#16 Transforming JSON data into HTML | Fundamentals of NODE JS | A Complete NODE JS Course
Summary
TLDRIn this lecture, the process of converting JSON data into a JavaScript object is demonstrated using Node.js. The instructor reads data from a JSON file, converts it into an array of JavaScript objects, and logs the data. They then explain how to display this data on a webpage by creating an HTML file and looping over the array to dynamically insert product details. The lesson concludes by addressing issues with rendering and properly setting content types to ensure the web page correctly displays the product information.
Takeaways
- 📥 The lecture demonstrates how to read JSON data and convert it into a JavaScript object using the `JSON.parse` method.
- 📂 The app reads from a `products.json` file and logs the JavaScript array formed from the JSON objects.
- 📝 A new HTML file `product_list.html` is created to display products dynamically on the web page.
- 🔄 Placeholders in the HTML file are used to replace hardcoded values with product data from the `products` array.
- 🔄 The map function is used in JavaScript to iterate over the `products` array and replace placeholders with the corresponding product properties.
- 💡 JavaScript's `replace` method is used to dynamically insert product data (like `name`, `model number`, etc.) into the HTML template.
- ⚙️ The `join` method is employed to combine the individual HTML elements from the products array into a single string.
- 🖥️ After combining, the content is set as the response for the `/products` route, displaying the product list dynamically.
- 🔧 A mistake with content type `application/json` is corrected to `text/html` to properly render the HTML on the web page.
- ✔️ The final result displays a list of products dynamically from the JSON data, rendered via Node.js and JavaScript.
Q & A
What method is used to convert JSON data into a JavaScript object in the script?
-The JSON data is converted into a JavaScript object using the `JSON.parse()` method.
How does the app handle displaying products on the web page?
-The app reads the `products.json` file, converts the JSON data into a JavaScript object, and then loops over the products to display them dynamically in the `product_list.html` file.
What function is used to loop through the products array?
-The `map()` function is used to loop through the `products` array, allowing transformations to be made for each product.
How are placeholders in the HTML file replaced with product data?
-Placeholders in `product_list.html` (e.g., `{{name}}`, `{{model_number}}`) are replaced with product properties (e.g., `prod.name`, `prod.model_number`) using the `replace()` method.
What does the `join()` method do in the script?
-The `join()` method is used to combine the elements of the `productHTMLArray` into a single string, removing any commas between the elements.
Why was the initial HTML response not rendered as expected?
-The initial response was not rendered correctly because the content type was set to `application/json`, which caused the browser to interpret the response as JSON instead of HTML.
How was the issue with rendering the HTML response fixed?
-The issue was fixed by changing the content type from `application/json` to `text/html`, which allowed the browser to render the HTML correctly.
What is the purpose of the `productResponseHTML` variable?
-The `productResponseHTML` variable stores the final HTML content with the product data after replacing the placeholders in the `product_list.html` file.
What does the `fs.readFileSync()` method do in this script?
-The `fs.readFileSync()` method is used to read the content of the `product_list.html` file and store it as a string in the `productListHTML` variable.
How does the server respond when a user navigates to the `/products` URL?
-When the user navigates to the `/products` URL, the server sends an HTML response that contains the list of products with their properties dynamically inserted into the template.
Outlines
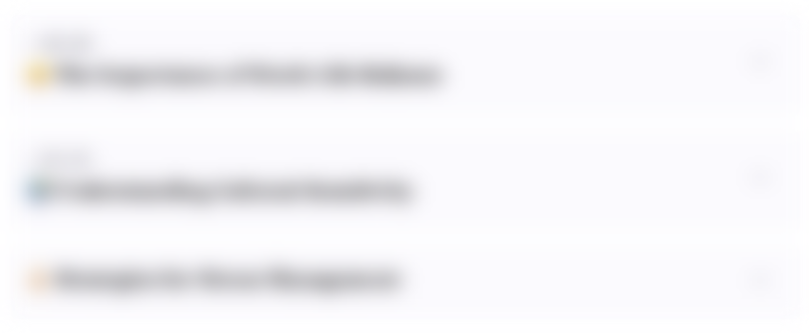
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
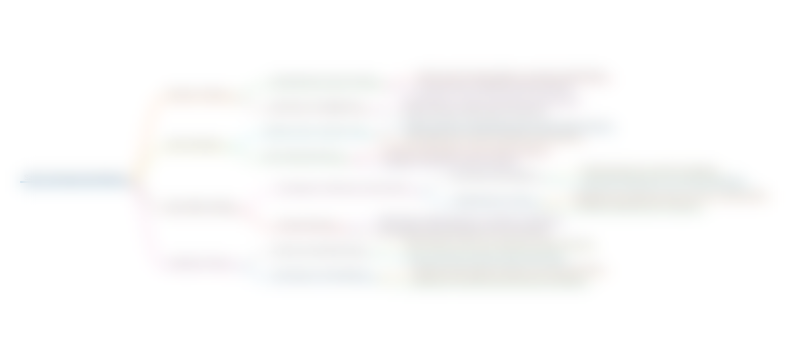
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
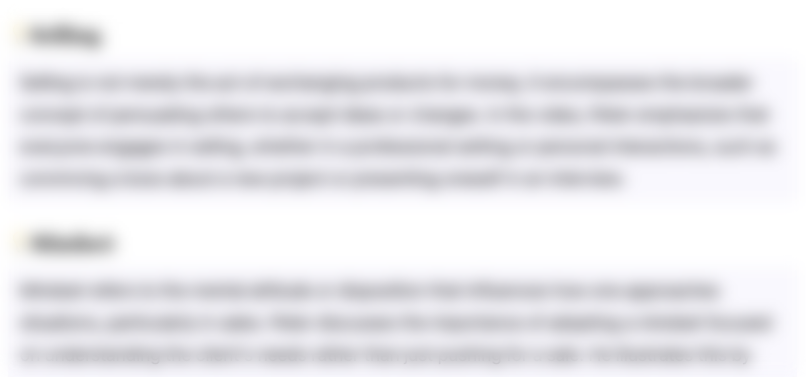
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
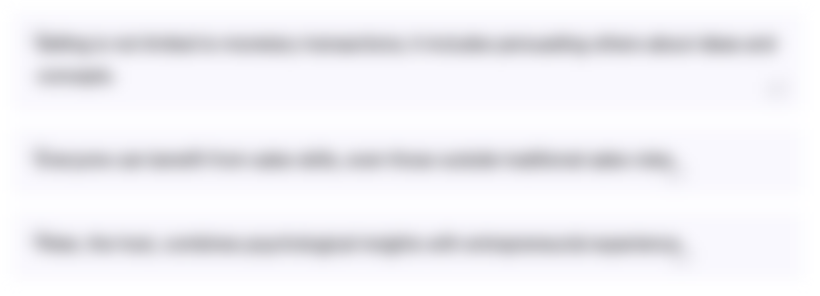
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
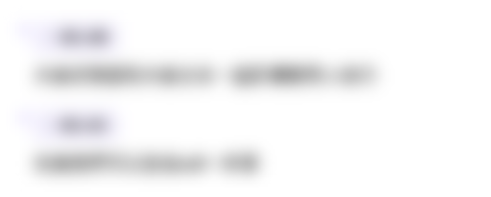
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
5.0 / 5 (0 votes)