Understanding memo | Lecture 242 | React.JS 🔥
Summary
TLDRThe video script introduces the concept of memoization in React, an optimization technique that stores the results of a pure function after the first execution, using the same inputs to retrieve the cached result instead of re-executing the function. This approach is applied to React components through the `memo` function, which prevents unnecessary re-renders when a component's props remain unchanged. The script also discusses the use of `useMemo` and `useCallback` hooks for optimizing objects and functions. However, memoization is only beneficial for heavy components that render frequently with the same props, and it does not prevent re-renders due to state changes or context updates. The lecture concludes by advising caution in overusing `memo`, as it is not always necessary and can be counterproductive for lightweight or infrequently rendered components.
Takeaways
- 📚 **Memoization Concept**: Memoization is an optimization technique that stores the results of a pure function after it's executed once with a set of inputs, avoiding re-computation with the same inputs.
- 🔄 **React's Memo Function**: In React, the `memo` function is used to prevent unnecessary re-renders of components when their props have not changed.
- 🚫 **No Re-render with Same Props**: A component memoized with `memo` will not re-render if the props remain the same as in the previous render.
- ⚠️ **State and Context Changes**: Memoization only affects props; a component will still re-render if its state changes or if there's a change in the context it subscribes to.
- 🔍 **Optimization for Heavy Components**: The `memo` function is particularly useful for heavy components that are resource-intensive to render.
- 🚦 **Frequency of Renders**: For memoization to be beneficial, the component should render frequently with the same props.
- 🔧 **No Need for Light Components**: Memoization does not provide benefits for lightweight or fast-rendering components.
- 📈 **Performance Considerations**: Memoization is most effective when the component re-renders too often or is slow to render, causing visible lag or delay.
- 🧐 **Understanding Memoization**: Knowing when and why to use memoization is crucial for optimizing React applications without causing unnecessary renders.
- 🏗️ **Use Memo and Use Callback Hooks**: In addition to the `memo` function, React provides `useMemo` and `useCallback` hooks to memoize objects and functions, respectively.
- 🛠️ **Preventing Wasted Renders**: Proper use of memoization techniques can prevent wasted renders and improve the speed of React applications.
Q & A
What is the fundamental concept behind the React tools mentioned in the lecture?
-The fundamental concept is memoization, which is an optimization technique that executes a pure function once and stores the result in memory, avoiding re-execution with the same inputs.
How does memoization apply to React components?
-Memoization in React is used to prevent components from re-rendering when their parent components re-render, as long as the props remain unchanged, by using the `memo` function.
What is the purpose of using the `useMemo` and `useCallback` hooks in React?
-These hooks are used to memoize objects and functions, helping to prevent unnecessary re-renders and improve application performance.
Why should we not memoize all components in a React application?
-Memoization should only be applied to heavy components that are frequently rendered with the same props, as it does not provide benefits for lightweight or infrequently rendered components.
How does a memoized component behave when its props change?
-A memoized component will re-render to reflect the new data when its props change, as the optimization only applies when props remain the same.
What are the conditions for memoization to be beneficial in a React component?
-Memoization is beneficial when the component is heavy, rendering frequently with the same props, and when avoiding unnecessary re-renders would significantly improve performance.
What happens if a memoized component's state changes?
-Even if a component is memoized, it will still re-render if its own state changes or if there is a change in the context it is subscribed to, as these changes represent new data to be displayed.
What is the primary difference between a pure function and a non-pure function in the context of memoization?
-A pure function always produces the same output given the same inputs and has no side effects, making it suitable for memoization. A non-pure function may produce different outputs with the same inputs or have side effects, which makes memoization less effective or inappropriate.
Why is it important to consider the performance characteristics of a component before deciding to use memoization?
-Considering performance characteristics ensures that memoization is used effectively to optimize performance. Applying memoization to components that are already fast or lightweight does not yield significant benefits and could be unnecessary.
How does the `memo` function in React differ from the `useMemo` hook?
-The `memo` function is used to prevent unnecessary re-renders of components by memoizing the component itself based on props, while the `useMemo` hook is used to memoize the results of expensive calculations or objects within a component's render method.
What is the role of the `useCallback` hook in optimizing React applications?
-The `useCallback` hook is used to memoize callbacks or functions, ensuring that they are not recreated on every render unless their dependencies change, which can help optimize performance by reducing unnecessary function re-creations.
Can memoization be used to optimize server-side rendering in React?
-While memoization primarily focuses on client-side rendering optimizations by reducing unnecessary re-renders, its principles could be applied to optimize server-side rendering as well, especially if the same component or function is rendered multiple times with the same inputs.
Outlines
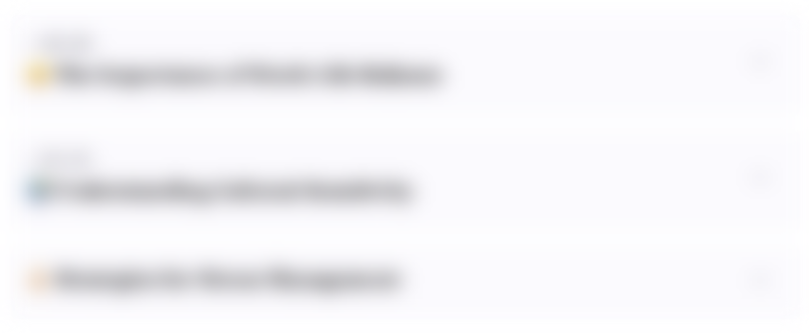
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
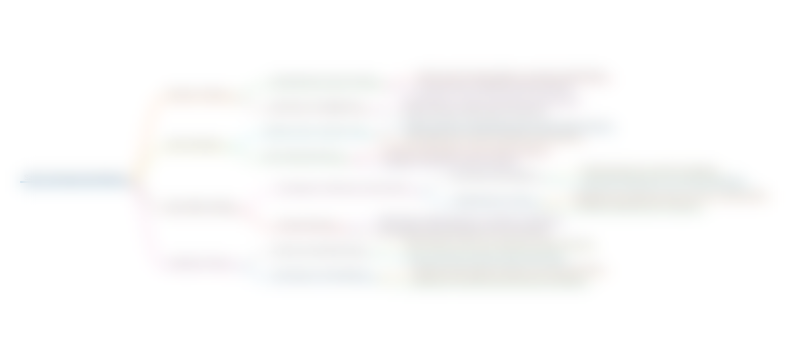
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
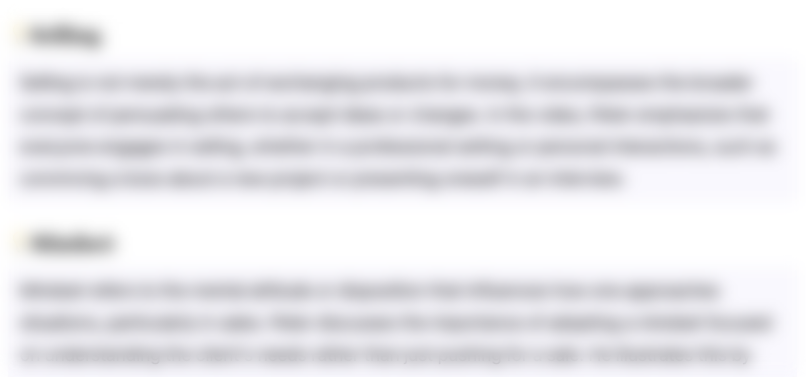
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
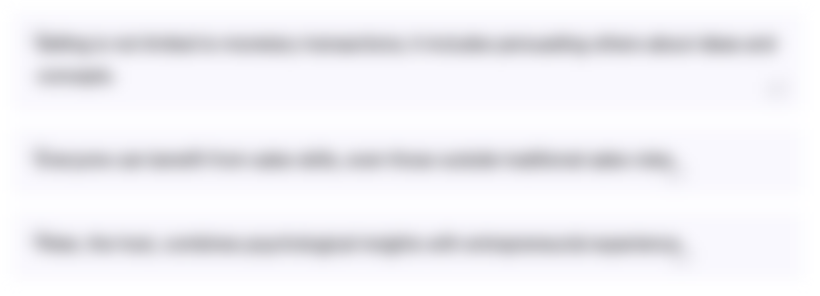
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
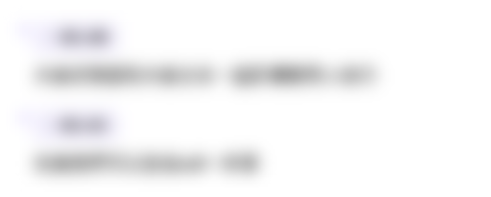
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Capítulo 2 - Funções de usuário: Como criar e ativar
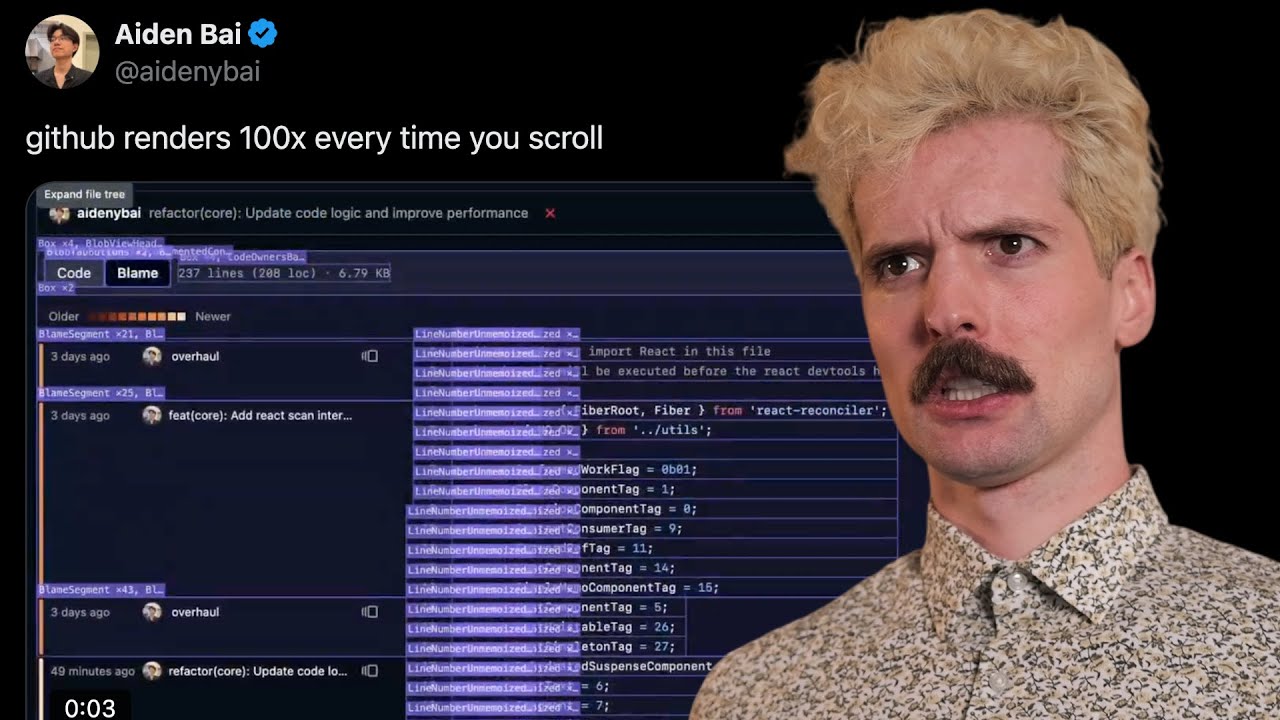
Why is every React site so slow?

Introduction To Optimization: Objective Functions and Decision Variables
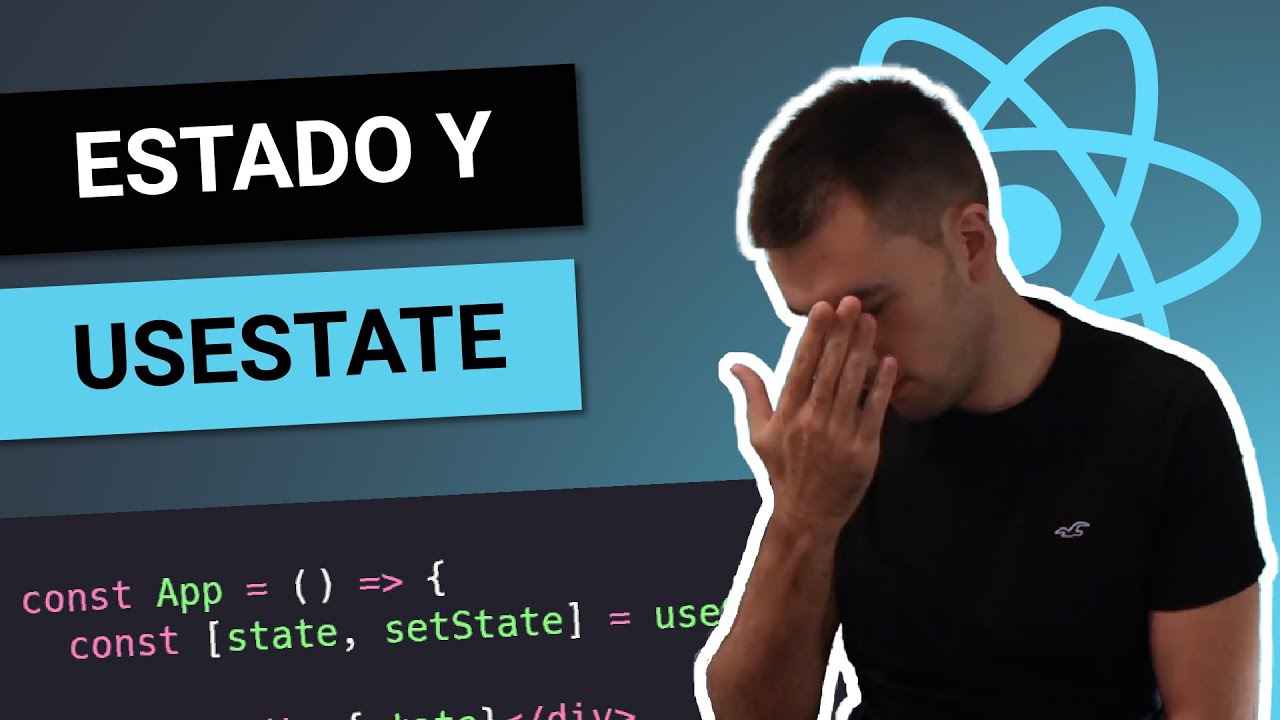
QUÉ es un ESTADO en REACT 🤔 CÓMO usar USESTATE 😎 Curso de React desde cero #9

UPDATED - Create Your First AWS Lambda Function | AWS Tutorial for Beginners

JavaScript - Throttle (節流) 常見的面試問題 (Scrollbar, Infinite Scroll) (前端優化)
5.0 / 5 (0 votes)