Namespaces in C++
Summary
TLDRThis lecture introduces namespaces in C++, explaining their role in providing scope to identifiers like functions and variables. It highlights the importance of namespaces in organizing code and preventing naming conflicts, particularly when multiple libraries are involved. The 'std' namespace is discussed, demonstrating how to use 'cout' and 'cin' for input and output. The lecture covers 'using' declarations to simplify code and the 'using namespace std' directive, which allows the use of all standard identifiers without explicit qualification. Overall, the session aims to clarify the concept and practical applications of namespaces in C++ programming.
Takeaways
- 😀 A namespace is a declarative region that provides scope to identifiers like types, functions, and variables.
- 😀 Namespaces help organize code into logical groups and prevent name collisions, especially when multiple libraries are used.
- 😀 The standard namespace, indicated as 'std', contains common identifiers like 'cout' and 'cin' used for output and input in C++.
- 😀 The scope operator (::) is used to specify that an identifier belongs to a particular namespace, e.g., 'std::cout'.
- 😀 Using declarations allow you to avoid repeatedly typing the namespace prefix by using 'using namespace std;' or specific identifiers like 'using std::cin;'.
- 😀 If you don't specify the namespace for an identifier like 'cin', it will result in an error, indicating that it's not declared in the current scope.
- 😀 Using 'using namespace std;' allows you to access all identifiers from the standard namespace directly, simplifying code.
- 😀 While 'using namespace std;' makes code cleaner, it can lead to name collisions if other libraries contain similar identifiers.
- 😀 An example program demonstrates how to take user input and display it using 'cout' and 'cin', showcasing the practical use of namespaces.
- 😀 Understanding namespaces in C++ is crucial for effectively managing the scope of identifiers and maintaining clear, conflict-free code.
Q & A
What is a namespace in C++?
-A namespace is a declarative region that provides a scope to identifiers, which can include names of types, functions, and variables.
Why are namespaces used in programming?
-Namespaces are used to organize code into logical groups and to prevent name collisions, especially when using multiple libraries.
What does 'std' stand for?
-'std' stands for the standard library in C++, indicating that identifiers like 'cout' and 'cin' are defined within this library.
How does the scope operator work in C++?
-The scope operator (::) is used to specify that a name, such as 'cout' or 'cin', belongs to a particular namespace, helping to avoid ambiguity.
What is a 'using' declaration in C++?
-A 'using' declaration allows you to use a name from a namespace without qualifying it with the namespace prefix, simplifying the code.
Can you give an example of a simple program using namespaces?
-Certainly! A simple program could look like this: ```cpp #include <iostream> using namespace std; int main() { int n1; cout << 'Enter a number: '; cin >> n1; cout << 'The entered number is ' << n1 << endl; return 0; } ```
What happens if you don't specify the namespace for 'cin'?
-If you don't specify the namespace for 'cin' and it is not defined in the current scope, the compiler will throw an error stating that 'cin' was not declared in this scope.
What is the benefit of using 'using namespace std;'?
-Using 'using namespace std;' allows you to use all names in the 'std' namespace without needing to prefix them with 'std::', making the code cleaner and more readable.
What is the potential downside of using 'using namespace std;'?
-The downside is that it may lead to name collisions if there are identifiers in other libraries with the same name as those in the 'std' namespace.
How can you avoid name collisions when using multiple libraries?
-To avoid name collisions, it's best to either specify the namespace for each identifier explicitly or to use 'using' declarations for specific identifiers instead of 'using namespace' for the entire library.
Outlines
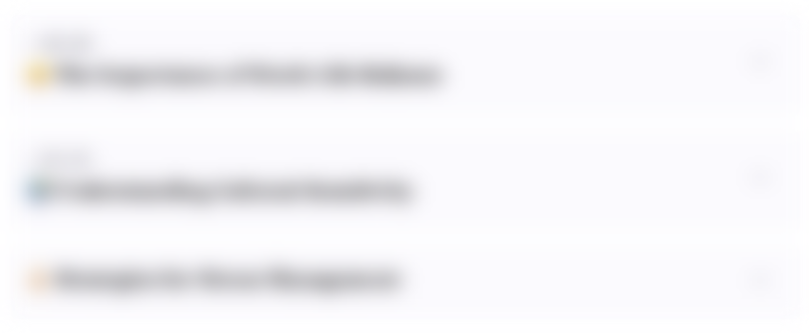
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
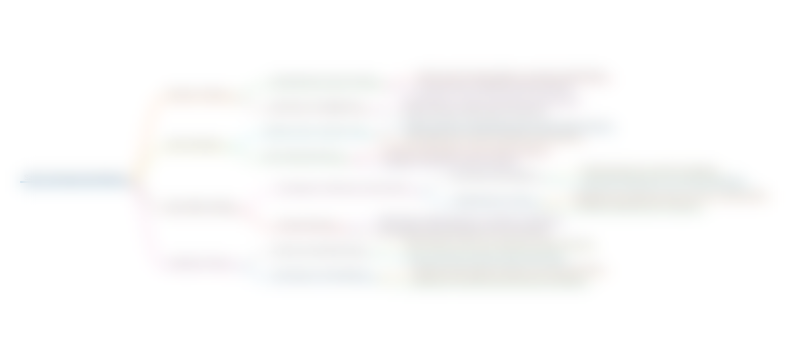
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
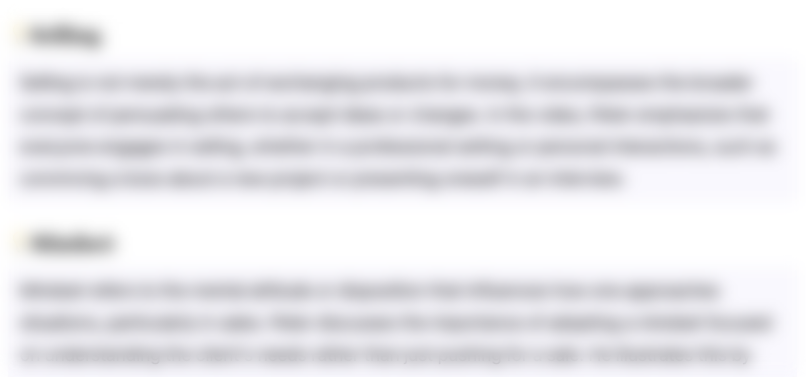
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
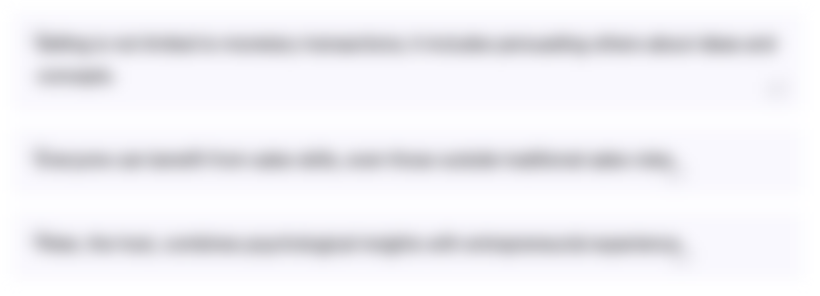
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
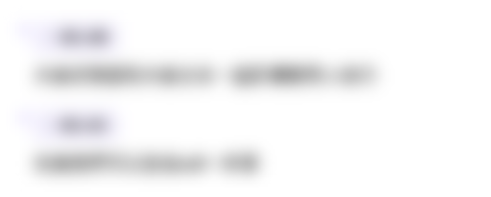
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
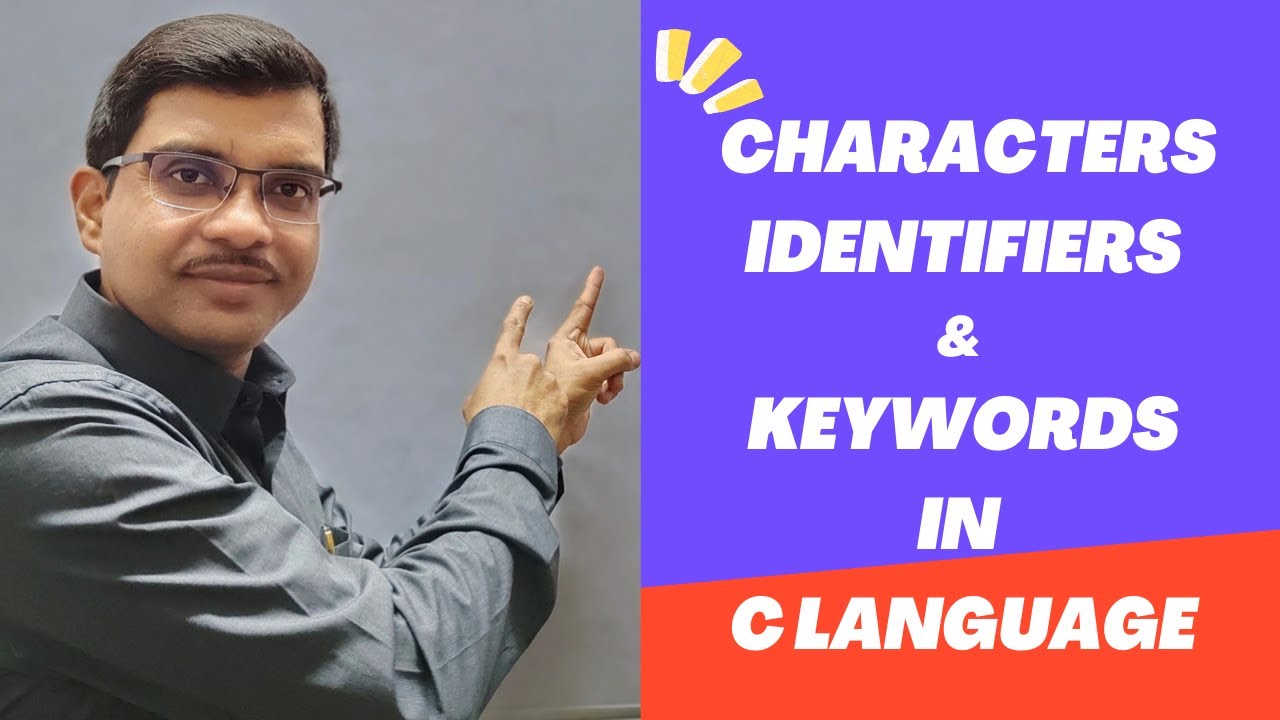
C_08 Characters | Identifiers & Keywords in C Language | C Programming Tutorials
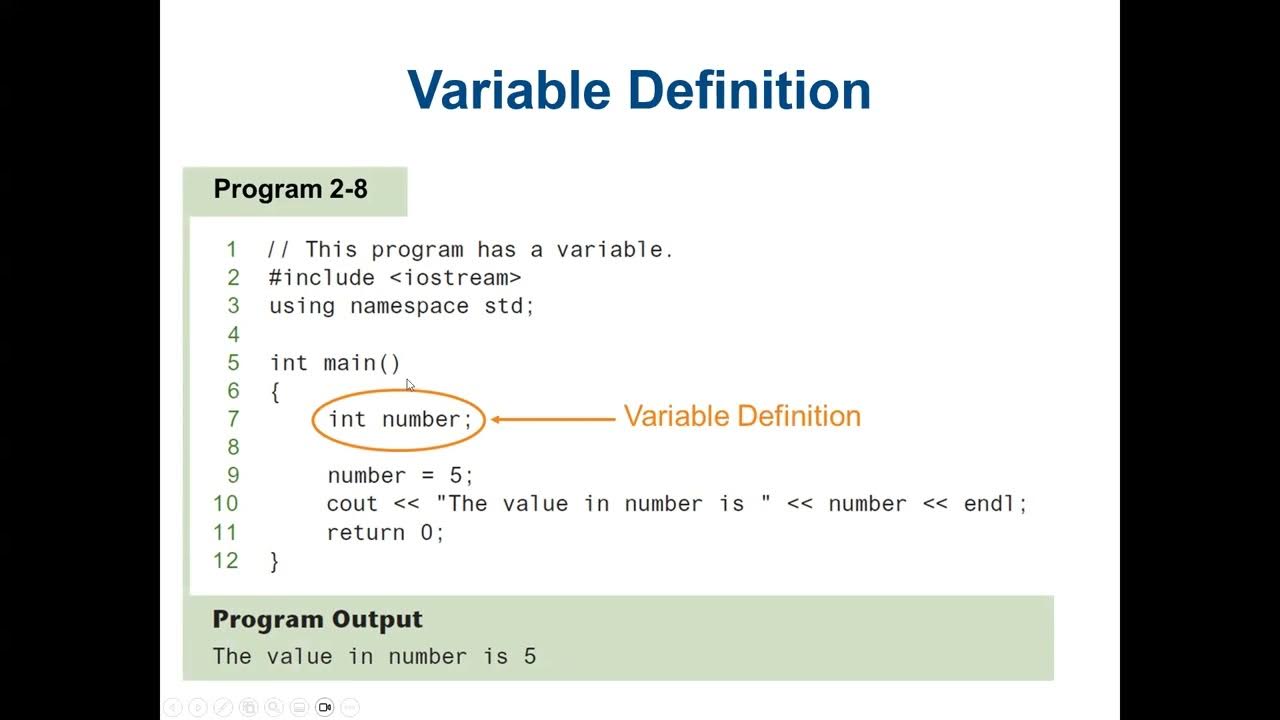
Introduction to C++, The Parts of a C++ Program, Identifier Naming Rules, output statements
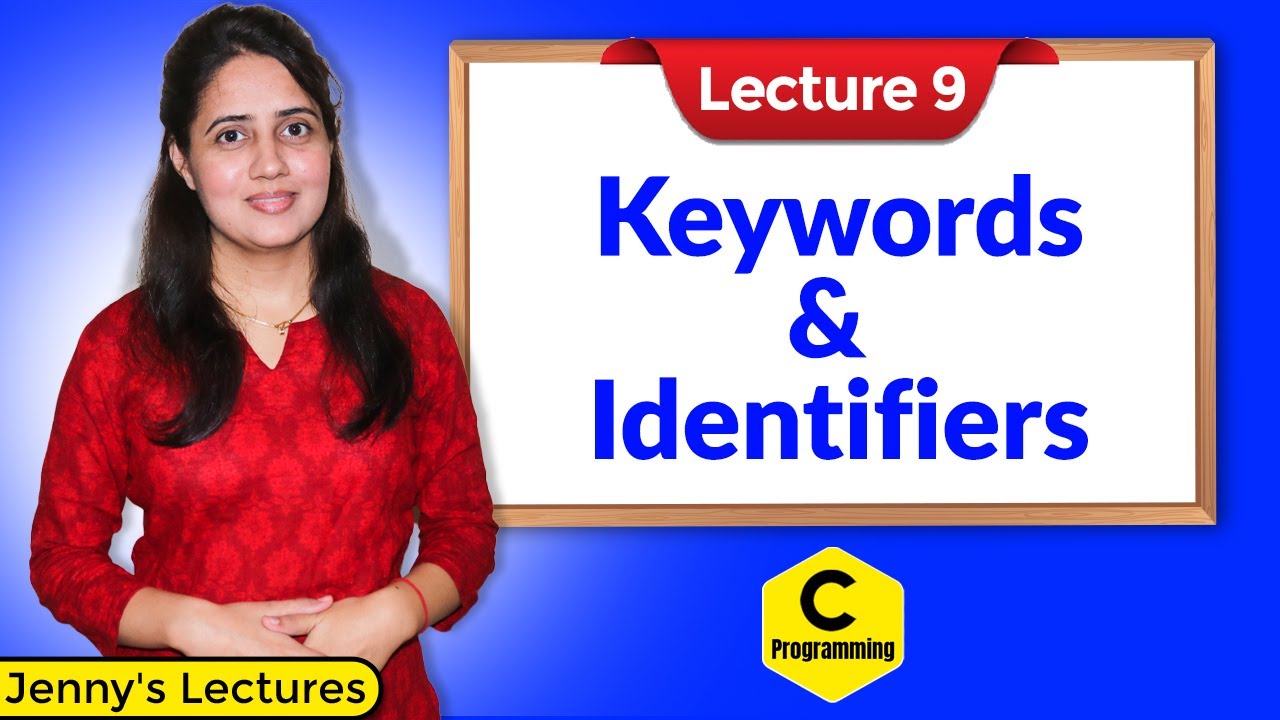
C_09 Keywords and Identifiers | Programming in C

COS 333: Chapter 11, Part 2

#17 C Standard Library Functions | C Programming For Beginners

COS 333: Chapter 5, Part 2
5.0 / 5 (0 votes)