metadata object allows for direct styling of the HTML elements within the view template.
- 🚫 CSS styles like `text-decoration: none` can be applied to remove the underline from anchor elements and add margin between them.
- 🖱️ Button elements can also be styled with CSS directly within the Styles array, like adding padding to the button.
- 📏 Classes and IDs can be used to style specific elements within the component, such as a `div` element using its class name.
- ⚠️ A major downside of using the 'Styles' array is the mixing of TypeScript and CSS, making the code harder to maintain.
- 🔍 Errors in the 'Styles' array won’t be caught during compile time, but only during runtime, posing debugging challenges.
- 📝 The 'styleUrls' property is a better approach for managing styles, as it allows linking to external CSS files, improving maintainability.
- 🌐 Styles defined in the 'styleUrls' will only apply to the specific component they are associated with and not affect child components.
- 💡 In Angular, unlike React, styles applied to a parent component do not automatically propagate to child components, ensuring scoped styling.
Q & A
What is a view template in Angular?
-A view template in Angular refers to the HTML file that defines the structure and layout of a component's user interface. For example, the 'header.component.html' file is the view template for the 'header' component.
How can you style HTML elements in a view template using the 'Styles' property?
-You can use the 'Styles' property within the component's metadata object to directly style HTML elements. The 'Styles' property accepts an array of strings, where each string contains the CSS styles for a specific HTML element. For example, to remove the underline from anchor tags, you can define CSS like 'text-decoration: none;' within the array.
What are the limitations of using the 'Styles' property in Angular?
-The main limitations are: 1) Mixing CSS with TypeScript code, which is not a good practice and makes code less maintainable. 2) CSS is treated as a string, so syntax errors are only detected at runtime, not compile time. 3) If the CSS grows, the array becomes large and unmanageable.
How can you style elements using the 'styleUrls' property in Angular?
-Instead of using the 'Styles' property, you can use the 'styleUrls' property to reference external CSS files. This is done by specifying the path to a CSS file within an array. The styles defined in this external file will be applied to the component's view template.
What are the benefits of using 'styleUrls' over 'Styles'?
-The main benefits are: 1) Keeping the CSS separate from TypeScript, which improves maintainability. 2) Syntax errors in CSS can be caught during compile time. 3) The CSS can be written in external files, which makes it easier to manage larger amounts of styling code.
How does Angular apply styles using 'styleUrls'?
-When using the 'styleUrls' property, the styles in the referenced CSS file are scoped to the component's view template. This means the styles will only affect the HTML within the component and will not affect other components.
Can styles from a parent component affect a child component in Angular?
-No, in Angular, styles applied in a parent component (such as in its CSS file) do not automatically propagate to child components. Each component's styles are scoped to that component unless explicitly shared.
How would you style an HTML element by its class name in Angular?
-You can style an HTML element by its class name in the 'Styles' array by using a dot (.) followed by the class name, and then defining the styles inside curly braces. For example, '.ecat-header { width: 100%; height: 70px; }' styles the div with the 'ecat-header' class.
What happens if you miss a colon or semicolon while using the 'Styles' property?
-If you miss a colon or semicolon in the 'Styles' property, no error will be shown at compile time because the CSS is treated as a string. The error will only surface at runtime when the styles fail to apply correctly.
Can multiple CSS files be used in the 'styleUrls' property?
-Yes, multiple CSS files can be used in the 'styleUrls' property by adding their file paths as strings in the array, separated by commas. Each specified file's styles will be applied to the component's view template.
Outlines
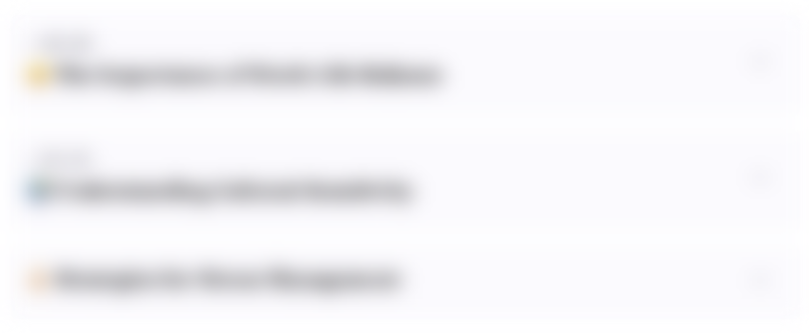
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
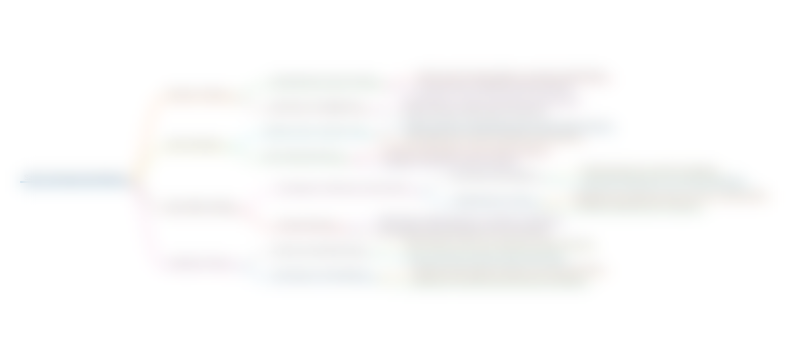
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
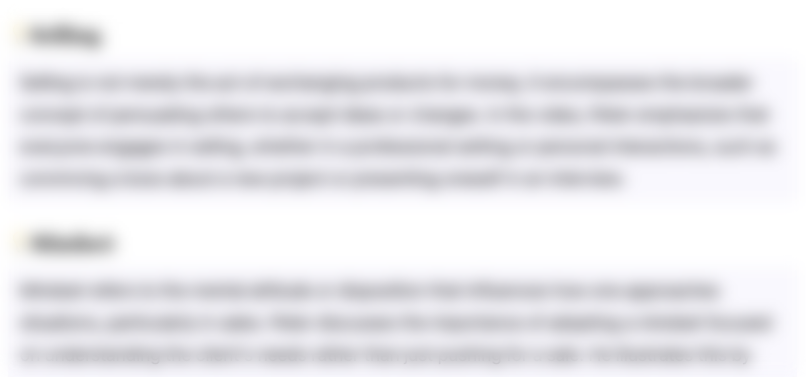
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
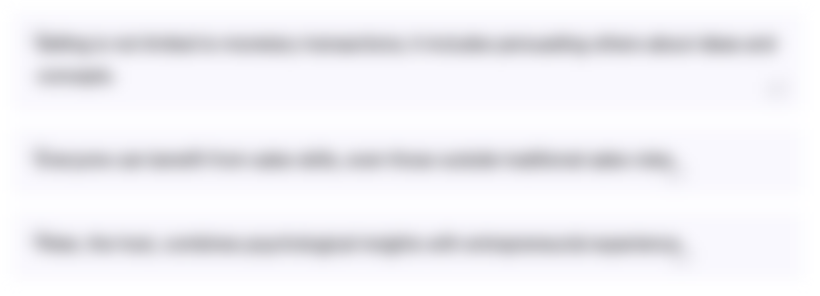
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
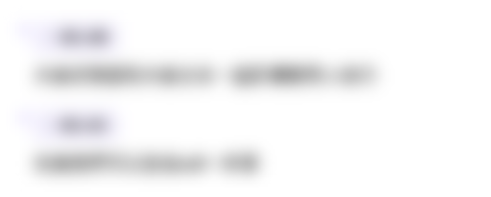
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频

Sintassi del Primo Programma - React Tutorial Italiano 04
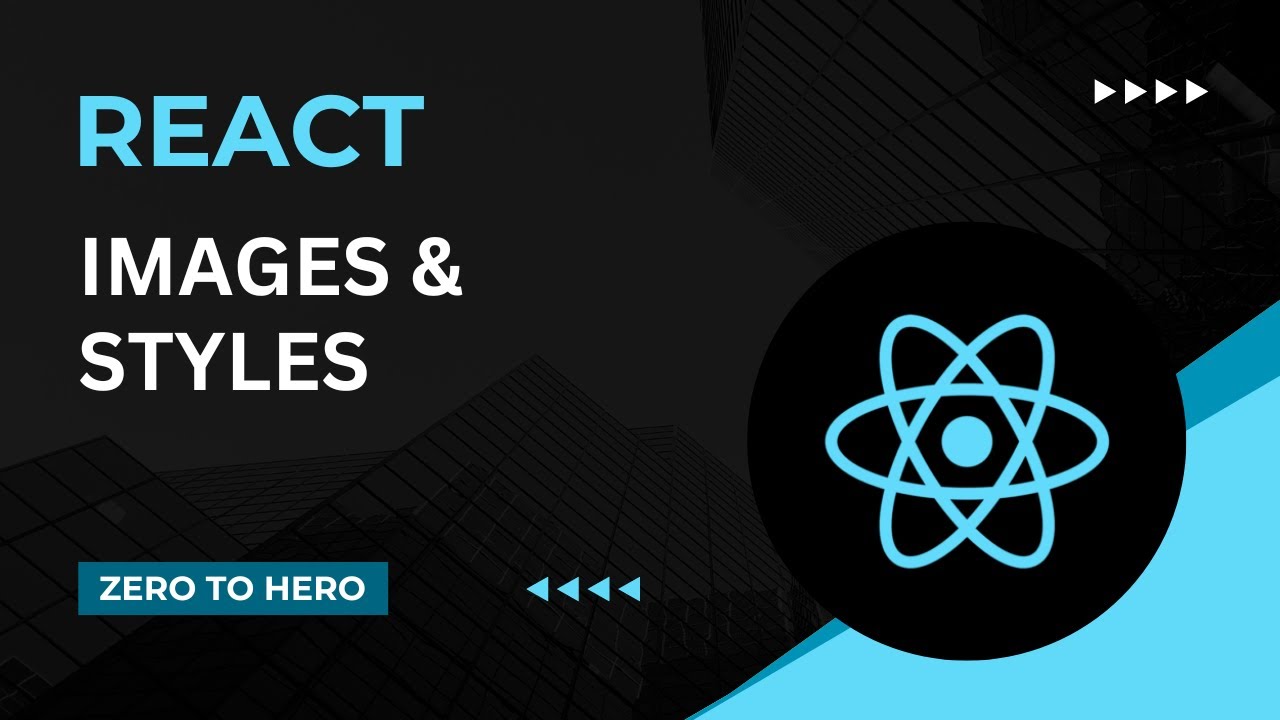
Images and Styles | Mastering React: An In-Depth Zero to Hero Video Series
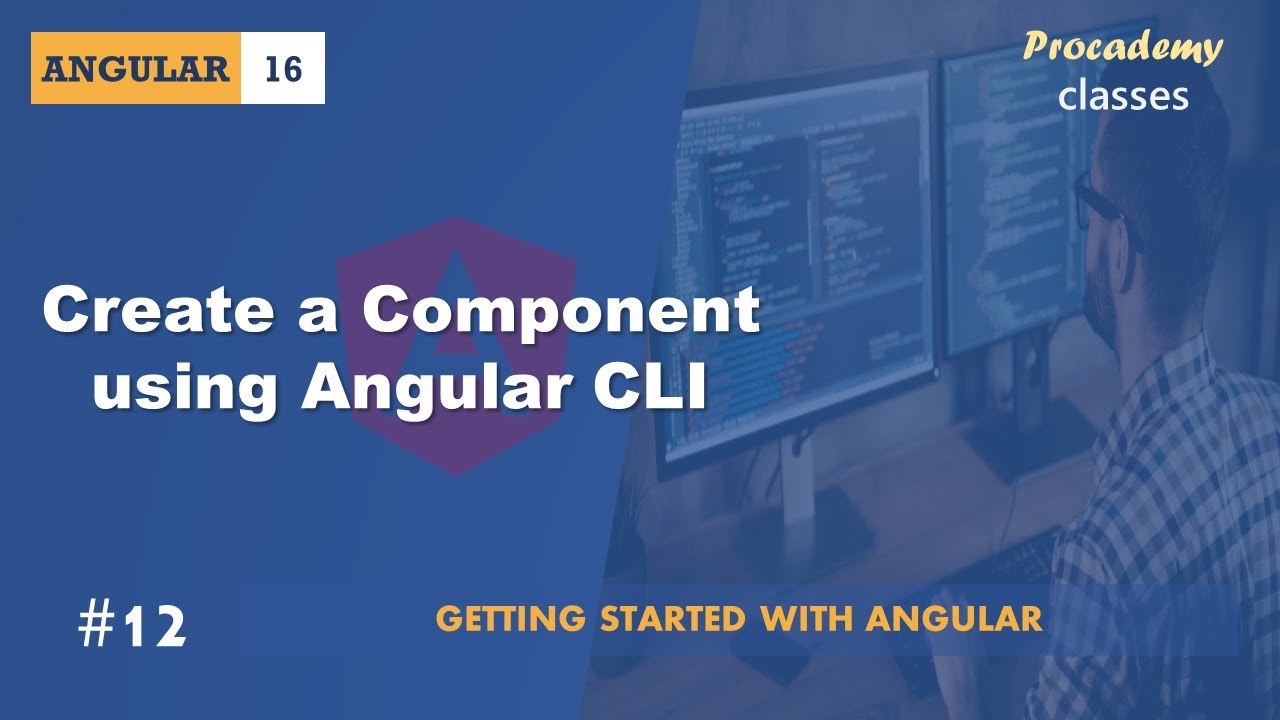
#12 Create Component using Angular CLI | Angular Components & Directives | A Complete Angular Course
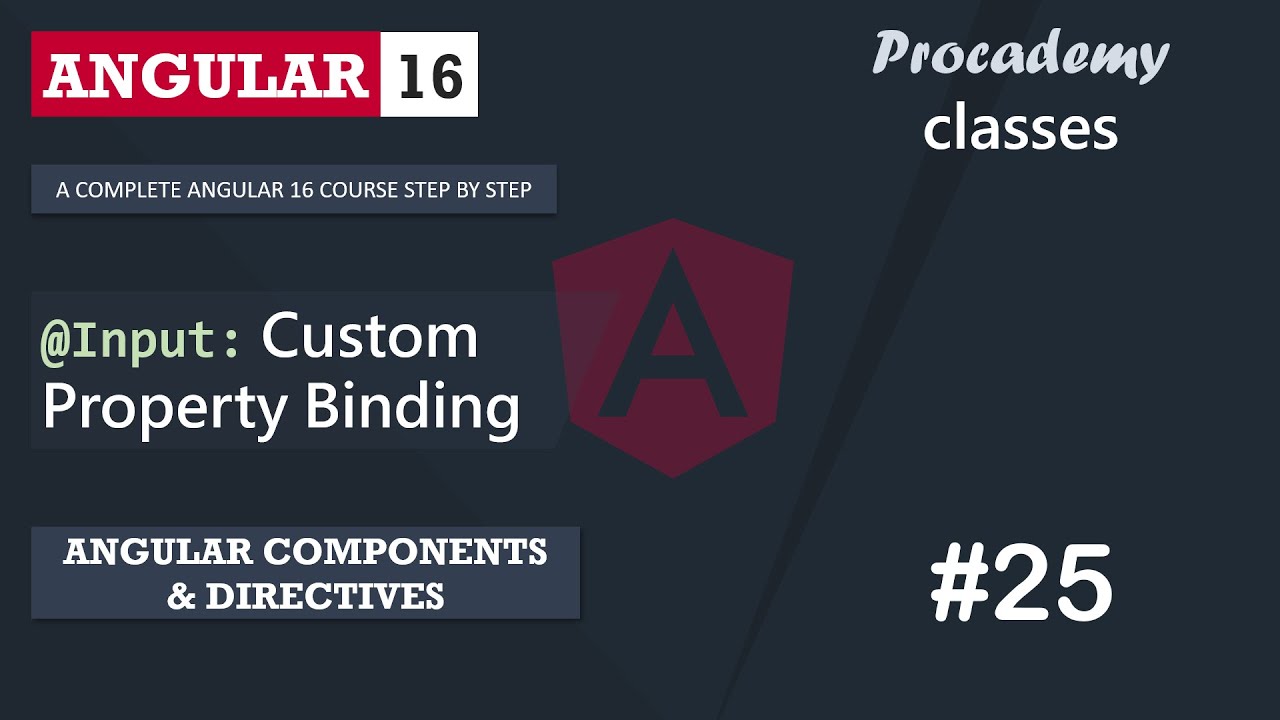
#25 @Input: Custom Property Binding | Angular Components & Directives | A Complete Angular Course
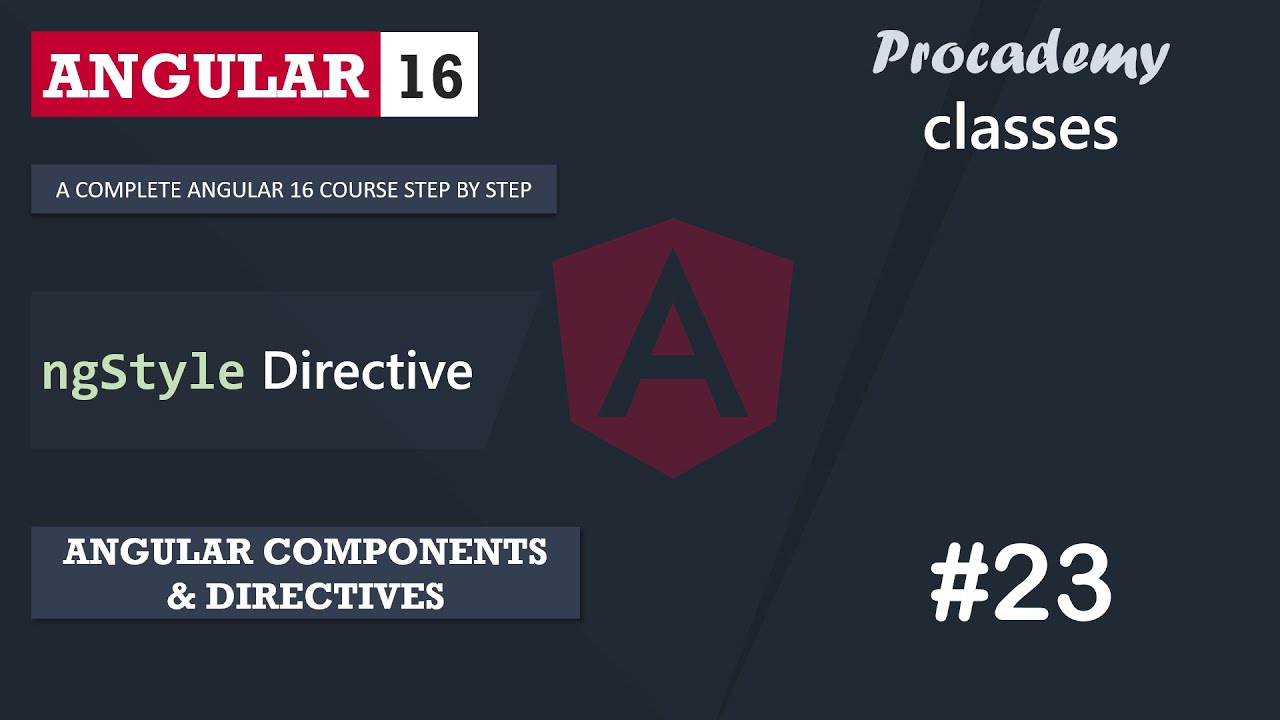
#23 ngStyle Directive | Angular Components & Directives | A Complete Angular Course

Learn CSS in 20 Minutes
5.0 / 5 (0 votes)