نفس الكود، ولكن 7 مستويات! و7 طرق للتفكير🧠
Summary
TLDRThe video script details a journey through seven levels of coding to solve a specific problem: identifying even numbers within a given range and returning them as a list or array. The first level uses a simple loop to iterate through numbers, checking for evenness by the modulus operator. Subsequent levels introduce various optimizations, such as binary system approximation, list comprehension, and filtering with the 'filter' function. The fifth level explores recursion as a method to determine even numbers. The sixth level leverages numpy arrays and filtering for efficiency. The seventh and final level simplifies the process by using a range function with a step of two, adjusting for odd starting numbers. Each level is tested for performance, with the seventh level demonstrating the most significant efficiency with a time complexity vastly improved over the initial approach. The script emphasizes the importance of thinking critically about solutions rather than relying solely on complex code or advanced techniques.
Takeaways
- 📈 The video demonstrates seven levels of coding to solve the problem of finding even numbers within a specified range.
- 🔍 The first level uses a simple loop to iterate through numbers, checking for evenness by dividing by two and using the modulus operation.
- ⏱️ Performance measurements show that the initial code takes 3.22 seconds to execute, which is considered slow for the task.
- 🧮 In the second level, binary system concepts are applied to check for even numbers by looking at the rightmost bit, which is faster than the first method.
- 🚀 The third level optimizes the code by replacing the loop with list comprehension, reducing the execution time to 2.48 seconds.
- 🔬 The fourth level introduces the use of Python's built-in `filter` function, which significantly improves performance, taking only 1.59 microseconds.
- 🛠 The fifth level explores recursion as a method to determine even numbers, although it is not efficient for this specific problem, taking over two hours to run.
- 📚 The sixth level uses numpy arrays and the `numpy.arange` function with a filter to find even numbers, achieving a performance of 366 milliseconds.
- 💡 The seventh and final level simplifies the process by using a range function with a step of two, bypassing the need for checking each number individually, and achieves an execution time of 38 milliseconds.
- 🤔 The video emphasizes the importance of thinking through solutions rather than just writing code, as more advanced methods are not always the most effective.
- 🌟 Using built-in functions and libraries, such as numpy, can lead to more efficient and cleaner code, as demonstrated by the significant performance improvements in the higher levels.
Q & A
What is the primary goal of the code discussed in the script?
-The primary goal of the code is to search for even numbers within a specified range and return a list or array of these numbers.
How does the first level of thinking approach the problem?
-The first level of thinking approaches the problem by iterating through numbers in a given range, checking if each number is even by dividing it by two and checking the remainder. If the remainder is zero, the number is added to a list.
What is the significance of using binary system approximation in the second level of thinking?
-The significance of using binary system approximation is that it allows for a more efficient check of even numbers by observing the rightmost bit of a binary number. Even numbers always end with a zero bit in binary representation.
How does the third level of thinking improve the performance of the code?
-The third level of thinking improves the performance by replacing the loop in the function with a list comprehension, which is a more efficient way to create lists in Python.
What is the main advantage of using the filter function in the fourth level of thinking?
-The main advantage of using the filter function is that it allows for a concise and efficient way to filter out even numbers from a range without the need for an explicit loop or conditional statements.
Why is the recursive approach in the fifth level of thinking not suitable for this problem?
-The recursive approach is not suitable for this problem because it involves repeatedly subtracting two from a number until it reaches zero, which does not scale well for large ranges and can lead to a high time complexity.
What is the key concept used in the sixth level of thinking to optimize the code?
-The key concept used in the sixth level of thinking is the use of NumPy arrays and the NumPy library's built-in functions, which are highly optimized for performance and can handle large datasets efficiently.
How does the seventh level of thinking simplify the problem and improve the efficiency of the code?
-The seventh level of thinking simplifies the problem by recognizing that even numbers can be generated in a sequence by adding two to the previous even number. This allows the use of a simple range function with a step of two, avoiding the need for complex checks or loops.
What is the importance of considering the starting number's parity when implementing the range in the seventh level of thinking?
-Considering the starting number's parity is important because if the starting number is odd, the range would initially generate odd numbers. By adding one to an odd starting number, it becomes even, and the range can then correctly generate even numbers in steps of two.
Why is the performance of the code in the seventh level of thinking significantly better than the previous levels?
-The performance is significantly better because the approach is highly optimized and takes advantage of Python's built-in range function with a step of two, which is a very efficient way to generate a sequence of even numbers without the need for additional checks or operations.
What is the main takeaway from the script regarding the relationship between code complexity and problem-solving efficiency?
-The main takeaway is that the most efficient solutions are not always the most complex ones. Simplifying the problem and using the right approach or tools can lead to more effective and faster solutions, emphasizing the importance of thinking and problem-solving skills in programming.
How does the script demonstrate the importance of understanding different levels of thinking in programming?
-The script demonstrates this by progressively showing how different approaches, from simple loops to advanced techniques like list comprehensions, filter functions, and NumPy arrays, can be used to solve the same problem with varying levels of efficiency, highlighting the need for a deep understanding of programming concepts.
Outlines
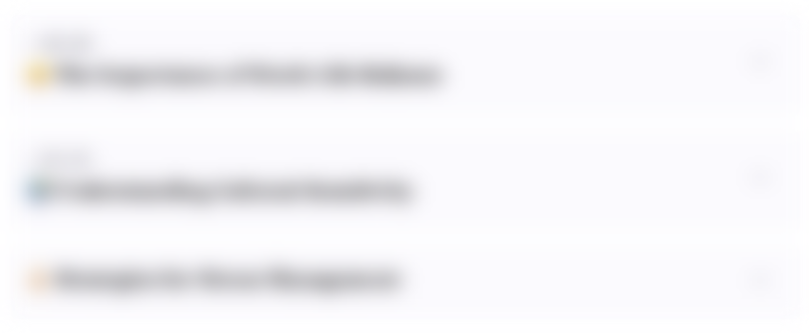
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
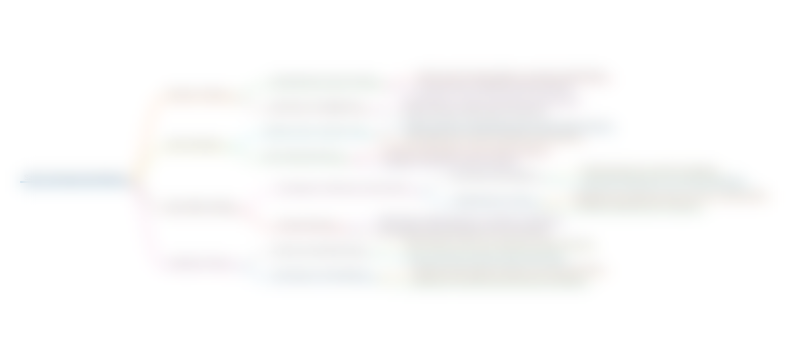
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
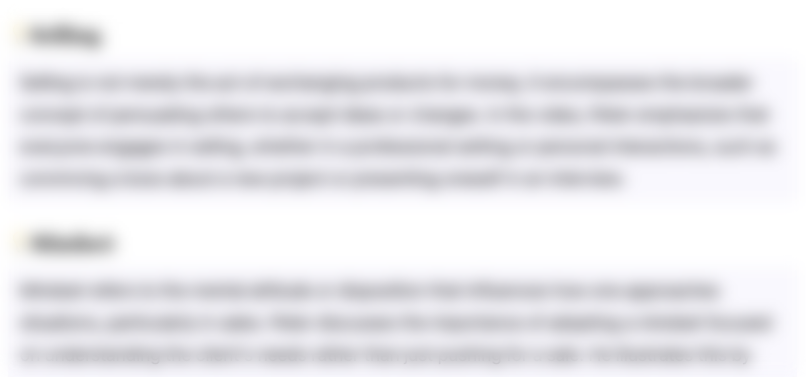
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
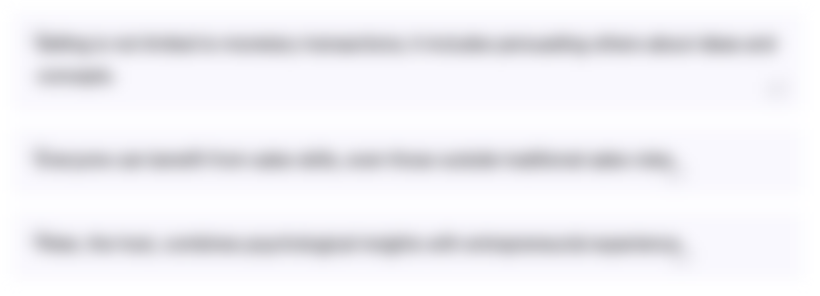
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
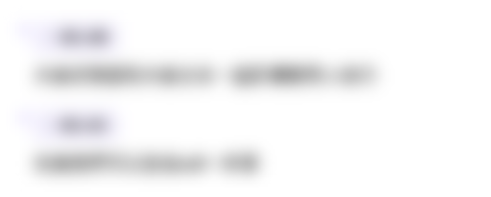
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
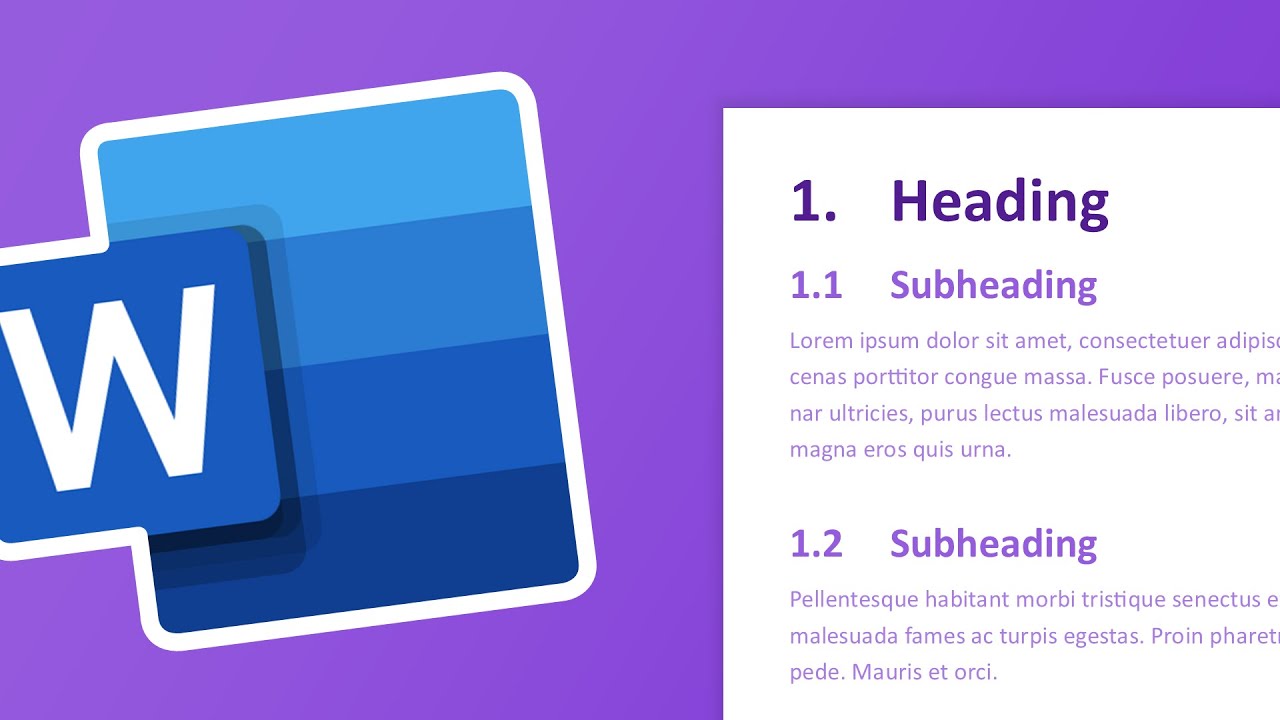
Numbered Headings and Subheadings | Microsoft Word Tutorial
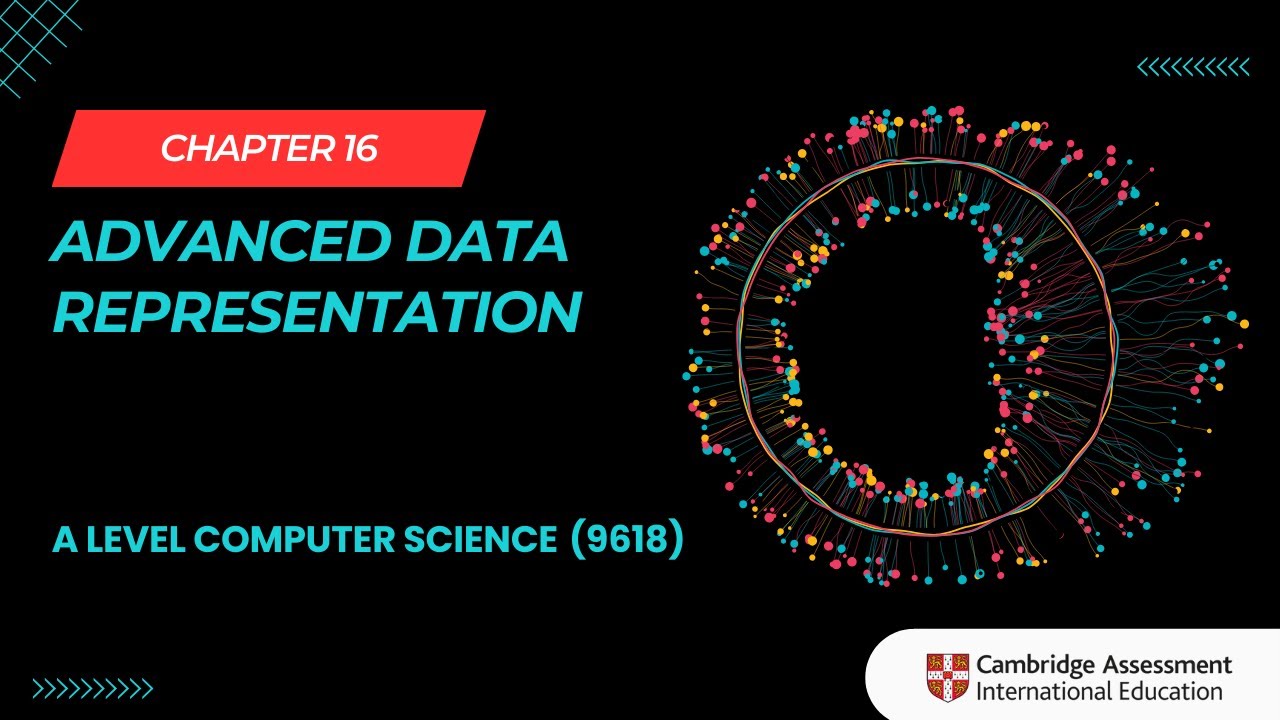
AS & A Level Computer Science (9618) - Chapter 16: ADVANCED Data Representation
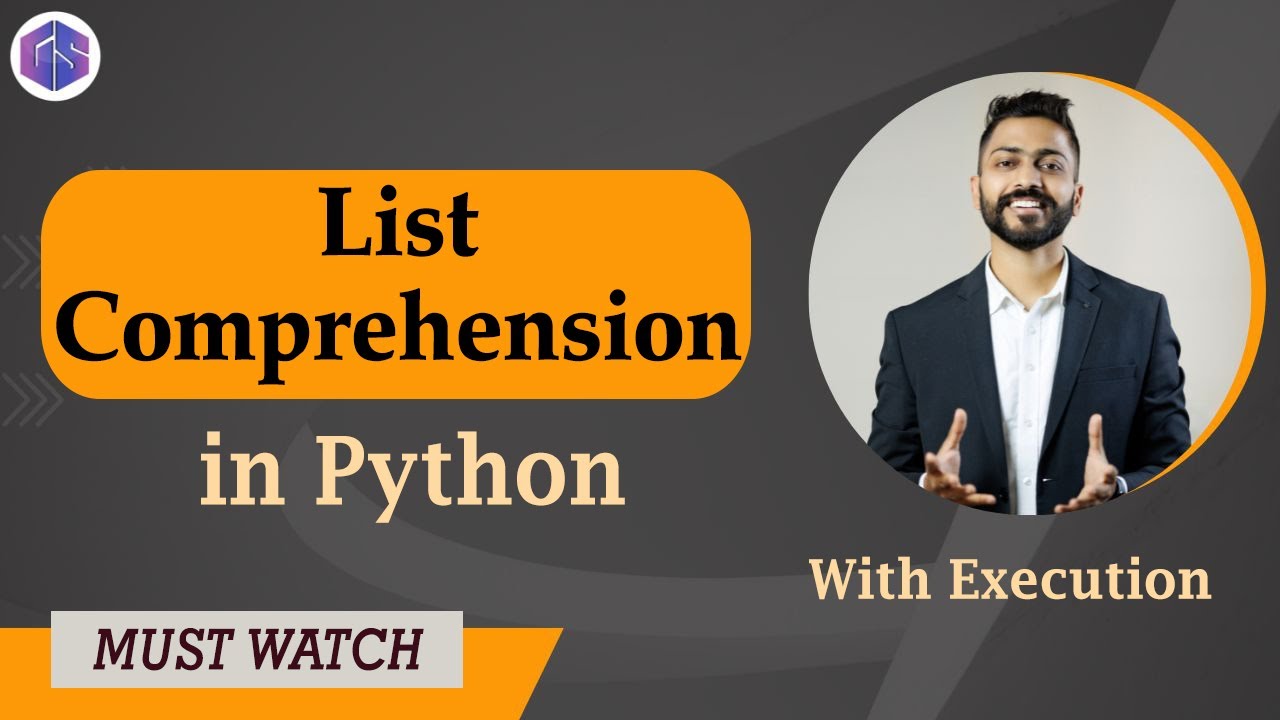
List Comprehension in Python 🐍 with Execution | Best Python Tutorials for Beginners
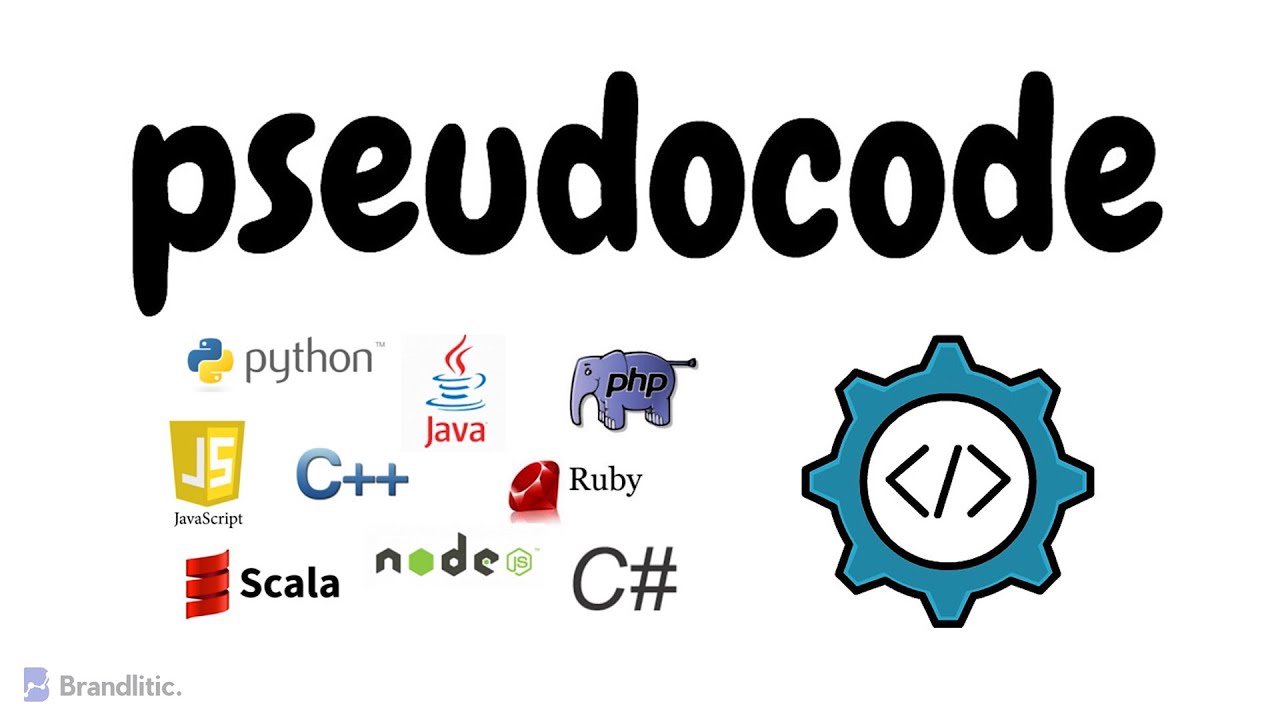
What is Pseudocode Explained | How to Write Pseudocode Algorithm | Examples, Benefits & Steps
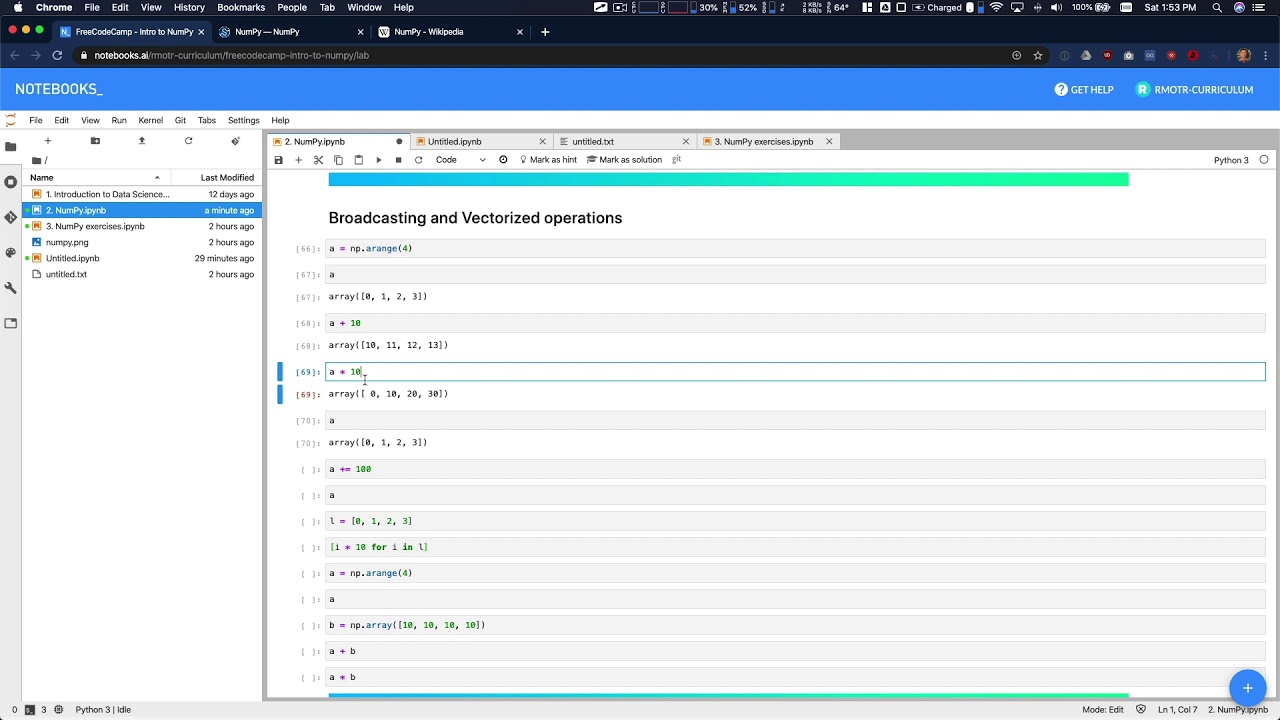
Numpy Operations - Data Analysis with Python Course
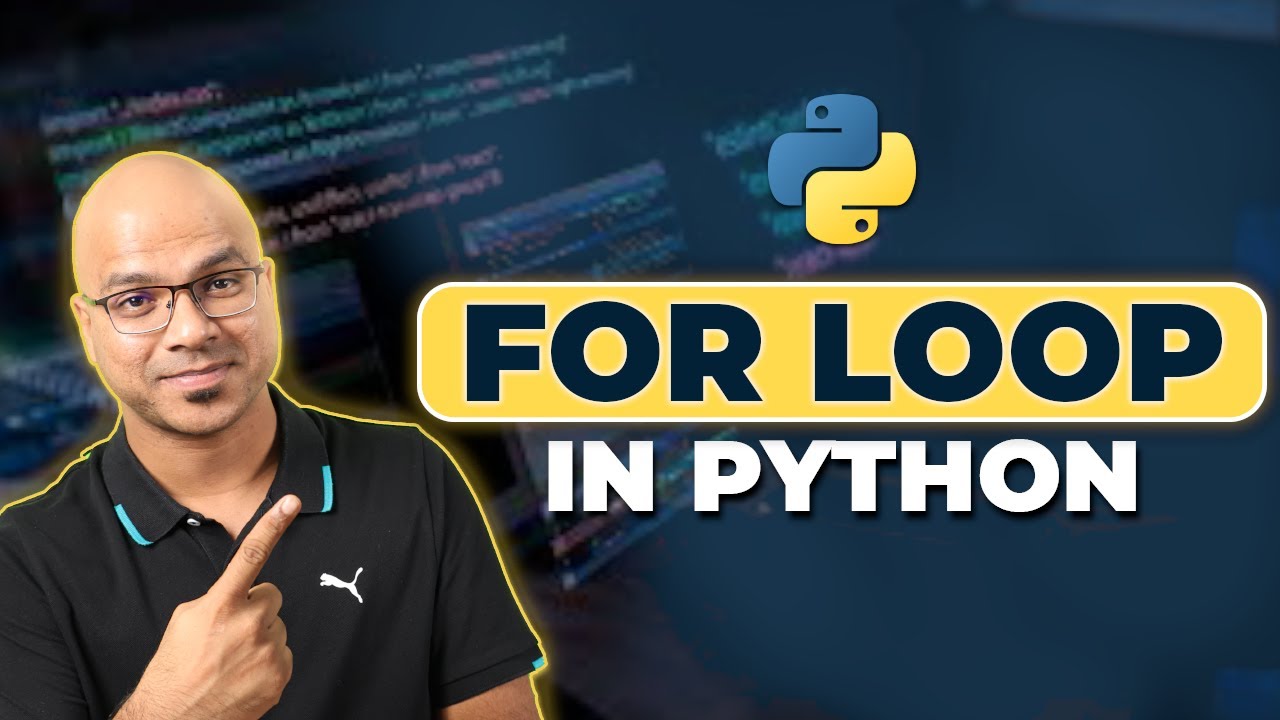
#21 Python Tutorial for Beginners | For Loop in Python
5.0 / 5 (0 votes)