G-28. Shortest Path in Undirected Graph with Unit Weights
Summary
TLDRIn this video, the problem of finding the shortest path in an undirected graph with unit edge weights is explained using a BFS algorithm. The process starts with defining the graph structure and initializing a distance array. The BFS algorithm is demonstrated step-by-step, where nodes are processed using a queue, and distances are updated accordingly. The explanation also covers how unreachable nodes are handled with a value of -1. A C++ implementation of the algorithm is shown, emphasizing the simplicity and efficiency of BFS for solving shortest path problems in unit-weighted graphs.
Takeaways
- ๐ The problem involves finding the shortest path in an undirected graph with unit distance edges.
- ๐ A breadth-first search (BFS) algorithm is applied to find the shortest path from a given source to all other nodes.
- ๐ The graph is represented using an adjacency list, and the source node is provided to begin the search.
- ๐ The BFS queue stores pairs of nodes and their corresponding distances from the source.
- ๐ Initially, the distance array is filled with infinity, and the distance to the source node is set to zero.
- ๐ The BFS algorithm explores each node, updates the distance array, and adds adjacent nodes with updated distances to the queue.
- ๐ The BFS guarantees that nodes are processed in the order of their shortest distance from the source.
- ๐ If a node is already reached with a shorter distance, further exploration for that node is skipped.
- ๐ If a node is unreachable from the source, it remains assigned as infinity, and a value of -1 is returned for that node.
- ๐ The BFS approach ensures that nodes are processed layer by layer, and the shortest distances are updated efficiently.
- ๐ The space and time complexity of this BFS approach is O(V + E), where V is the number of vertices and E is the number of edges in the graph.
Q & A
What is the main objective of the problem described in the video?
-The main objective is to find the shortest distance from a given source node to all other nodes in an undirected graph with unit edge weights. If a node is unreachable, return -1.
How is the graph represented in the solution?
-The graph is represented using an adjacency list, where each node has a list of adjacent nodes (its neighbors).
What is the significance of the queue in the BFS algorithm used in the solution?
-The queue is used to store nodes along with their current distance from the source. The BFS algorithm processes nodes layer by layer, ensuring that the shortest path is found for each node.
Why does the distance array need to be initialized with infinity?
-The distance array is initialized with infinity to signify that no node has been visited yet. Only when a node is reached through BFS will its distance be updated.
What happens when a node is already visited with a shorter distance?
-If a node is already visited with a shorter distance, it is not reprocessed. This ensures that the BFS algorithm doesn't explore paths that would result in longer distances.
How does the algorithm determine if a node is reachable from the source?
-A node is considered reachable if its distance in the distance array is updated from infinity to a finite value. If it remains at infinity, the node is unreachable.
What is the time complexity of the BFS algorithm used in the solution?
-The time complexity of the BFS algorithm is O(V + E), where V is the number of vertices and E is the number of edges in the graph.
Why is the BFS algorithm used for this problem instead of Dijkstra's algorithm?
-BFS is chosen because all edges have unit weights, meaning the shortest path is simply determined by the number of edges traversed. Dijkstraโs algorithm would be overkill for this scenario, as it is designed for weighted edges.
What happens if a node is unreachable in the graph?
-If a node is unreachable, its distance value remains as infinity, and in the final output, it is converted to -1 to indicate that the node cannot be reached from the source.
How does the queue maintain the order of nodes in BFS?
-The queue maintains the order of nodes by processing them in the order they are added. As BFS explores each node's neighbors, it ensures that nodes at increasing distances from the source are processed in sequence.
Outlines
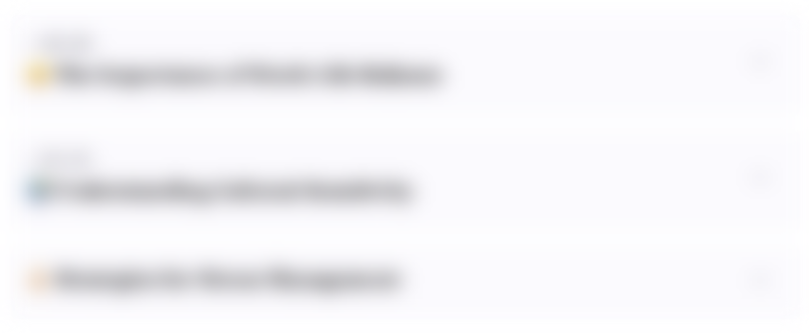
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
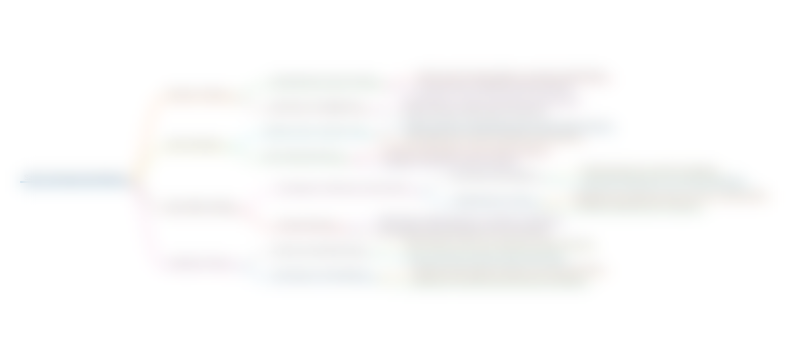
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
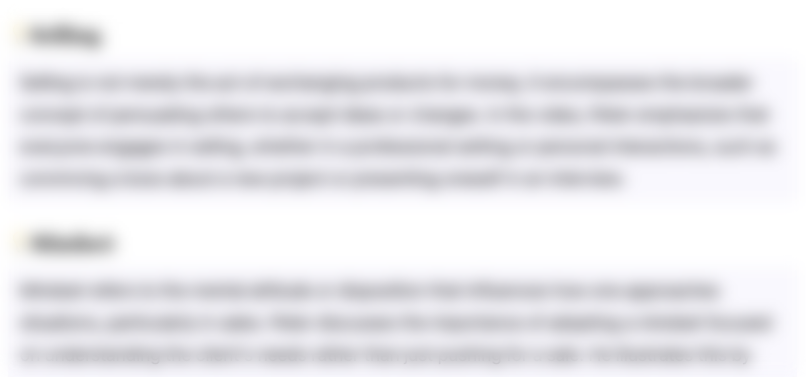
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
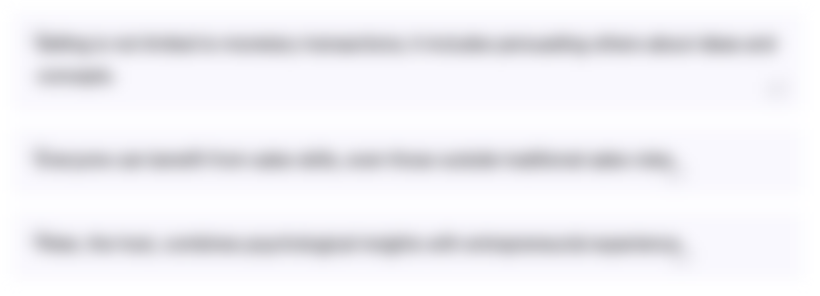
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
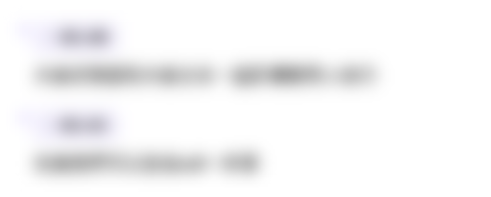
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)