Getting User Input | Java | Tutorial 9
Summary
TLDRIn this Java tutorial, you'll learn how to take user input using the `Scanner` class. The video covers creating and using a `Scanner` object to prompt the user for information, like their name and age. It demonstrates how to handle different types of inputs, such as strings and integers, and explains the key methods like `nextLine()` and `nextInt()`. By the end, you'll be able to build interactive Java programs that respond to user input, making your programs more dynamic and engaging.
Takeaways
- 😀 You can allow users to interact with your program by getting input from them in Java.
- 😀 To get user input, you need to create a `Scanner` object in Java.
- 😀 The `Scanner` object is initialized with `System.in` to read input from the keyboard.
- 😀 Always import the `Scanner` class with `import java.util.Scanner;` to use it in your program.
- 😀 Use `System.out.print()` to display prompts without adding a newline after the message.
- 😀 The method `keyboardInput.nextLine()` is used to capture a line of text input from the user.
- 😀 For numerical input, use `keyboardInput.nextInt()` to capture an integer.
- 😀 Store the captured input in variables such as `String name` or `int age` to use them later in the program.
- 😀 You can concatenate the user's input with other strings to create dynamic output messages.
- 😀 Using `System.out.println()` will print the result and move to the next line for clean output formatting.
- 😀 Input functions like `nextLine()` and `nextInt()` are used based on the type of data expected from the user (string or integer).
Q & A
What is the purpose of using a scanner in Java?
-A scanner is used in Java to allow users to input information into a program. It helps in capturing user input from the console and storing it for further processing.
How do you create a scanner in Java?
-To create a scanner in Java, you declare it with the type 'Scanner' and then initialize it with 'new Scanner(System.in)'. This creates a scanner that can read input from the keyboard.
What does the 'import java.util.Scanner' statement do?
-The 'import java.util.Scanner' statement imports the Scanner class into the program, allowing you to use its functionality to read input from the user.
What is the difference between 'System.out.print' and 'System.out.println'?
-'System.out.print' displays text without moving to a new line, while 'System.out.println' prints text and moves the cursor to the next line, adding a line break after the text.
How does the 'keyboardInput.nextLine()' method work?
-'keyboardInput.nextLine()' waits for the user to input a line of text, and it captures the entire input and stores it as a string.
What is the role of the variable 'name' in this script?
-The 'name' variable stores the text input by the user, representing their name, which can be accessed later in the program.
How do you display the input value stored in a variable?
-You can display the value stored in a variable by printing it out using 'System.out.print' or 'System.out.println', appending the variable name within the print statement.
Why does the script use 'keyboardInput.nextInt()' when asking for age?
-The 'keyboardInput.nextInt()' method is used to capture integer input from the user, specifically when asking for numerical input, like the user's age.
What would happen if you used 'nextLine()' instead of 'nextInt()' for the age input?
-If 'nextLine()' was used instead of 'nextInt()', the program would expect a string input instead of an integer. This would lead to a mismatch if the user tries to input a number.
Can you modify the script to accept more inputs from the user?
-Yes, you can modify the script by adding more prompts and using methods like 'nextLine()', 'nextInt()', or other appropriate Scanner methods to capture different types of input from the user.
Outlines
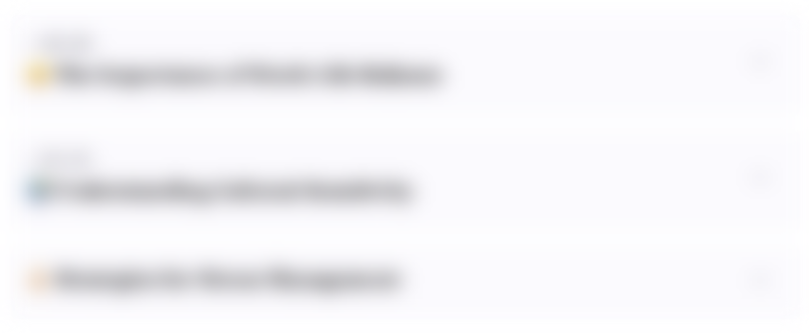
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
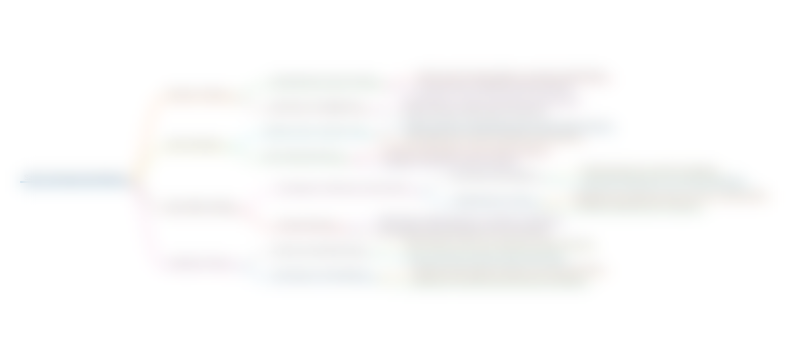
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
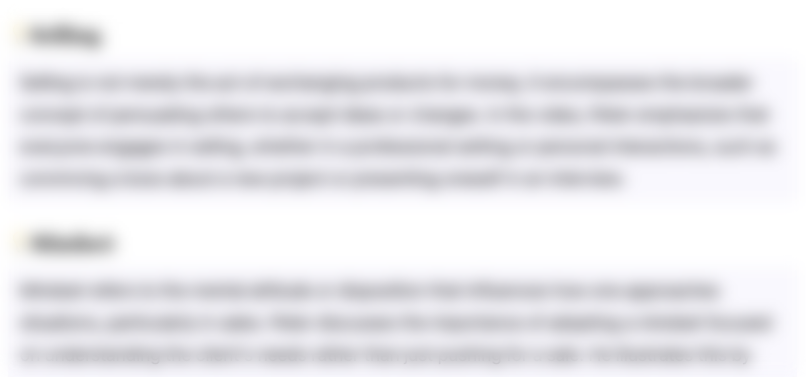
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
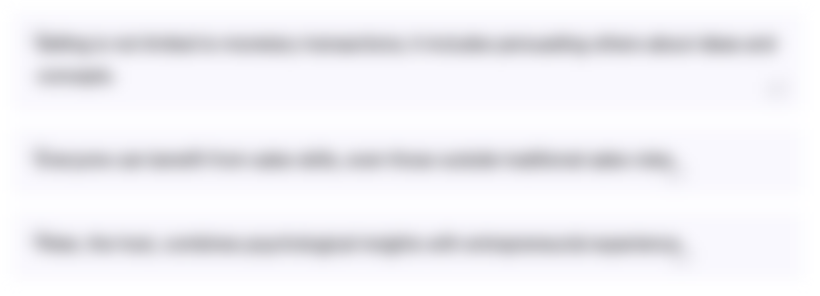
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
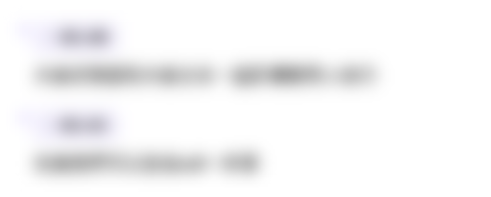
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
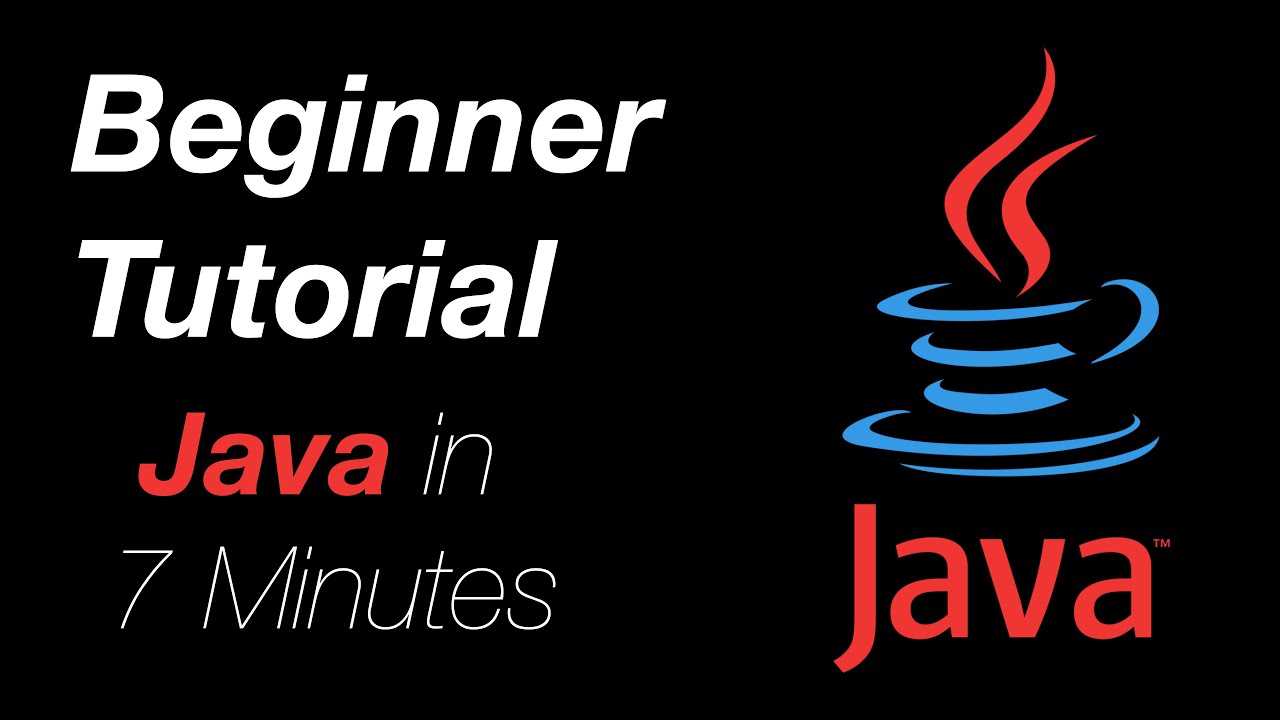
The Basics of Java in 7 Minutes, Quick And Easy Tutorial
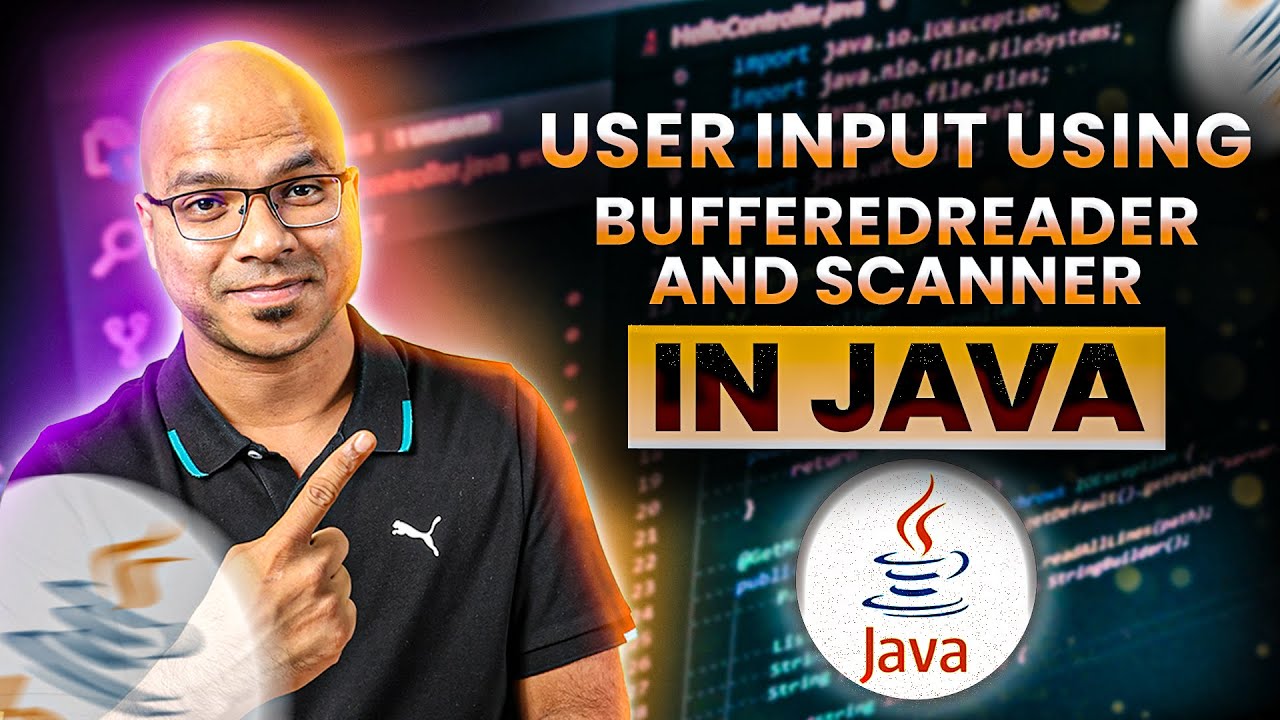
#83 User Input using BufferedReader and Scanner in Java
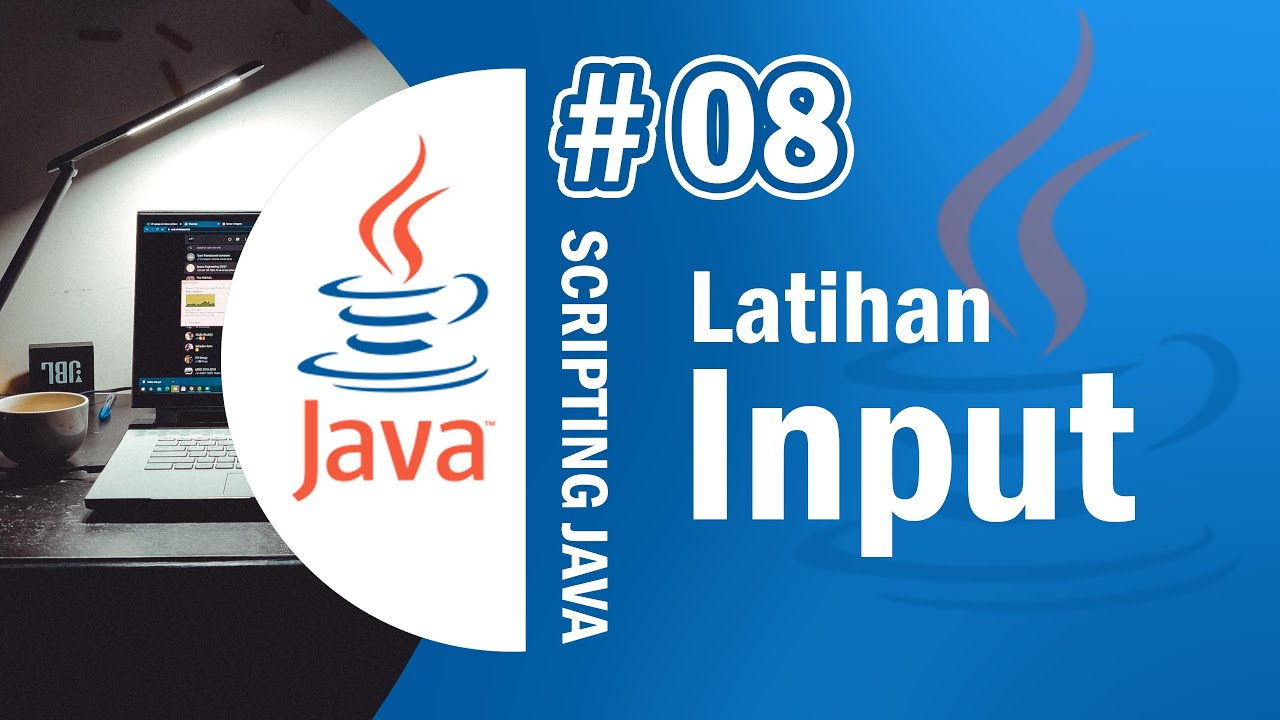
Java 08 - Latihan Input (Membuat Program Sederhana dengan Java) - Tutorial Java Netbeans Indonesia
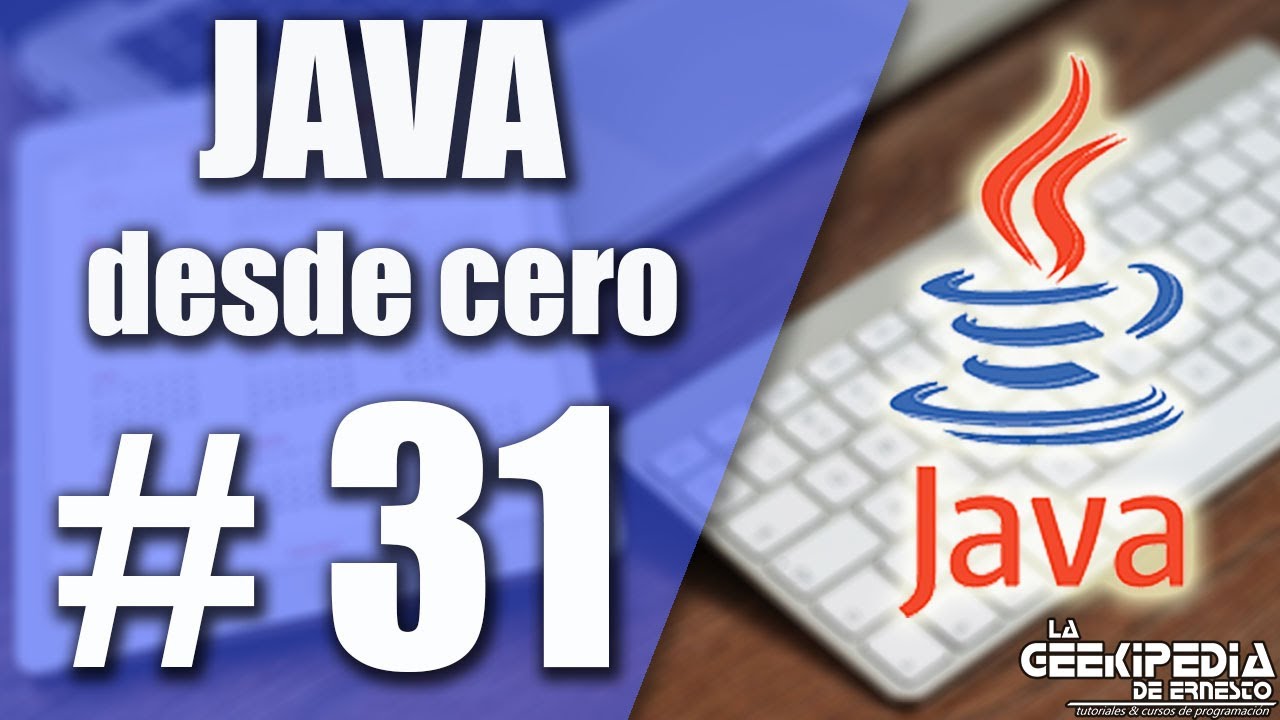
Curso Java desde cero #31 | Interfaces gráficas (Swing - JScrollPane)

Inputan

#4: Get User Input in C Programming
5.0 / 5 (0 votes)