20 Advanced Coding Tips For Big Unity Projects
Summary
TLDRThis video script offers a wealth of Unity coding tips for advanced developers, aiming to avoid common pitfalls in large-scale game development. It covers best practices like meaningful variable names, commenting, encapsulation, and planning. The script delves into more complex topics such as using enums, co-routines, Singletons, interfaces, inheritance, and scriptable objects for better code structure and maintainability. It also emphasizes the importance of version control and refactoring for scalable game development.
Takeaways
- 📝 **Clear Naming Conventions**: Use concise, meaningful variable names and follow a strict naming convention for consistency, especially in a team environment.
- 🗒️ **Frugally Use Comments**: Comments should explain the purpose of complex variables or code blocks, but avoid using them for obvious explanations or as a crutch for old code.
- 🔍 **Encapsulate with Functions**: Break down long methods and complex logic into smaller, manageable functions to maintain code readability and abstraction.
- 📘 **Plan Before Coding**: Sketch out diagrams, flowcharts, and pseudocode before writing the actual code to save time on debugging and rewriting.
- 🔏 **Use Getters and Setters**: Employ getters and setters to control access to variables, preventing unintended modifications and maintaining encapsulation.
- 🔄 **Component-Based Architecture**: Design scripts as components, allowing for flexibility and independence, which can be easily added or removed without affecting other features.
- 🔑 **Enums for Fixed Choices**: Utilize enums to represent a set of predefined choices, making it easy to manage and modify options within the Unity Inspector.
- ⏱️ **Coroutines for Delayed Tasks**: Use coroutines to handle tasks that require delays or sequential execution without blocking the main program flow.
- 📚 **Singleton Pattern**: Implement the Singleton pattern for classes that should have only one instance throughout the application, providing global access while maintaining control.
- 🎯 **Event-Driven Programming**: Utilize C# events to handle situations where scripts need to react to certain triggers or changes without creating tight coupling between systems.
- 🔄 **Inheritance and Interfaces**: Apply inheritance to share code between related classes and interfaces to ensure that different classes conform to a common structure or behavior.
Q & A
What common issue does the video script address in game development?
-The script addresses the issue of poor coding practices leading to unorganized, unmanageable, and confusing code, often referred to as 'spaghetti code,' which can halt progress and force developers to abandon their projects.
What is the first coding tip provided in the script for naming variables?
-The first coding tip suggests that variable names should be concise, meaningful, and follow a consistent naming convention, such as using Pascal case for public fields, camel case for private instance variables, and all caps for constants.
Why is it recommended to use comments in code?
-Comments are recommended to describe the purpose of complicated variables, explain confusing blocks of code, or remind the developer of things to implement later. However, they should not be used to explain obvious things or to hoard old code.
What is the purpose of encapsulating code in functions?
-Encapsulating code in functions helps maintain a level of abstraction, making it easier to understand what the program is doing at a high level. It also allows for better organization and readability of the code.
Why is it advised to plan out code before writing it?
-Planning out code before writing it can save time spent on debugging and rewriting. It allows developers to think through the logic and structure of the code, leading to more efficient and error-free development.
What is the recommended approach for accessing a variable in a separate script?
-The recommended approach is to make the variable private and then add a public function, known as a getter, which returns the variable's value. This prevents external scripts from modifying the variable directly and causing logic errors.
How can the 'SerializeField' attribute be used in Unity?
-The 'SerializeField' attribute can be used to allow a variable to be accessed in the Unity Inspector without making it publicly accessible from other classes, thus preventing unintended modifications.
What is the advantage of using components in Unity's component architecture?
-Using components allows for a more organized and modular approach to scripting, where each script handles a specific feature or system. This makes it easier to add or remove features without impacting other parts of the game.
What is an enum and how can it be used in Unity?
-An enum is a data type that represents a closed set of choices. In Unity, enums can be set inside the Inspector with a dropdown menu, allowing developers to easily change the value of the enum without directly modifying the code.
Why are coroutines useful in game development?
-Coroutines are useful for spreading a task over multiple frames, allowing for code execution with delays without blocking the main program. They are particularly helpful for methods that contain sequences of events or procedural animations.
What is a Singleton pattern and when should it be used?
-A Singleton pattern is a design pattern that restricts the instantiation of a class to one single instance. It should be used when a script needs to exist within the Unity scene and be referenced by many different scripts, ensuring that only one instance of the script is available at a time.
How can interfaces help in object-oriented programming?
-Interfaces help in object-oriented programming by defining a contract for methods that must be implemented by any class that adopts the interface. This allows for similar behavior across different classes without enforcing a relationship between them.
What is the benefit of using inheritance in game development?
-Inheritance allows a class to extend another class, inheriting its public and protected members. This promotes code reuse and organization, preventing the need to rewrite shared code across different classes.
How can scriptable objects improve game development workflow?
-Scriptable objects allow for bundling data that can be accessed in the Inspector without attaching them to a prefab or object in the scene. They are beneficial for managing game data and assets that need to be editable in the Unity Editor.
What is the importance of using version control software in game development?
-Version control software is crucial for backing up project files at every stage of development, allowing for easy collaboration and the ability to revert to previous versions if needed. It prevents loss of work and streamlines the development process.
Why is it important to refactor code and write it well from the start?
-Refactoring and writing code well from the start is important because it reduces the likelihood of needing to rewrite code later, which can be a daunting and time-consuming task. It also ensures that the code is maintainable and scalable.
Outlines
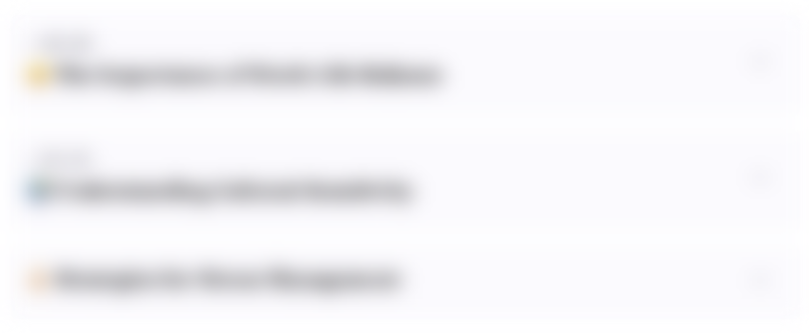
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
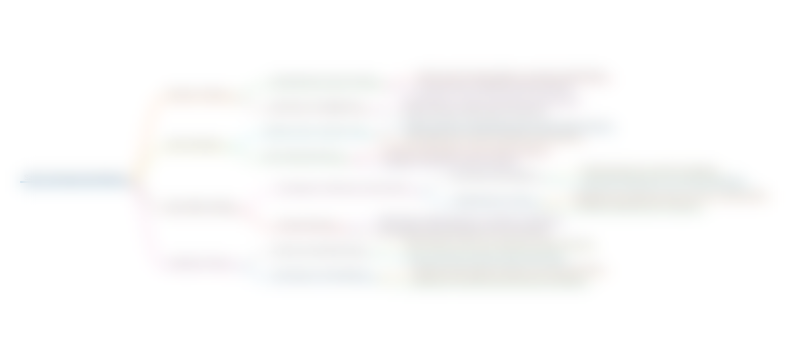
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
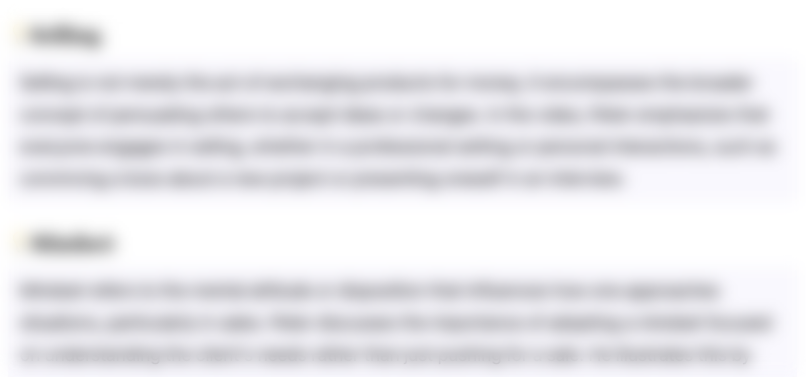
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
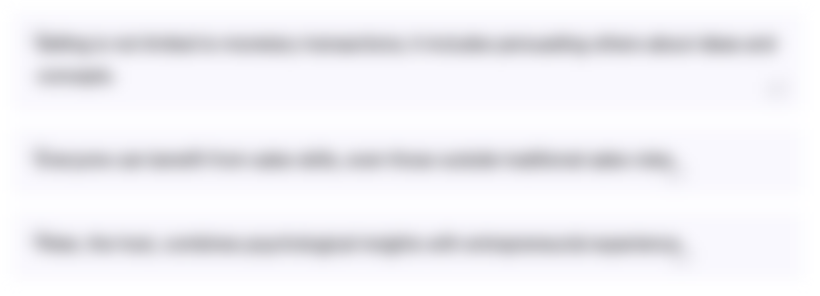
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
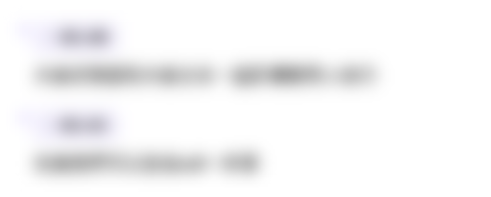
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
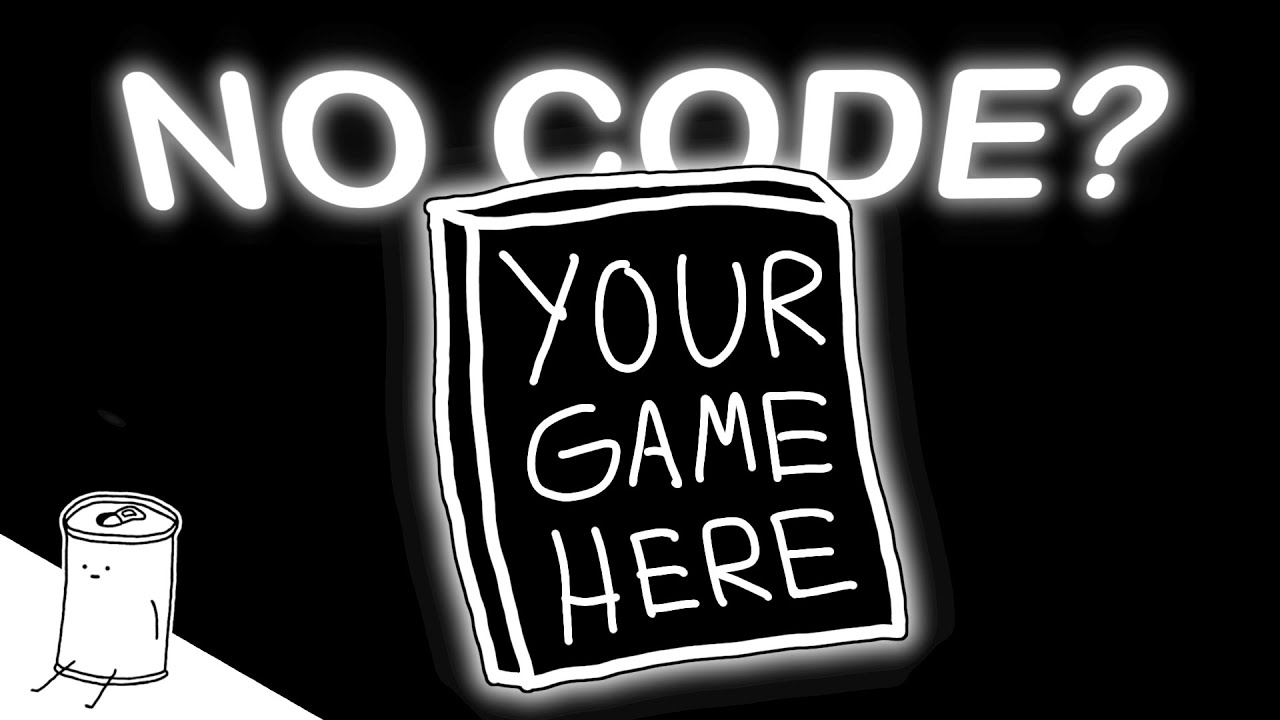
How to make YOUR dream game with no experience

This Problem Changes Your Perspective On Game Dev
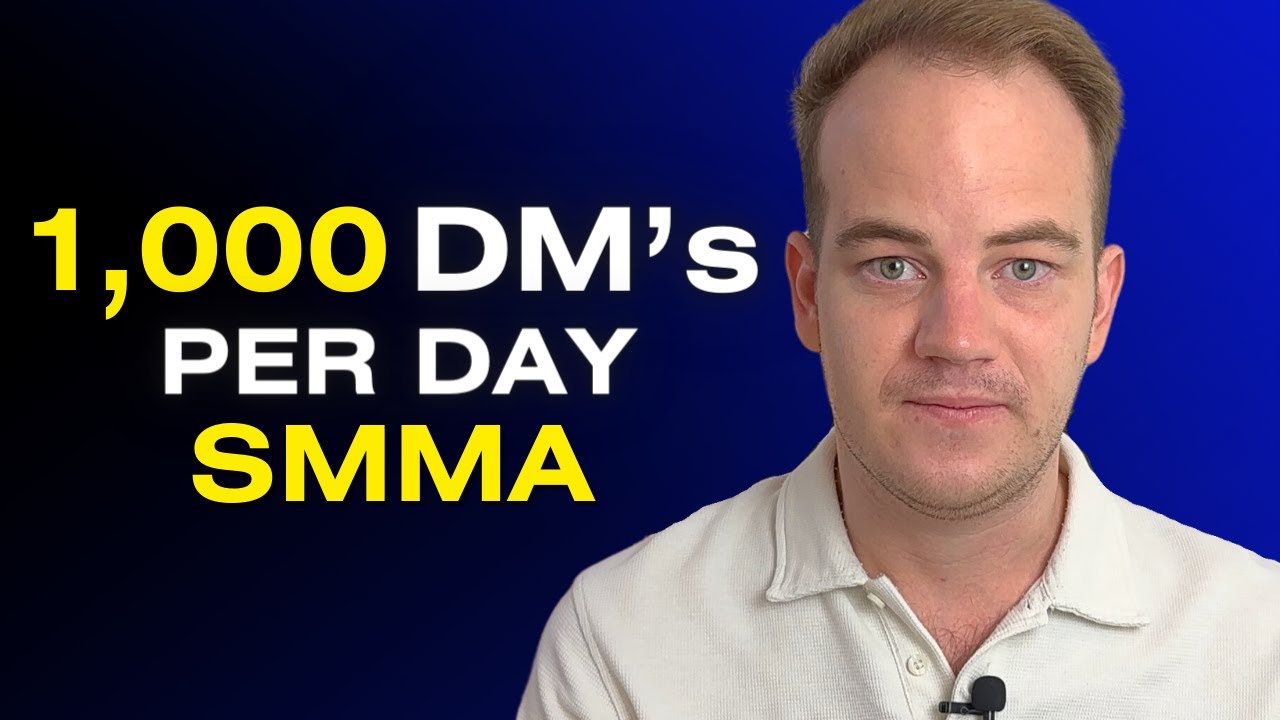
How I send 1,000 IG DM's per day SMMA (autopilot)

Game Dò Mìn (Minesweeper) JAVA - #7 Xử lý mở ô, thắng thua P.2. Thêm chức năng Cắm cờ
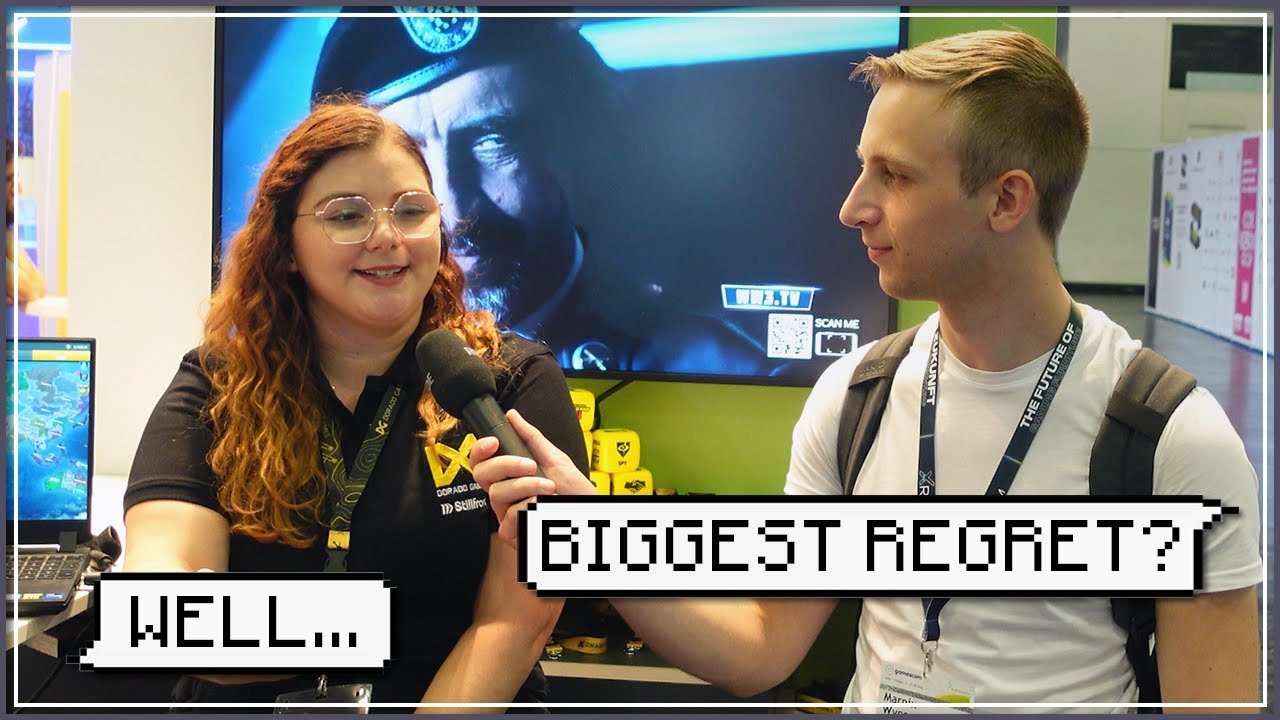
I asked 100 gamedevs their best beginner advice

Google Gemini vs Gemini Advanced: Which is Better? (2025)
5.0 / 5 (0 votes)