Python Lambda Function - 19 | Lambda Function In Python Explained | Python Tutorial | Simplilearn
Summary
TLDRIn this Python tutorial, the presenter introduces lambda functions, emphasizing their simplicity and usefulness for defining anonymous functions. The video covers the syntax of lambda functions, provides examples of their application (such as adding numbers, using lambda in filtering, mapping, and reducing operations), and explores their pros and cons. It also demonstrates how lambda functions can be combined with user-defined functions and higher-order functions. With practical examples, viewers learn how to use lambda functions for different purposes, from mathematical operations to string manipulations, making the tutorial a valuable resource for Python enthusiasts.
Takeaways
- π Lambda functions are anonymous functions in Python, defined using the 'lambda' keyword and can have any number of arguments but only one expression.
- π The syntax for a lambda function includes the 'lambda' keyword, followed by arguments, a colon, and the expression to evaluate.
- π Lambda functions can simplify tasks by reducing multiple lines of code into a single line, making the code more concise.
- π Unlike regular functions defined using the 'def' keyword, lambda functions don't require a function name and are ideal for short, single-expression functions.
- π Lambda functions can be passed as arguments to higher-order functions such as map, filter, and reduce.
- π You can create lambda functions that work with default arguments, keyword arguments, and even variable numbers of arguments using *args.
- π Higher-order functions are functions that take other functions as arguments or return functions. Lambda functions are often used in these cases.
- π Lambda functions are useful for operations like adding or multiplying numbers, filtering lists, or applying transformations to data in one line of code.
- π The 'filter' function can be used with lambda to filter out elements from a list based on a specific condition, such as selecting numbers greater than 30.
- π The 'map' function, when used with lambda, applies the lambda function to each element in a list, returning a new list with modified values.
- π Lambda functions can be used within user-defined functions, such as calculating the value of a quadratic equation in a single line of code.
Q & A
What is a lambda function in Python?
-A lambda function in Python is an anonymous function defined without a name. It is created using the 'lambda' keyword and can accept any number of arguments, but it can only have one expression. It is used for short and simple operations.
How does a lambda function differ from a regular function in Python?
-A lambda function is defined using the 'lambda' keyword and does not require the 'def' keyword. It is anonymous (without a name) and can only have a single expression, unlike a regular function that can have multiple expressions and use the 'def' keyword.
What are the pros of using lambda functions?
-Lambda functions are suitable for simple logical operations where only one expression is needed. They are often used as parameters in higher-order functions like map, filter, and reduce. They also allow for compact and concise code.
What are the cons of using lambda functions?
-The main disadvantage of lambda functions is that they can only evaluate a single expression, limiting their functionality. They are also less readable and harder to debug when compared to regular functions, especially if more complex operations are needed.
Can you assign a lambda function to a variable?
-Yes, you can assign a lambda function to a variable. This allows you to reuse the lambda function by calling the variable, as demonstrated with the 'add' variable in the script.
What is an immediately invoked function expression (IIFE) in Python?
-An immediately invoked function expression (IIFE) is a function that is defined and called immediately in a single expression. In Python, this can be done with a lambda function, where the function is defined and called in one line without assigning it to a variable.
How do you use lambda functions with higher-order functions?
-Lambda functions can be used with higher-order functions like map, filter, and reduce. These functions accept another function (like a lambda) as an argument and apply it to elements in a list or iterable. For example, lambda functions can filter numbers greater than a certain value or map values by applying a transformation.
What is the difference between the map and filter functions in Python?
-The 'map' function applies a given function (like a lambda) to every item in an iterable and returns a new iterable with the modified items. The 'filter' function, on the other hand, filters elements from an iterable based on a condition and returns only the elements that satisfy the condition.
How can you pass default arguments to a lambda function?
-You can pass default arguments to a lambda function by specifying default values for the parameters. For example, in the script, 'lambda x, y=15, z=24: x + y + z' defines default values for 'y' and 'z'. If you do not pass values for 'y' and 'z', the default values will be used.
What is the reduce function, and how is it used with lambda?
-The 'reduce' function from the 'functools' module applies a rolling calculation to a list, reducing it to a single result. When used with lambda, it can perform operations like summing or multiplying all elements of a list. For example, 'reduce(lambda x, y: x + y, list)' sums all elements in 'list'.
Outlines
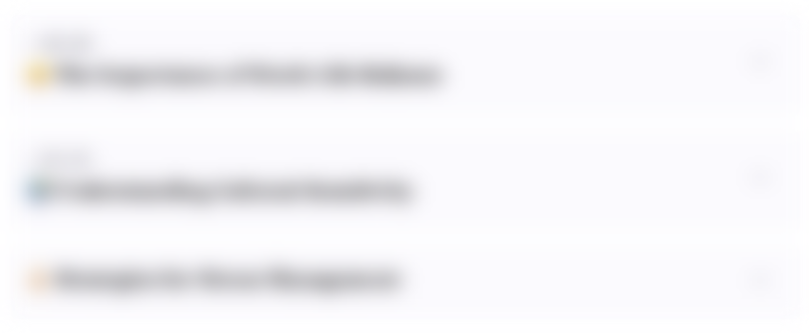
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
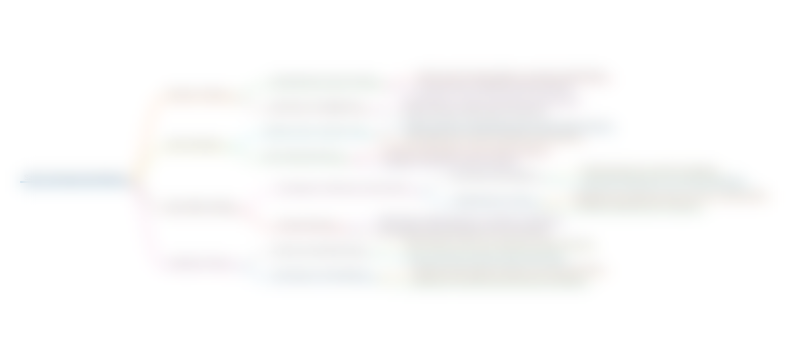
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
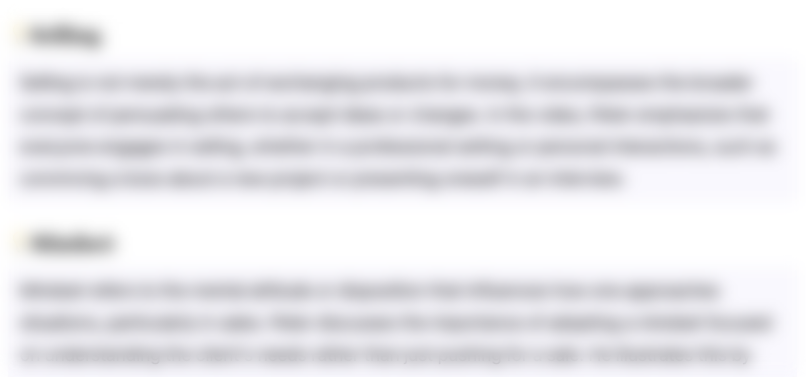
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
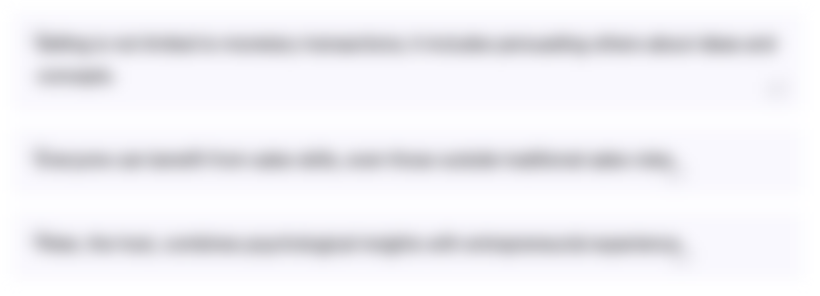
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
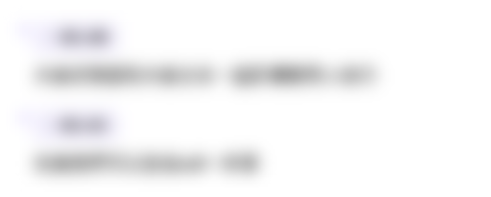
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)