Packages
Summary
TLDRThe video script delves into Java's organizational structure, emphasizing the role of packages in avoiding naming conflicts and managing class hierarchies. It explains how packages are used to differentiate classes with the same name in different contexts and how they are reflected in file system directories. The script also touches on import statements, the visibility of classes and methods within packages, and introduces the 'protected' access modifier, which allows subclasses to access certain members while restricting access to other classes.
Takeaways
- π Java organizes code using a hierarchy of classes, with one public class per file and potential private classes within those files.
- π Packages in Java serve as an organizational unit to avoid name conflicts between classes with the same name but different contexts.
- π The package structure helps to disambiguate class names, ensuring that classes from different packages can coexist without naming conflicts.
- π Java uses a dot-separated hierarchy for package names, reflecting a parent-child relationship similar to internet domain names but in reverse.
- π Importing classes from other packages can be done by specifying the package name, allowing access to functions from packages like `java.math.BigDecimal`.
- π The package hierarchy is mirrored in the file system organization, with directories corresponding to package names and subdirectories for sub-packages.
- π The use of `java.*` allows for importing all classes within the `java` package, but `java.*` cannot be used to import everything from Java, only specific sub-packages.
- π When creating a class, you can specify its package with a package declaration at the top of the class file, which is essential for disambiguation.
- π If no package name is explicitly given, classes belong to an anonymous package, which includes all classes in the same directory.
- π Java allows omission of visibility modifiers like public and private for classes, methods, and variables, defaulting to package-private visibility.
- π The `protected` modifier provides a level of visibility that is public within a class hierarchy but private to classes outside of that hierarchy.
Q & A
What is a package in Java and why is it used?
-A package in Java is an organizational unit used to group related classes and interfaces, helping to avoid name conflicts by providing a namespace. It is used to organize code into logical groups and to distinguish between classes with the same name but in different contexts.
How does Java handle classes with the same name but in different contexts?
-Java uses packages to differentiate between classes with the same name. By placing classes into different packages, it ensures that each class has a unique fully qualified name, thus avoiding naming conflicts.
What is the typical terminology for packages in Java?
-Java uses a dot-separated hierarchy for package names, which reflects the hierarchical structure of the packages. For example, 'java.math.BigDecimal' indicates that BigDecimal is a package inside the 'math' package, which in turn is inside the 'java' package.
How can you import functions from other packages in Java?
-In Java, you can import functions or classes from other packages using the 'import' statement followed by the package name. For example, 'import java.math.BigDecimal;' imports the BigDecimal class from the java.math package.
What does the package name reflect in terms of file storage on disk?
-The package name reflects a hierarchy in how files are stored on disk. Each package is typically represented as a directory, and sub-packages as sub-directories, mirroring the package hierarchy.
What is the significance of using 'java.*' in an import statement?
-Using 'java.*' in an import statement imports all the classes from the 'java' package and its sub-packages. However, it's important to note that the '*' can only be used at the bottom level of the package hierarchy and cannot be used to import everything from 'java'.
What is the purpose of the 'protected' access modifier in Java?
-The 'protected' access modifier in Java allows a member to be accessible within its own class, any subclasses, and within the same package. It is a way to provide more visibility than 'private' but less than 'public'.
What is the default visibility of a class, method, or variable in Java if no access modifier is specified?
-If no access modifier is specified for a class, method, or variable in Java, it is 'package-private' by default, meaning it is accessible within the package but not outside of it.
How does Java handle the organization of classes within the same directory?
-In Java, classes within the same directory that do not explicitly specify a package belong to the same anonymous package. This means they are implicitly part of the same package and can access each other's members without the need for explicit imports.
Why is it important to use a package name in a Java class file?
-Using a package name in a Java class file is important for organizing the class within a specific namespace, preventing naming conflicts, and making it clear which package the class belongs to. It also helps in maintaining the hierarchical structure of the code.
What is the relationship between the package structure and the class hierarchy in Java?
-The package structure and the class hierarchy in Java are related in terms of visibility and organization. Packages help organize classes into namespaces, while the class hierarchy determines the inheritance and access to members. The 'protected' modifier, for example, allows access to members within the same instance hierarchy but restricts access outside of it.
Outlines
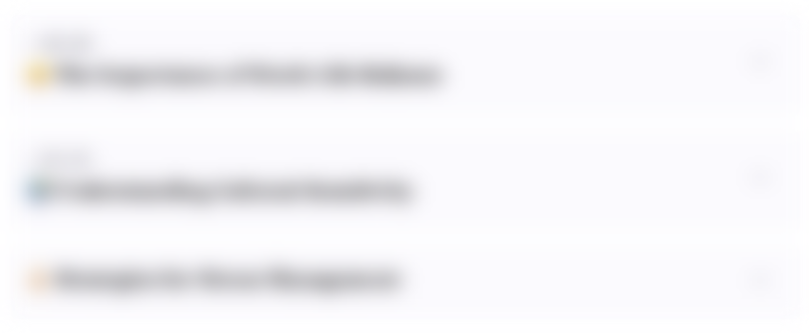
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
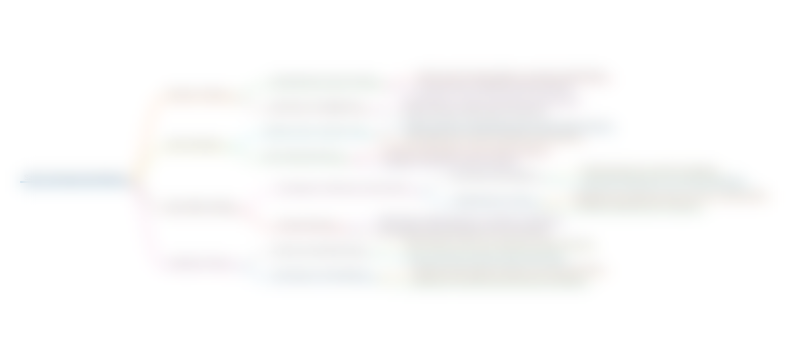
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
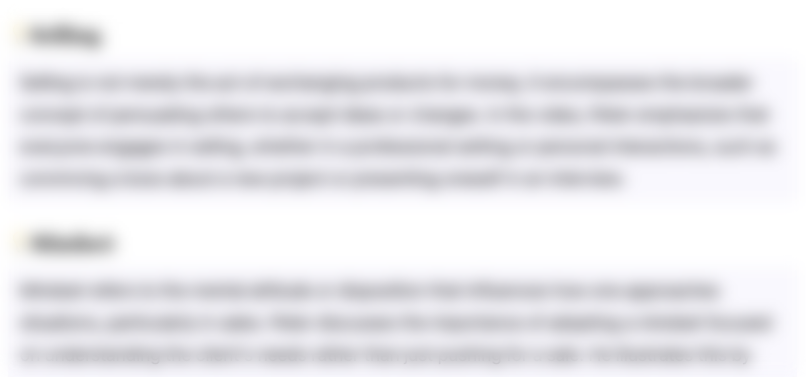
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
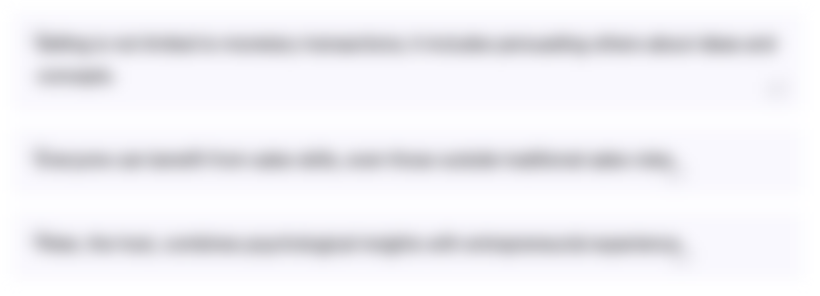
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
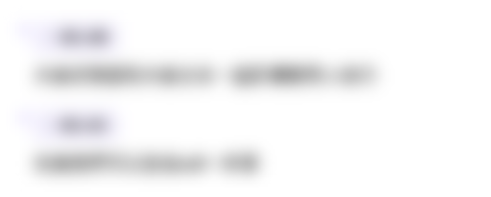
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
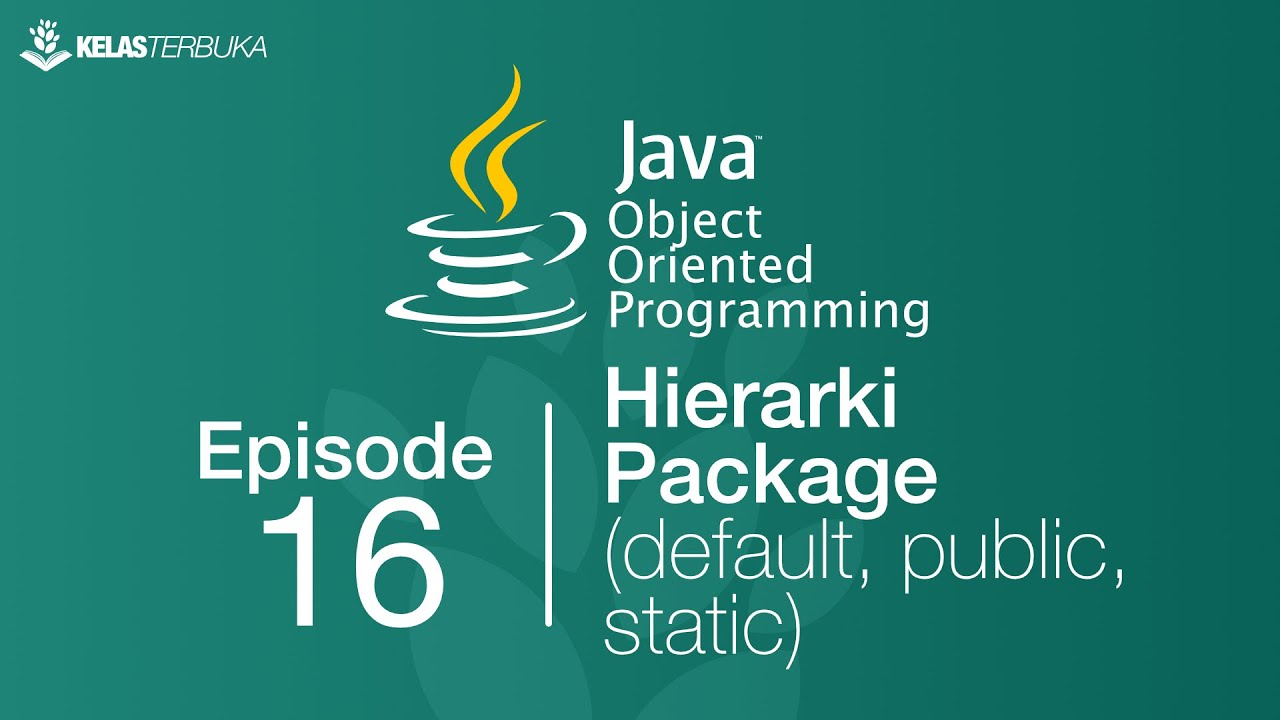
Belajar Java [OOP] - 16 - Hierarki Package (default, public, static)
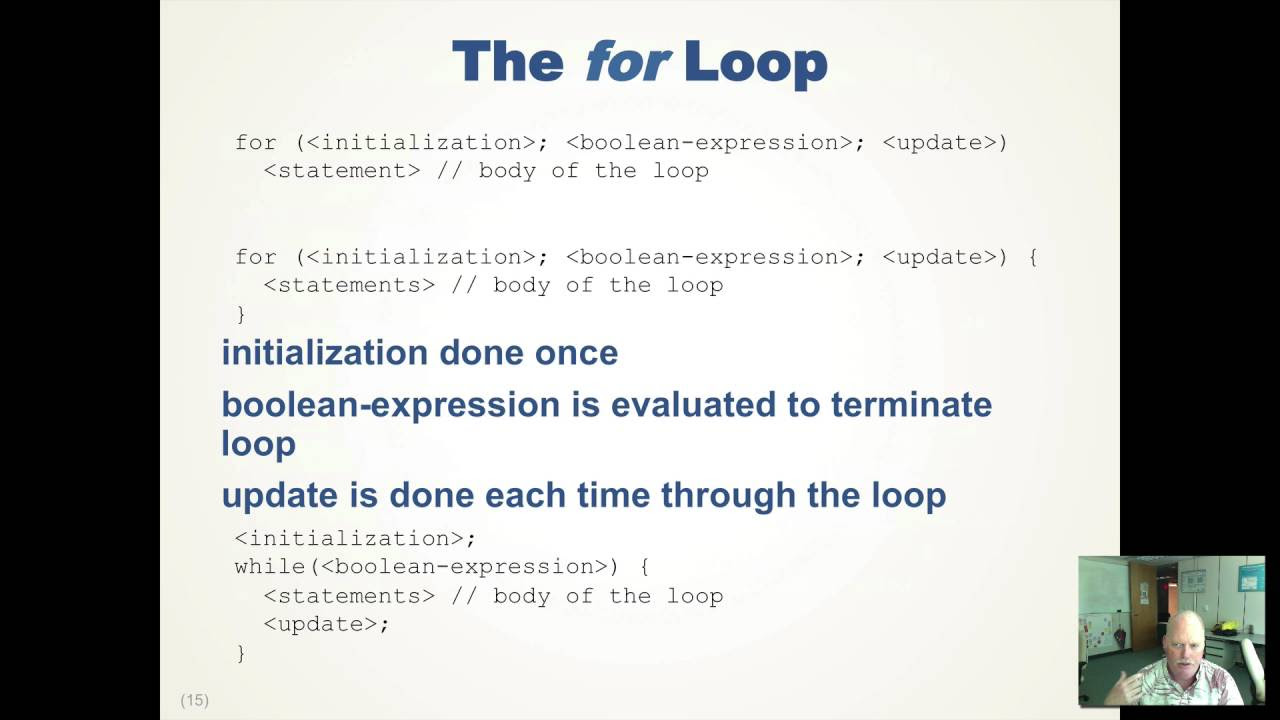
ICS 111 Midterm Review
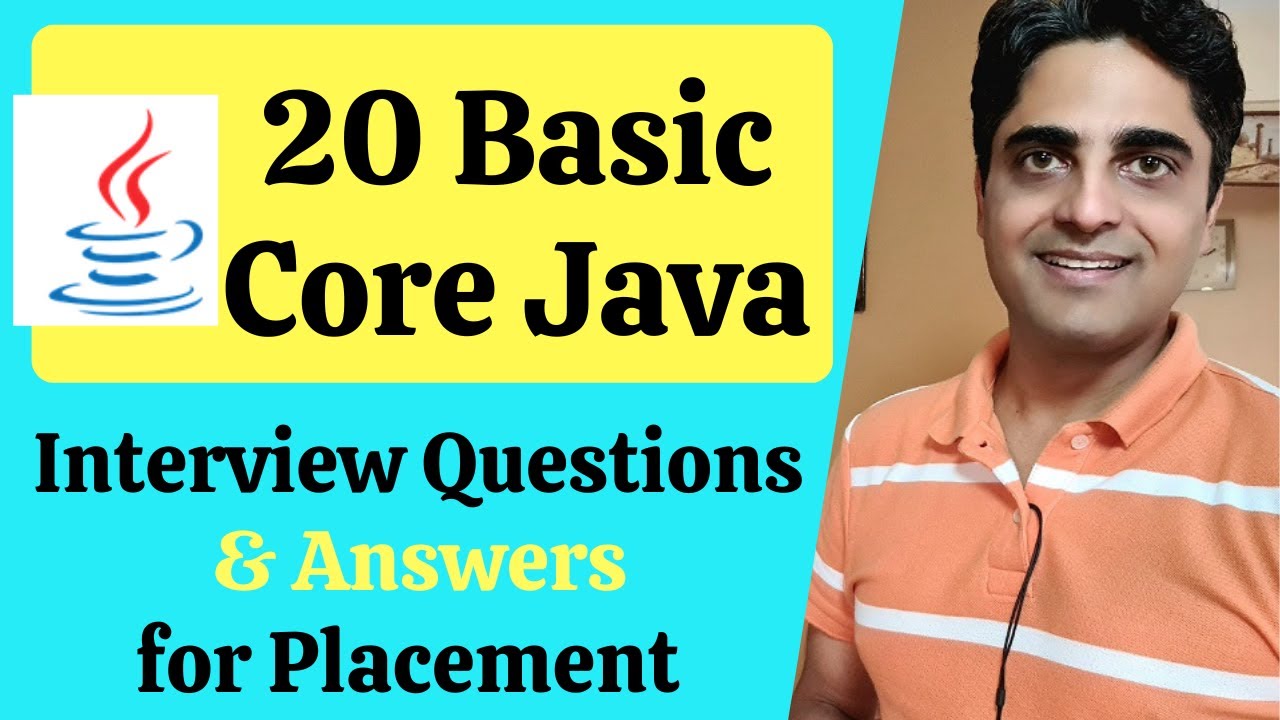
20 Basic Core Java Interview Questions & Answers- TCS, Accenture, Cognizant, Infosys, Wipro, HCL
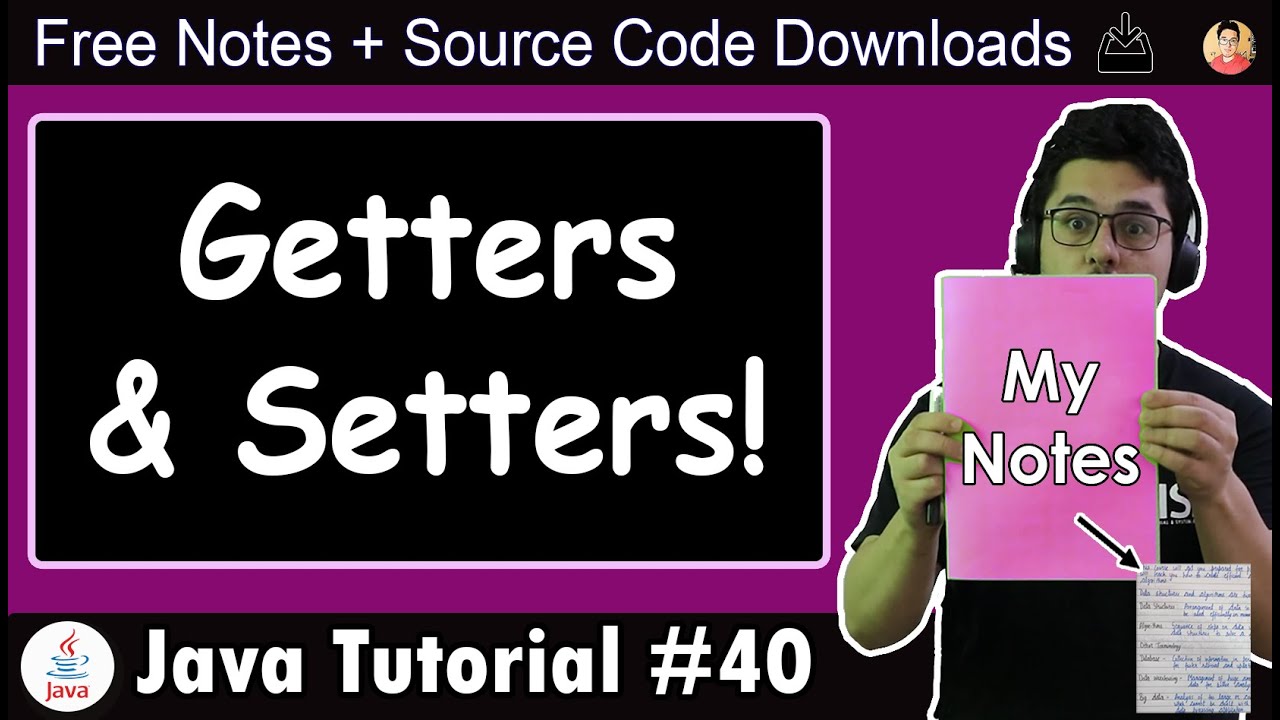
Java Tutorial: Access modifiers, getters & setters in Java
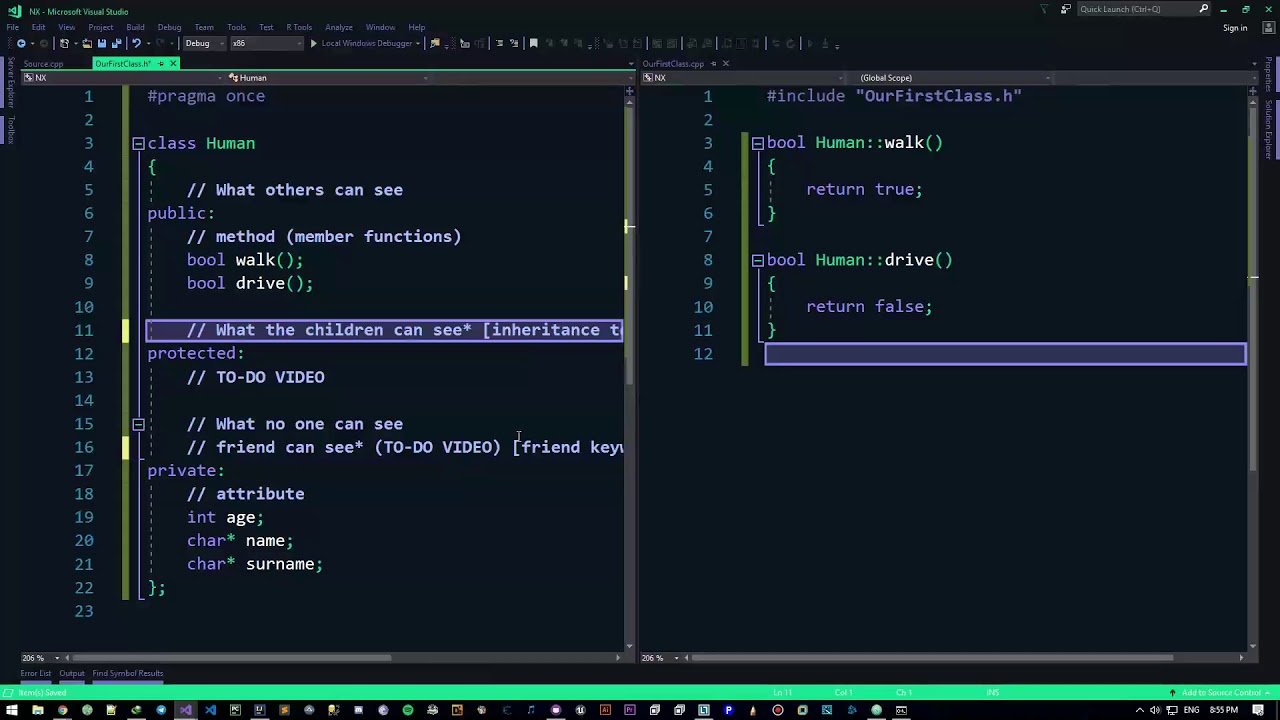
01 - [Classes] C++ Intermediate Programming Tutorial

C# Classes Tutorial | Mosh
5.0 / 5 (0 votes)