Coding Challenge #50.1: Animated Circle Packing - Part 1
Summary
TLDRIn this coding challenge, Dan introduces a creative project using Processing, a Java-based programming environment, to explore animated circle packing. He demonstrates how to create a 'Circle' class and use an ArrayList to manage multiple circles. The challenge involves growing circles that stop expanding upon touching the window edges or overlapping with others. Dan also hints at future enhancements, such as using a source image to guide circle placement for forming letter shapes and dynamically generating letter paths for personalized animations.
Takeaways
- ๐ The video is a coding challenge focused on animated circle packing to form letter forms using the programming environment Processing, which is built on top of Java.
- ๐ The presenter, Dan, plans to release the source code in both Processing Java and JavaScript using the p5.js library for those interested in the project.
- ๐จ The inspiration for the project comes from the work of artist Marius Watts, who is known for engaging computational art involving circle packing.
- ๐ The fundamental concept of circle packing involves fitting as many circles of varying sizes into a space without overlapping.
- ๐พ Dan introduces a 'Circle' class with properties for x and y coordinates for the center and a radius, and methods for displaying and growing the circles.
- ๐ ๏ธ The coding process includes creating a constructor for the circle class, handling edge cases like circles touching the window edges, and managing a list of circles using an ArrayList in Java.
- ๐ The video demonstrates the iterative growth of circles and the logic to stop their growth when they reach the window edge or overlap with other circles.
- ๐ A key feature implemented is the dynamic creation of new circles at random locations, ensuring they do not overlap with existing circles.
- ๐ Dan discusses the possibility of refining the algorithm to handle pixel width and overlap more gracefully, adjusting the growth rate for better resolution.
- ๐ผ๏ธ The video concludes with an advanced technique of using a source image to determine the initial placement of circles, specifically using white pixels as starting points.
- ๐ฎ Future considerations mentioned include dynamically computing letter paths for circle packing, allowing users to input text that is then filled with circles.
Q & A
What is the main focus of this coding challenge?
-The main focus of this coding challenge is to create an animated circle packing algorithm that forms letter shapes.
Which programming environment is being used in this challenge?
-The challenge uses Processing, a programming environment built on top of Java.
What is the purpose of the Circle class in this code?
-The Circle class is used to define the properties and behaviors of a circle, including its x and y coordinates and radius.
How does the code ensure circles do not overlap?
-The code checks the distance between each new circle and existing circles. If the distance is less than the sum of their radii, it stops the new circle from growing.
What is the function of the ArrayList 'circles'?
-The ArrayList 'circles' is used to keep track of all the circles created and manage their growth and display.
How does the grow function work?
-The grow function increases the radius of the circle by one pixel each time it is called, unless the circle touches an edge or another circle.
What method is used to detect if a circle has touched an edge of the window?
-The edges method checks if the circle's x or y coordinate plus its radius is greater than the window width or height, or if it's less than zero.
How does the code add new circles to the canvas?
-The code uses a random location generator to place new circles, ensuring they do not overlap with existing circles by checking their distances.
What is the role of the spots ArrayList?
-The spots ArrayList stores potential starting points for new circles, based on the white pixels in a source image.
How does the script handle the completion of the circle packing process?
-The script includes a counter and an attempt limit to stop the loop if it can't find new spots for circles, printing 'finished' when done.
Outlines
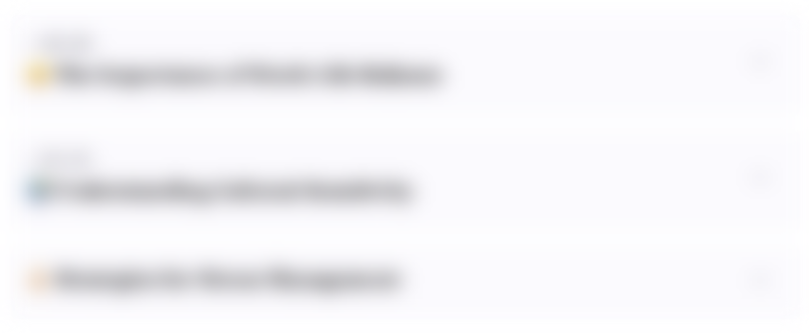
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
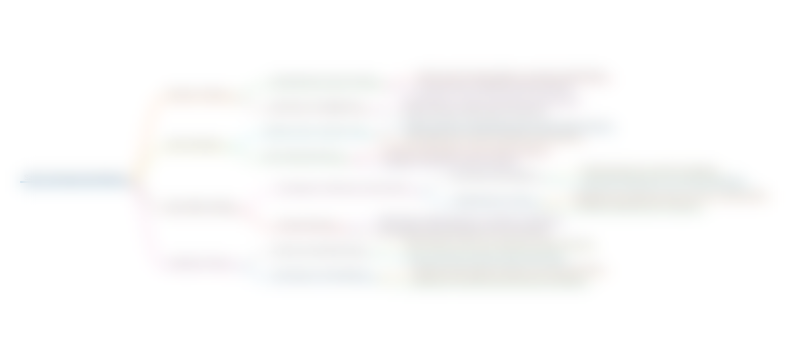
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
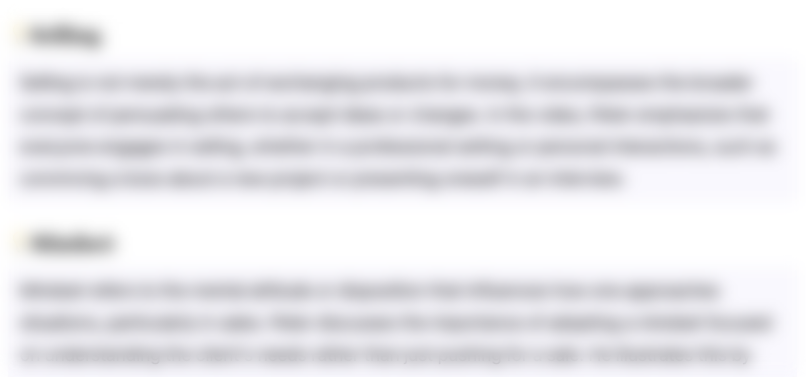
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
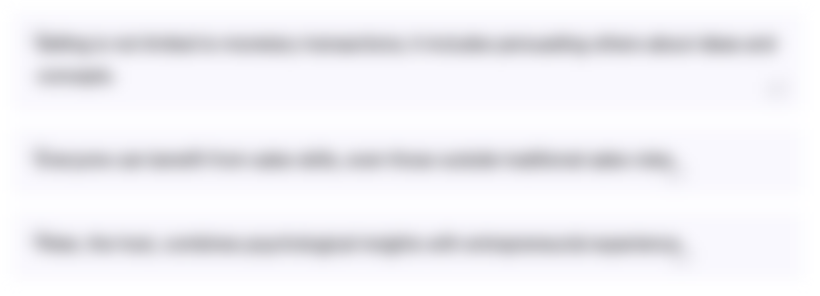
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
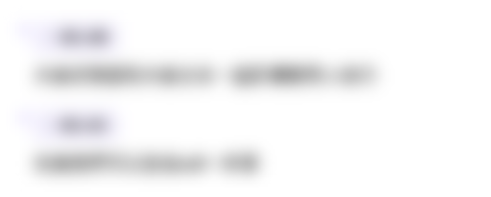
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
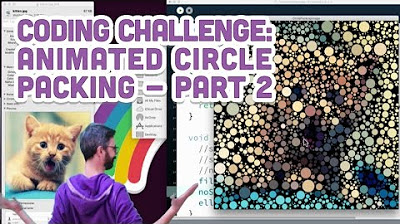
Coding Challenge #50.2: Animated Circle Packing - Part 2 (Kitten Addendum)
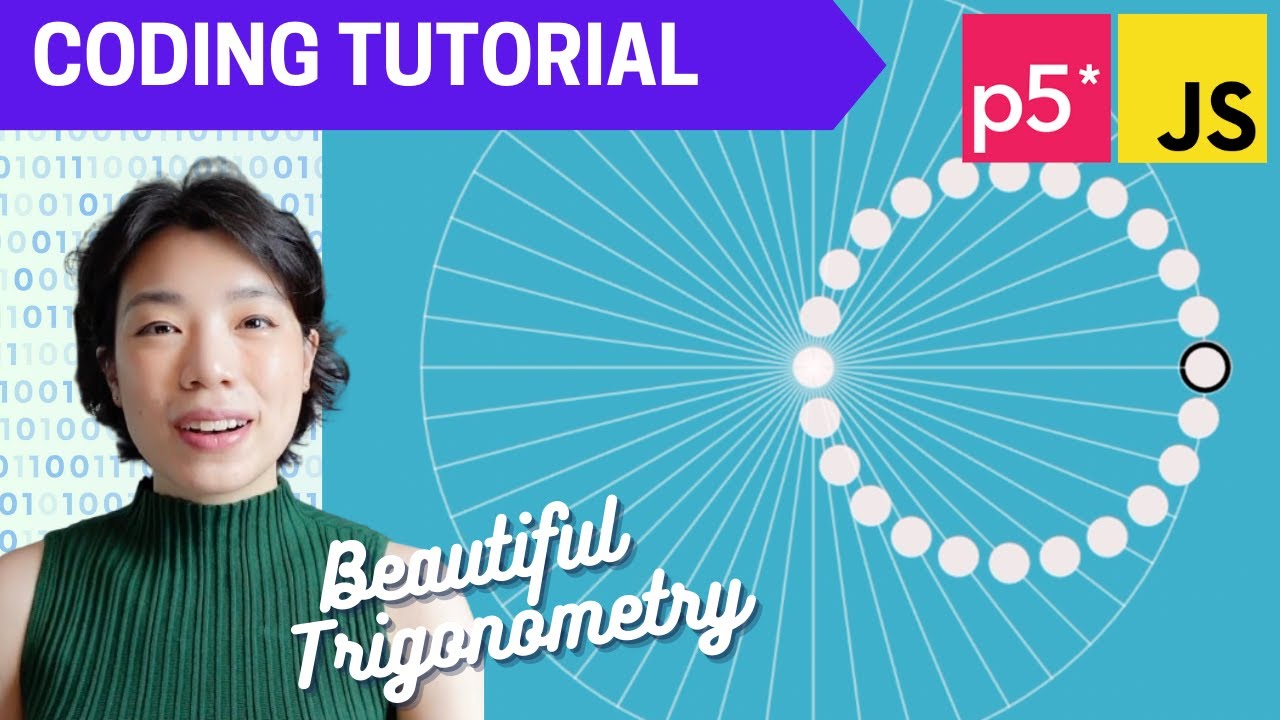
p5.js Coding Tutorial | The Making of Animation - Beautiful Trigonometry
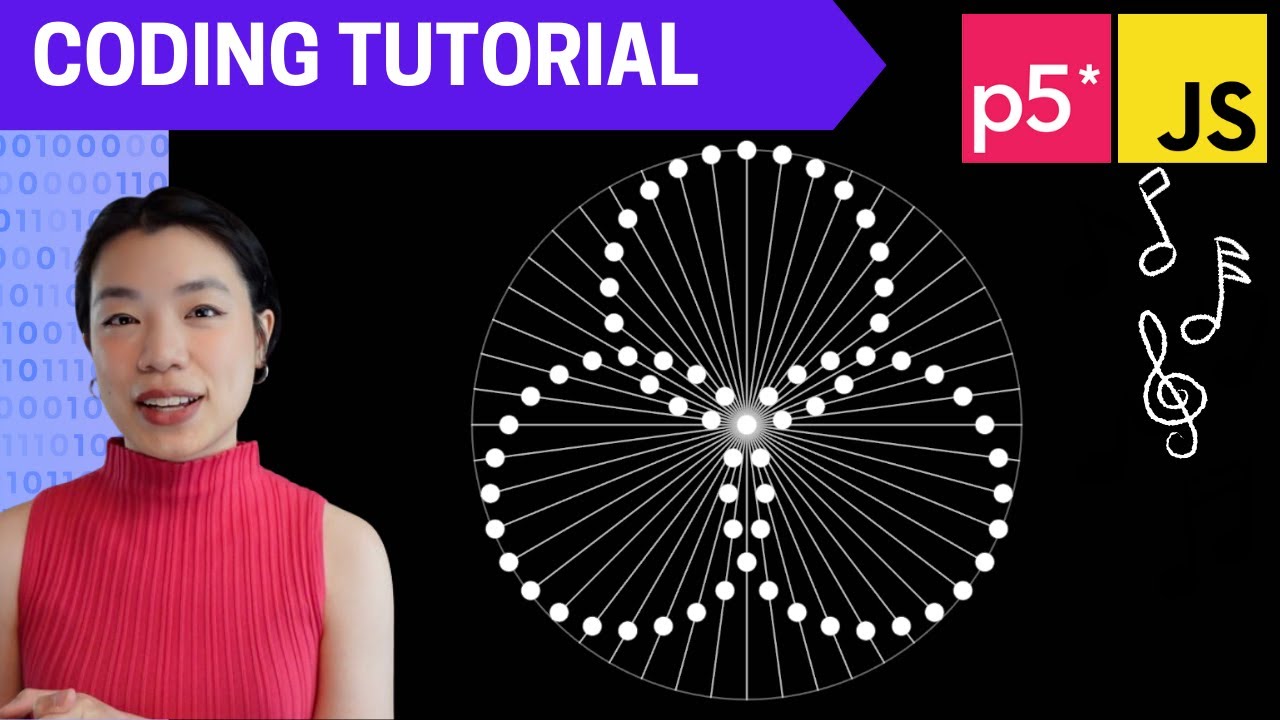
p5.js Coding Tutorial | Optical Illusion with Sound ๐ต
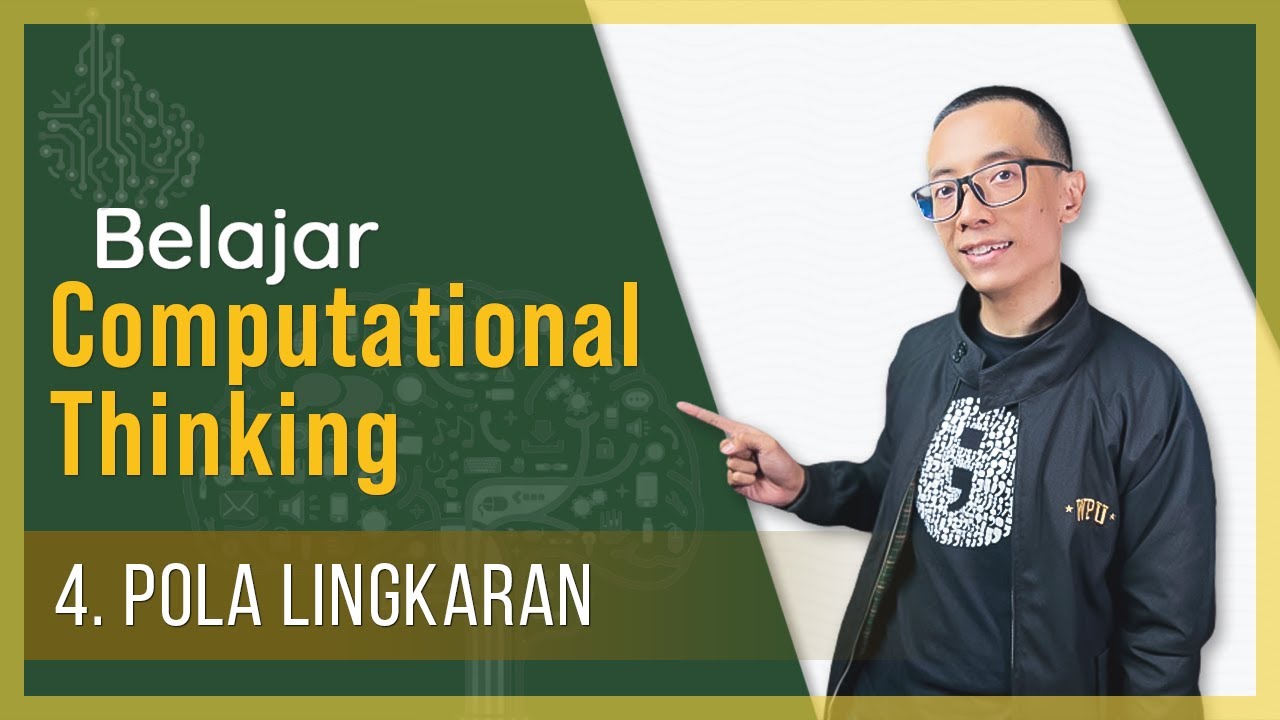
4. Pola Lingkaran | Latihan Computational Thinking

ATPL General Navigation - Class 4: Convergency.
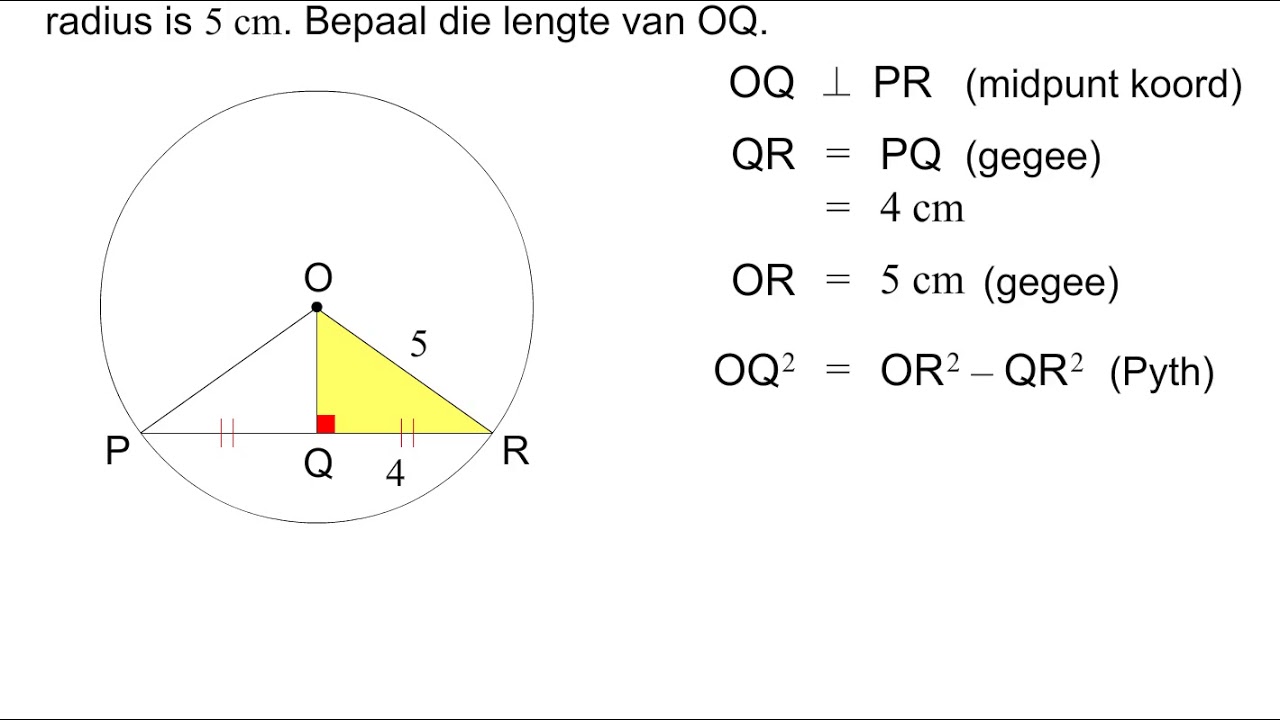
Graad 11 Meetkunde
5.0 / 5 (0 votes)