Formatting Output - fixed, showpoint, setprecision
Summary
TLDRThis video demonstrates the use of format manipulators in a simple C++ program. It begins with displaying values of two variables, x and y, then introduces the iomanip library for formatting. The 'fixed' and 'showpoint' manipulators ensure decimal points are always shown. 'Setprecision' is used to control the number of decimal places displayed, which is demonstrated by adjusting precision levels. The video also shows that 'setprecision' affects floating-point values but not integers. The explanation is clear and includes running the program to illustrate the effects of these format manipulators.
Takeaways
- 📚 The script demonstrates the use of format manipulators in a simple program.
- 🔢 Two variables, x and y, are defined with values 123.456 and 987.654, respectively.
- 💻 The program displays the values of x and y using cout.
- 📘 To use format manipulators, the iomanip library is included in the program.
- 🔧 Adding 'fixed' and 'showpoint' ensures values are displayed in fixed notation with decimal points.
- 🔍 'setprecision' is used to control the number of decimal places displayed.
- ✏️ Setting precision to 2 rounds the value of x to 123.46 and y remains 987.65.
- 🔄 Precision can be changed at any point in the program.
- 🔢 Setting precision to 1 rounds x to 123.5 and y to 987.7.
- ➖ Setting precision to 0 displays the decimal point without any decimal places.
- 🔢 Adding an integer variable z with a value of 10 shows that 'setprecision' does not affect integers.
Q & A
What are the variables used in the example program?
-The variables used in the example program are x and y.
What does the program initially display?
-The program initially displays the value of x and y, which are 123.456 and 987.654 respectively.
What library needs to be included to use format manipulators?
-The IO manip library needs to be included to use format manipulators.
Why is the 'fixed' manipulator used in the program?
-The 'fixed' manipulator is used to ensure that the values are displayed in fixed notation, avoiding exponential notation.
What is the purpose of the 'showpoint' manipulator?
-The 'showpoint' manipulator ensures that the decimal point is always displayed, even for values like 4.0.
How does 'setprecision' affect the output?
-'setprecision' sets the number of decimal places for the output. For example, setting precision to 2 will display two places after the decimal.
What happens when the precision is set to 2 for the initial values of x and y?
-When the precision is set to 2, the value of x is rounded to 123.46 and the value of y is displayed as 987.65.
Can the precision be changed at different points in the program?
-Yes, the precision can be changed at different points in the program to affect subsequent outputs.
What is the output when the precision is set to 1 for y?
-When the precision is set to 1 for y, it is displayed as 987.7.
Does 'setprecision' affect integer values?
-'setprecision' does not affect integer values; it only works with floating-point values.
What is the value of the integer variable z in the program?
-The value of the integer variable z in the program is 10.
Outlines
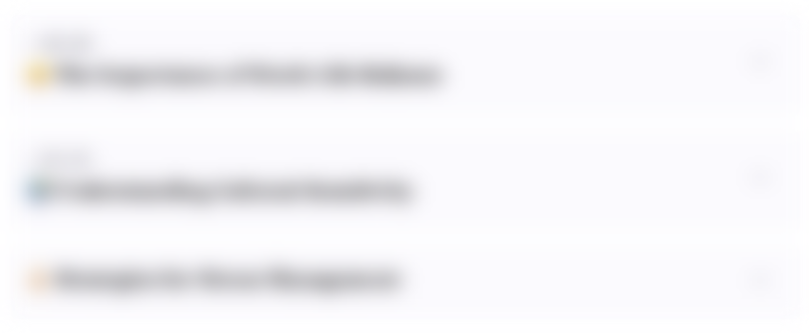
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
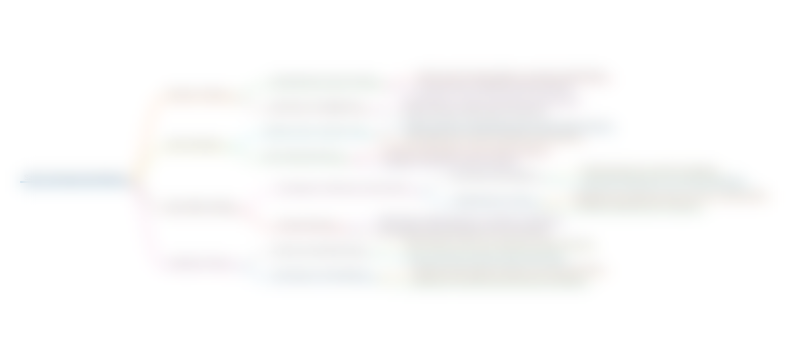
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
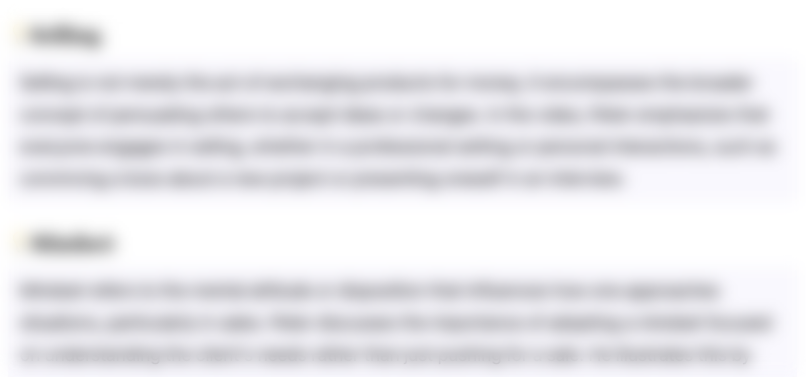
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
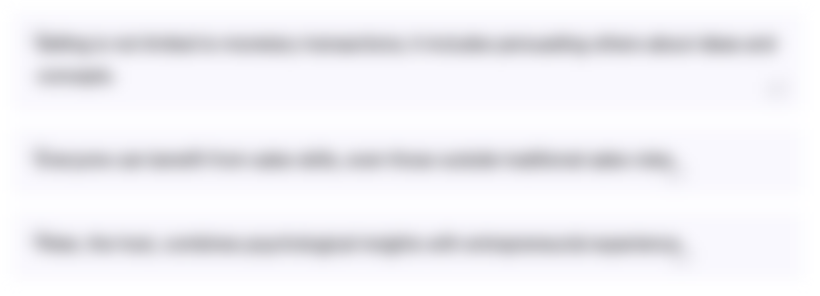
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
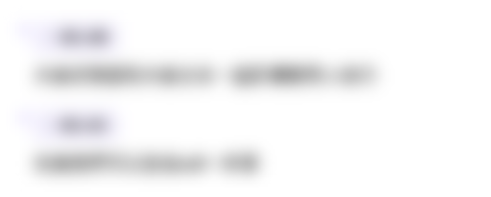
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

🚨🎯 TryHackMe Flag Vault 2 | Format String Vulnerability Exploitation | Hackfinity CTF 🚨🎯

02 - Programação em linguagem C - Criando o primeiro programa
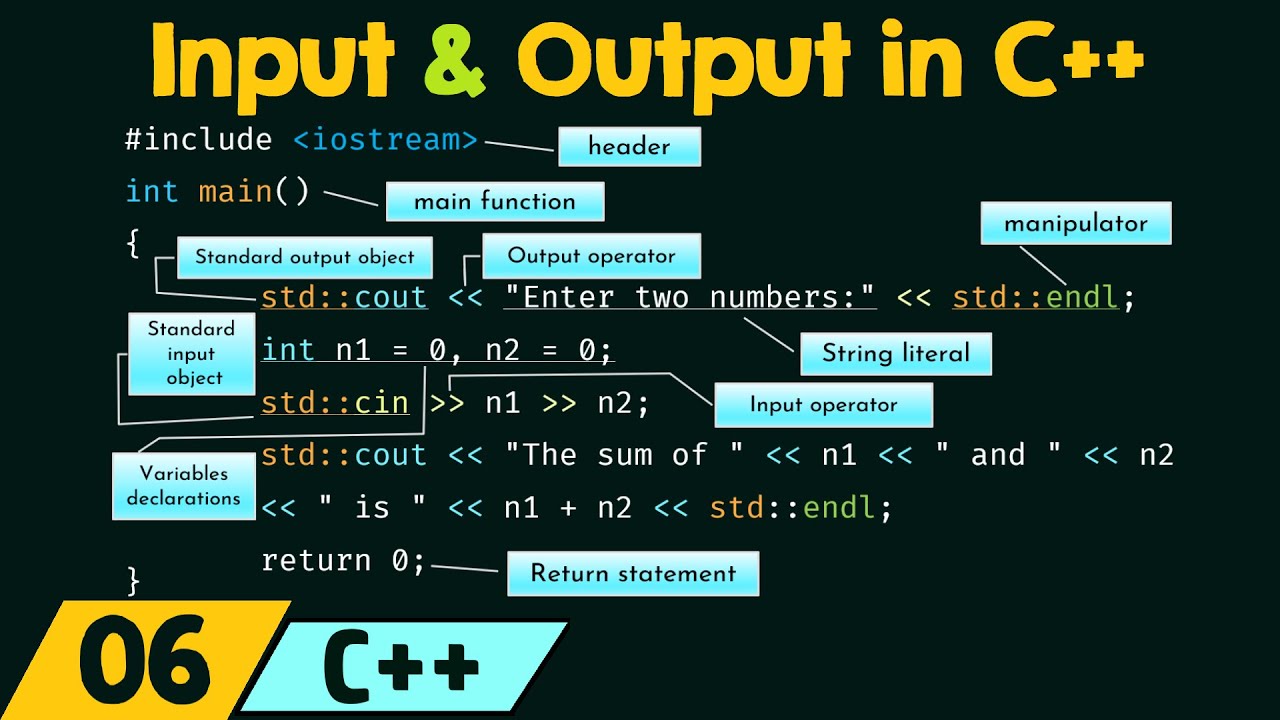
Input and Output in C++
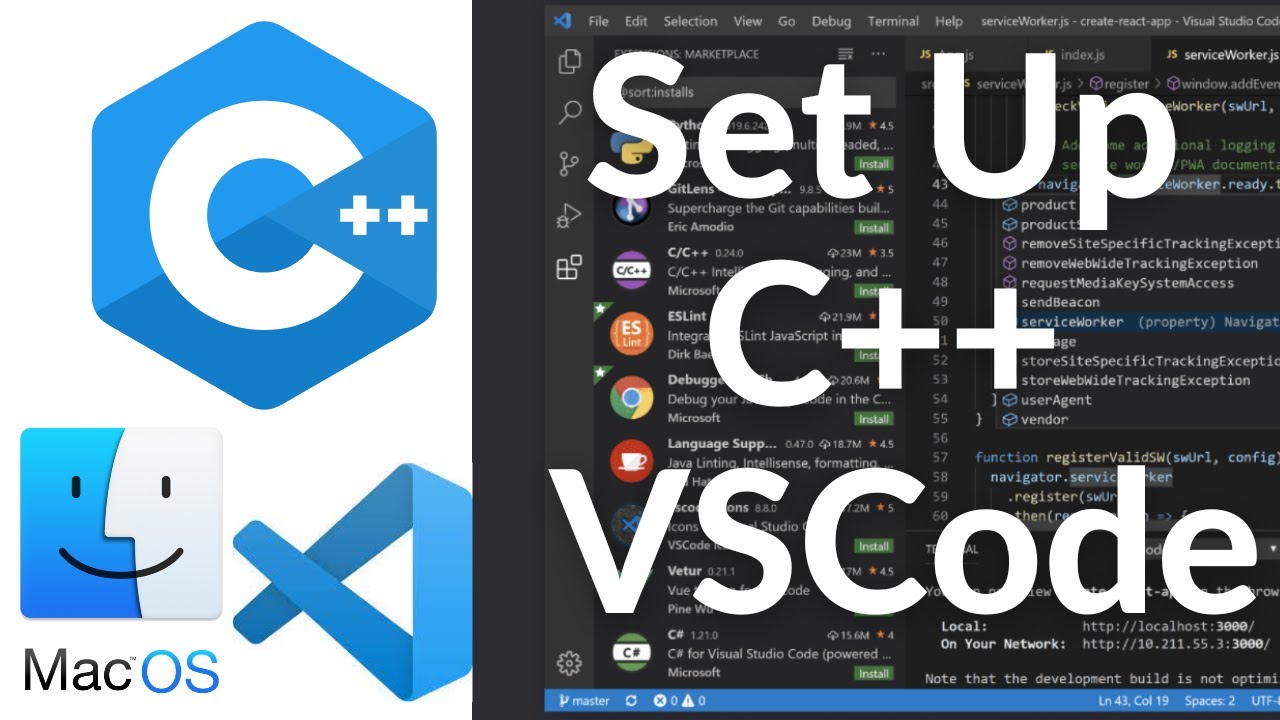
Set Up C++ Development With Visual Studio Code on Mac | VSCode C++ Development Basics MacOS (2024)
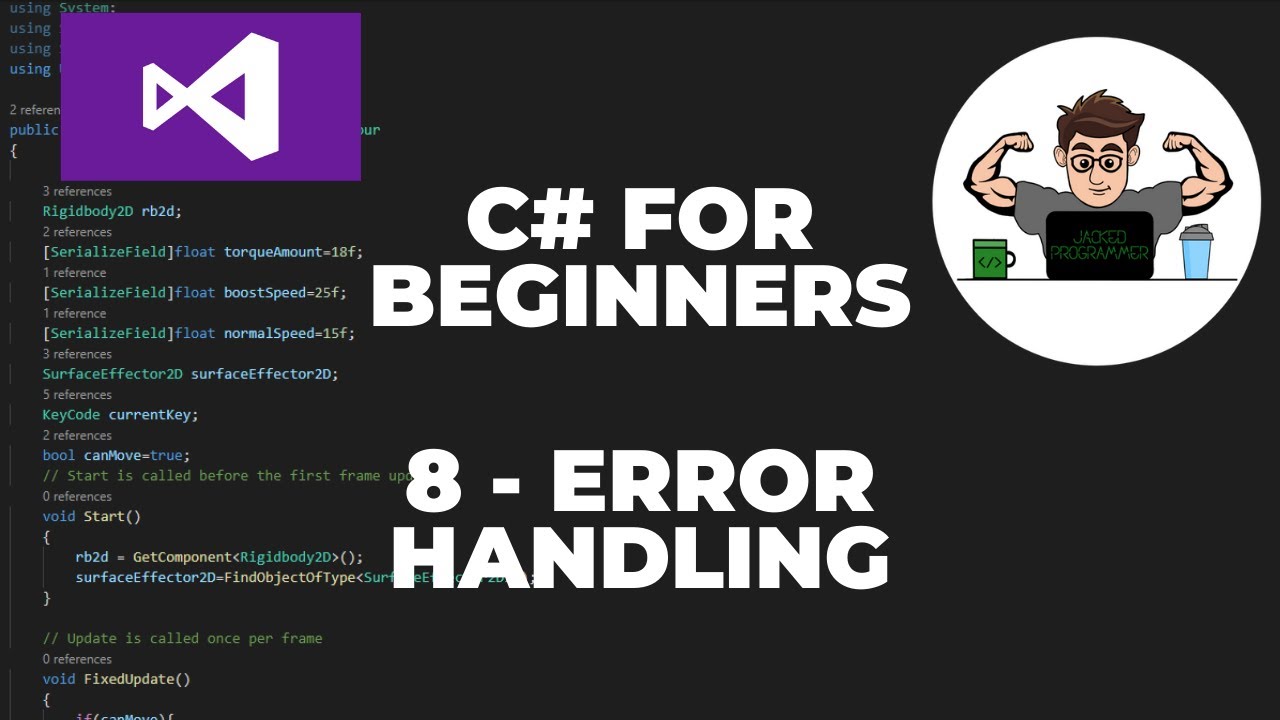
C# Tutorial for Beginners 8 : Error handling (Try Catch Finally)
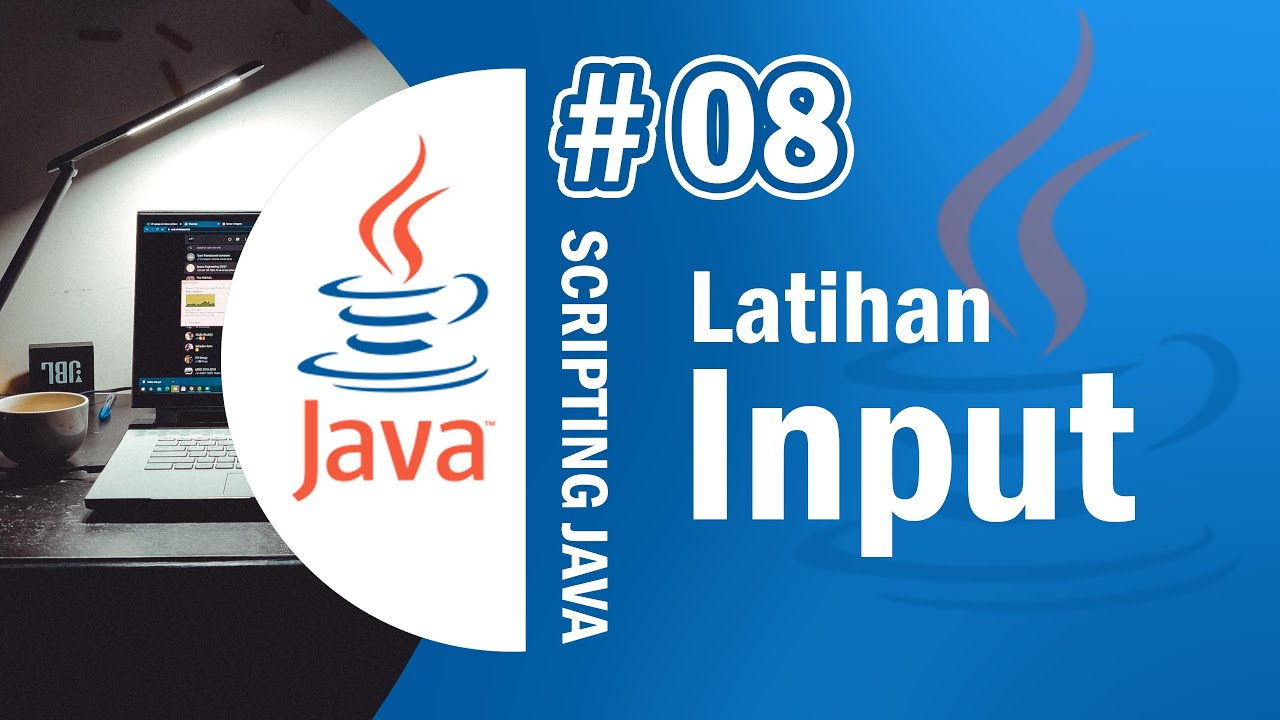
Java 08 - Latihan Input (Membuat Program Sederhana dengan Java) - Tutorial Java Netbeans Indonesia
5.0 / 5 (0 votes)