Python Pandas Tutorial 5: Handle Missing Data: fillna, dropna, interpolate
Summary
TLDRThis tutorial explores handling missing data in pandas, a Python library. It demonstrates using fill, interpolate, and drop methods on a dataset of NYC weather data with missing values. The video guides through converting string dates to a datetime index, replacing missing values with specified values or forward/backward filling, and using interpolation for estimates. It also covers advanced techniques like axis filling, limit parameter for fill, and inserting missing dates with reindexing, offering a comprehensive guide for data preprocessing.
Takeaways
- π Handling missing data in pandas is crucial when working with datasets that have incomplete values.
- πΎ The tutorial uses New York City's weather data as an example to demonstrate handling missing data.
- π Converting a string date column to a datetime column is done using the 'parse_dates' argument.
- π Setting a column as an index in a DataFrame requires the 'set_index' method with 'inplace=True'.
- β Missing values can be handled using methods like 'fillna', 'interpolate', and 'dropna'.
- π’ 'fillna' can replace all NaN values with a specified value or a dictionary of values for specific columns.
- β‘οΈ The 'ffill' method in 'fillna' carries forward the previous day's value to fill missing data.
- β¬ οΈ 'bfill' is another method in 'fillna' that uses the next day's value to fill missing data.
- π Interpolation methods like linear, time, and others can provide better estimates for missing values.
- βοΈ The 'dropna' method can be used to drop rows or columns with missing values, with options to specify conditions.
- π Missing dates can be inserted into the DataFrame using 'date_range' and 'reindex'.
Q & A
What is the main topic of the tutorial?
-The main topic of the tutorial is how to handle missing data in pandas, a Python library for data analysis.
What kind of data does the CSV file in the tutorial contain?
-The CSV file contains New York City's weather data with some missing values, including data for 2nd and 3rd January.
What are the three methods covered in the tutorial for dealing with missing data in pandas?
-The three methods covered are fillna, interpolate, and dropna.
Why might the tutorial recommend converting a string column to a date column?
-Converting a string column to a date column allows for better data manipulation and analysis, especially when setting the date as an index for a DataFrame.
What does the fillna method do in pandas?
-The fillna method in pandas is used to replace missing values (NaNs) with a specified value or a method for estimation.
How can you specify different fill values for different columns using the fillna method?
-You can specify different fill values for different columns by passing a dictionary to the fillna method, where the keys are the column names and the values are the fill values.
What does the forward fill method do when dealing with missing data?
-The forward fill method carries forward the value from the previous day's non-missing data to fill in the missing values.
What is the purpose of the 'limit' parameter in the fillna method?
-The 'limit' parameter in the fillna method restricts the number of consecutive NaNs to be filled with the specified fill value.
What is interpolation and how is it used in pandas to handle missing data?
-Interpolation is a method used to estimate intermediate values between two known data points. In pandas, the interpolate method can be used to fill missing values with estimated values based on different interpolation methods like linear, quadratic, or time-based.
How can you drop rows with missing data in pandas?
-You can drop rows with missing data in pandas using the dropna method. You can specify parameters like 'how' to determine if rows with any or all missing values should be dropped, and 'thresh' to define the minimum number of non-NA values required to keep a row.
What is the process of re-indexing in pandas and why might you need to do it?
-Re-indexing in pandas is the process of conforming a DataFrame to a new set of labels for its index. You might need to re-index if you want to insert missing dates or align the data with a complete date range.
Outlines
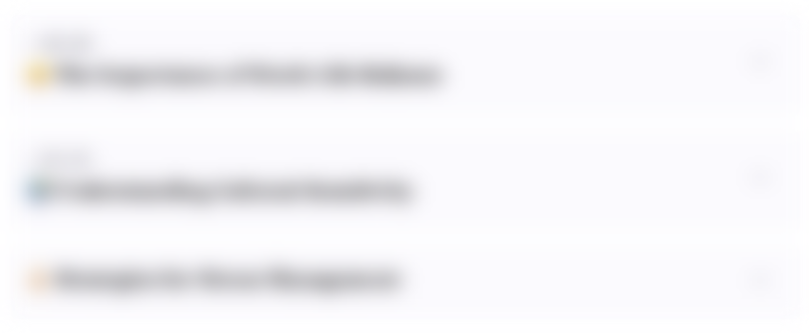
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
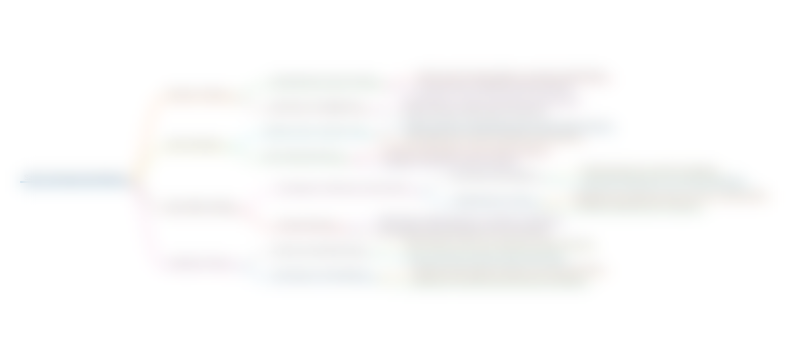
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
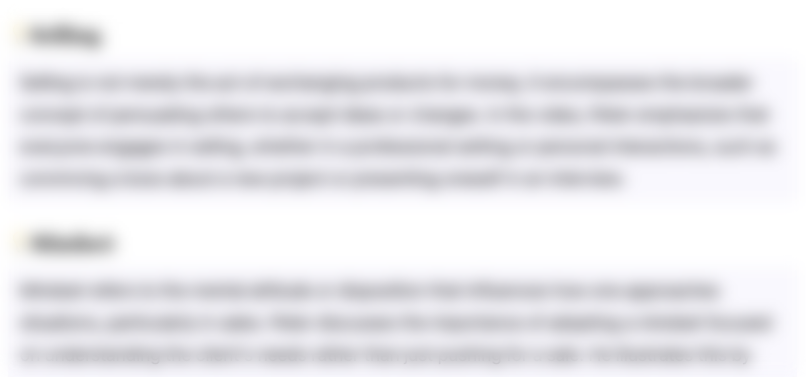
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
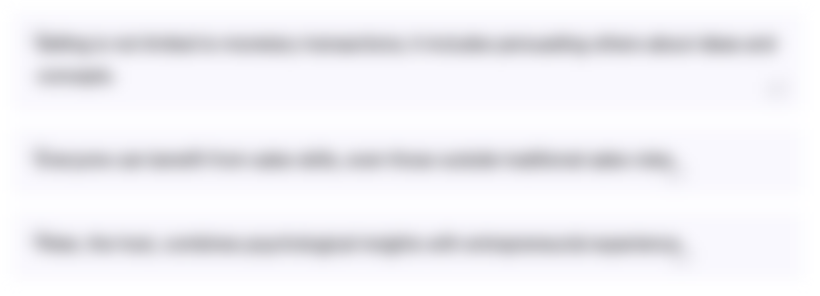
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
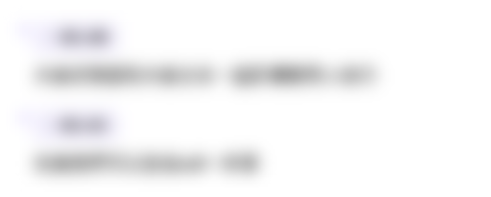
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)