Build an API with Django // Part 1 - What is an API?
Summary
TLDRThis video introduces APIs and Django REST framework for building APIs. It explains what APIs are, their purpose, and how they allow different applications to communicate. The tutorial then demonstrates creating a simple API with Django, using JSON responses, and setting up a project with Django REST framework. It also covers the basics of API views and responses, providing a foundation for further exploration in the series.
Takeaways
- 😀 Introduction to building APIs with Django Rest Framework (DRF) and the basics of APIs.
- 📘 API stands for Application Programming Interface, enabling two processes to communicate through programming.
- 🔄 APIs allow services like websites and mobile apps to interact with a central service to send and receive data.
- 📡 APIs return data in formats like JSON, which is used to exchange information between systems.
- 🌐 APIs are essential for integrating multiple services and applications, like web and mobile apps.
- 📋 REST APIs return data only (no HTML or front-end components), allowing developers to use that data in various applications.
- 📦 Django Rest Framework simplifies building APIs by providing built-in tools like serializers, views, and authentication methods.
- 💻 Django’s JSONResponse is used to return data from APIs in JSON format, which is the standard for API data exchange.
- 🔧 The series covers topics such as authentication, permissions, serializers, API views, and integration with front-end frameworks like React and mobile frameworks like Flutter.
- 🚀 DRF provides a user-friendly interface for working with API responses, making API development faster and more intuitive.
Q & A
What is the main focus of the video series?
-The main focus of the video series is to discuss APIs, specifically building APIs with the Django REST framework.
What prerequisite knowledge is recommended before diving into the Django REST framework?
-It is recommended to have a good understanding of Django itself, including views, URLs, templates, and forms.
What is suggested for those who are new to Django?
-For those new to Django, it is suggested to start with courses that help understand Django basics before moving on to the Django REST framework.
What does the acronym API stand for?
-API stands for Application Programming Interface.
What is the primary function of an API?
-The primary function of an API is to allow two processes to communicate with each other by sending and receiving messages.
Why would someone use or build an API?
-People use or build APIs to share applications with others, integrate with services, or to enable communication between multiple services.
How does an API differ from a normal website?
-An API differs from a normal website by only providing the data, typically in a JSON response, rather than rendering HTML, CSS, JavaScript, or images.
What is the significance of returning data in JSON format from an API?
-Returning data in JSON format allows for easy integration with multiple services, as the data can be manipulated in various ways by the receiving application.
What does the Django REST framework offer that normal Django does not?
-The Django REST framework offers tools that make building APIs easier and quicker, including classes and methods specifically designed for handling API requests and responses.
How can one get started with the Django REST framework?
-To get started with the Django REST framework, one can install it using pip, create a virtual environment, and then integrate it into an existing Django project.
What is the purpose of the 'response' import from the Django REST framework?
-The 'response' import from the Django REST framework is used to return responses from API views, similar to Django's built-in JSON response but with additional functionality provided by the framework.
Outlines
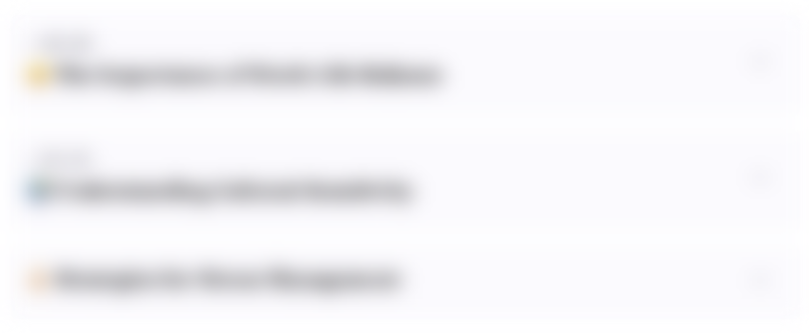
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
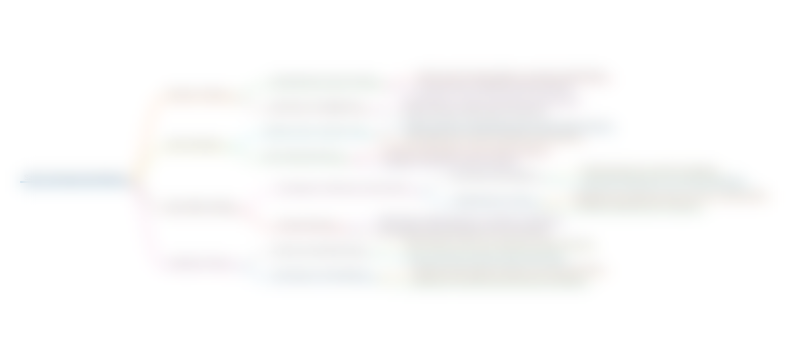
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
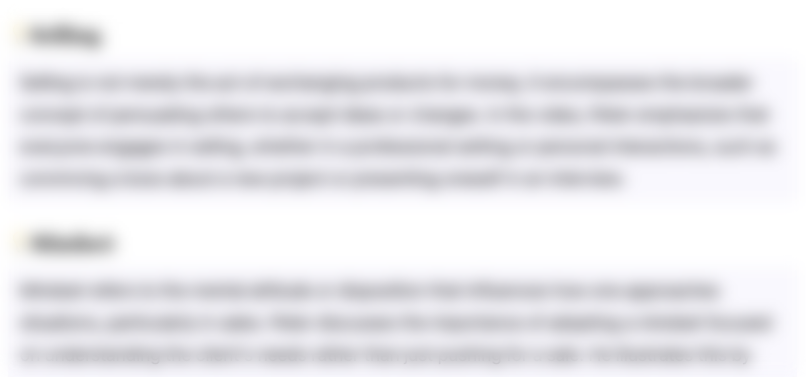
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
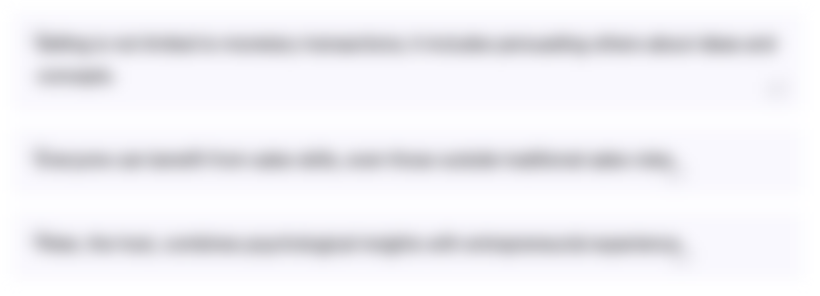
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
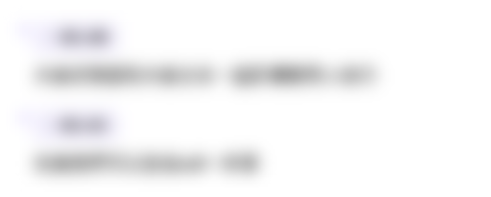
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
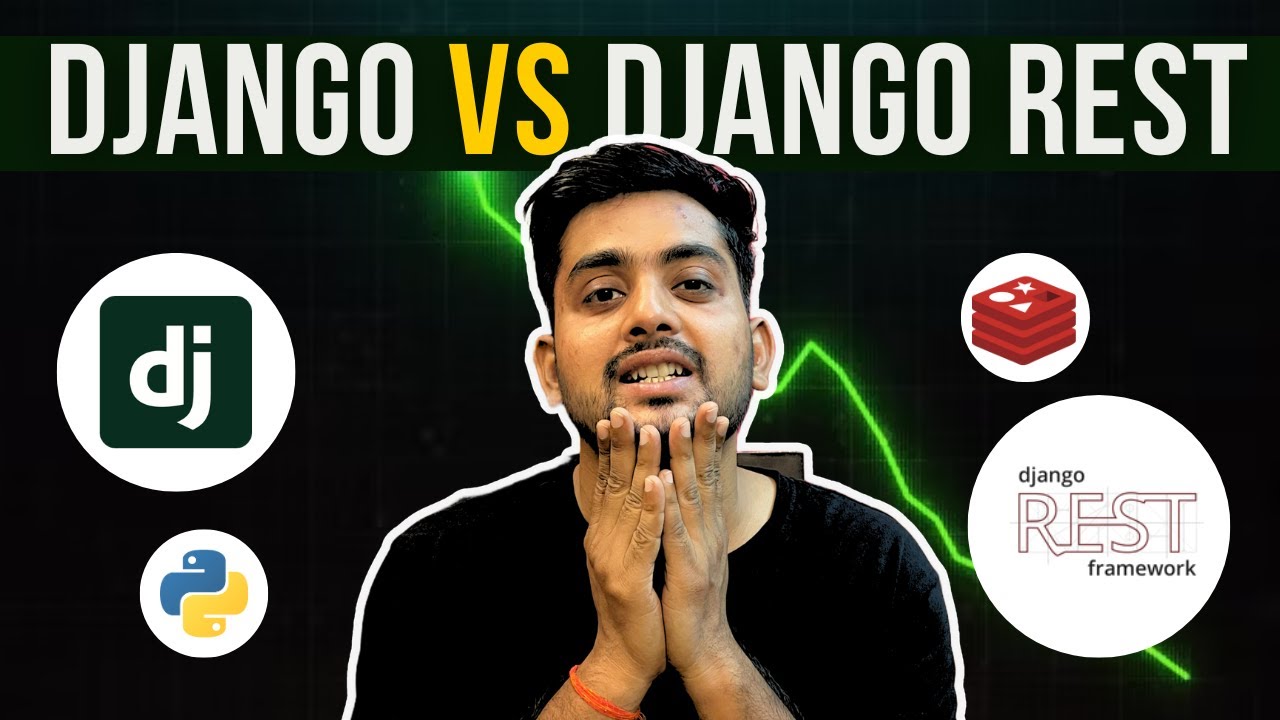
Django vs Django REST Framework: Which One Should You Use in 2024? 🚀

What Is REST API? Examples And How To Use It: Crash Course System Design #3
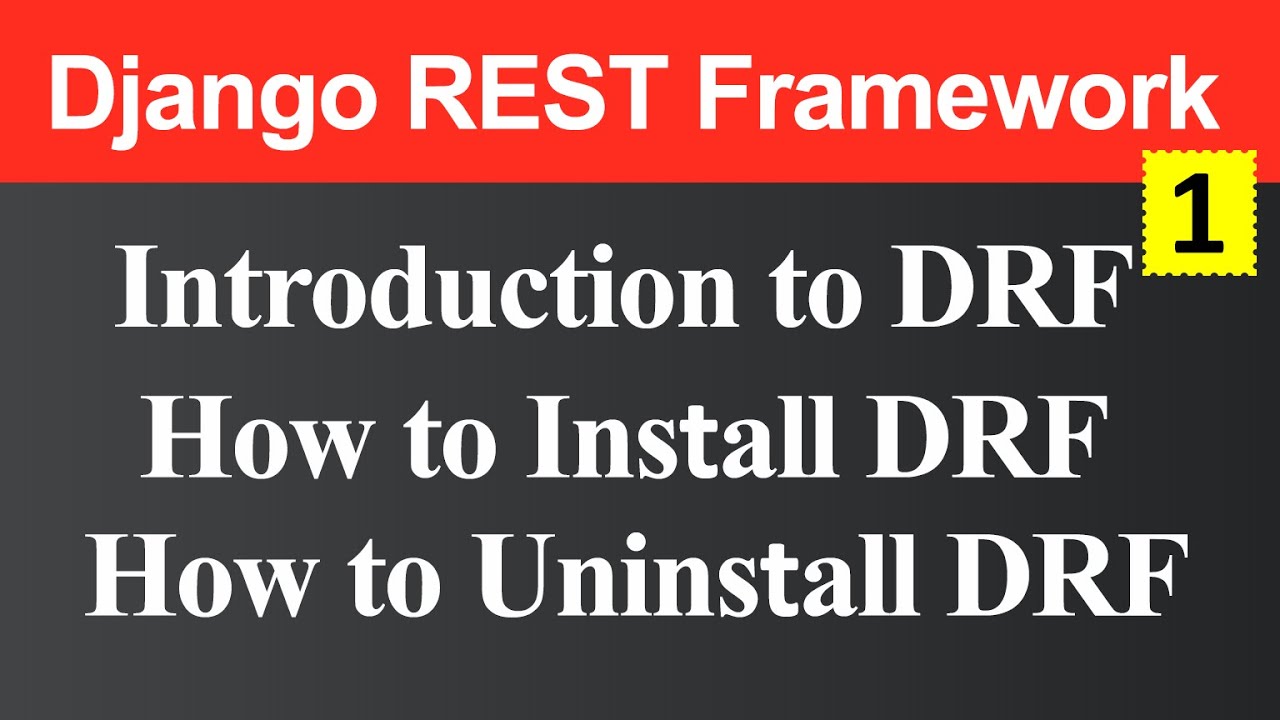
Django Rest Framework and How to Install Uninstall Django Rest Framework (Hindi)
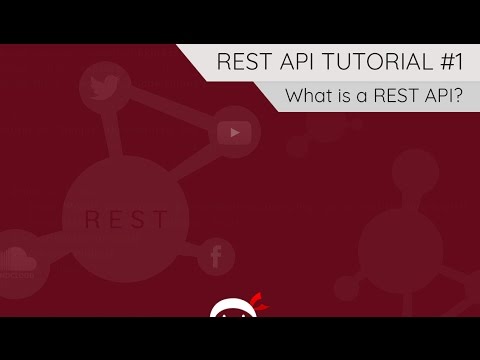
REST API Tutorial #1 - What is a REST API?

The Right Way To Build REST APIs
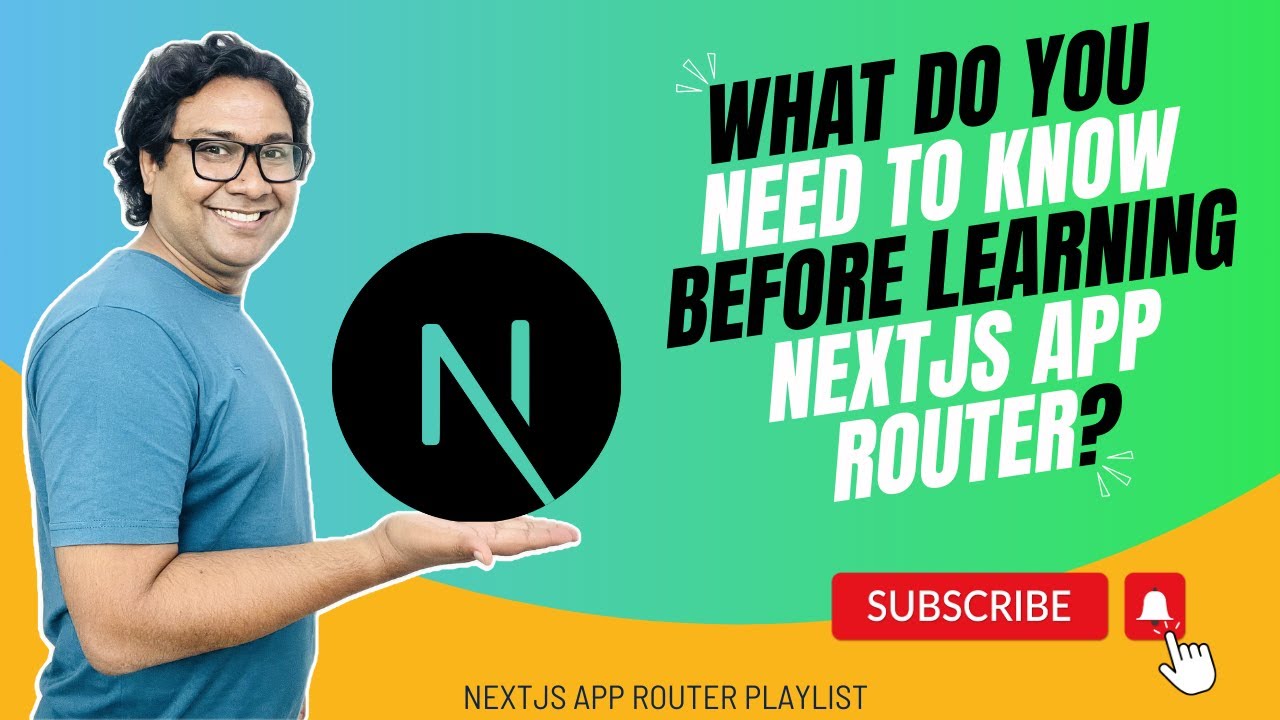
What Do You Need To Know Before Learning NextJS App Router?
5.0 / 5 (0 votes)