Learn JavaScript Programming Basics: Logical Operators in JavaScript
Summary
TLDRThis tutorial introduces JavaScript's logical operators, including `AND`, `OR`, and `NOT`, and demonstrates how they work with boolean values. The `AND` operator checks if all conditions are true, the `OR` operator returns true if any condition is true, and the `NOT` operator inverts boolean values. The video uses practical examples such as checking if students pass exams based on multiple conditions and testing user login credentials. These operators are essential for controlling program flow and decision-making in JavaScript, helping beginners understand logic operations in real-world scenarios.
Takeaways
- 😀 Logical operators in JavaScript are used to combine multiple conditions and return a boolean value (true or false).
- 😀 The AND (`&&`) operator returns `true` only if all conditions are true; otherwise, it returns `false`.
- 😀 The OR (`||`) operator returns `true` if at least one of the conditions is true, and `false` only if all conditions are false.
- 😀 The NOT (`!`) operator reverses the boolean value, turning `true` into `false` and vice versa.
- 😀 When using the AND operator, both conditions must be true for the result to be true.
- 😀 The OR operator checks if at least one of the conditions is true to return `true`, even if the other is false.
- 😀 The NOT operator is useful for negating conditions and inverting boolean results.
- 😀 A practical example using the AND operator checks if a student passes both theoretical and practical exams, requiring both scores to be above 70.
- 😀 The OR operator can be used to allow access based on one condition being true, like a user being logged in, even without having points.
- 😀 The NOT operator can be used to check if a user is not logged in, reversing the boolean value to show the opposite status.
Q & A
What is the purpose of logical operators in JavaScript?
-Logical operators in JavaScript are used to combine two or more conditions and return a Boolean value (`true` or `false`), helping in decision-making based on multiple conditions.
What does the `&&` (AND) operator do in JavaScript?
-The `&&` (AND) operator returns `true` if **both** conditions being evaluated are true; otherwise, it returns `false`.
Can you provide an example of how the `&&` operator works?
-For example, if `nilaiTeori > 70` and `nilaiPraktik > 70`, the expression `nilaiTeori > 70 && nilaiPraktik > 70` will return `true` if both conditions are true.
What is the `||` (OR) operator used for in JavaScript?
-The `||` (OR) operator returns `true` if **at least one** of the conditions is true. If all conditions are false, it returns `false`.
Can you explain with an example how the `||` operator works?
-For example, if `login = true` and `poin = false`, the expression `login || poin` will return `true` because at least one condition (`login`) is true.
What does the `!` (NOT) operator do in JavaScript?
-The `!` (NOT) operator inverts the Boolean value. If the value is `true`, it becomes `false`; if it is `false`, it becomes `true`.
How does the `!` (NOT) operator work in practice?
-For instance, if `sudahLogin = false`, the expression `!sudahLogin` will return `true` because it inverts the original value.
How can you use logical operators to check if both username and password match in a login system?
-You can use the `&&` operator to ensure both conditions are true, such as checking if `username === 'admin'` and `password === '1234'`. If both conditions are true, it returns `true`.
What will happen if one condition is false in a logical `&&` check?
-If one condition in a logical `&&` check is false, the whole expression will return `false` because both conditions must be true for it to return `true`.
What are some practical uses for the logical operators in JavaScript as shown in the script?
-Some practical uses include checking login status (e.g., whether the user is logged in or has sufficient points) or verifying if a student passes based on both theory and practice exam scores.
Outlines
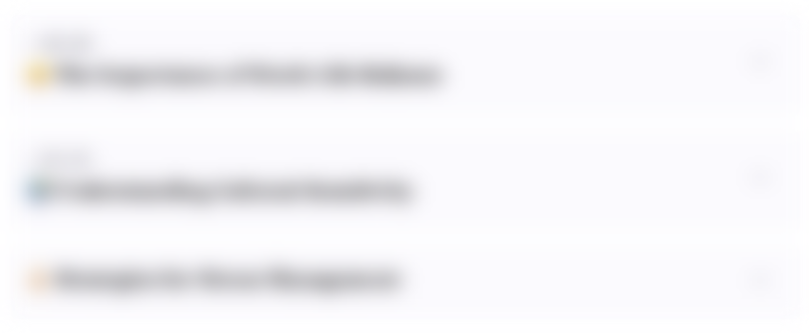
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
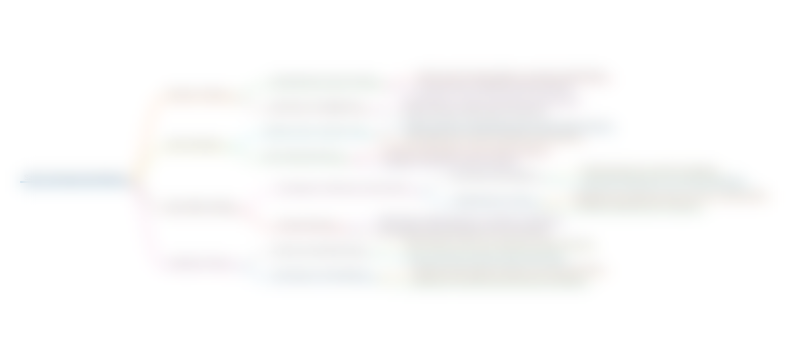
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
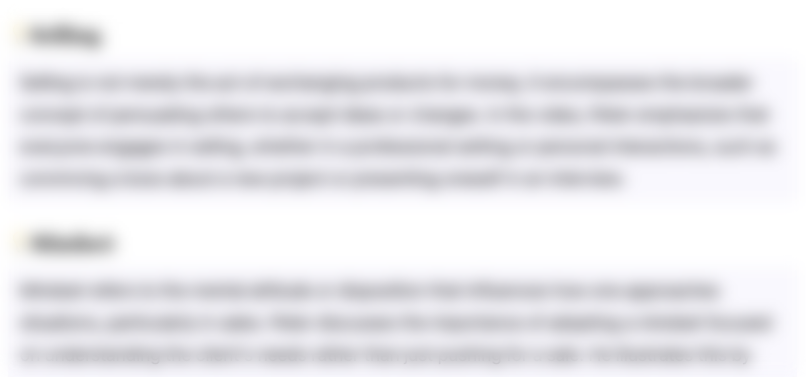
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
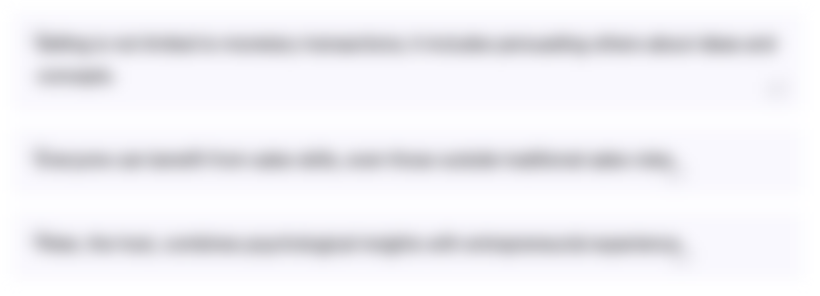
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
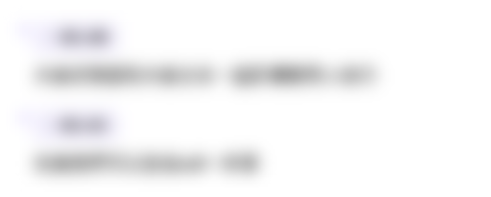
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
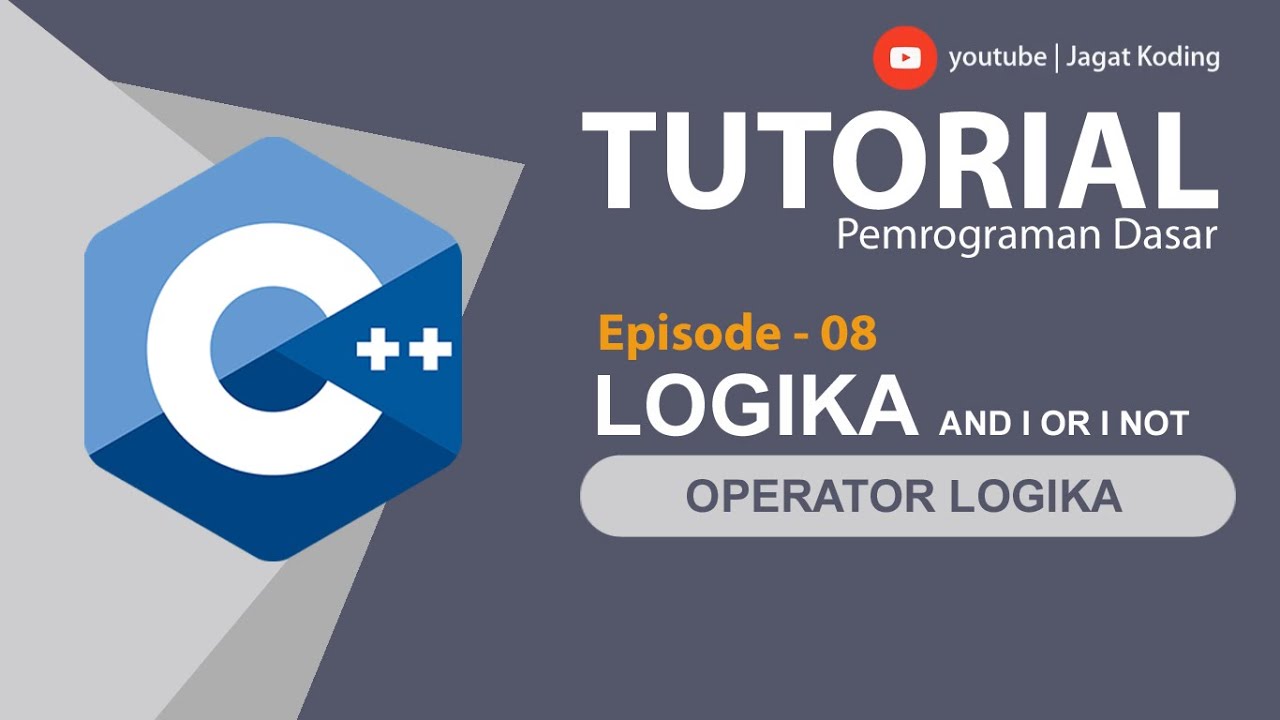
C++ 08 | Operator Logika | Tutorial Dev C++ Indonesia

Comparison, Logical, and Membership Operators in Python | Python for Beginners

#11 Python Tutorial for Beginners | Operators in Python

Tutorial 2 - Python List and Boolean Variables
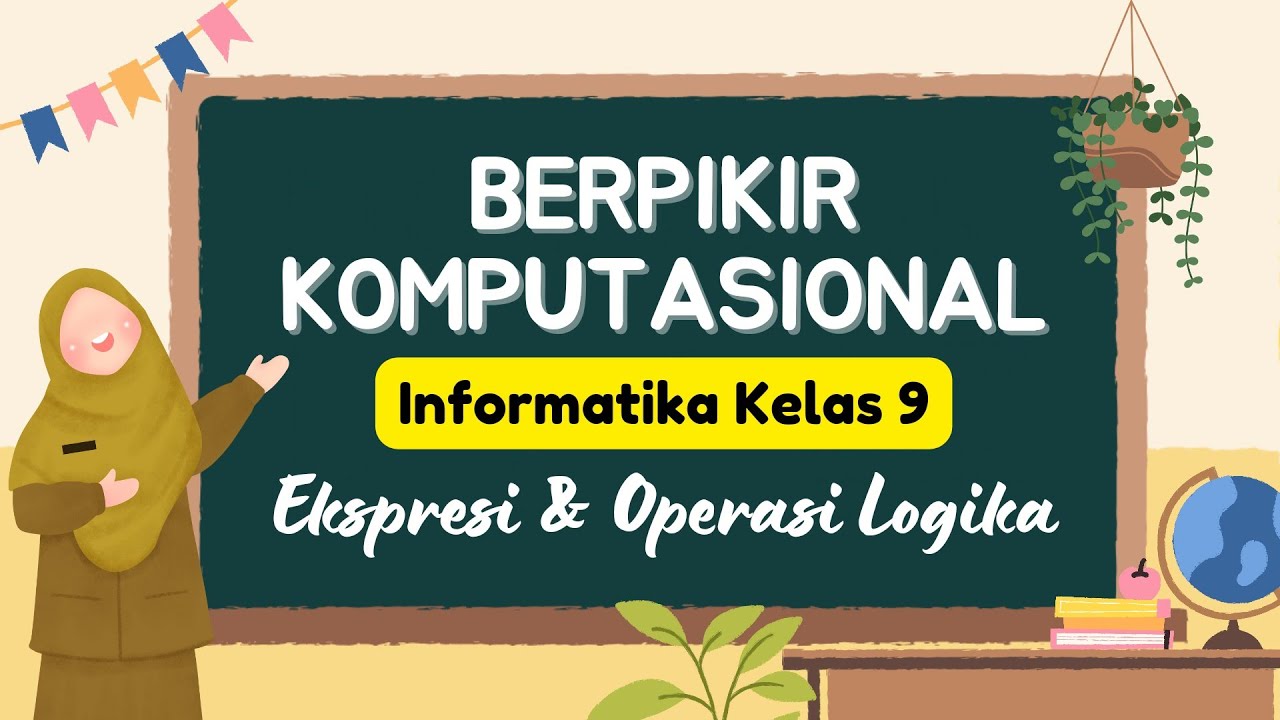
Informatika Kelas 9 Gak Ribet! Pahami Ekspresi dan Operasi Logika | Materi Berpikir Komputasional

Minggu 3 - Eksekusi Kondisional
5.0 / 5 (0 votes)