#6 Dependency Injection using Spring Boot
Summary
TLDRIn this instructional video, the host, D Ready, guides viewers through implementing dependency injection in a Spring Boot project. Starting with a basic Spring Boot setup without web dependencies, the tutorial progresses to explain the concept of dependency injection and the role of the Spring container, or IoC container. The host demonstrates creating a 'Dev' class, annotating it with @Component to signal Spring to manage its lifecycle, and retrieving it from the container using ApplicationContext's getBean method. The video concludes with a simple example of how Spring Boot can inject dependencies, setting the stage for further exploration in subsequent videos.
Takeaways
- 😀 The video discusses implementing dependency injection using Spring Boot.
- 🛠 The presenter guides through creating a new Spring project without web dependencies to focus on dependency injection.
- 📚 The script explains the concept of a container in Spring, which is an IoC (Inversion of Control) container managing object creation.
- 🌟 The video clarifies that Spring Boot does not automatically create objects for all classes by default to avoid unnecessary burden on the JVM.
- 🔍 It demonstrates how to use the `@SpringBootApplication` annotation to bootstrap a Spring application and create the container.
- 👾 The term 'aliens' is used by the presenter as a nickname for students or developers, emphasizing the virtual world of software development.
- 🛑 The script highlights the error that occurs when trying to use an object not managed by Spring, leading to a 'no qualifying bean' exception.
- 🔑 The solution to the error is using the `@Component` annotation to mark a class as a bean that Spring should manage and instantiate.
- 🔄 The video shows how to retrieve a bean from the Spring container using `getBean` method of the `ApplicationContext`.
- 🔗 The importance of configuring the Spring context properly to manage dependencies is emphasized, which is key to successful dependency injection.
- 🚀 The presenter teases the next video, which will explore more complex scenarios involving multiple layers of dependency injection.
Q & A
What is the main topic of the video?
-The main topic of the video is implementing dependency injection using Spring Boot.
What is the purpose of the Spring Initializer mentioned in the video?
-The Spring Initializer is used to create a new Spring Boot project with the necessary configurations.
Why is the speaker creating a new project without web dependency in the video?
-The speaker is creating a new project without web dependency to focus on implementing dependency injection without the complexity of web-related configurations.
What is the role of the '@SpringBootApplication' annotation in a Spring Boot project?
-The '@SpringBootApplication' annotation is a convenience annotation that adds '@Configuration', '@EnableAutoConfiguration', and '@ComponentScan' annotations to the class, which helps in creating the Spring container and enabling auto-configuration.
What is the term used to describe the container that Spring Boot creates for managing bean lifecycles?
-The term used to describe the container that Spring Boot creates for managing bean lifecycles is the 'IoC container' or 'Spring container'.
What does the speaker mean by 'dependency injection' in the context of this video?
-In the context of this video, 'dependency injection' refers to the process where Spring Boot automatically creates and manages the dependencies of a class, rather than the class creating its own dependencies.
How does the speaker initially try to call the 'build' method in the 'Dev' class?
-The speaker initially tries to call the 'build' method in the 'Dev' class by creating a new instance of the 'Dev' class using 'new Dev()' and then calling 'obj.build()'.
What is the problem with manually creating an object of 'Dev' in the 'main' method as shown in the video?
-The problem with manually creating an object of 'Dev' in the 'main' method is that it goes against the principle of dependency injection, where Spring should manage the creation and lifecycle of objects, not the developer.
Why does the speaker suggest getting the 'ApplicationContext' from the result of the 'SpringApplication.run()' method?
-The speaker suggests getting the 'ApplicationContext' from the result of the 'SpringApplication.run()' method because this method returns an object of 'ConfigurableApplicationContext', which can be used to retrieve beans managed by Spring.
What is the error encountered when trying to get the 'Dev' bean from the 'ApplicationContext' without declaring it as a component?
-The error encountered is 'No qualifying bean of type', which indicates that Spring does not have a bean of the specified type 'Dev' in the container because it has not been marked as a component to be managed by Spring.
What annotation does the speaker add to the 'Dev' class to make Spring Boot create and manage its instance?
-The speaker adds the '@Component' annotation to the 'Dev' class to make Spring Boot create and manage its instance.
Outlines
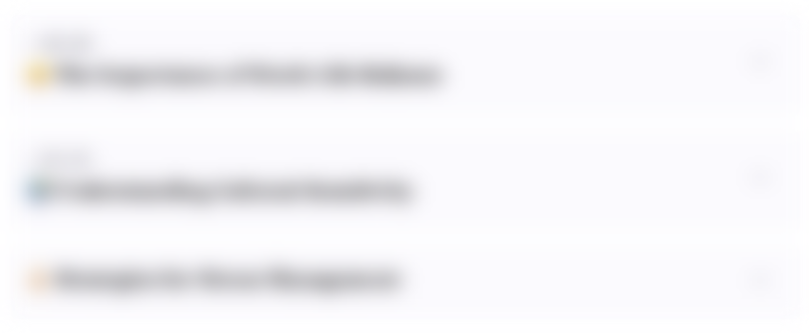
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
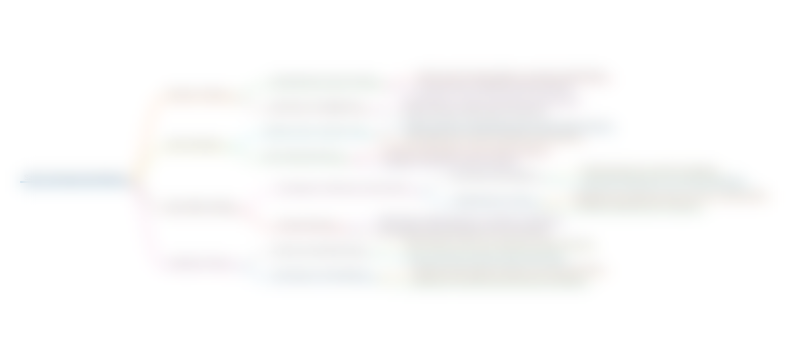
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
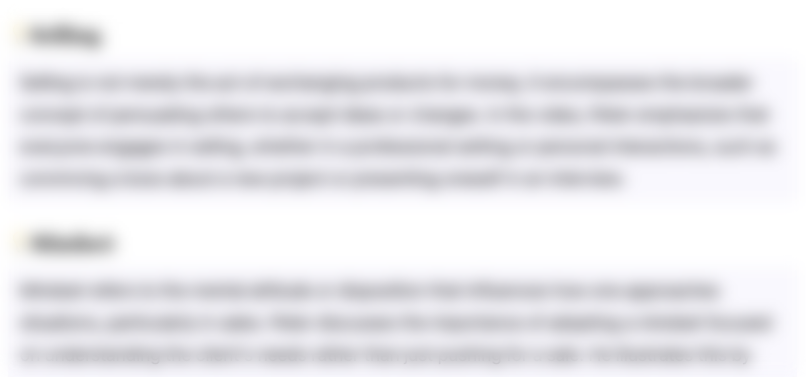
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
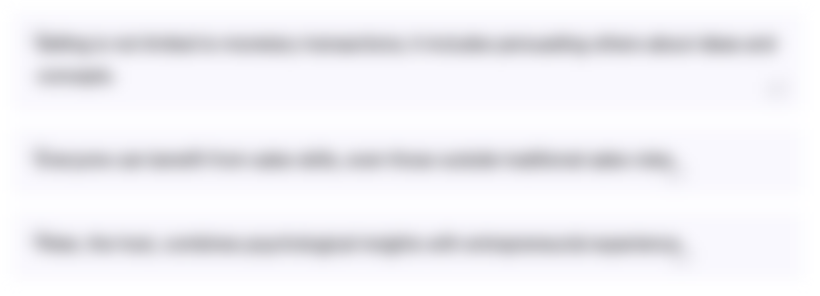
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
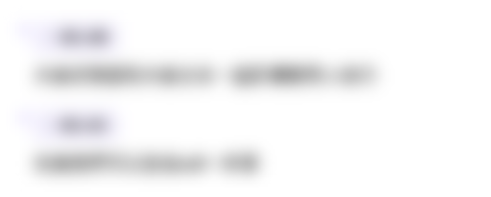
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)