Python args and kwargs Tutorial: How to Use *args and **kwargs
Summary
TLDRIn this video, Josh breaks down the concept of `args` and `quars` in Python, explaining how they enable functions to handle variable numbers of arguments. He provides clear, step-by-step examples demonstrating how `args` allows functions to accept any number of positional arguments, while `quars` works with keyword arguments in the form of key-value pairs. The video shows how both techniques can make your code more flexible, reusable, and efficient. By the end, viewers will understand when and how to use `args` and `quars` to optimize their Python code.
Takeaways
- 😀 `args` allow you to pass a variable number of positional arguments to a function, making your code more flexible.
- 😀 The `*args` syntax is used in Python to collect positional arguments into a tuple that can be iterated over.
- 😀 By using `args`, you can avoid rewriting functions each time the number of arguments changes, increasing reusability.
- 😀 The `*args` approach simplifies the code and allows you to handle more or fewer arguments dynamically.
- 😀 The `**kwargs` syntax allows you to pass any number of keyword arguments (key-value pairs) to a function.
- 😀 `kwargs` store the arguments as a dictionary, providing access to both the keys and their associated values.
- 😀 Using `kwargs` can save space and improve code speed by avoiding the need for separate dictionaries when handling data.
- 😀 `args` and `kwargs` help optimize Python code by making it reusable, easier to manage, and more flexible.
- 😀 Standard practice is to name the variable for positional arguments `args` and for keyword arguments `kwargs`, but they can be named anything as long as the syntax with `*` or `**` is followed.
- 😀 Frameworks often handle `kwargs` internally, meaning that developers can pass key-value pairs without needing to worry about implementation details.
- 😀 Learning to use `args` and `kwargs` can significantly improve your coding efficiency by making functions adaptable to changing inputs.
Q & A
What are `args` in Python?
-`args` stands for positional arguments, allowing you to pass a variable number of arguments to a function without specifying exactly how many in advance. It’s commonly used in functions where you don’t know the exact number of parameters needed.
How do `args` improve the flexibility of functions in Python?
-`args` allow functions to accept any number of positional arguments, making them more flexible. This means that the same function can be reused in different contexts where the number of arguments may vary, reducing the need to rewrite the function for each case.
Can you name the syntax used to define `args` in a Python function?
-The syntax for defining `args` in a Python function is to use an asterisk (*) followed by a name (commonly `args`). For example, `def multiply(*args):`.
What does `args` behave like internally in Python?
-`args` behaves like a tuple. It can be iterated over and treated just like any other iterable, allowing you to process the passed arguments within a loop or other constructs.
What does the following code do? `result = 1 for number in args: result *= number`
-This code multiplies all of the arguments passed to the function. It starts with `result` set to 1 and iterates over each argument in `args`, multiplying them together to get the final result.
What are `quars` in Python?
-`quars` stands for keyword arguments. They allow a function to accept any number of key-value pairs, effectively allowing you to pass named arguments to the function in the form of a dictionary.
How do `quars` improve function flexibility in Python?
-`quars` provide the ability to pass an arbitrary number of keyword arguments to a function. This allows the function to handle different inputs without explicitly defining each parameter. It also reduces code duplication and enhances readability.
What is the syntax for defining `quars` in a Python function?
-The syntax for defining `quars` in a Python function is to use double asterisks (**) followed by a name (commonly `quars`). For example, `def print_user_info(**quars):`.
How do keyword arguments differ from positional arguments in Python?
-Positional arguments (like those in `args`) are passed based on their position in the function call, while keyword arguments (like those in `quars`) are passed with a specific key-value pair, where the key is the argument name and the value is the argument itself.
What happens if you mix `args` and `quars` in a single function?
-When you mix `args` and `quars`, the function can accept both positional arguments (as a tuple) and keyword arguments (as a dictionary). This allows for greater flexibility, letting you pass different types of arguments to a single function.
Outlines
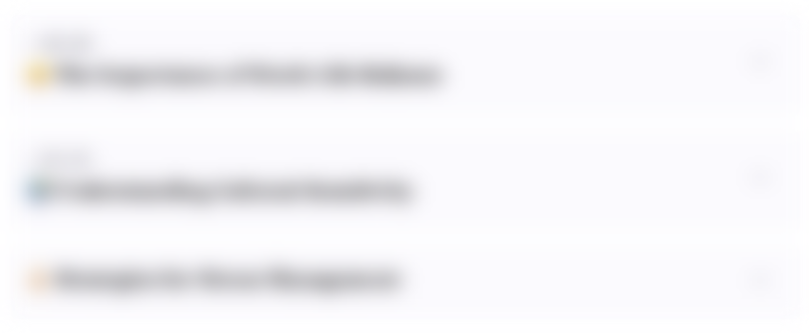
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
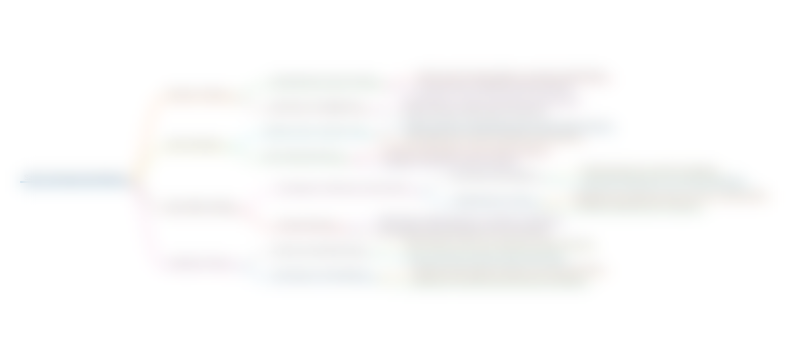
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
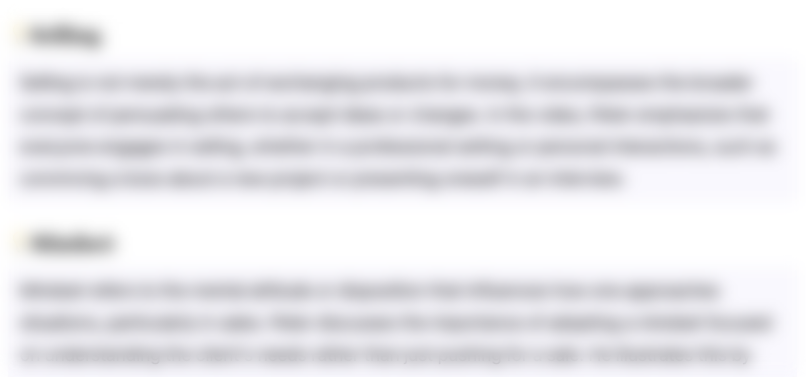
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
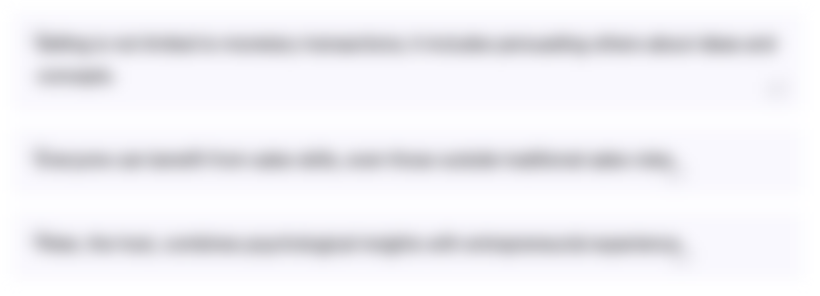
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
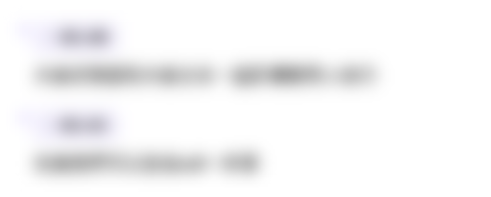
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)