Lexical Analyzer | Lexical Analysis | Compiler Design
Summary
TLDRThe provided script discusses the role of the lexical analyzer, the first phase of a compiler, in detail. It explains how the lexical analyzer, also known as the scanner, scans the source program and converts it into meaningful tokens such as identifiers, keywords, operators, and constants. The script further delves into the process of syntax analysis, error handling, and the importance of the lexical analyzer in providing error messages with line and column numbers, which is crucial for debugging. It also touches upon the concept of semantic analysis and type checking in the context of a compiler.
Takeaways
- π The Lexical Analyzer, also known as the Scanner, is the first phase of the compiler which divides the given program into meaningful words called tokens.
- π The Lexical Analyzer scans the program and converts it into tokens such as identifiers, keywords, operators, constants, and special symbols.
- π Identifiers can be variable names and keywords are predefined words in a programming language like 'if', 'else', 'while', 'for', etc.
- β The Lexical Analyzer eliminates white space characters like blank spaces and tabs, which are not significant in the program.
- π The script discusses the importance of the Lexical Analyzer in providing error messages with line and column numbers, which help in debugging.
- π The process of the Lexical Analyzer involves accessing the symbol table to understand the context of tokens and identifiers within the program.
- π The script mentions the iterative process of the compiler, where each phase like the parser and semantic analyzer builds upon the tokens provided by the Lexical Analyzer.
- π The Parser, as part of the compiler, constructs a syntax tree based on the tokens received from the Lexical Analyzer and defined grammar rules.
- π The Semantic Analyzer checks for type matching and other semantic rules after the syntax tree is constructed by the Parser.
- π οΈ The script explains the interaction between the Lexical Analyzer, Parser, and Semantic Analyzer in the context of compiling a program.
- β οΈ The Compiler does not stop at syntax errors; it scans the entire program and lists all errors, which is crucial for understanding the compilation process.
Q & A
What is the role of a Lexical Analyzer in a compiler?
-The Lexical Analyzer, also known as the scanner, is the first phase of a compiler. It reads the source code and converts it into meaningful tokens, which are the smallest units of the programming language that carry meaning.
What are tokens in the context of lexical analysis?
-Tokens are the meaningful sequences of characters identified by the lexical analyzer. They can represent identifiers, keywords, symbols, operators, and constants from the source code.
How does the Lexical Analyzer process the input program?
-The Lexical Analyzer scans the input program character by character, groups them into tokens, and eliminates whitespace characters such as spaces and tabs.
What are the different types of tokens that a Lexical Analyzer can generate?
-The types of tokens include identifiers, keywords, operators, constants (like numeric values), and special symbols (like commas and semicolons).
What is the purpose of the Syntax Analyzer in a compiler?
-The Syntax Analyzer, also known as the parser, checks the sequence of tokens to ensure they follow the grammar rules of the programming language, constructing a parse tree or syntax tree in the process.
How does the Syntax Analyzer interact with the Lexical Analyzer?
-The Syntax Analyzer requests tokens from the Lexical Analyzer using a function like 'Get Token'. Based on the current context and the token received, the Syntax Analyzer decides which production rule to apply.
What happens if there is a mismatch between expected and actual tokens during syntax analysis?
-If a mismatch occurs, it indicates a syntax error. The compiler typically has an error handler that records the error, provides information about the location of the error, and may continue parsing the rest of the program.
Why is it important for the Lexical Analyzer to provide line and column information for errors?
-Line and column information is crucial for developers as it helps them locate and fix syntax errors in the source code more easily.
Can you explain the implicit type conversion that the Semantic Analyzer checks for?
-Implicit type conversion is when the compiler automatically converts one data type to another without explicit programmer instruction. The Semantic Analyzer checks for situations like assigning a smaller integer value to a floating-point variable, which can be done without data loss.
What is the role of the Semantic Analyzer in the compilation process?
-The Semantic Analyzer is responsible for checking the semantic validity of the program, including type checking, scope resolution, and other semantic rules defined by the programming language.
How does the compiler handle syntax errors during the compilation process?
-The compiler does not stop the compilation process upon encountering a syntax error. Instead, it records the error, provides details about the location, and continues to scan and analyze the rest of the program to identify additional errors.
Outlines
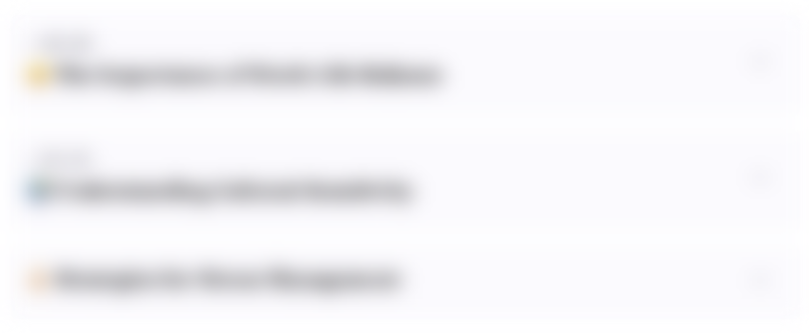
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
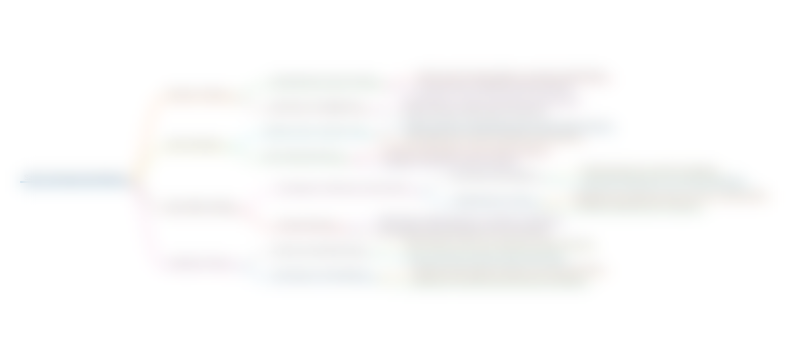
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
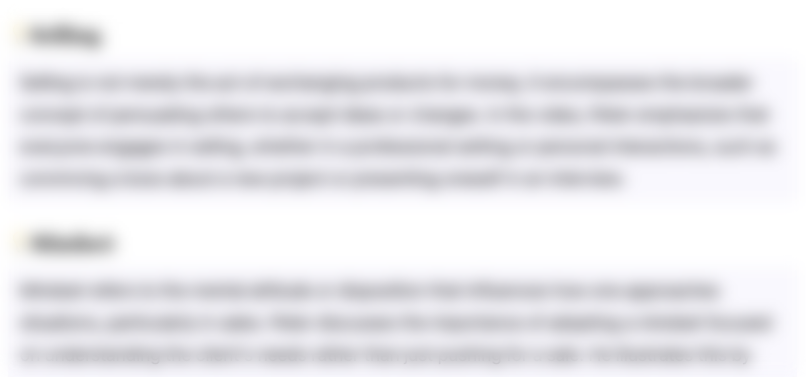
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
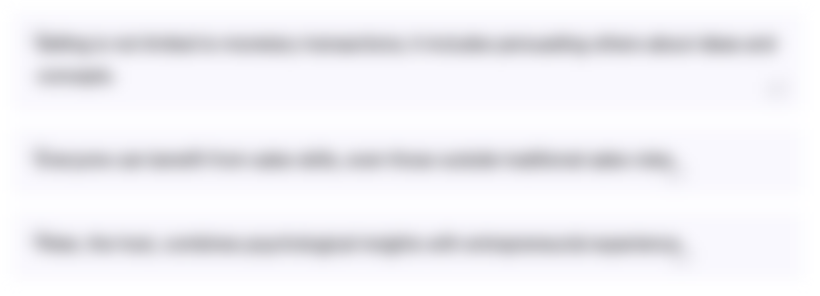
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
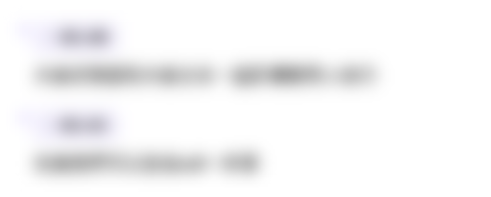
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)