Comments, Escape Sequences & Print Statement | Python Tutorial - Day #5
Summary
TLDRThis video script from the '100 Days Of Code' series focuses on Python's Comments, Escape Sequence Characters, and Print Statements. It explains how to use comments to add non-executable text for clarity or reminders. The script also covers escape sequences for including special characters like new lines and quotes in strings. Additionally, it explores the print statement's functionality, including how to print multiple values with customizable separators and end characters, and mentions the optional 'file' parameter for writing to files.
Takeaways
- 💡 Comments are used in code to explain functionality or to avoid executing certain parts of the code during testing.
- 🔑 Escape Sequence Characters like '\n' and '\"' allow programmers to include characters in strings that would otherwise cause errors.
- 📝 Print statements in Python can print text enclosed in double quotes by using the escape character '\"'.
- 🚫 The error 'EOL while scanning string literal' occurs when a new line is introduced in a string without using an escape sequence.
- ✅ The 'print' function can print multiple values separated by a specified separator, defaulting to a space.
- 🔄 The 'end' parameter in 'print' allows customization of what is printed at the end of a statement, defaulting to a new line '\n'.
- 📁 Comments can be added to code using the '#' symbol for single-line comments or triple quotes for multi-line comments.
- 🛠️ IDEs like Replit provide shortcuts like 'Ctrl + /' or 'Command + /' for commenting and uncommenting lines of code.
- 📑 The 'file' parameter in 'print' allows writing directly to a file object, with 'sys.stdout' as the default for console output.
- 🔍 The video emphasizes the importance of understanding comments and escape sequences for effective Python programming.
Q & A
What is the purpose of comments in programming?
-Comments are used in programming to add text that the interpreter does not execute. They are helpful for explaining blocks of code, reminding the programmer or other developers about the purpose and functionality of the code, and for avoiding the execution of specific parts of code during testing.
How do you create a single-line comment in Python?
-In Python, a single-line comment is created by prefixing the comment text with a hash symbol (#). Anything following the hash symbol on the same line is ignored by the Python interpreter.
What is an Escape Sequence Character and why is it used?
-An Escape Sequence Character is a sequence introduced by a backslash (\) that represents a special character or instruction. It is used to include characters in a string that cannot be directly used, such as a new line (\n) or a double quote (\").
How do you print a new line in a string using an Escape Sequence Character?
-To print a new line in a string, you use the Escape Sequence Character '\n'. When this sequence is included in a string, Python will interpret it as a command to start a new line when the string is printed.
What is the default behavior of the 'end' parameter in the print statement?
-The default behavior of the 'end' parameter in the print statement is to print a new line (\n) at the end of the output. This means that by default, when a print statement is executed, the cursor moves to the next line.
How can you change the default separator in the print statement?
-You can change the default separator in the print statement by using the 'sep' parameter followed by the separator character you want to use. By default, the separator is a space.
What is the purpose of the 'file' parameter in the print statement?
-The 'file' parameter in the print statement specifies a file object to which the output should be directed. By default, it is set to 'sys.stdout', which means the output is directed to the standard output, typically the console.
How do you print multiple values in a single print statement?
-You can print multiple values in a single print statement by separating them with commas. The 'sep' parameter can be used to specify the separator between these values.
What is the difference between 'sep' and 'end' parameters in the print statement?
-The 'sep' parameter specifies the separator between multiple values in a single print statement, while the 'end' parameter specifies what to print at the end of the print statement. The default separator is a space, and the default end character is a new line (\n).
How can you prevent the automatic new line after a print statement?
-You can prevent the automatic new line after a print statement by setting the 'end' parameter to an empty string ('end=""'). This will cause the next print statement to start on the same line.
What is the significance of the shortcut (Ctrl + /) mentioned in the script?
-The shortcut (Ctrl + /) is used to quickly comment or uncomment lines of code in many Integrated Development Environments (IDEs). This is a time-saving feature that allows developers to toggle comments on or off for selected lines of code.
Outlines
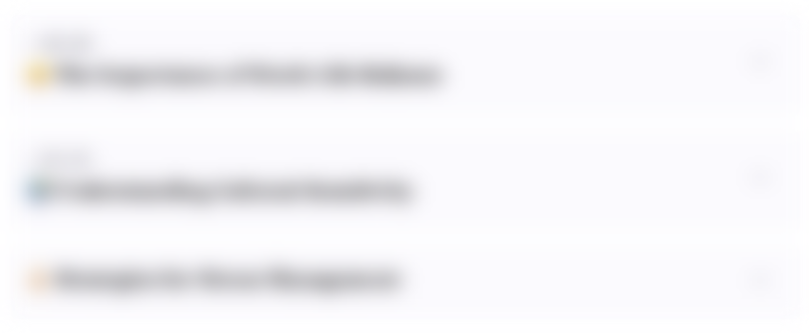
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
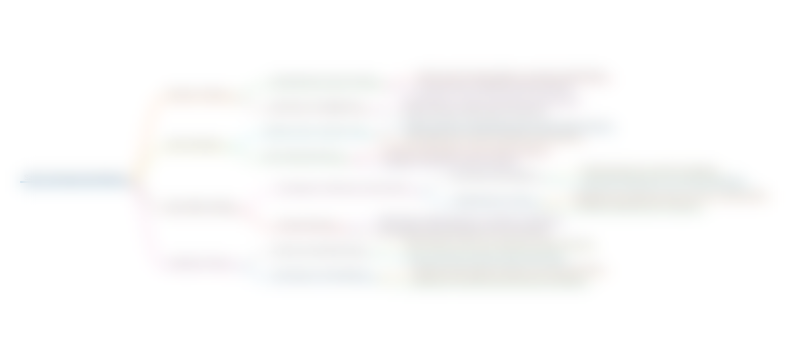
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
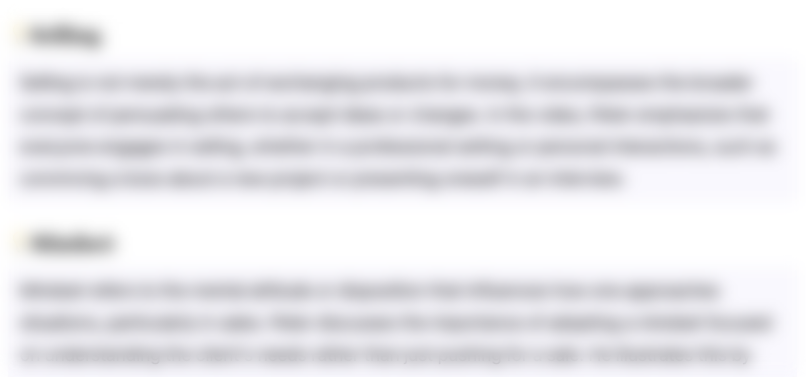
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
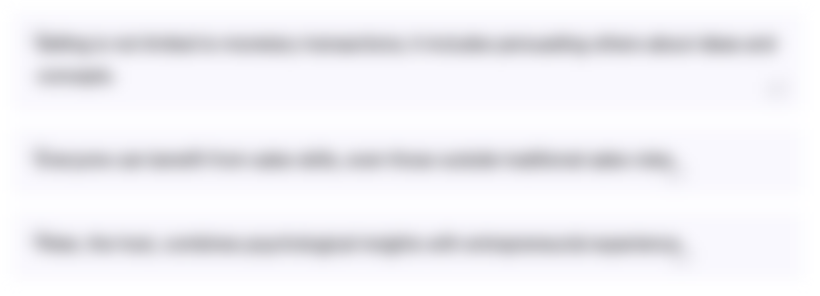
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
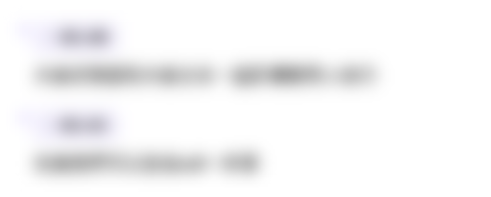
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)