Functions in Python | Introduction | Python for beginners #lec56
Summary
TLDRThis video script introduces the concept of functions in Python programming. It explains the necessity and benefits of using functions through real-life analogies, such as sharing a recipe or a mother's multitasking. The script outlines how to define and call functions, emphasizing code reusability, readability, and time-saving aspects. It also distinguishes between built-in and user-defined functions, providing examples and demonstrating the process with a practical coding exercise in Reebok's Blockly World.
Takeaways
- 😀 Functions in Python are blocks of code that perform specific tasks when called.
- 🔑 Functions help in organizing code by grouping related statements together, making the code more modular and easier to maintain.
- 🍲 The analogy of a recipe for idli is used to explain the concept of functions, where the recipe (function) can be followed (called) multiple times to achieve the same result.
- 👩🏫 The importance of functions is emphasized by showing how they can save time and effort by avoiding the repetition of code.
- 📝 The definition of a function in Python uses the 'def' keyword, followed by the function name and parentheses, which may include parameters.
- 🔄 Functions can be called with parameters to perform different tasks based on the input, demonstrating their versatility.
- 🔧 Built-in functions are pre-defined in Python and can be used directly, such as 'print', 'len', 'max', and 'min'.
- 🛠 User-defined functions are created by the programmer to perform specific tasks as needed, enhancing the functionality of the program.
- 📈 The benefits of using functions include increased code reusability, improved readability, and time-saving by avoiding code duplication.
- 🤖 A practical example using a robot in Reebok World is provided to illustrate how defining and calling functions can control actions and simplify programming tasks.
Q & A
What is the main topic discussed in this video script?
-The main topic discussed in this video script is functions in Python programming language.
Why are functions important in programming?
-Functions are important in programming because they promote code reusability, increase code readability, and save time by avoiding the need to repeat code blocks.
Can you give an example of a real-life analogy for functions mentioned in the script?
-The script uses the analogy of a recipe for making idli, a South Indian food, to explain the concept of functions. Just as a recipe can be written down and followed multiple times without repeating the steps, functions in programming can be defined once and called multiple times to perform a specific task.
What are the two major steps involved in using a function in Python?
-The two major steps involved in using a function in Python are defining the function using the 'def' keyword and then calling the function by its name followed by parentheses.
What is the purpose of the 'def' keyword in Python?
-The 'def' keyword in Python is used to define a function, marking the start of the function's declaration.
What is the term used for functions that are already defined in Python?
-The term used for functions that are already defined in Python is 'built-in functions'.
How does defining a function help in reducing the number of lines in a code?
-Defining a function helps in reducing the number of lines in a code by allowing you to encapsulate a block of code that performs a specific task and then reuse that block by simply calling the function, instead of repeating the code block multiple times.
What is the significance of indentation in Python function definitions?
-Indentation in Python function definitions is significant because it demarcates the body of the function, indicating which lines of code are part of the function's execution block.
What is the difference between built-in functions and user-defined functions in Python?
-Built-in functions are pre-defined in Python and can be used directly without any additional code. User-defined functions, on the other hand, are created by the programmer to perform specific tasks as per the program's requirements.
Can a function in Python accept parameters?
-Yes, a function in Python can accept parameters. Parameters are specified within the parentheses in the function definition and can be used to pass values into the function to make it more versatile.
What is the purpose of the 'return' statement in a Python function?
-The 'return' statement in a Python function is used to send a value back to the caller of the function. It is optional and not all functions need to return a value.
Outlines
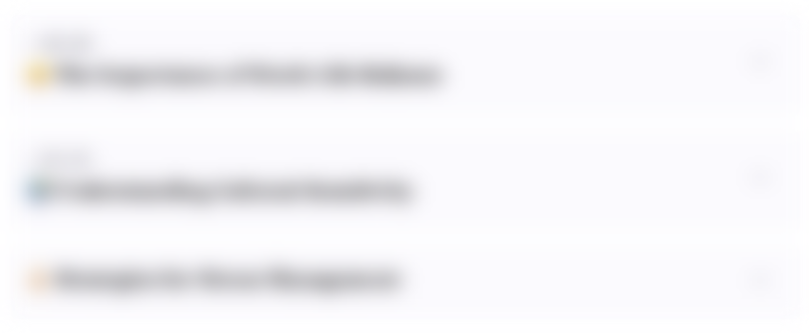
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
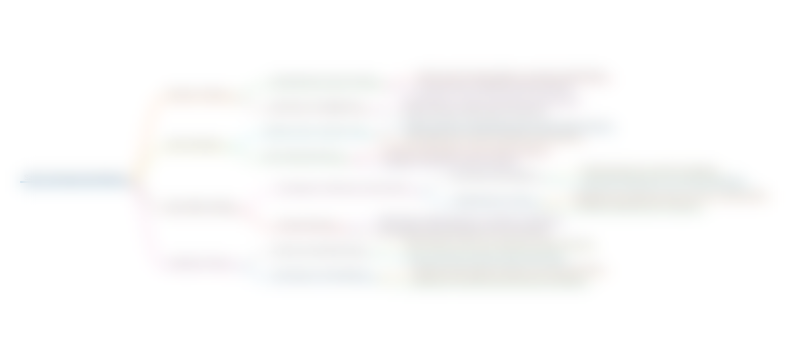
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
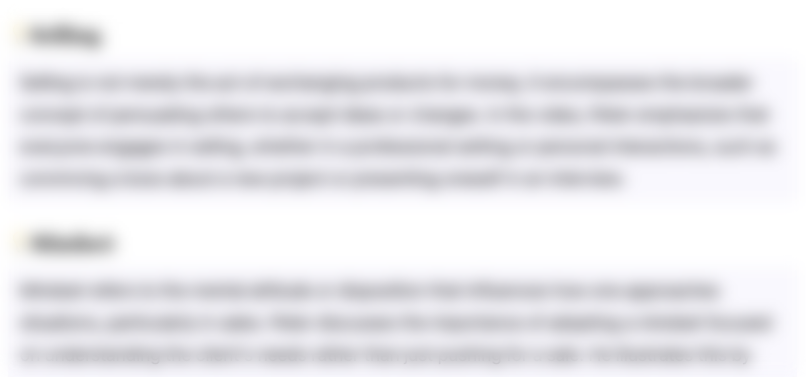
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
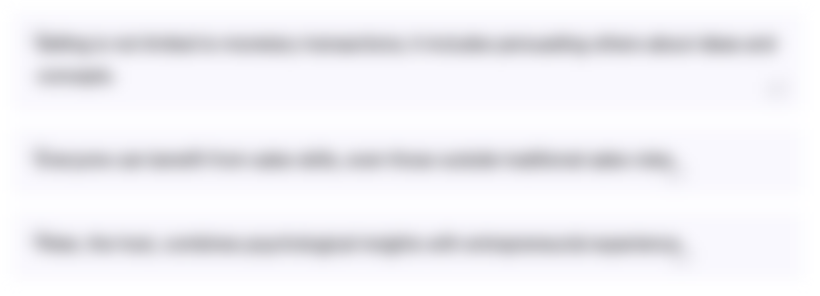
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
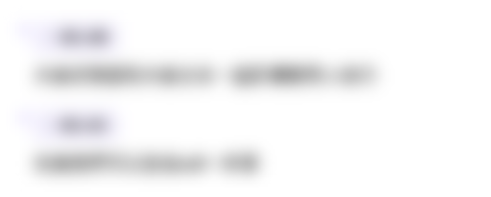
此内容仅限付费用户访问。 请升级后访问。
立即升级5.0 / 5 (0 votes)